Optimizing Stream Processing with RxJava 1 and 2
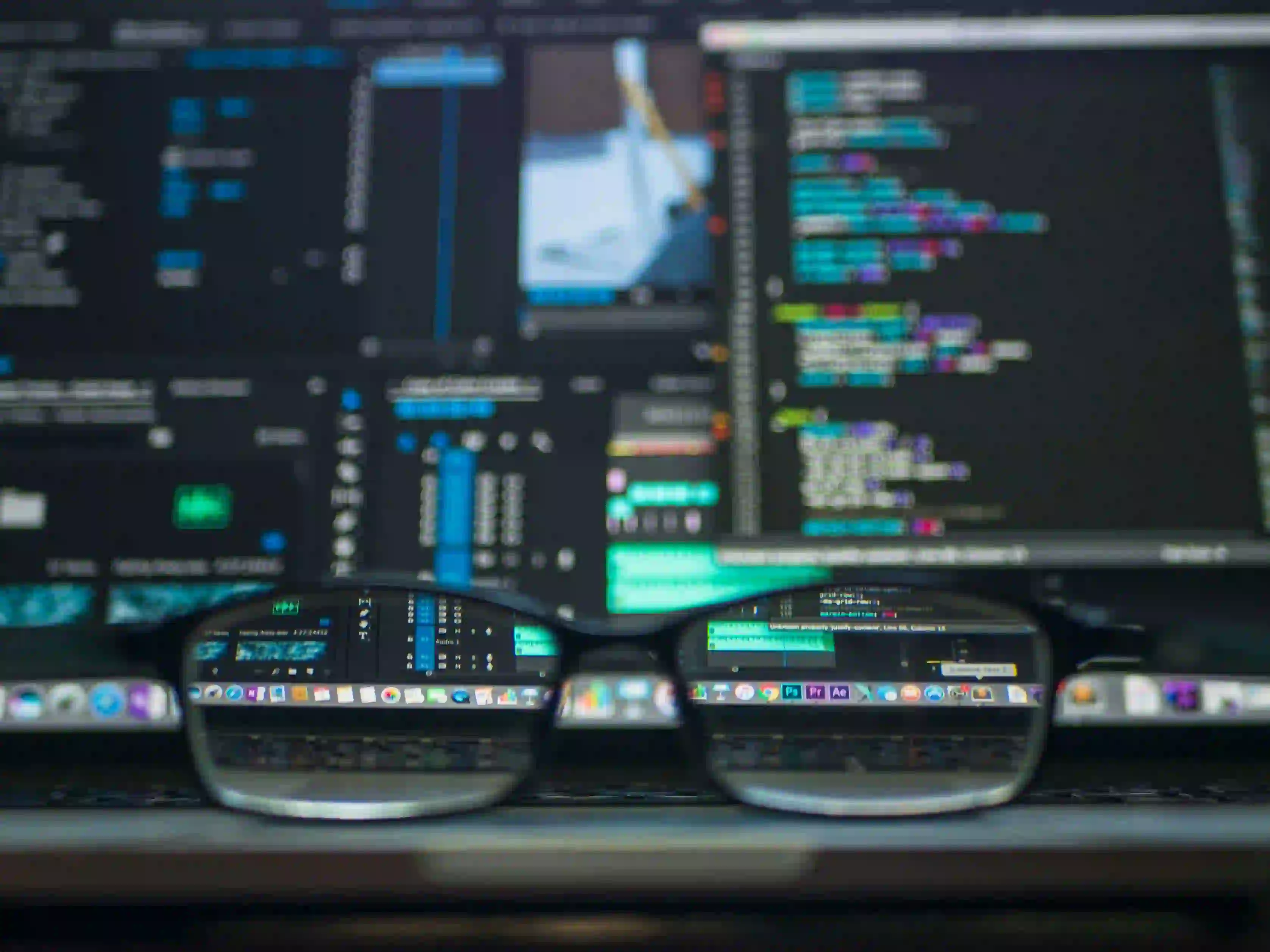
Optimizing Stream Processing with RxJava 1 and 2
In the world of Java development, handling asynchronous operations and stream processing is a common task. With the advent of RxJava, handling these operations has become more manageable and efficient. RxJava provides a powerful toolkit for working with asynchronous data streams, enabling developers to create robust and responsive applications.
