Critical log4j Bug Impacting Application Performance
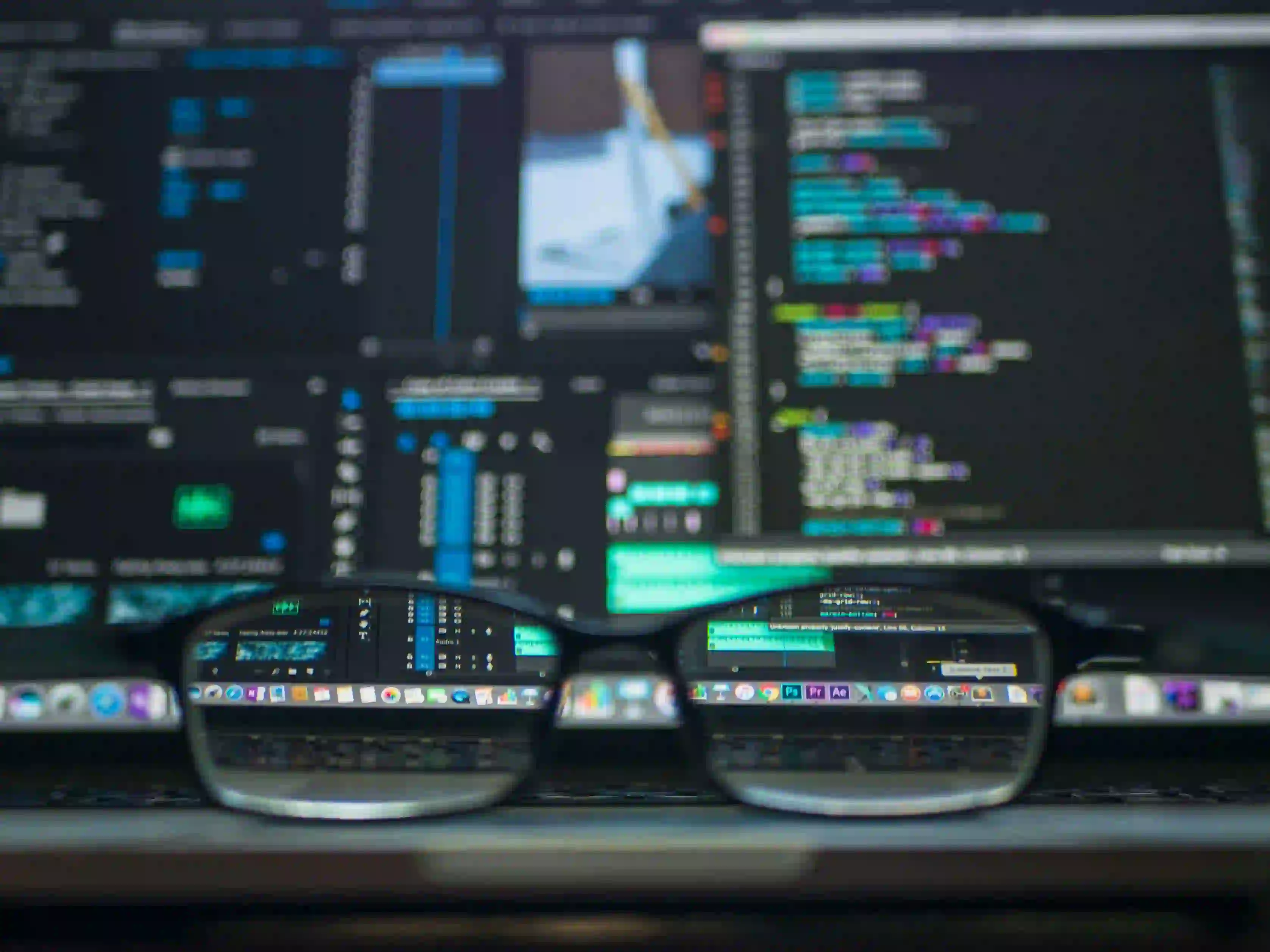
How to Optimize Java Logging for Improved Application Performance
Logging is an essential aspect of application development, providing visibility into the system's behavior, debugging, and error tracking. However, improper logging practices can impact an application's performance. In the wake of the critical log4j vulnerability, which has underscored the importance of securing logging frameworks, it is crucial to optimize Java logging for improved performance.
In this blog post, we will delve into best practices for optimizing Java logging to ensure minimal impact on application performance while maintaining effective visibility into its behavior.
Understanding the Impact of Logging on Application Performance
Logging is not without its costs. Excessive logging operations, inefficient log formatting, and inappropriate log levels can all contribute to performance degradation. Each logging operation incurs a computational cost, from the generation of log messages to their propagation and storage. As a result, an application's logging behavior must be carefully managed to strike a balance between necessary visibility and minimal performance overhead.
