Mastering Regex: Common Pitfalls in Java Pattern Matching
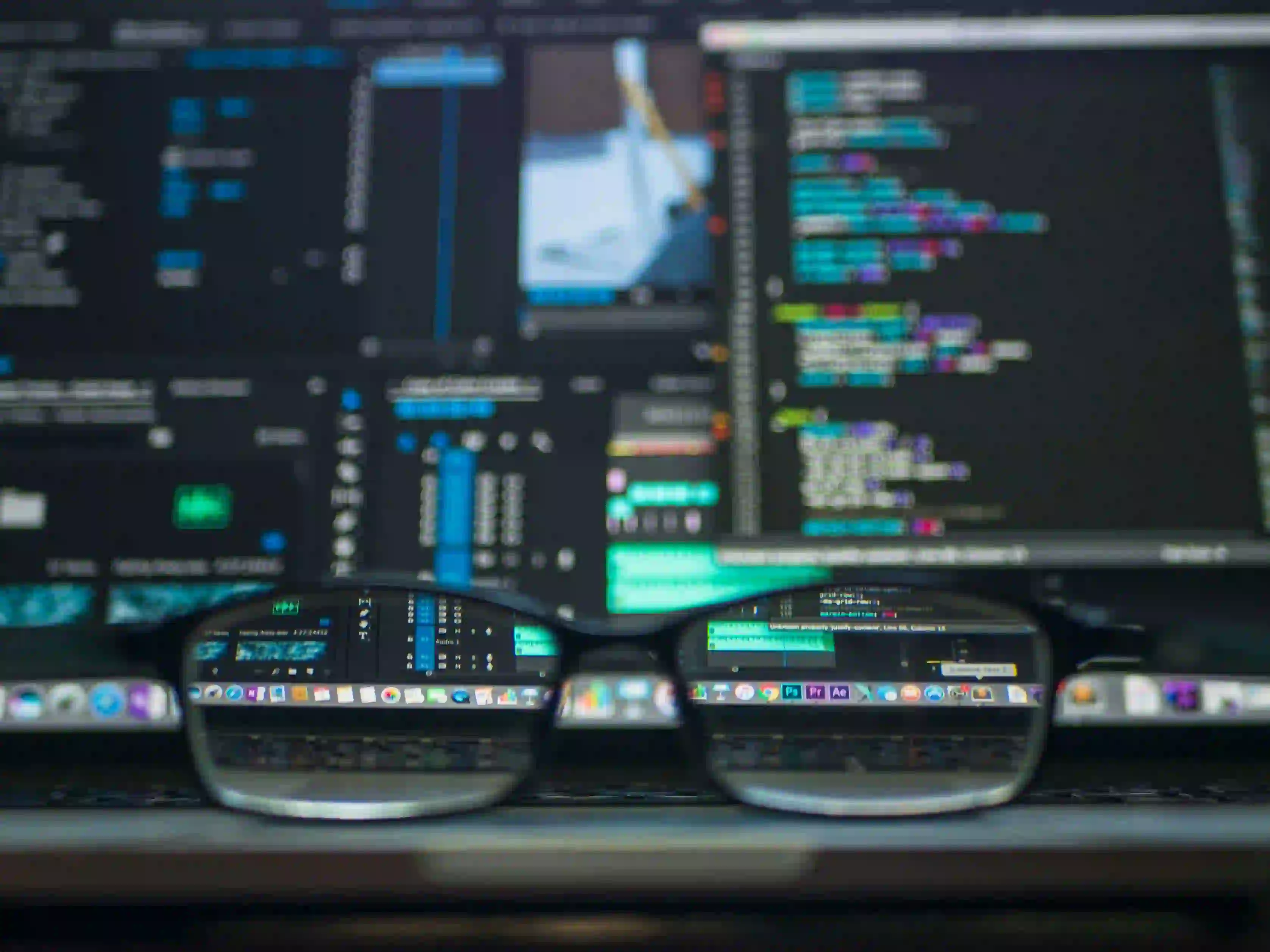
Mastering Regex: Common Pitfalls in Java Pattern Matching
Regular expressions (regex) are powerful tools for text processing and manipulation in Java. They enable developers to search, match, and handle string data effectively. However, they are also famously intricate and prone to errors. In this blog post, we will explore common pitfalls in Java pattern matching using regex and how to avoid these issues. Maintain clarity through concise examples, and by the end, you will have a robust understanding of regex in Java.
What is Regex?
A regular expression is a sequence of characters that forms a search pattern. It can be used for string searching algorithms for "find" or "find and replace" operations on strings. In Java, regex is used through the java.util.regex
package, which provides a powerful way to request complex string manipulations.
