Implicit vs Explicit Type Declaration in Programming
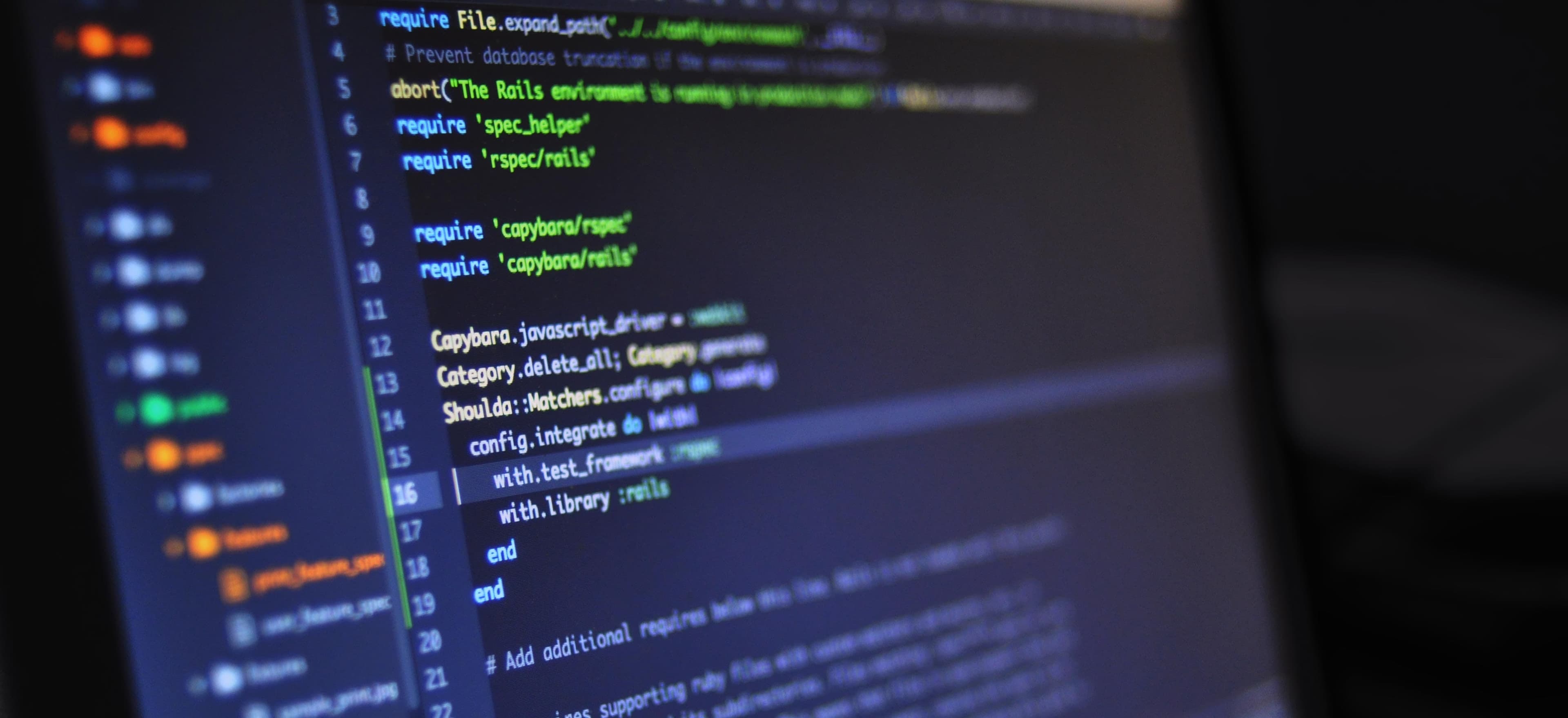
- Published on
Implicit vs Explicit Type Declaration in Java
When writing code in Java, developers have the option to declare variables with implicit or explicit type. While both approaches achieve the same result, they differ in readability, flexibility, and best practices. In this article, we will delve into the differences between implicit and explicit type declaration in Java and explore their impact on code quality and maintenance.
Implicit Type Declaration
In Java, implicit type declaration is achieved using the keyword var
. Introduced in Java 10, var
allows the compiler to infer the type of a variable based on the assigned value. This means that developers do not need to explicitly specify the type of the variable during declaration.
Benefits of Implicit Type Declaration
Using implicit type declaration can lead to cleaner and more concise code. It reduces redundancy by allowing the developer to focus on the intent of the code rather than the specific type of each variable. Additionally, it enables flexibility when dealing with complex data types, as the developer does not need to remember or manually specify intricate type names.
Example of Implicit Type Declaration
var message = "Hello, World!"; // Compiler infers type as String
var numbers = List.of(1, 2, 3, 4, 5); // Compiler infers type as List<Integer>
In the above example, the type of the variables message
and numbers
is implicitly inferred by the Java compiler based on the assigned values.
Explicit Type Declaration
Explicit type declaration, on the other hand, involves explicitly specifying the data type of a variable during its declaration. This is the traditional approach to declaring variables in Java and involves using the actual type name such as String
, int
, or user-defined classes/interfaces.
Benefits of Explicit Type Declaration
While explicit type declaration may appear more verbose, it enhances readability by providing direct insight into the data type of a variable. This can be particularly helpful in larger codebases or when collaborating with other developers. Furthermore, explicitly declaring the type can serve as documentation and improve the understanding of the code for future maintenance.
Example of Explicit Type Declaration
String message = "Hello, World!";
List<Integer> numbers = List.of(1, 2, 3, 4, 5);
In the above example, the type of the variables message
and numbers
is explicitly declared using the actual type names.
Best Practices and Considerations
When deciding between implicit and explicit type declaration, developers should consider readability, maintainability, and specific use cases. Both approaches have their own merits, and the choice between them often depends on the context and coding standards of the project or organization.
Best Practices for Implicit Type Declaration
- Use
var
when the assigned value makes the variable's type obvious. - Employ
var
for cases where the type name is repetitive or overly verbose. - Ensure that the inferred type through
var
is still clear and understandable.
Best Practices for Explicit Type Declaration
- Prefer explicit type declaration when the variable type may not be immediately obvious from the assigned value.
- Use explicit type declaration for public APIs and when enhancing code clarity is a priority.
- Stick to a consistent style guide regarding explicit type declaration within the codebase.
The Closing Argument
In conclusion, both implicit and explicit type declaration in Java offer distinct advantages and should be chosen judiciously based on the particular requirements of a project. While implicit type declaration can lead to more concise code, explicit type declaration enhances readability and maintainability. By understanding the nuances of each approach, developers can make informed decisions to write clear, efficient, and maintainable code.
In summary, the decision on whether to use implicit or explicit type declaration in Java should be driven by a balance of code clarity, maintainability, and adherence to best practices within the development environment.
By leveraging the appropriate type declaration approach, developers can streamline their codebase and contribute to a more robust and understandable code structure.
Remember, the choice between implicit and explicit type declaration is not a one-size-fits-all decision. It’s crucial to evaluate the context, consider the impact on readability and maintainability, and align with the standards and best practices of the project or organization.
For further information on type declaration in Java, you can refer to the official Oracle documentation.