Utilizing Proper Log Levels
One of the fundamental principles of efficient logging is the appropriate use of log levels. Each log message should be assigned an appropriate severity level, such as DEBUG, INFO, WARN, ERROR, or FATAL, based on its significance. By adhering to a structured approach in determining the log level for each message, unnecessary logging operations can be avoided, thereby mitigating performance impact.
// Example of using proper log levels in Java
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class ExampleClass {
private static final Logger LOGGER = LogManager.getLogger(ExampleClass.class);
public void performTask() {
// Perform task
LOGGER.debug("Task performed successfully");
}
}
In the above example, the LOGGER.debug
statement demonstrates the use of the DEBUG log level for a message related to the successful execution of a task, ensuring that less critical events do not incur unnecessary overhead.
Async Logging
Java logging frameworks, such as Log4j 2, offer asynchronous logging capabilities to minimize the impact of logging on application performance. By offloading the actual logging operations to a separate thread or utilizing asynchronous appenders, the primary application thread is not impeded by the overhead of logging.
// Example of configuring async logging in Log4j 2
<?xml version="1.0" encoding="UTF-8"?>
<Configuration status="warn" name="AsyncConfig" packages="">
<Appenders>
<Async name="Async">
<AppenderRef ref="Console"/>
</Async>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="Async"/>
</Root>
</Loggers>
</Configuration>
The provided XML configuration demonstrates the setup of asynchronous logging in Log4j 2. By employing the <Async>
appender, log messages are processed in a separate thread, diminishing the impact on the application's main execution flow.
Efficient Log Formatting
Log messages often include contextual information such as timestamps, thread IDs, and log levels. While providing valuable insights, the generation of this additional data incurs computational costs. Employing efficient log formatting mechanisms, such as parameterized logging, can substantially reduce the overhead associated with log message construction.
// Example of efficient log message formatting in Java
private static final Logger LOGGER = LogManager.getLogger(ExampleClass.class);
public void performTask(int taskId) {
// Perform task
LOGGER.info("Task {} performed successfully", taskId);
}
In the above example, the use of parameterized logging with {}
as a placeholder for the taskId
reduces the computational burden of string concatenation, resulting in more efficient log message construction.
Log Message Sampling
In scenarios where high-frequency logging is necessary, log message sampling can be employed to selectively capture a subset of log events. Sampling strategies, such as periodic sampling or probabilistic sampling, allow for the reduction of logging overhead without completely sacrificing visibility into the application's behavior.
// Example of log message sampling in Java
private static final Logger LOGGER = LogManager.getLogger(ExampleClass.class);
public void processIncomingRequest(RequestData requestData) {
// Process request
if (shouldLogEvent()) {
LOGGER.debug("Request processed: {}", requestData);
}
}
private boolean shouldLogEvent() {
// Implement sampling logic
// Return true for desired log events, false for others
}
In the provided example, the processIncomingRequest
method employs the shouldLogEvent
method to determine whether a particular log message should be emitted, demonstrating the selective capture of log events based on a defined sampling strategy.
Leveraging Conditional Logging
Conditional logging allows for the dynamic determination of whether a log message should be emitted based on specified conditions. By incorporating conditional checks into logging statements, unnecessary log operations can be circumvented, further curtailing performance impact.
// Example of conditional logging in Java
private static final Logger LOGGER = LogManager.getLogger(ExampleClass.class);
public void performCriticalOperation() {
if (isDebugModeEnabled()) {
LOGGER.debug("Critical operation initiated");
}
}
private boolean isDebugModeEnabled() {
// Implement logic to check debug mode status
}
In the above scenario, the performCriticalOperation
method employs the isDebugModeEnabled
condition to determine whether the DEBUG-level log message should be emitted, thereby dynamically controlling logging behavior based on runtime conditions.
Lessons Learned
Optimizing Java logging for improved application performance involves a thoughtful approach to mitigating the overhead associated with logging operations while preserving essential visibility into the system's behavior. By utilizing proper log levels, asynchronous logging, efficient log formatting, log message sampling, and conditional logging, developers can strike a balance between logging overhead and runtime performance, ensuring a robust yet lightweight logging infrastructure.
In the wake of the log4j vulnerability, optimizing Java logging practices has become even more critical to ensure both performance efficiency and security resilience within applications. Embracing these best practices can safeguard against performance degradation while maintaining the necessary visibility into the runtime behavior of Java applications.
By implementing these strategies, developers can enhance the performance of Java applications, mitigate the impact of logging, and fortify the overall robustness of their systems.
For more in-depth information on Java logging best practices, refer to the official Log4j 2 documentation.
In summary, optimizing Java logging is not only beneficial for performance but also crucial in light of recent security concerns, making it an integral aspect of modern application development.