Overcoming Ambiguity: Using Acceptance Tests as Clear Specifications
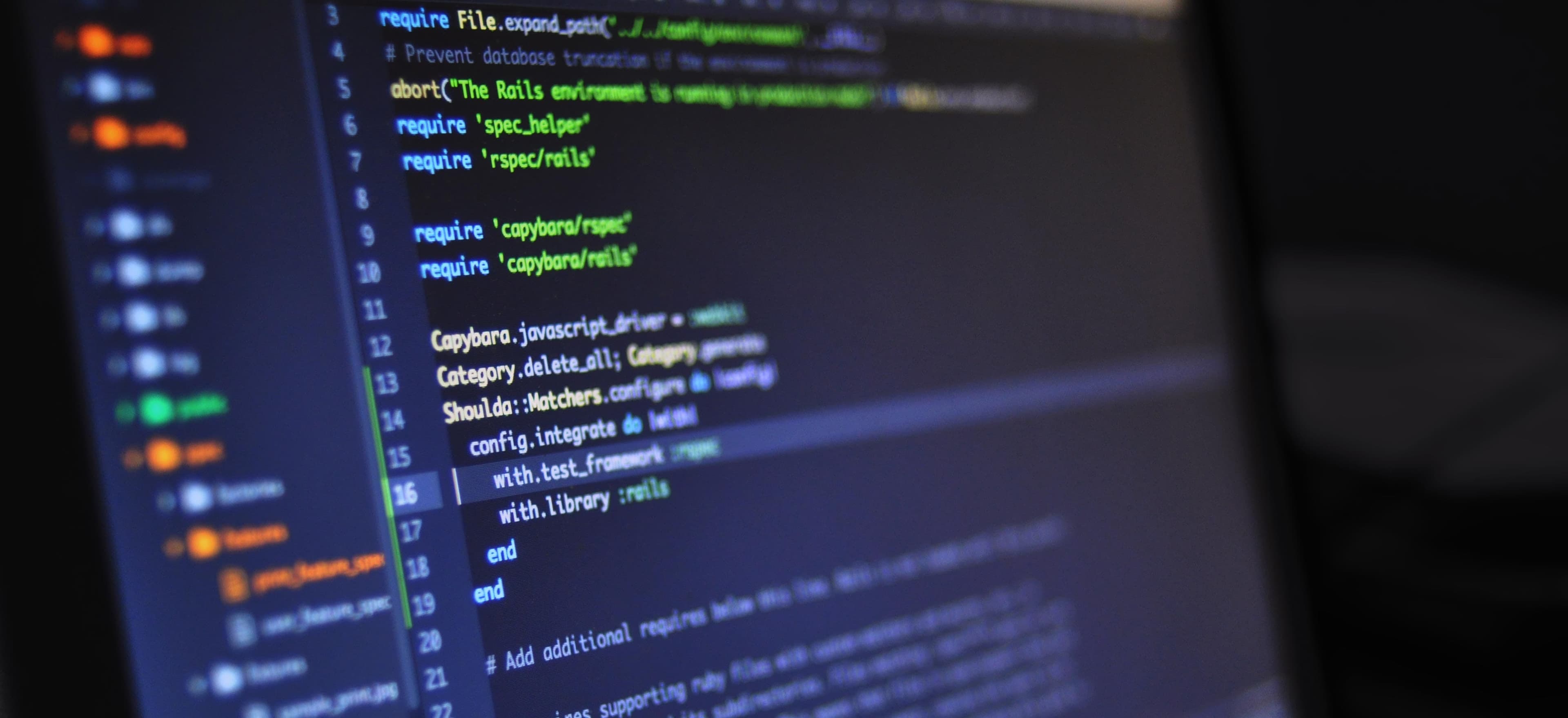
- Published on
Overcoming Ambiguity: Using Acceptance Tests as Clear Specifications
In software development, ambiguity is the enemy of efficiency. It leads to misunderstandings, rework, and ultimately, a product that does not meet the needs of its users. This is where acceptance tests come in. Acceptance tests provide a clear and unambiguous way to define the expected behavior of a system. By using acceptance tests as clear specifications, developers can ensure that they are building the right thing and that it meets the requirements of the end users.
What are Acceptance Tests?
Acceptance tests, also known as functional tests or end-to-end tests, are a type of software testing that evaluates the system's compliance with business requirements. These tests are written based on the user's perspective and are meant to simulate real-world scenarios, ensuring that the software behaves as expected when used by the end user.
The Problem of Ambiguity
Ambiguity in software requirements can lead to a variety of issues. It can result in misunderstanding and misinterpretation of the requirements, leading to the development of a product that does not align with the users' needs. This can lead to wasted time and resources, as well as frustration for both the development team and the end users.
Using Acceptance Tests as Specifications
Acceptance tests can serve as clear and unambiguous specifications for the software being developed. They provide a concrete way to define the expected behavior of the system, using real-world examples and scenarios. By writing acceptance tests early in the development process, teams can ensure that they have a shared understanding of the requirements and that the software they are building meets those requirements.
Example of Acceptance Test as Specification
@Test
void givenUserExists_whenLoginWithValidCredentials_thenLoginSuccessful() {
// Arrange
User testUser = new User("username", "password");
userRepository.save(testUser);
// Act
boolean loginResult = authService.login("username", "password");
// Assert
assertTrue(loginResult);
}
In this example, the acceptance test serves as a specification for the "login" feature of the system. It clearly defines the expected behavior: when a user with valid credentials attempts to log in, the login should be successful. This provides a clear and unambiguous definition of what the system should do, from the perspective of the end user.
Aligning Development with Business Requirements
By using acceptance tests as specifications, development teams can ensure that they are building software that aligns with the business requirements. Acceptance tests are written based on real-world scenarios and examples, which helps to bridge the gap between technical requirements and business needs. This alignment is crucial for delivering software that provides real value to the end users.
Improving Communication and Collaboration
Acceptance tests also serve as a communication tool between different stakeholders involved in the development process. By writing acceptance tests, developers, QA engineers, product owners, and other stakeholders can collaborate to define and understand the expected behavior of the system. This collaboration helps to identify and resolve any misunderstandings or ambiguities in the requirements early in the development process.
Ensuring Quality and Reducing Rework
Using acceptance tests as clear specifications can help to ensure the quality of the software being developed. By defining the expected behavior in a clear and unambiguous way, teams can catch and address any discrepancies early in the development process, reducing the need for rework later on. This ultimately leads to a more efficient development process and a higher-quality end product.
Bringing It All Together
In the world of software development, ambiguity is the enemy of progress. By using acceptance tests as clear specifications, development teams can ensure that they are building the right thing and that it meets the needs of the end users. Acceptance tests provide a concrete way to define the expected behavior of the system, align development with business requirements, improve communication and collaboration, and ensure the quality of the software being developed. Overall, using acceptance tests as clear specifications can help to overcome ambiguity and deliver software that provides real value to its users.
By leveraging the power of acceptance tests, developers can pave the way for a more efficient and effective development process, ultimately leading to the delivery of high-quality software that meets the needs of its end users.
To delve deeper into acceptance tests and their role in software development, consider exploring JUnit for Java for creating and running acceptance tests.
Remember, when clarity is the goal, acceptance tests can be the key to overcoming ambiguity in software development.