Optimizing Database Queries with Spring Data JPA
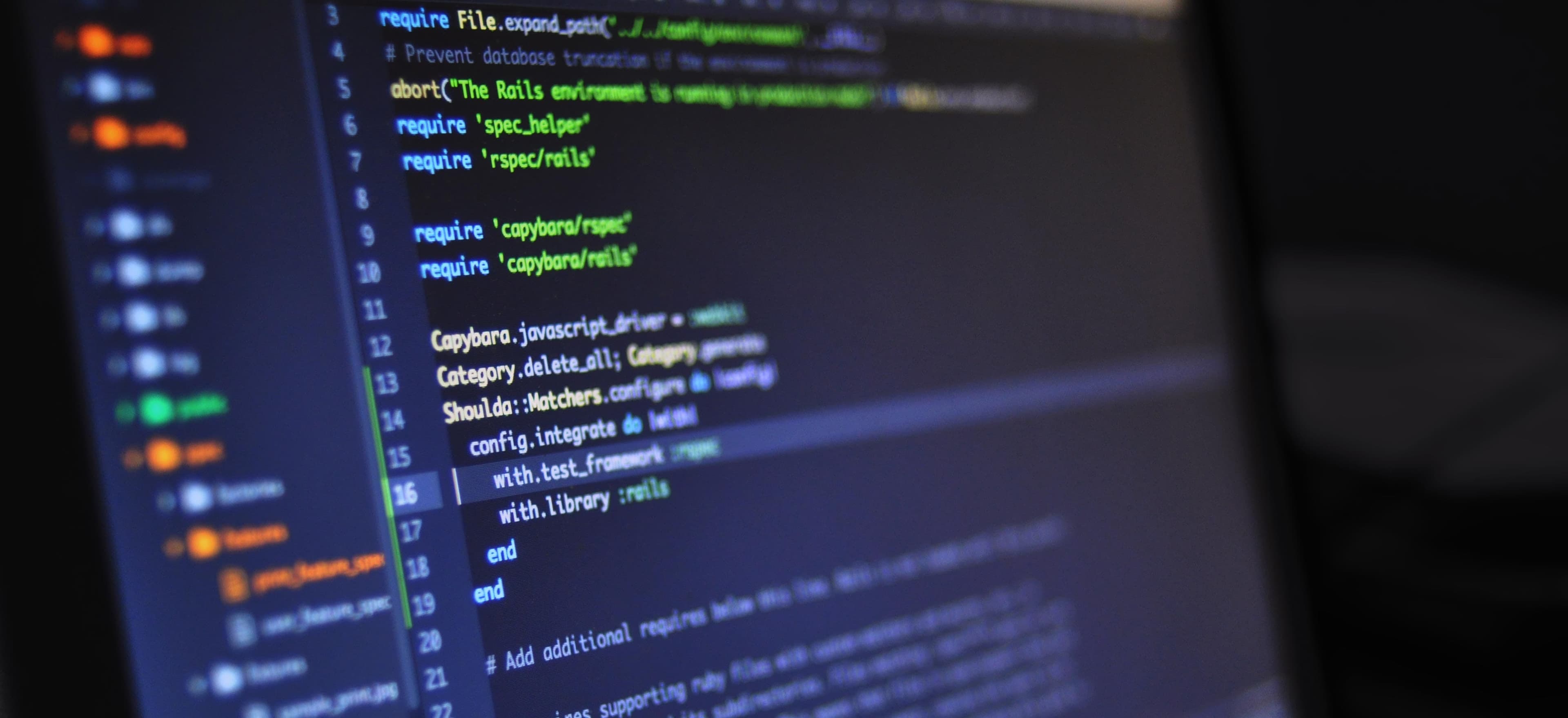
- Published on
Optimizing Database Queries with Spring Data JPA
When it comes to building robust enterprise applications in Java, efficient database access is crucial for performance. In this blog post, we will explore how to optimize database queries using Spring Data JPA, a powerful tool for working with JPA in the Spring Framework. We'll cover some best practices and techniques to improve query performance and reduce database load.
Understanding the Importance of Query Optimization
In modern web applications, database queries often play a critical role in determining the overall performance of the system. Poorly optimized queries can lead to increased response times, decreased scalability, and unnecessary resource consumption. By optimizing our database queries, we can minimize the time required to fetch data and reduce the load on the database server, resulting in a more responsive and efficient application.
