Lambda Exception Handling: A Clean Solution
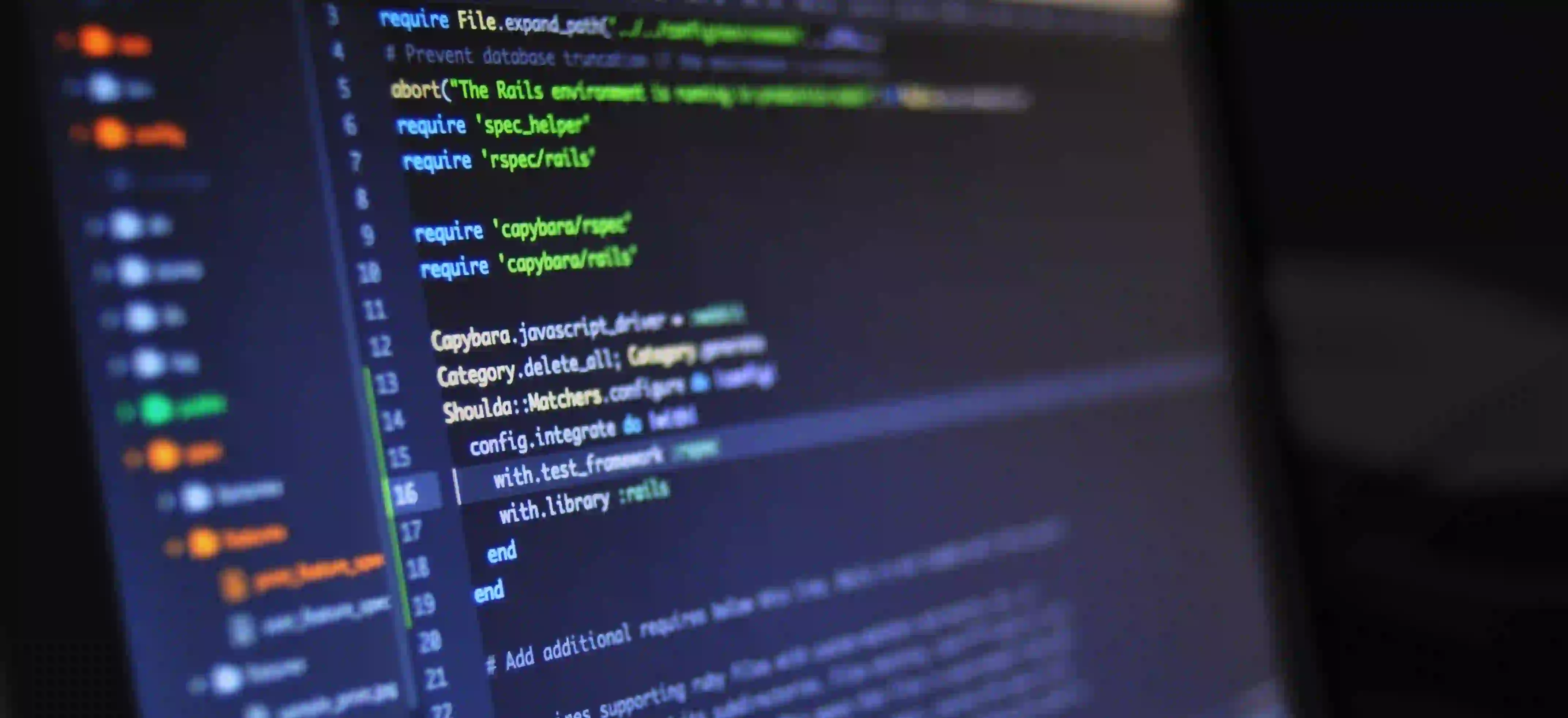
Lambda Exception Handling: A Clean Solution
Exception handling within lambda expressions has been a long-standing challenge for many Java developers. Traditional try-catch blocks do not function within lambda bodies, and this limitation can lead to convoluted, error-prone code. However, with the introduction of Java 8, the language has provided a more streamlined and elegant approach to handle exceptions within lambda expressions. In this article, we will explore the concept of lambda exception handling in Java and how to implement a clean and effective solution.
The Need for Lambda Exception Handling
Prior to Java 8, handling exceptions within lambda expressions was cumbersome. Any checked exceptions that the functional interface might throw needed to be caught, leading to verbose and cluttered code. This went against the principle of lambda expressions, which aimed to provide a more concise and readable way of writing code.
With the arrival of Java 8's functional interfaces and lambda expressions, the need for a more streamlined approach to exception handling within lambdas became increasingly apparent. The introduction of functional interfaces paved the way for the use of lambda expressions, thereby necessitating a cleaner exception handling mechanism within these constructs.
