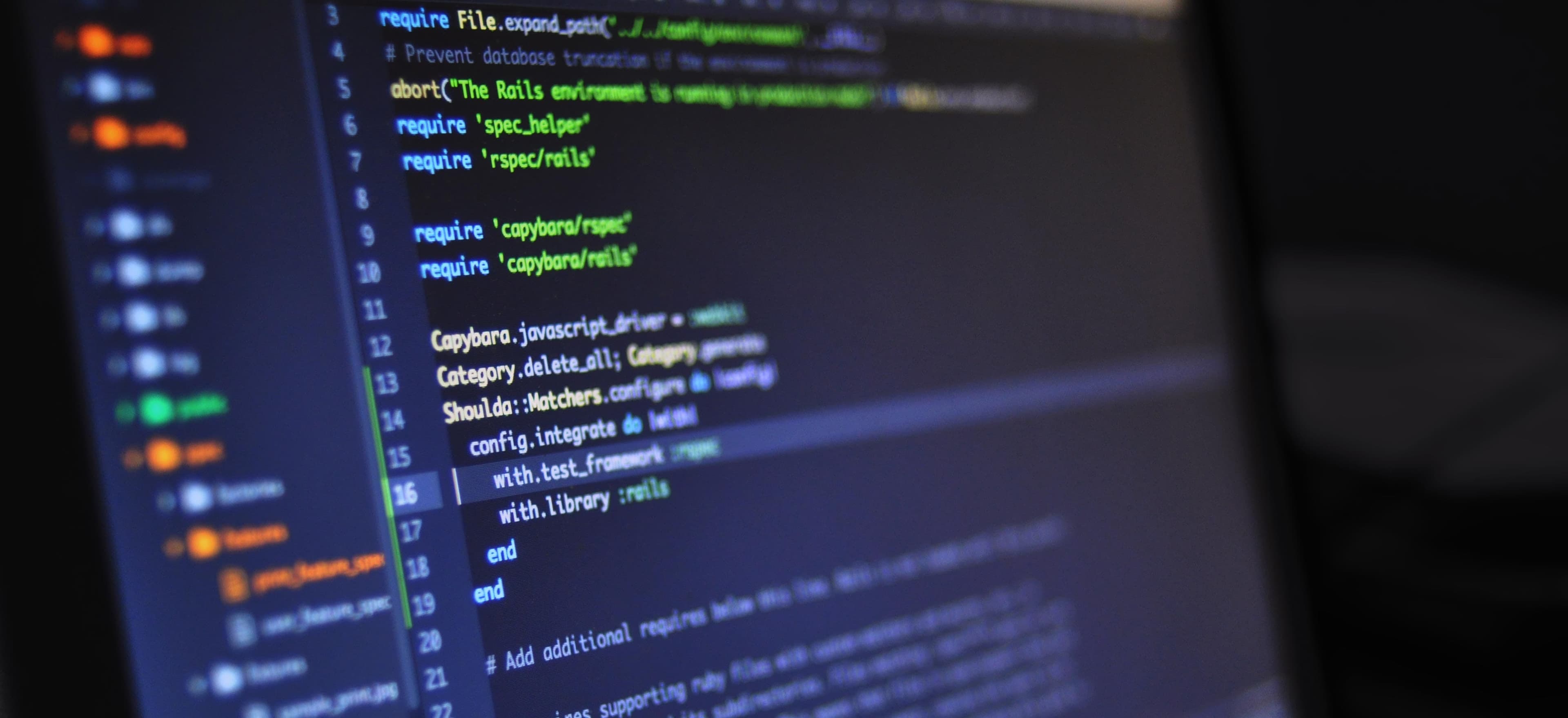
- Published on
Real-time Data Updates with PrimeFaces Push
In today’s fast-paced world, real-time data updates have become a necessity for modern web applications. Users expect to see changes as they happen without the need to refresh the page constantly. Java developers can accomplish this using PrimeFaces Push, a powerful library that simplifies real-time communication in web applications.
In this article, we will explore the concept of real-time data updates and demonstrate how to implement PrimeFaces Push in a Java web application. We will also discuss the benefits of real-time communication and provide a step-by-step guide to integrating PrimeFaces Push into your project.
Understanding Real-time Data Updates
Real-time data updates refer to the seamless and instantaneous delivery of new information to users as it becomes available. Traditionally, web applications relied on the user to manually refresh the page to see the latest data. However, with real-time updates, changes are pushed to the user interface without requiring any action from the user.
This capability is particularly valuable in scenarios such as live chats, stock market updates, sports scores, and collaborative editing, where users need to see changes in real time. By adopting real-time data updates, developers can significantly enhance the user experience and make their applications more dynamic and engaging.
Introducing PrimeFaces Push
PrimeFaces Push is an extension of PrimeFaces, a widely used open-source UI component library for JavaServer Faces (JSF) web applications. PrimeFaces Push leverages the WebSocket protocol to enable real-time communication between the server and the client, allowing for seamless data updates.
By integrating PrimeFaces Push into a Java web application, developers can achieve real-time functionality without having to manage complex low-level WebSocket interactions. The library provides high-level abstractions and powerful components, making it straightforward to implement real-time features in JSF-based projects.