In this article, we'll explore how to optimize stream processing with RxJava 1 and 2. We'll cover various techniques and best practices for maximizing the performance and efficiency of stream processing using RxJava.
Understanding RxJava
RxJava is a Java implementation of ReactiveX, which is a library for composing asynchronous and event-based programs using observable sequences. It provides a functional and reactive programming paradigm for working with streams of data.
The primary components of RxJava are Observables, Observers, and Operators.
- Observable: Represents a stream of data or events.
- Observer: Listens to the data emitted by the Observable.
- Operators: Manipulate and transform the data emitted by Observables.
Optimization Techniques
1. Avoiding Unnecessary Subscriptions
In RxJava, it's crucial to avoid unnecessary subscriptions to Observables. Subscribing multiple times to the same Observable can lead to redundant processing and unnecessary resource consumption.
// Bad practice: Subscribing to the same Observable multiple times
Observable<String> dataSource = getData(); // Assuming this method returns an Observable
dataSource.subscribe(data -> process(data));
dataSource.subscribe(data -> logData(data));
In the above example, getData()
is called twice, resulting in two separate subscriptions to the dataSource
Observable. To optimize this, you can use the share()
operator to create a single shared subscription.
// Good practice: Using the share() operator to create a single shared subscription
Observable<String> dataSource = getData().share();
dataSource.subscribe(data -> process(data));
dataSource.subscribe(data -> logData(data));
By using the share()
operator, you ensure that the getData()
method is called only once, and the emitted data is shared among all the subscriptions.
2. Using Backpressure Strategies
In scenarios where the Observable emits data at a higher rate than the Observer can process, backpressure may occur, leading to buffer overflows or memory issues. RxJava 1 and 2 provide different solutions for handling backpressure.
In RxJava 1, the onBackpressureBuffer()
operator can be used to buffer emissions in case of backpressure.
Observable.range(1, 1000)
.onBackpressureBuffer()
.observeOn(Schedulers.io())
.subscribe(data -> process(data));
RxJava 2 introduces the Flowable
class, which natively supports backpressure strategies.
Flowable.range(1, 1000)
.onBackpressureBuffer()
.observeOn(Schedulers.io())
.subscribe(data -> process(data));
By using backpressure strategies, you can prevent overwhelming the system with a high rate of emissions.
3. Proper Resource Management
When working with resources such as file handles, network connections, or database connections within RxJava, it's important to manage these resources efficiently to avoid leaks and ensure proper cleanup.
RxJava 2 introduces the using()
operator, which allows for the creation and disposal of resources within an Observable stream.
Observable.using(
() -> acquireResource(), // Resource acquisition function
resource -> Observable.just(processData(resource)), // Observable emitting the data
resource -> releaseResource(resource) // Resource disposal function
)
.subscribe(data -> processData(data));
By utilizing the using()
operator, you can ensure that resources are acquired, utilized, and released in a controlled manner within the Observable stream.
4. Scheduling and Threading
Proper scheduling and threading can significantly impact the performance of stream processing in RxJava. It's important to choose the appropriate schedulers for performing computational tasks, I/O operations, and UI interactions.
Observable.just("data")
.subscribeOn(Schedulers.io()) // Perform the operation on an I/O thread
.observeOn(AndroidSchedulers.mainThread()) // Observe the result on the main UI thread
.subscribe(data -> updateUI(data));
By using the subscribeOn()
and observeOn()
operators with the appropriate schedulers, you can ensure that the tasks are executed on the right threads, optimizing performance and responsiveness.
To Wrap Things Up
In conclusion, optimizing stream processing with RxJava involves adhering to best practices, using appropriate operators, and managing resources effectively. By employing techniques such as avoiding unnecessary subscriptions, handling backpressure, managing resources, and utilizing proper scheduling, developers can maximize the performance and efficiency of their RxJava stream processing.
RxJava continues to evolve, with the release of RxJava 2 providing additional features and improvements for stream processing optimization. By staying updated with the latest advancements in RxJava, developers can leverage its capabilities to build responsive and scalable applications.
Stream processing with RxJava is a powerful tool in the Java developer's arsenal, and optimizing its usage can lead to significant performance improvements in asynchronous and event-based applications.
For further reading on RxJava, you can explore the official documentation on RxJava GitHub and the RxJava Wiki for more in-depth insights into the library's functionalities and best practices.