Utilizing Spring Data JPA for Efficient Querying
Spring Data JPA simplifies the development of data access layers by providing a set of abstractions for working with JPA repositories. It allows developers to define repository interfaces that automatically generate the necessary SQL queries based on method names, without having to write boilerplate code for basic CRUD operations. This reduces the amount of code that needs to be maintained, and also promotes consistency across the application.
Example: Creating a Simple JPA Repository Interface
import org.springframework.data.jpa.repository.JpaRepository;
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
In this example, the UserRepository
interface extends JpaRepository
and defines a custom query method findByUsername
. Spring Data JPA will automatically generate the SQL query to retrieve a User
entity by its username
property based on the method name.
Techniques for Query Optimization
Now, let's delve into some techniques for optimizing database queries with Spring Data JPA.
1. Use Proper Indexing
One of the most fundamental aspects of database query optimization is ensuring that the database tables are properly indexed. Indexes allow the database engine to quickly locate rows in a table based on the values of certain columns. When defining entity classes in Spring Data JPA, it's essential to identify the fields that are commonly used in queries and annotate them with @Indexed
or @Index
to indicate that they should be indexed in the database.
Example: Adding Index Annotations to Entity Fields
import javax.persistence.Entity;
import javax.persistence.Index;
import javax.persistence.Table;
@Entity
@Table(name = "users", indexes = {
@Index(name = "idx_username", columnList = "username"),
@Index(name = "idx_email", columnList = "email")
})
public class User {
// Entity fields and methods
}
In this example, the User
entity class specifies indexes for the username
and email
columns using the @Index
annotation. This helps the database engine optimize query execution for operations involving these columns.
2. Fetch Only What You Need
When querying entities with relationships in Spring Data JPA, it's essential to fetch only the data that is required. By default, JPA @ManyToOne
and @OneToOne
relationships are fetched eagerly, meaning that associated entities are automatically loaded when the parent entity is retrieved. This can lead to unnecessary data retrieval and performance overhead, especially when dealing with large datasets.
To address this, we can use the @ManyToOne(fetch = FetchType.LAZY)
and @OneToOne(fetch = FetchType.LAZY)
annotations to specify lazy fetching for these relationships. Lazy fetching defers the loading of associated entities until they are explicitly accessed, thus avoiding unnecessary data retrieval and improving query performance.
Example: Using Lazy Fetching for Relationships
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.ManyToOne;
@Entity
public class Order {
// other fields
@ManyToOne(fetch = FetchType.LAZY)
private Customer customer;
// getters and setters
}
In this example, the customer
field in the Order
entity is annotated with fetch = FetchType.LAZY
to indicate that the Customer
entity should be lazily loaded when accessed, reducing the amount of data fetched during queries involving the Order
entity.
3. Limiting and Paginating Results
In scenarios where large result sets are expected, it's advisable to limit the number of records returned by a query. This can be achieved using the LIMIT
clause in SQL or by leveraging Spring Data JPA's built-in support for result set pagination. Pagination allows us to fetch a subset of the result set based on a defined page size and page number, thus reducing the memory footprint and improving query performance.
Example: Implementing Pagination with Spring Data JPA
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.jpa.repository.JpaRepository;
public interface UserRepository extends JpaRepository<User, Long> {
Page<User> findAll(Pageable pageable);
}
In this example, the UserRepository
interface declares a method that returns a Page
of User
entities by utilizing the Pageable
parameter. When invoking this method, we can pass a PageRequest
object specifying the desired page size and page number to enable result set pagination.
Monitoring and Profiling Query Performance
Lastly, it's essential to monitor and profile the performance of database queries in our Spring Data JPA-based application. By using tools like the Spring Boot Actuator, we can gather insights into the execution times and resource utilization of database queries. Additionally, integrating a database query profiler such as Hibernate's built-in statistics or third-party monitoring tools can provide valuable metrics for identifying bottlenecks and optimizing query execution.
My Closing Thoughts on the Matter
In this blog post, we have explored the importance of optimizing database queries in Java applications and discussed various techniques for improving query performance with Spring Data JPA. By leveraging proper indexing, efficient data fetching strategies, pagination, and query monitoring, we can significantly enhance the efficiency and responsiveness of our database interactions.
Optimizing database queries is a continuous process that requires careful analysis, testing, and refinement. By integrating these best practices and techniques into our Spring Data JPA-based applications, we can ensure that our database access layer operates at peak performance, leading to a more seamless and efficient user experience.
Stay tuned for more insightful discussions on Java development and database optimization!