Implementing PrimeFaces Push in Java
To demonstrate how to implement real-time data updates with PrimeFaces Push, let’s consider a simple example of a stock price monitoring application. In this scenario, the application needs to display real-time updates of stock prices to the user interface as soon as they are available.
Step 1: Setting up the Project
First, create a new Java web project using a framework such as Java EE, Spring MVC, or any other framework that supports JSF. Ensure that PrimeFaces is included as a dependency in the project.
Step 2: Establishing a WebSocket Endpoint
Next, define a WebSocket endpoint to handle the real-time communication between the server and the client. This endpoint will be responsible for broadcasting stock price updates to the connected clients.
@ServerEndpoint(value = "/stockUpdates")
public class StockUpdatesEndpoint {
@Inject
private StockPriceService stockPriceService;
@OnOpen
public void onOpen(Session session) {
// Register the session for updates
StockPriceService.register(session);
}
@OnClose
public void onClose(Session session) {
// Unregister the session
StockPriceService.unregister(session);
}
@OnMessage
public void onMessage(String message, Session session) {
// Handle incoming messages if needed
}
}
In this example, the StockUpdatesEndpoint
class establishes a WebSocket endpoint at the /stockUpdates
path. It injects a StockPriceService
to handle the stock price updates and defines methods to register and unregister client sessions.
The @OnOpen
and @OnClose
methods are invoked when a client connects or disconnects, allowing for session management. Meanwhile, the @OnMessage
method can be used to handle incoming messages from the client if needed.
Step 3: Broadcasting Stock Price Updates
Create a service class, such as StockPriceService
, responsible for retrieving and broadcasting real-time stock price updates to the connected clients.
@Singleton
public class StockPriceService {
private static Set<Session> sessions = new HashSet<>();
public void broadcastStockUpdate(StockPriceUpdate update) {
for (Session session : sessions) {
if (session.isOpen()) {
session.getAsyncRemote().sendObject(update, result -> {
if (result.getException() != null) {
// Handle failure
}
});
}
}
}
public static void register(Session session) {
sessions.add(session);
}
public static void unregister(Session session) {
sessions.remove(session);
}
}
In this example, the StockPriceService
class maintains a set of active client sessions and provides methods to register and unregister sessions. The broadcastStockUpdate
method sends real-time stock price updates to all connected clients using asynchronous messaging.
Step 4: Displaying Real-time Updates in the UI
Integrate PrimeFaces Push components into the JSF pages to receive and display real-time stock price updates as they are broadcasted from the server.
<h:form>
<p:panel header="Real-time Stock Prices">
<p:growl widgetVar="stockUpdates" showDetail="true" />
</p:panel>
</h:form>
<!-- Define PushContext in the UI -->
<p:socket onMessage="handleStockUpdate" channel="/stockUpdates" autoConnect="true" />
<script type="text/javascript">
function handleStockUpdate(data) {
// Process and display stock price updates
// Example: show updates using p:growl
PF('stockUpdates').renderMessage({severity: 'info', summary: 'Stock Update', detail: data});
}
</script>
In this snippet, we utilize the <p:socket>
component to establish a WebSocket connection and specify the JavaScript function handleStockUpdate
to process incoming stock price updates. The handleStockUpdate
function can update the UI using PrimeFaces components, such as p:growl
, to display the real-time stock price information to the user.
By following these four steps, developers can successfully integrate PrimeFaces Push into a Java web application to achieve real-time data updates, enhancing the overall user experience.
Benefits of Real-time Data Updates
Implementing real-time data updates in a Java web application offers several benefits, including:
-
Enhanced User Experience: Users can see live updates without needing to refresh the page, making the application more dynamic and engaging.
-
Increased Interactivity: Real-time data updates enable interactive features such as live chats, collaborative editing, and multiplayer gaming.
-
Timely Information: Users receive timely information, particularly useful for applications dealing with stock market data, news updates, or real-time monitoring.
-
Efficient Resource Usage: Real-time communication reduces the need for continuous polling of the server, leading to more efficient resource utilization.
Bringing It All Together
In conclusion, real-time data updates are a crucial feature for modern web applications, and PrimeFaces Push provides a straightforward way to implement this functionality in Java web projects. By leveraging PrimeFaces Push, developers can create dynamic, interactive, and engaging applications with seamless real-time communication.
With the step-by-step guide and examples provided in this article, developers can confidently integrate PrimeFaces Push into their Java web applications and harness the power of real-time data updates to deliver a superior user experience.
By embracing real-time communication, Java web applications can stay ahead of the curve and meet the evolving expectations of users in an increasingly interconnected digital world.
Implementing real-time data updates with PrimeFaces Push is a transformational step towards building modern, interactive, and responsive web applications. Don’t miss out on the opportunity to elevate your Java projects with real-time communication capabilities. Start integrating PrimeFaces Push today and unleash the full potential of real-time data updates in your web applications!
For more information on PrimeFaces Push, you can refer to the official PrimeFaces documentation.
Remember, real-time communication is not just a luxury; it’s a necessity in today’s digital landscape. Stay ahead of the curve and delight your users with seamless, instantaneous updates. Happy coding!