Optimizing Docker Commands for Java Developers
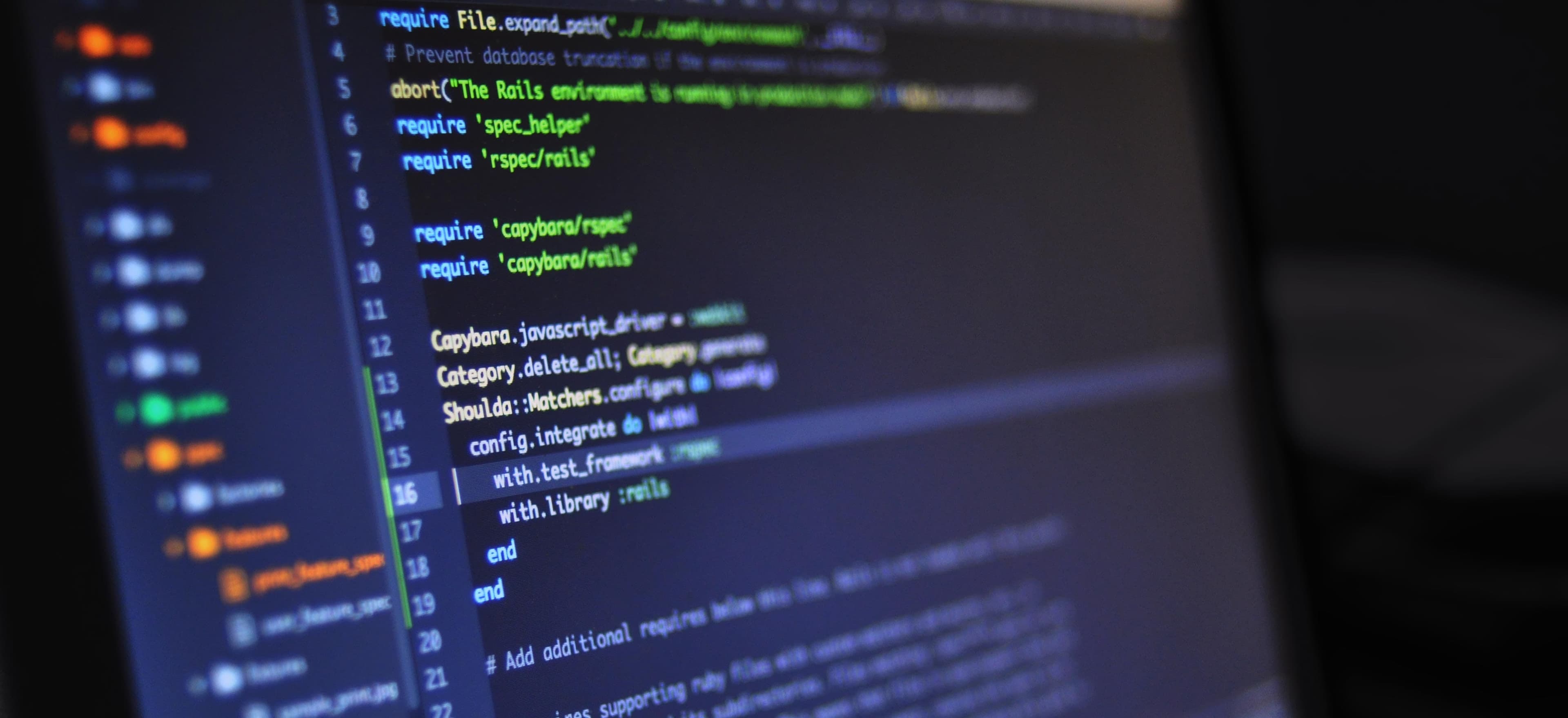
- Published on
Optimizing Docker Commands for Java Developers
Docker has revolutionized the way we build, ship, and run applications. Java developers, in particular, have embraced Docker for its ability to streamline the deployment process and create consistent environments across different stages of development. In this blog post, we will explore some advanced techniques to optimize Docker commands specifically for Java developers, allowing for a more efficient and seamless development workflow.
Why Optimize Docker Commands?
Optimizing Docker commands is crucial for Java developers aiming to streamline their development process. By using optimized Docker commands, developers can reduce build times, enhance container performance, and improve the overall development experience. This optimization ultimately leads to faster iterations, quicker feedback loops, and more reliable deployments.