Utilizing Functional Interfaces and Lambdas
Functional interfaces are a key feature introduced in Java 8, providing a single abstract method which can represent a lambda expression. The introduction of functional interfaces set the stage for handling exceptions within lambda expressions, as the interface definition can specify the checked exceptions it might throw.
Consider the following functional interface, Executable
, which throws an Exception
:
@FunctionalInterface
interface Executable {
void execute() throws Exception;
}
Here, the @FunctionalInterface
annotation specifies that Executable
is a functional interface, and the execute()
method is the single abstract method it contains. The method is declared to throw an Exception
, showcasing how a checked exception can be incorporated within the functional interface.
Handling Exceptions within Lambda Expressions
To handle exceptions within lambda expressions, developers can leverage the functional interface's ability to declare checked exceptions. By doing so, lambda expressions can propagate exceptions outside their bodies, allowing for cleaner and more maintainable code.
Consider the following lambda expression which utilizes the Executable
functional interface:
Executable executable = () -> {
// Code that might throw an exception
Files.readAllLines(Paths.get("file.txt"));
};
try {
executable.execute();
} catch (Exception e) {
// Exception handling logic
System.err.println("An exception occurred: " + e.getMessage());
}
In this example, the Executable
functional interface is implemented using a lambda expression. Within the lambda body, the Files.readAllLines
method is called, which might throw an IOException
. By declaring the execute()
method in the functional interface to throw an Exception
, the lambda expression can propagate any thrown exceptions.
The Executable
instance executable
is then executed within a try-catch block, allowing for the handling of any exceptions that may be thrown within the lambda expression. This approach provides a cleaner and more centralized way of handling exceptions within lambda expressions.
Exception Handling and Functional Interfaces
When designing functional interfaces, it is crucial to consider exception handling. By incorporating checked exceptions within the functional interface's method signature, developers can ensure that any potential exceptions thrown within the lambda expression are accounted for.
Consider a scenario where a functional interface does not declare any checked exceptions:
@FunctionalInterface
interface Operation {
void perform();
}
In this case, if the perform()
method encounters a checked exception, there is no way to propagate it outside the lambda expression. As a result, developers might be tempted to resort to cumbersome try-catch blocks within the lambda body, leading to code that deviates from the elegance and conciseness that lambda expressions aim to provide.
By contrast, a functional interface that declares checked exceptions provides a clear contract regarding the potential exceptions that the lambda expression might throw. This facilitates better error handling and promotes more readable and maintainable code.
Introducing Wrapper Functional Interfaces
To address scenarios where a lambda expression might need to work with code that throws checked exceptions, wrapper functional interfaces can be introduced. These interfaces act as a bridge, enabling the lambda expression to propagate any thrown exceptions via a functional interface that accounts for checked exceptions.
Consider the following wrapper functional interface, UncheckedExecutable
, which encapsulates an Executable
and handles any potential exceptions:
@FunctionalInterface
interface UncheckedExecutable {
void executeUnchecked() throws RuntimeException;
static UncheckedExecutable from(Executable executable) {
return () -> {
try {
executable.execute();
} catch (Exception e) {
throw new RuntimeException(e);
}
};
}
}
In this example, the UncheckedExecutable
interface declares executeUnchecked()
to throw a RuntimeException
, thereby allowing the lambda expression to propagate any exceptions. The from()
static method acts as a factory method, wrapping an Executable
within an UncheckedExecutable
and handling any potential exceptions by rethrowing them as `RuntimeExceptions.
Implementing Lambda Exception Handling with Wrapper Interfaces
With the introduction of the UncheckedExecutable
wrapper functional interface, lambda expressions can now seamlessly handle checked exceptions. Let's revisit the previous example utilizing this wrapper interface:
UncheckedExecutable uncheckedExecutable = UncheckedExecutable.from(() -> {
Files.readAllLines(Paths.get("file.txt"));
});
uncheckedExecutable.executeUnchecked();
In this revised example, the UncheckedExecutable
is used to wrap the lambda expression, enabling it to handle any potential exceptions transparently. The lambda body itself remains free of explicit exception handling, maintaining its readability and conciseness.
To Wrap Things Up
In conclusion, Java 8's introduction of functional interfaces and lambda expressions has brought about a more elegant and concise way of writing code. However, the handling of exceptions within lambda expressions has been a point of concern for many developers. By leveraging functional interfaces that declare checked exceptions and introducing wrapper functional interfaces, it is possible to achieve clean and effective exception handling within lambda expressions.
By adhering to best practices in designing functional interfaces and utilizing wrapper interfaces when necessary, developers can maintain the readability and conciseness of lambda expressions while ensuring robust exception handling. This approach facilitates more maintainable code and a clearer separation of concerns, ultimately leading to a more cohesive and manageable codebase.