Maximizing Regression Test Code Coverage Without Scripts
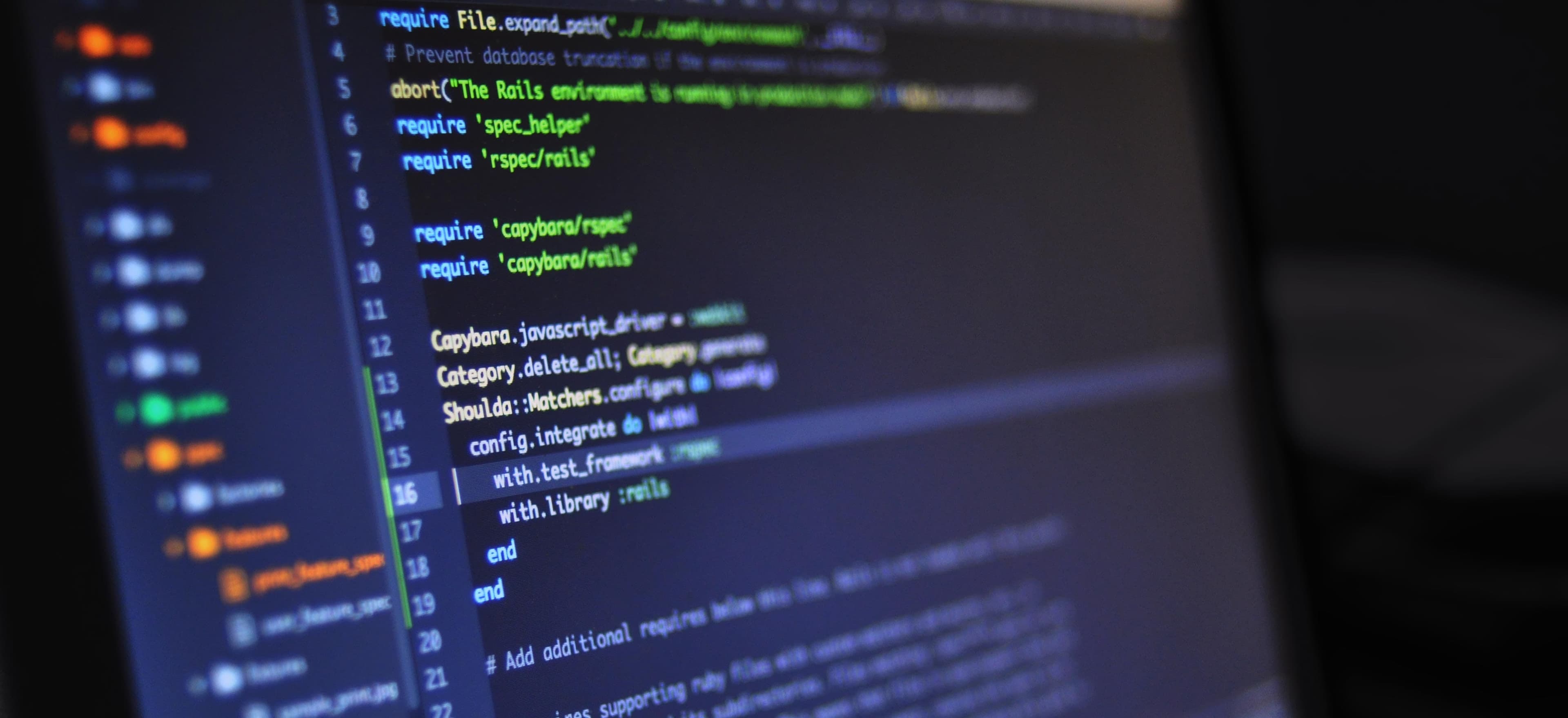
- Published on
Maximizing Regression Test Code Coverage Without Scripts
In the world of software development, the importance of regression testing cannot be overstated. It is the process of validating that recent changes to the codebase have not adversely affected existing functionalities. However, writing and maintaining test scripts for every possible scenario can be a daunting task, especially as the codebase grows. In this blog post, we'll explore how to maximize regression test code coverage without relying solely on scripts by leveraging Java and its powerful testing capabilities.
Code Coverage and Its Importance
Before diving into the techniques for maximizing regression test code coverage without scripts, let's first understand the concept of code coverage. Code coverage measures the degree to which the source code of a program has been tested. It helps developers identify areas of the codebase that are not adequately covered by tests. There are different types of code coverage, including statement coverage, branch coverage, and path coverage.
Leveraging JUnit for Parameterized Tests
JUnit, the popular Java testing framework, provides a powerful feature called parameterized tests. Parameterized tests allow you to run the same test logic with multiple inputs. This is particularly useful for maximizing test coverage without creating separate test cases for each input.
