Optimizing Java Performance with Thread-Local Allocation Buffers
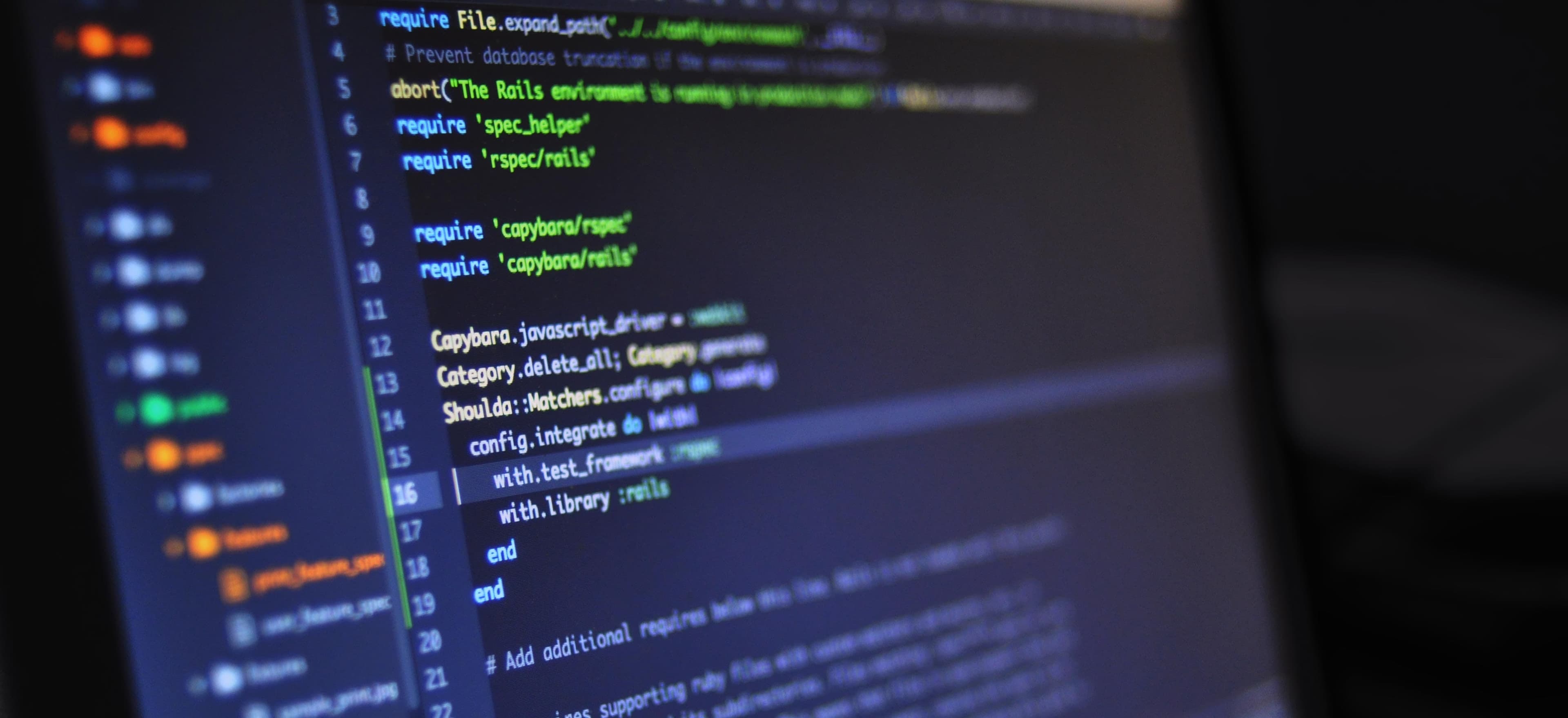
- Published on
Optimizing Java Performance with Thread-Local Allocation Buffers
In today's fast-paced software development landscape, optimizing performance is crucial to delivering responsive and efficient applications. Java, as a widely-used programming language, offers several tools and techniques to enhance performance. One such technique is the use of Thread-Local Allocation Buffers (TLABs) to reduce the overhead of object allocation and improve memory access patterns.
Understanding Thread-Local Allocation Buffers
TLABs are a memory allocation optimization technique used by the Java Virtual Machine (JVM) to speed up the creation of short-lived objects. When a new object is requested in Java, the JVM allocates memory for the object from the heap. This allocation process involves synchronization and memory management overhead, which can impact performance, especially in multi-threaded applications.
