Improving Data Access Code Testing for Reliable Builds
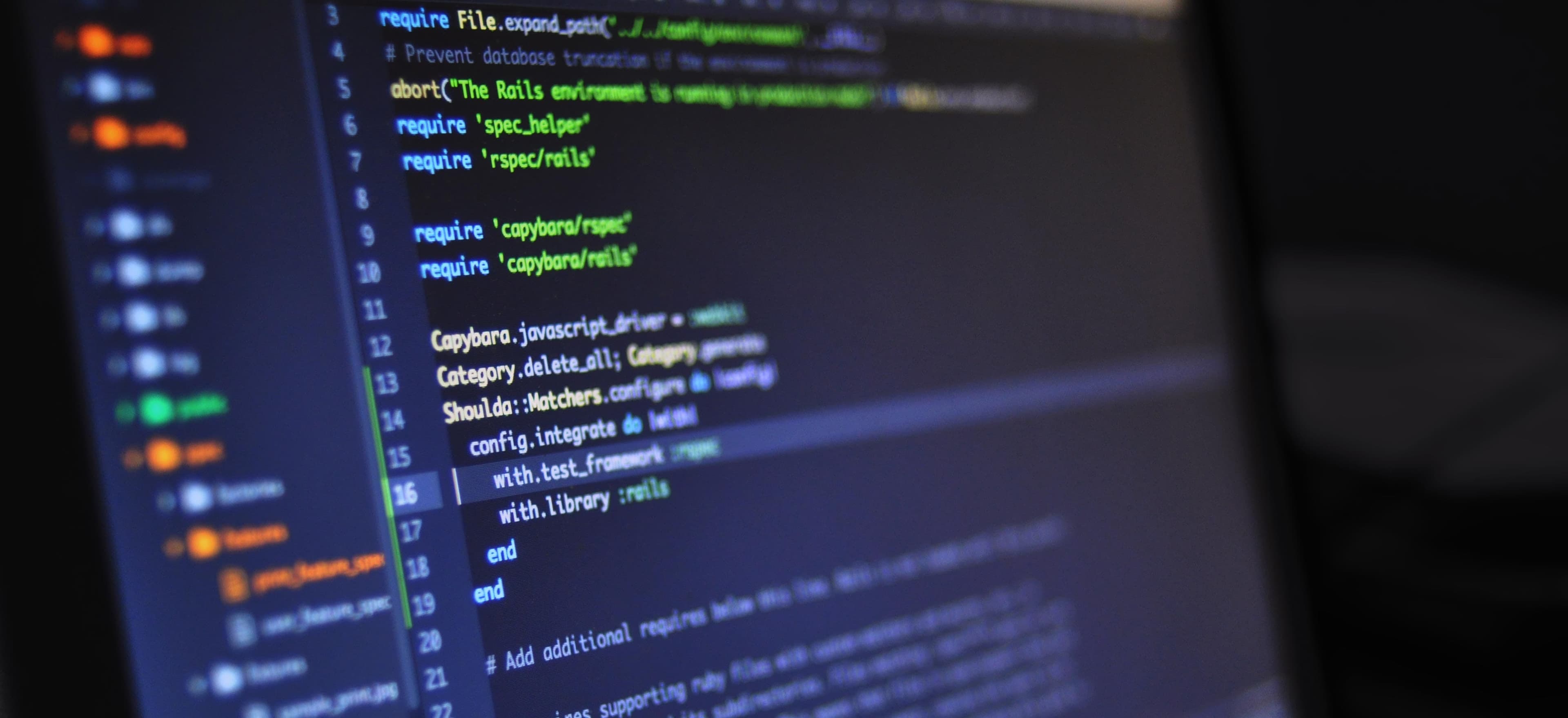
- Published on
Improving Data Access Code Testing for Reliable Builds
In the world of software development, one of the critical aspects of building reliable and robust applications is testing. And when it comes to data access code, the importance of thorough testing cannot be overstated. In this article, we will delve into strategies and best practices for testing data access code in Java to ensure reliable builds and minimize the risk of unexpected behavior in production.
Understanding the Importance of Testing Data Access Code
Data access code, which includes interactions with databases, file systems, and external services, plays a pivotal role in most applications. Any issues or bugs in this layer can have far-reaching consequences, potentially leading to data corruption, security vulnerabilities, and application instability. Thus, comprehensive testing of data access code is crucial for maintaining the overall health and reliability of an application.
Writing Testable Data Access Code
Before delving into testing strategies, it's essential to emphasize the significance of writing testable data access code. Testability should be a primary consideration during the design and implementation of data access components. By adhering to best practices such as separation of concerns, dependency injection, and use of interfaces, developers can significantly simplify the process of testing data access code.
When it comes to testing database interactions, using an in-memory database, such as H2 or HSQLDB, can streamline the testing process. In-memory databases enable fast and reliable execution of tests without the need for a separate database instance, thereby promoting a more efficient testing workflow.
Unit Testing Data Access Code
Unit testing forms the cornerstone of any robust testing strategy, and the same holds true for data access code. When it comes to unit testing data access code in Java, utilizing tools such as JUnit and Mockito can greatly facilitate the process.
Let's take a look at an example of unit testing a simple UserRepository class using JUnit and Mockito.
public class UserRepositoryTest {
@InjectMocks
private UserRepository userRepository;
@Mock
private EntityManager entityManager;
@Test
public void testFindUserById() {
// Arrange
long userId = 1L;
User expectedUser = new User(userId, "John Doe");
when(entityManager.find(User.class, userId)).thenReturn(expectedUser);
// Act
User actualUser = userRepository.findUserById(userId);
// Assert
assertNotNull(actualUser);
assertEquals(expectedUser.getId(), actualUser.getId());
assertEquals(expectedUser.getName(), actualjsonObject_user.getName());
}
}
In this example, we're using Mockito to mock the EntityManager, allowing us to isolate the UserRepository for testing. By setting up the expected behavior of the EntityManager using when
and specifying the desired return value, we can effectively test the logic within the UserRepository.
Integration Testing Data Access Code
While unit tests focus on isolated components, integration tests are essential for validating the interactions and behavior of different parts of the system, including data access components and their integration with external resources.
For integration testing of data access code that involves database interactions, frameworks such as Spring Boot provide robust support through technologies like @DataJpaTest
and an embedded database configuration. Using an embedded database for integration tests ensures that the tests run reliably and consistently in different environments without relying on a separate database instance.
Here's an example of an integration test for a simple UserRepository using Spring Boot and an embedded H2 database.
@DataJpaTest
public class UserRepositoryIntegrationTest {
@Autowired
private TestEntityManager entityManager;
@Autowired
private UserRepository userRepository;
@Test
public void testFindUserById() {
// Arrange
User expectedUser = new User(1L, "Jane Smith");
entityManager.persistAndFlush(expectedUser);
// Act
User actualUser = userRepository.findUserById(expectedUser.getId());
// Assert
assertNotNull(actualUser);
assertEquals(expectedUser.getId(), actualUser.getId());
assertEquals(expectedUser.getName(), actualUser.getName());
}
}
In this scenario, the @DataJpaTest
annotation configures the application context with a test-specific EntityManager, making it seamless to perform database operations without explicitly managing database connections and transactions.
Testing Data Migration Scripts
In addition to testing data access logic, it's crucial to verify the correctness of data migration scripts when evolving the database schema. Tools like Flyway and Liquibase offer convenient mechanisms for versioning and executing database migrations as part of the application's build process.
By including automated tests for data migration scripts, developers can ensure that database schema changes are applied accurately and do not introduce regressions or data loss. Writing test cases to validate the state of the database before and after running migration scripts is pivotal for maintaining the integrity of the application's data layer.
Continuous Integration and Testing
An integral part of ensuring reliable builds for data access code is integrating testing into the continuous integration (CI) pipeline. Leveraging CI platforms like Jenkins, CircleCI, or GitLab CI/CD enables automated execution of tests upon every code commit, providing prompt feedback to developers and preventing the introduction of faulty data access logic into the codebase.
Integrating code quality analysis tools, such as SonarQube or Checkstyle, into the CI pipeline further enhances the reliability of the build process by identifying potential issues, anti-patterns, or vulnerabilities in the data access code.
The Closing Argument
In conclusion, testing data access code in Java is a critical aspect of building reliable and robust applications. By adopting a comprehensive testing strategy that includes unit testing, integration testing, validation of data migration scripts, and seamless integration with continuous integration pipelines, developers can instill confidence in the correctness and stability of the data access layer.
Writing testable data access code, leveraging appropriate testing frameworks and tools, and integrating testing into the CI pipeline are all essential practices for ensuring the reliability of data access code. Embracing these strategies empowers development teams to deliver high-quality, resilient applications with a well-tested data access layer, ultimately enhancing the overall user experience and minimizing the risk of data-related issues in production.
By prioritizing testing and reliability in data access code, developers pave the way for building applications that inspire trust and perform consistently in demanding real-world scenarios.