The Basics of Regex in Java
Importing the Regex Package
Before getting into more complex examples, let's ensure you know how to start with regex in Java:
import java.util.regex.Pattern;
import java.util.regex.Matcher;
These imports allow you to compile regex patterns (Pattern
) and match those patterns against strings (Matcher
).
A Simple Example
Here’s a straightforward example to get you started:
String text = "Hello, World!";
Pattern pattern = Pattern.compile("Hello");
Matcher matcher = pattern.matcher(text);
if (matcher.find()) {
System.out.println("Match found!");
} else {
System.out.println("No match found.");
}
This code checks if the string text
contains the word "Hello". If found, it prints "Match found!".
Why is Regex Complexity a Common Pitfall?
Regex can get complicated due to its syntax and various quantifiers. Misunderstanding these can lead to unexpected results. Let’s dive into some common pitfalls.
Common Pitfalls in Java Regex
1. Overusing Wildcards
The dot (.
) is a wildcard that matches any character except line terminators. For example, the regex a.b
would match aXb
, aYb
, or a b
. However, using it excessively can lead to unexpected matches.
Example:
String text = "a b c a.d e";
Pattern pattern = Pattern.compile("a.b");
Matcher matcher = pattern.matcher(text);
while (matcher.find()) {
System.out.println("Found: " + matcher.group());
}
Output:
Found: a b
Found: a.d
Why It Matters:
While this matches more than expected, you might only need specific patterns. If you strictly want "a" followed directly by "b", use a_b
.
2. Neglecting Escape Sequences
In regex, some characters are reserved (like .
, *
, +
, etc.), and if you want to match these characters, they need to be escaped with a backslash (\
). Failing to do so is a common pitfall.
Example:
String text = "1 + 1 = 2";
Pattern pattern = Pattern.compile("1 + 1");
Matcher matcher = pattern.matcher(text);
System.out.println(matcher.find()); // This will return false
This won't find a match due to the lack of escape sequences around the +
operator.
Corrected code:
Pattern pattern = Pattern.compile("1 \\+ 1");
Why It Matters: Escape sequences are crucial for accurate matching involving special characters.
3. Forgetting to Specify Quantifiers
Quantifiers like *
(zero or more) and +
(one or more) define how many instances of a character or group must be matched. Forgetting these can yield incorrect matches.
Example:
String text = "aaaab";
Pattern pattern = Pattern.compile("ab");
Matcher matcher = pattern.matcher(text);
System.out.println(matcher.find()); // This will return false
To correct this, consider the quantifier:
Pattern pattern = Pattern.compile("a+b");
Why It Matters: Understanding quantifiers allows for precise string matching.
4. Using Case-Sensitive Matching
By default, regex is case-sensitive. Therefore, if your text contains varied case patterns, you might miss out on matches.
Example:
String text = "Hello World";
Pattern pattern = Pattern.compile("hello");
Matcher matcher = pattern.matcher(text);
System.out.println(matcher.find()); // This will return false
Solution:
You can modify the pattern to be case-insensitive:
Pattern pattern = Pattern.compile("hello", Pattern.CASE_INSENSITIVE);
5. Not Utilizing Character Classes
Using [ ]
in regex allows you to specify a set of characters to match. Neglecting to use character classes can lead to overly complex patterns.
Example:
String text = "cat bat mat";
Pattern pattern = Pattern.compile("c|b|m");
Matcher matcher = pattern.matcher(text);
while (matcher.find()) {
System.out.println("Found: " + matcher.group());
}
Output:
Found: c
Found: b
Found: m
Instead, you can condense it to:
Pattern pattern = Pattern.compile("[cbm]at");
Why It Matters: Character classes can greatly simplify your regex patterns.
Advanced Tips for Regex in Java
Compile Once, Use Multiple Times
To optimize performance, compile a regex pattern once and reuse the Pattern
object. This is especially important in a loop.
Pattern pattern = Pattern.compile("your-regex-pattern");
for (String str : stringsToMatch) {
Matcher matcher = pattern.matcher(str);
// Perform matching
}
Use Verbose Mode for Clarity
For complex expressions, consider using the (?x)
mode, which allows for comments and whitespace within regex patterns for better readability.
Example:
String regex = "(?x) # Match a word\n"
+ "([a-zA-Z]+) # Alphanumeric characters";
This comment explains what each section of the regex does.
In Conclusion, Here is What Matters
Mastering regex is a journey through a landscape filled with pitfalls. By understanding the common pitfalls discussed in this post, you can sharpen your regex skills and avoid frustrating errors. Regex is more than just matching patterns—it's about understanding the nuance of pattern definitions.
To further your knowledge, consider reading Java Pattern Matching Documentation and practice using online regex testers. The deeper you dive into the intricacies of regex, the more effective you'll become at text processing in Java. Happy coding!