Overcoming Communication Hurdles in Pair Programming
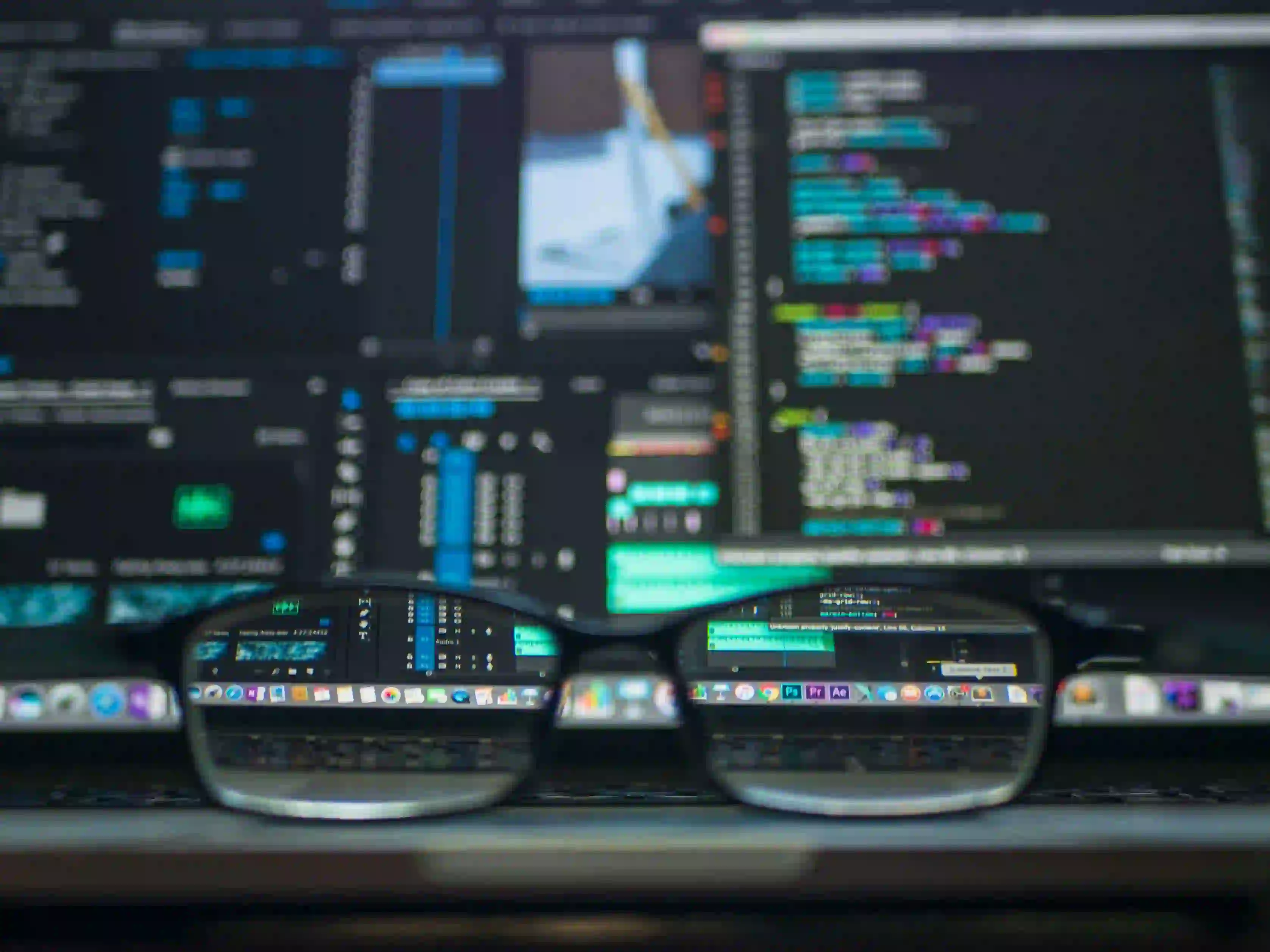
Overcoming Communication Hurdles in Pair Programming
Pair programming is a vital practice in the Agile software development methodology. It involves two developers collaborating on the same code to leverage each other's strengths and improve the code quality. However, effective communication often becomes a hurdle, leading to frustrations and inefficiencies. In this blog post, we will explore common communication barriers in pair programming and practical techniques to overcome them.
Understanding Pair Programming
Before we delve into communication challenges, it's important to understand what pair programming entails. In simple terms, it is a software development technique where two programmers work together on the same task at one computer. One assumes the role of the "driver," writing the code, while the other takes on the role of the "navigator," reviewing the work and providing suggestions.
This collaboration can significantly enhance developers' work quality through collective ownership and immediate feedback. To glean the full benefits of this practice, however, clear communication is essential.
Common Communication Challenges
While pair programming can be rewarding, it presents several communication hurdles:
-
Differing Skill Levels: Pairing a senior developer with a junior can lead to frustration if the duo does not communicate effectively. The junior might feel overwhelmed or incapable, while the senior might struggle to convey complex ideas simply.
-
Personality Clashes: Each developer has a unique personality and work preference. Sometimes these differences can lead to conflict or miscommunication.
-
Distractions: The programming environment often includes external distractions - workstation clutter, alerts, unexpected interruptions - that can lead to fragmented communication.
-
Language Barriers: In diverse teams, language proficiency can sometimes hinder effective communication. Some team members might struggle to articulate their thoughts clearly.
With this foundation in mind, let's discuss strategies to tackle these challenges head-on.
Strategies to Overcome Communication Hurdles
1. Establish Clear Objectives
To reduce confusion, it is vital to set clear goals before starting a pair programming session. Discuss what you plan to achieve and break down the tasks accordingly. This step will help both team members stay focused on the objective.
Example 1: Setting Goals
// Example for a pair programming session
// Developers Alice and Bob are working on implementing a new feature
// Before starting, they agree on the following goals:
1. Define the outline of the feature.
2. Create and review the code for data retrieval.
3. Write unit tests for the newly created functionality.
4. