Optimizing Session Management with Spring Session
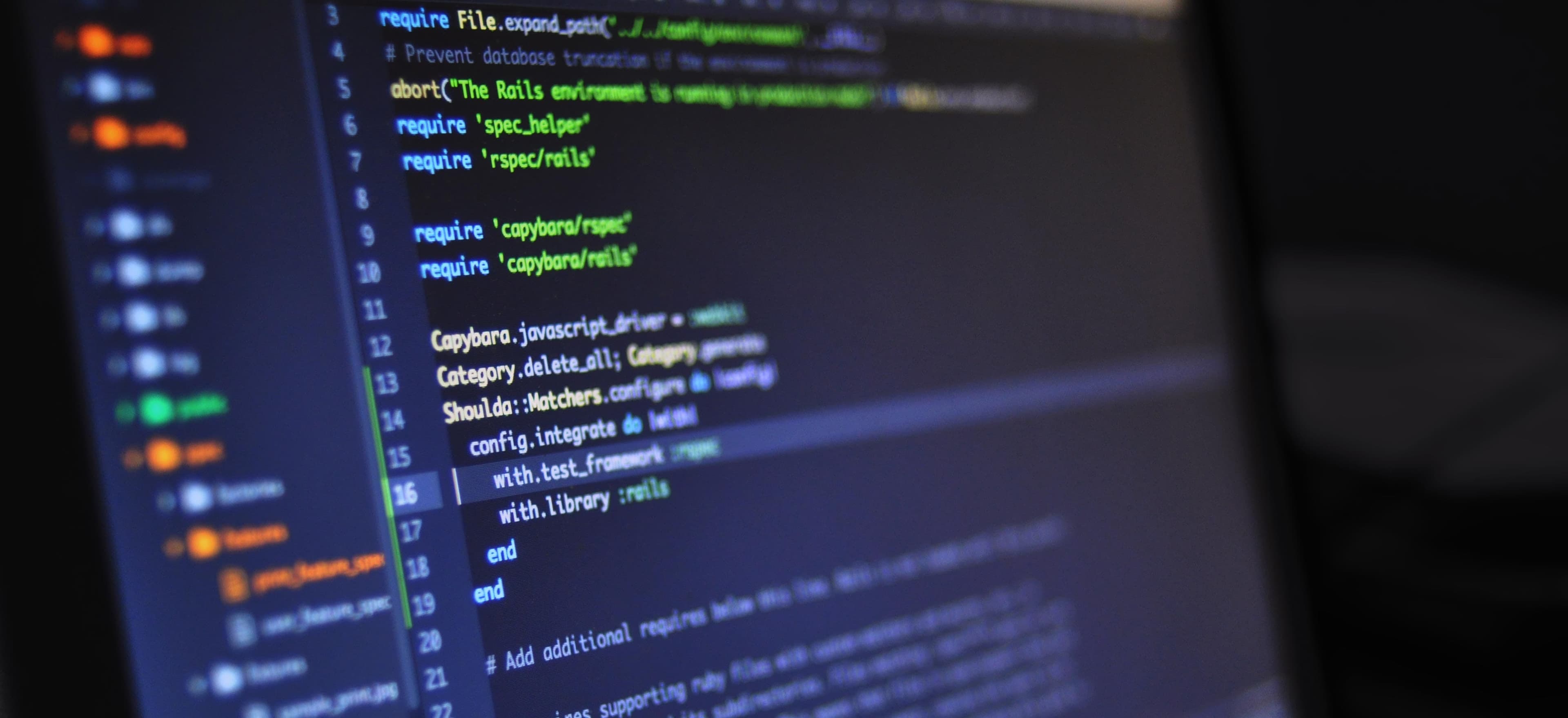
- Published on
Optimizing Session Management with Spring Session
In a web application, session management is a critical aspect that directly affects the user experience and the overall performance of the application. Traditionally, session management in Java web applications relies on the servlet container's default behavior, which involves storing user session data in-memory. However, as the application scales and adopts a distributed architecture, this in-memory storage becomes a bottleneck.
To address the challenges associated with traditional session management, developers can leverage Spring Session, a powerful module within the Spring Framework that provides support for managing user sessions in a distributed environment. Spring Session offers a scalable solution to session management by externalizing the session data storage, thus ensuring that the application can handle a large number of users without compromising performance.
In this article, we'll explore the benefits of using Spring Session for session management and demonstrate how to integrate it into a Java web application.
Benefits of Spring Session
1. Distributed Session Management
Traditional session management stores session data in-memory, which becomes problematic in a distributed system where multiple instances of the application are running. Spring Session addresses this issue by providing pluggable session storage options, such as Redis, MongoDB, and JDBC, enabling distributed session management across all instances of the application.
2. Improved Scalability
By externalizing session data storage, Spring Session allows the application to scale horizontally without worrying about session replication and synchronization issues. This results in improved scalability and better handling of increased user traffic.
3. Reduced Memory Footprint
With in-memory session management, the servlet container allocates memory for each user session, which can lead to high memory usage as the number of active sessions grows. Spring Session mitigates this by offloading session data to an external data store, reducing the memory footprint of the application.
Integrating Spring Session
To integrate Spring Session into a Java web application, follow these steps:
Step 1: Add Spring Session Dependency
Add the Spring Session dependency to your project's build configuration file (e.g., Maven pom.xml
or Gradle build file).
<dependency>
<groupId>org.springframework.session</groupId>
<artifactId>spring-session-core</artifactId>
<version>2.4.2</version>
</dependency>
Step 2: Configure Session Storage
Configure the session storage mechanism by choosing one of the supported data stores (e.g., Redis, MongoDB) and adding its corresponding configuration to the application properties file.
# Example configuration for Redis session storage
spring:
session:
store-type: redis
redis:
namespace: myapp:sessions
flush-mode: on-save
Step 3: Enable Spring Session
Enable Spring Session in the application configuration by annotating the main configuration class with @EnableSpringHttpSession
.
@Configuration
@EnableSpringHttpSession
public class SessionConfig {
// Additional session-related configuration can be added here
}
Step 4: Accessing Session Data
Once Spring Session is integrated, accessing and manipulating session data is seamless. Use the standard HttpSession
interface or Spring's Session
interface to interact with session attributes, just as you would with traditional session management.
@GetMapping("/user/profile")
public String userProfile(HttpSession session) {
// Access session attributes
String username = (String) session.getAttribute("username");
// Manipulate session attributes
session.setAttribute("isLoggedIn", true);
// Perform other session operations
session.invalidate();
return "profile";
}
Wrapping Up
In conclusion, effective session management is crucial for maintaining the stability and performance of a Java web application, especially as the application scales. Spring Session offers a robust solution for managing user sessions in a distributed environment, providing benefits such as distributed session management, improved scalability, and reduced memory footprint.
By integrating Spring Session into your Java web application, you can ensure that session data is efficiently stored and managed, regardless of the application's scale and complexity.
Incorporating Spring Session into your project empowers you to optimize session management with minimal effort, allowing you to focus on building a responsive and scalable web application.
For further exploration of Spring Session and its capabilities, refer to the official documentation.
Ensure to leverage the power of Spring Session to enhance the performance and scalability of your Java web application's session management.
By implementing Spring Session, you can elevate the efficiency and scalability of your Java web application's session management.