Struggling with Type Safety in JPA's Native Queries?
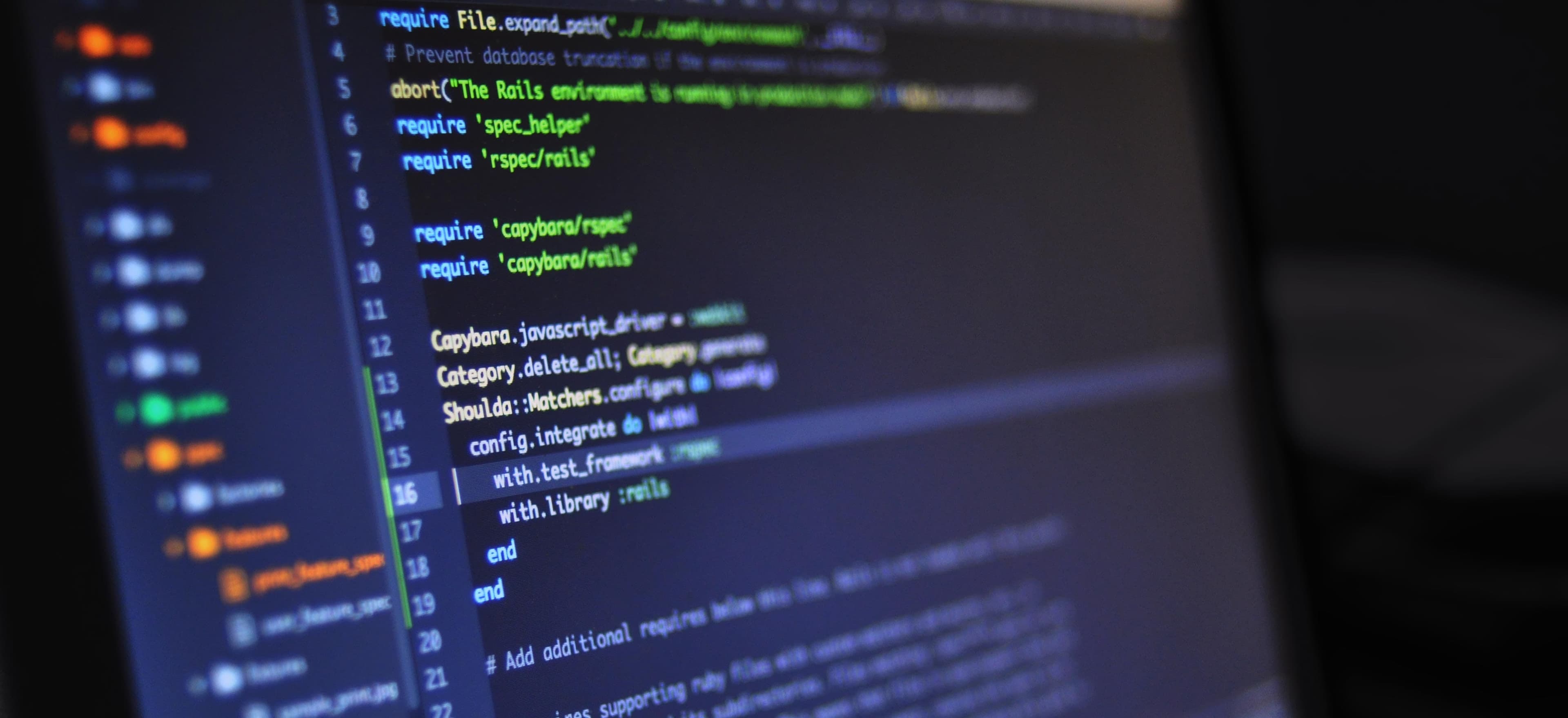
- Published on
Struggling with Type Safety in JPA's Native Queries?
Java Persistence API (JPA) is one of the most powerful frameworks for managing relational data in Java applications. However, developers often encounter challenges related to type safety, particularly when it comes to native queries. In this blog post, we'll explore these challenges and provide practical solutions to enhance type safety.
Understanding JPA and Native Queries
JPA is an abstraction layer over JDBC, designed to simplify database access and reduce boilerplate code. It does this by mapping Java objects to database tables. While JPA provides a rich query language (JPQL), there are situations where you want to leverage native SQL queries. This might be necessary for complex queries, specific database functions, or optimizing performance.