TLABs address this issue by assigning a small buffer to each thread, allowing the thread to allocate memory for new objects within its designated buffer without contending with other threads. This reduces contention and improves allocation performance, particularly for short-lived objects created within a specific thread's scope.
Implementing Thread-Local Allocation Buffers in Java
To demonstrate the use of TLABs in Java, let's consider a simplified example where we have a multi-threaded application that frequently creates short-lived objects.
public class TLABDemo {
public static void main(String[] args) {
final int numThreads = 4;
final int iterations = 1000000;
Thread[] threads = new Thread[numThreads];
for (int i = 0; i < numThreads; i++) {
threads[i] = new Thread(() -> {
for (int j = 0; j < iterations; j++) {
Object obj = new Object(); // Object allocation
// Perform operations with the object
}
});
}
for (Thread thread : threads) {
thread.start();
}
for (Thread thread : threads) {
try {
thread.join();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
}
In this example, we have a simple multi-threaded application with four threads, each creating a large number of short-lived objects. However, without TLABs, the object allocation process can incur significant synchronization overhead.
To enable TLABs, we can utilize the JVM flag -XX:+UseTLAB
which instructs the JVM to enable TLAB allocation. This flag is automatically enabled in most modern JVM implementations, but explicitly specifying it ensures TLABs are utilized.
java -XX:+UseTLAB TLABDemo
By running the application with TLABs enabled, the allocation of short-lived objects within each thread's buffer will significantly reduce contention and improve the overall allocation performance.
Key Benefits of Thread-Local Allocation Buffers
-
Reduced Contention
TLABs allow threads to allocate memory for objects without contending with other threads, reducing synchronization overhead and contention in multi-threaded applications.
-
Improved Memory Access Patterns
By allocating memory within a thread's designated buffer, TLABs promote better memory access patterns, leading to improved cache locality and reduced memory access latency.
-
Enhanced Allocation Performance
The use of TLABs results in faster object allocation, particularly for short-lived objects, contributing to overall performance optimization in Java applications.
Best Practices for Using Thread-Local Allocation Buffers
While TLABs offer significant performance benefits, it's important to consider best practices for their usage:
-
Profile and Measure
Before and after enabling TLABs, profile and measure the application's performance using tools like JMH (Java Microbenchmarking Harness) or Java Mission Control to quantify the impact of TLABs on object allocation and overall application performance.
-
Monitor TLAB Usage
Utilize JVM monitoring tools to track TLAB allocation and usage metrics. This can help identify TLAB-related performance improvements and potential tuning opportunities.
-
Evaluate Object Lifetimes
TLABs are most effective for short-lived objects, so consider the lifespan of objects in your application. Long-lived objects may not benefit as much from TLAB allocation.
-
JVM Configuration
Understand and experiment with JVM flags related to TLAB configuration, such as
-XX:TLABSize
and-XX:TLABRefillWasteFraction
, to optimize TLAB behavior based on specific application requirements. -
Thread Management
Consider thread management strategies to ensure TLAB utilization aligns with the application's threading model. For example, in highly concurrent scenarios, adjusting TLAB sizing and allocation policies may be beneficial.
In Conclusion, Here is What Matters
Thread-Local Allocation Buffers (TLABs) offer a valuable mechanism for improving memory allocation performance in Java applications, especially in multi-threaded environments. By reducing contention, enhancing memory access patterns, and accelerating object allocation, TLABs contribute to overall performance optimization.
When considering performance optimizations in Java, leveraging TLABs alongside other techniques such as garbage collection tuning, JIT compilation, and data structure optimizations can lead to substantial performance gains. As with any optimization effort, thorough testing, measurement, and continuous evaluation are essential to ensure the chosen optimizations align with the specific needs and characteristics of the application.
Optimizing Java performance through TLABs empowers developers to deliver more responsive, efficient, and scalable applications, enhancing the overall user experience and operational efficiency. Embracing performance optimizations like TLABs reflects a commitment to delivering high-quality, performant software in today's demanding technological landscape.
By incorporating Thread-Local Allocation Buffers (TLABs) into Java applications, developers can significantly enhance memory allocation and improve application performance. This technique reduces contention, enhances memory access patterns, and accelerates object allocation, ultimately contributing to an optimized and responsive application. If you're interested in further optimizing your Java application, consider exploring the benefits of Java Just-In-Time (JIT) compiler and garbage collection tuning as complementary performance optimization strategies.