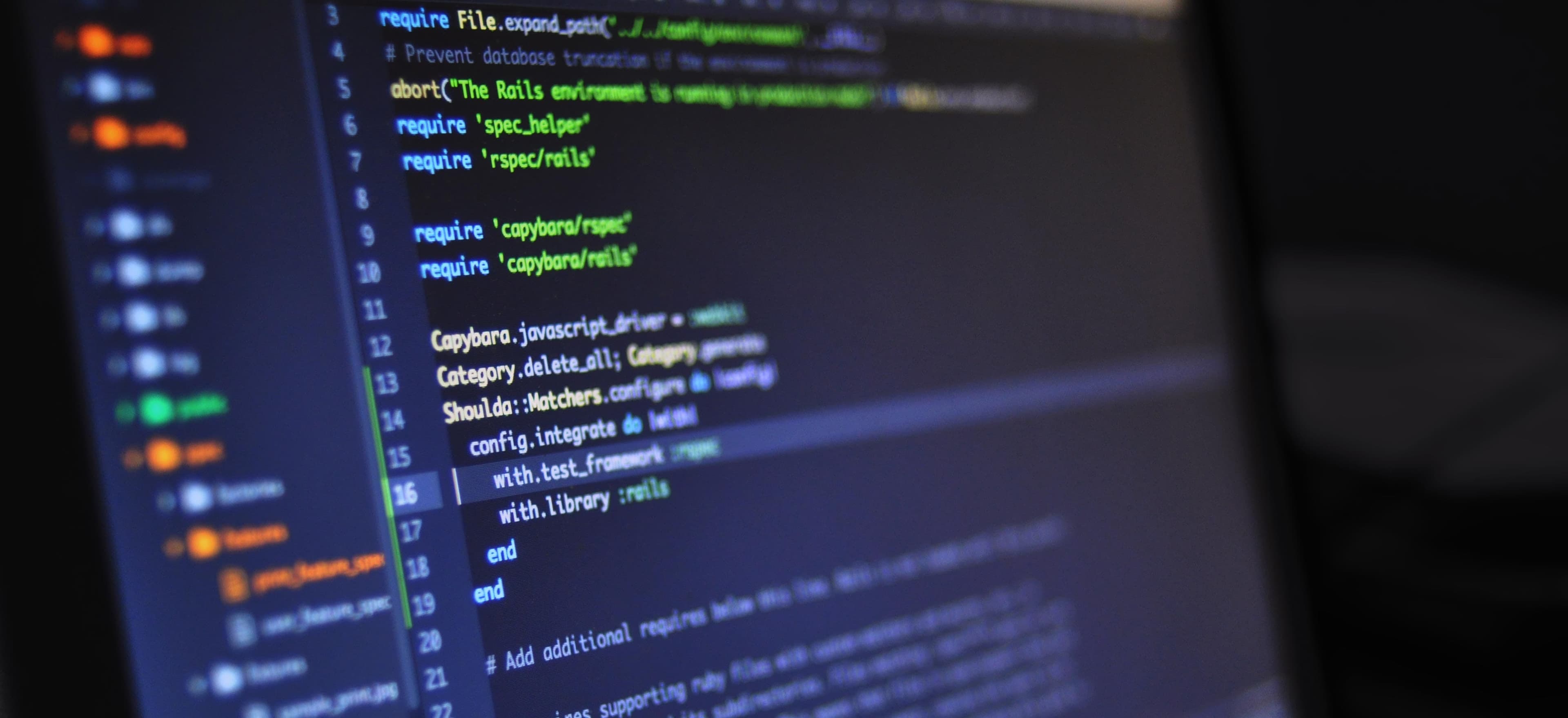
- Published on
Identifying Security Vulnerabilities in Spring MVC Web Apps
When building Spring MVC web applications, security should always be a top priority. With the increasing number of cyber threats, it's crucial to identify and address potential vulnerabilities in your applications. In this blog post, we'll explore some common security vulnerabilities in Spring MVC web apps and how to identify and mitigate them.
1. SQL Injection
SQL injection is a type of security vulnerability that occurs when an attacker is able to manipulate the SQL query in a web application. This can lead to unauthorized access to the database, data manipulation, and in some cases, complete takeover of the application.
How to Identify
One way to identify potential SQL injection vulnerabilities is to look for user inputs that are directly concatenated into SQL queries. For example:
String query = "SELECT * FROM users WHERE username='" + userInput + "'";
In this case, if userInput
is not properly sanitized, it can be manipulated to inject malicious SQL code.
Mitigation
The best way to mitigate SQL injection vulnerabilities in Spring MVC applications is by using parameterized queries or prepared statements. By using PreparedStatement in JDBC or using ORM frameworks like Hibernate, you can prevent attackers from manipulating the SQL queries.
2. Cross-Site Scripting (XSS)
Cross-Site Scripting is another common security vulnerability in web applications, including Spring MVC apps. It occurs when an attacker is able to inject malicious scripts into web pages viewed by other users.
How to Identify
To identify potential XSS vulnerabilities, look for places where user inputs are directly embedded into HTML output without proper encoding. For example:
String userInput = request.getParameter("input");
out.println("Output: " + userInput);
Mitigation
To mitigate XSS vulnerabilities, it's essential to properly encode user inputs before rendering them in HTML. In Spring MVC, you can use libraries like Thymeleaf or JSP's <c:out>
tag to automatically escape user inputs.
3. Cross-Site Request Forgery (CSRF)
Cross-Site Request Forgery is a type of attack that occurs when a user unintentionally performs an action on a different website, usually initiated by a malicious web page.
How to Identify
Identifying CSRF vulnerabilities can be challenging, but a common pattern is to look for actions in your web application that can be triggered via a simple HTTP GET request.
Mitigation
In Spring MVC, you can mitigate CSRF vulnerabilities by using the csrf()
method provided by the WebSecurityConfigurerAdapter
. This method enables CSRF protection by default, adding a unique token to forms and validating it on form submission.
4. Authentication and Session Management
Proper authentication and session management are crucial for securing Spring MVC web applications. Insecure practices in this area can lead to unauthorized access and compromised user data.
How to Identify
Look for instances where authentication and session management are implemented manually, without following best practices.
Mitigation
In Spring MVC, use Spring Security to handle authentication and session management. Spring Security provides robust features for authentication, authorization, and session handling, reducing the risk of common vulnerabilities.
5. Input Validation
Improper input validation can lead to a variety of security vulnerabilities, including SQL injection, XSS, and other injection attacks.
How to Identify
Look for instances where user inputs are not properly validated or sanitized before being used in the application.
Mitigation
In Spring MVC, you can use Hibernate Validator or Spring's validation annotations to enforce proper input validation. By validating user inputs at the controller level, you can prevent many common security vulnerabilities.
Key Takeaways
Securing Spring MVC web applications is an ongoing effort that requires vigilance and regular audits for potential security vulnerabilities. By proactively identifying and mitigating common vulnerabilities such as SQL injection, XSS, CSRF, authentication and session management flaws, and input validation issues, you can significantly reduce the risk of security breaches in your applications.
Remember, staying informed about the latest security best practices and continuously updating your knowledge of security threats is essential for keeping your Spring MVC web apps secure.
Implementing a robust security strategy not only protects your application and its users but also enhances your reputation as a responsible developer in the industry.
For further reading and best practices, you can refer to OWASP Top 10 for a comprehensive understanding of common web application security risks and how to address them in your Spring MVC applications.