However, native queries pose a critical issue: type safety. When you execute a native SQL query, the mapping between the result set and your entity or DTO objects needs to be carefully managed to avoid runtime exceptions.
What is Type Safety?
Type safety ensures that you only use data types that are valid for a given context in your code. In the context of JPA and native queries, this means ensuring that the results of the query match the expected object structure without casting issues.
The Challenge with Native Queries
When you write a native query:
String sql = "SELECT * FROM users WHERE age > ?";
Query query = entityManager.createNativeQuery(sql);
query.setParameter(1, 18);
List<Object[]> results = query.getResultList();
You might find yourself dealing with an array of Object
types. This can lead to:
-
Runtime Exceptions: If you expect a specific type but the data retrieved does not match, it could lead to a
ClassCastException
. -
Verbose Code: You may end up with code that's harder to read and maintain due to repeated casting.
-
Limited IDE Support: IDEs may not provide suggestions or warnings about potential issues, leading to bugs that could be caught at compile time.
Solutions to Enhance Type Safety
Let's explore several strategies to improve type safety when using native queries in JPA.
1. Using Entity Graphs
By leveraging JPA entity graphs, you can define a blueprint for how your entities should be populated, even when using native queries. Although this doesn't directly enforce type safety, it provides a structured way to fetch data.
Example:
@Entity
@NamedEntityGraph(name = "User.detail", attributePaths = {"roles", "profile"})
public class User {
// fields, getters, setters
}
This way, if you use a native query to retrieve users, you can reference the specific attributes you need.
2. Mapping Results Directly to DTOs
Instead of returning raw Object[]
arrays, you can map results directly to Data Transfer Objects (DTOs). For this, you can use the new
keyword within your SQL statement or a more explicit result transformer.
Example DTO Class:
public class UserDTO {
private String username;
private String email;
// Constructor, getters, setters
public UserDTO(String username, String email) {
this.username = username;
this.email = email;
}
}
Modified Query:
String sql = "SELECT new com.example.dto.UserDTO(u.username, u.email) FROM users u WHERE u.age > ?1";
List<UserDTO> users = entityManager.createQuery(sql, UserDTO.class)
.setParameter(1, 18)
.getResultList();
This approach leverages type safety while allowing you to define explicit structures for your results.
3. Using SQLResultSetMapping
Another solution is to use @SqlResultSetMapping
, which allows you to define how the results of the native query should be mapped to your entities or DTOs.
Example:
@SqlResultSetMapping(
name = "UserDTOMapping",
classes = @ConstructorResult(
targetClass = UserDTO.class,
columns = {
@ColumnResult(name = "username"),
@ColumnResult(name = "email")
}
)
)
@Entity
public class User {
// fields, getters, setters
}
Then you can execute the native query as follows:
String sql = "SELECT username, email FROM users WHERE age > ?";
List<UserDTO> users = entityManager.createNativeQuery(sql, "UserDTOMapping")
.setParameter(1, 18)
.getResultList();
This method provides a clear mapping configuration, resulting in better type safety.
4. Strongly Typed Projections
For even better type safety, consider using a JPA criteria query projection if applicable to your use-case. This may not always suit native queries; however, keep it in mind when building more complex queries.
Example:
CriteriaBuilder cb = entityManager.getCriteriaBuilder();
CriteriaQuery<UserDTO> cq = cb.createQuery(UserDTO.class);
Root<User> userRoot = cq.from(User.class);
cq.select(cb.construct(UserDTO.class, userRoot.get("username"), userRoot.get("email")))
.where(cb.greaterThan(userRoot.get("age"), 18));
List<UserDTO> users = entityManager.createQuery(cq).getResultList();
Using the criteria API allows you to leverage compile-time checks for your queries, significantly increasing type safety.
Final Considerations
Type safety in JPA native queries doesn’t have to be a source of frustration. By implementing the strategies we discussed, from leveraging DTOs and result set mappings to exploring entity graphs and criteria queries, you can enhance type safety and make your code more robust and maintainable.
Understanding and addressing these challenges will not only lead to fewer bugs but will enhance the overall quality of your Java application. For further reading on JPA and native queries, consider the official Java EE documentation and Spring Data JPA documentation.
With these tools in your toolkit, you can navigate native queries with confidence!
Checkout our other articles