Here's an example of a parameterized test using JUnit:
import org.junit.Test;
import org.junit.runner.RunWith;
import org.junit.runners.Parameterized;
import java.util.Arrays;
import java.util.Collection;
@RunWith(Parameterized.class)
public class MathUtilParameterizedTest {
private int input;
private boolean expected;
public MathUtilParameterizedTest(int input, boolean expected) {
this.input = input;
this.expected = expected;
}
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][]{
{1, true},
{2, true},
{3, false},
{4, true}
});
}
@Test
public void testIsPrime() {
assertEquals(expected, MathUtil.isPrime(input));
}
}
In this example, we have a parameterized test for a method isPrime
in a MathUtil
class. The test runs with multiple inputs and their corresponding expected outcomes. By using parameterized tests, we can cover a wide range of input scenarios without duplicating test logic.
Embracing TestNG for Data-Driven Testing
TestNG is another popular testing framework for Java that offers support for data-driven testing. Data-driven testing is a technique that involves providing test data from external sources such as CSV files, Excel spreadsheets, or databases. This approach can be a game-changer when it comes to maximizing regression test code coverage without writing extensive scripts.
Let's take a look at a simple data-driven test using TestNG:
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
public class LoginTest {
@Test(dataProvider = "loginData")
public void testLogin(String username, String password) {
// Test logic for login with username and password
}
@DataProvider
public Object[][] loginData() {
return new Object[][]{
{"user1", "password1"},
{"user2", "password2"},
// More test data
};
}
}
In this example, we have a LoginTest
class with a data-driven test for the login functionality. The testLogin
method takes username and password as parameters, and the data is provided by the loginData
data provider method. By leveraging TestNG's data-driven testing capabilities, we can cover a wide range of login scenarios without hardcoding them into the test scripts.
Utilizing Code Coverage Tools
In addition to leveraging testing frameworks, using code coverage tools can provide insights into which parts of the code are exercised by the tests. Tools like JaCoCo and Emma can generate reports that show the percentage of code covered by tests. By analyzing these reports, developers can identify areas of the codebase that need additional test coverage.
For example, using JaCoCo with Maven:
<build>
<plugins>
<plugin>
<groupId>org.jacoco</groupId>
<artifactId>jacoco-maven-plugin</artifactId>
<version>0.8.7</version>
<executions>
<execution>
<id>prepare-agent</id>
<goals>
<goal>prepare-agent</goal>
</goals>
</execution>
<execution>
<id>report</id>
<phase>prepare-package</phase>
<goals>
<goal>report</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
By integrating a code coverage tool into the build process, developers can get visibility into the effectiveness of their tests and identify areas for improvement.
Fuzz Testing with QuickTheories
Fuzz testing, also known as property-based testing, is a testing technique that involves generating random and potentially invalid inputs to find unforeseen edge cases. QuickTheories is a library for property-based testing in Java, which can be used to perform fuzz testing. By using QuickTheories, developers can uncover unexpected behaviors and maximize test coverage without the need to explicitly write test cases for every input variation.
Here's an example of fuzz testing with QuickTheories:
import org.quicktheories.core.Gen;
import org.quicktheories.generators.SourceDSL;
import org.quicktheories.QuickTheory.qt;
public class StringUtilsFuzzTest {
@Test
public void testReverseString() {
Gen<String> strings = SourceDSL.strings().basicLatinAlphabet().ofLengthBetween(0, 100);
qt().forAll(strings).check(str -> {
return StringUtils.reverse(StringUtils.reverse(str)).equals(str);
});
}
}
In this example, we use QuickTheories to fuzz test the reverseString
method of a StringUtils
class. The strings
generator produces random strings of varying lengths to test the behavior of the method. Fuzz testing with QuickTheories can help uncover edge cases and potential issues that might not be covered by traditional test cases.
Closing Remarks
Maximizing regression test code coverage without scripts is achievable through various techniques and tools available in the Java ecosystem. By leveraging parameterized tests, data-driven testing, code coverage tools, and fuzz testing libraries, developers can ensure comprehensive test coverage without writing extensive test scripts. These approaches not only improve the effectiveness of tests but also contribute to the overall quality and stability of the software product.
In conclusion, while test scripts are valuable, Java provides a plethora of features and tools that can help achieve comprehensive test coverage without solely relying on script-based testing. By embracing the versatility of Java along with the available testing frameworks and libraries, developers can maximize regression test code coverage and ensure the robustness of their software applications.
By adopting these techniques, developers can iteratively improve test coverage and maintain code quality without getting entangled in the complexity of creating and managing a large number of test scripts.
To learn more about the concepts and tools discussed in this blog post, you can check out the official documentation for JUnit, TestNG, JaCoCo, and QuickTheories.