Multi-Stage Builds for Java Applications
One of the most powerful features of Docker is multi-stage builds, which can be particularly beneficial for Java applications. By using multi-stage builds, Java developers can separate the build environment from the runtime environment, resulting in smaller and more efficient Docker images.
Example of a Multi-Stage Dockerfile for Java
# Use a maven image to build the application
FROM maven:3.6.3-openjdk-11 AS build
COPY src /usr/src/app/src
COPY pom.xml /usr/src/app
RUN mvn -f /usr/src/app/pom.xml clean package
# Use an adoptopenjdk image for the runtime environment
FROM adoptopenjdk:11-jre-hotspot
COPY --from=build /usr/src/app/target/app.jar /usr/app/app.jar
CMD ["java", "-jar", "/usr/app/app.jar"]
In this example, the multi-stage Dockerfile first uses a Maven image to build the application and then copies the built JAR file into a lightweight adoptopenjdk image for the runtime environment. This results in a smaller final image that only contains the necessary runtime components, without any build dependencies.
By optimizing the Dockerfile with multi-stage builds, Java developers can significantly reduce the size of their Docker images while maintaining a clean separation between the build and runtime environments.
Leveraging Build Caching
Build caching is another essential optimization technique for Docker. When building Java applications in Docker, leveraging build caching can drastically reduce build times by only rebuilding the necessary parts of the application when changes are made.
Example of Leveraging Build Caching in Dockerfile
# Use a maven image to build the application
FROM maven:3.6.3-openjdk-11 AS build
COPY src /usr/src/app/src
COPY pom.xml /usr/src/app
RUN mvn -f /usr/src/app/pom.xml dependency:go-offline
RUN mvn -f /usr/src/app/pom.xml package
In this example, the dependency:go-offline
Maven goal is executed before the package
goal. By doing so, Docker can take advantage of build caching for the dependencies, ensuring that they are only re-downloaded when the pom.xml
file changes.
By strategically leveraging build caching in the Dockerfile, Java developers can significantly reduce build times, especially in large projects with complex dependency graphs.
Persistent Volume Mapping for Development
During development, it's common for Java developers to make frequent changes to their code. To avoid rebuilding the entire Docker image every time a change is made, persistent volume mapping can be employed to mount the local code directory into the Docker container.
Example of using Persistent Volume Mapping with Docker Run Command
docker run -v /path/to/local/code:/usr/src/app your-java-image
In this example, the -v
flag is used to map the local code directory into the container's /usr/src/app
directory. This allows any changes made locally to immediately reflect inside the running Docker container, without requiring a rebuild of the image.
By utilizing persistent volume mapping during development, Java developers can benefit from a much faster feedback loop, as code changes are instantly reflected within the running container.
Utilizing Docker Compose for Service Orchestration
For Java applications that rely on multiple services such as databases, message brokers, or caching systems, Docker Compose can be a powerful tool for orchestrating these services together. With Docker Compose, Java developers can define and run multi-container Docker applications with ease.
Example of a Docker Compose File for Java Application and MySQL Database
version: '3.8'
services:
app:
build: .
ports:
- "8080:8080"
depends_on:
- db
db:
image: mysql:5.7
environment:
MYSQL_ROOT_PASSWORD: example
MYSQL_DATABASE: app_db
MYSQL_USER: app_user
MYSQL_PASSWORD: app_password
In this example, a Docker Compose file is used to define two services: the Java application and a MySQL database. The depends_on
directive ensures that the database service is started before the application service. With Docker Compose, Java developers can easily spin up and orchestrate complex multi-container applications with a single command.
By utilizing Docker Compose for service orchestration, Java developers can simplify the management of multi-container applications, making it easier to set up development and testing environments.
Closing the Chapter
Optimizing Docker commands for Java development can significantly improve the efficiency and reliability of the development workflow. By leveraging multi-stage builds, build caching, persistent volume mapping, and Docker Compose, Java developers can streamline the process of building, shipping, and running their applications in Docker.
Incorporating these advanced techniques into Docker commands not only boosts productivity but also enhances the overall development experience for Java developers. As Docker continues to be the de facto standard for containerization, mastering these optimization techniques is crucial for staying ahead in the world of Java development.
By mastering these optimization techniques, Java developers can maximize the benefits of Docker and take their development process to the next level.
Remember, understanding the architecture and the code you are writing is crucial to making the right optimizations. If you want to delve deeper into Java optimization techniques, take a look at this comprehensive guide on Java Performance Optimization.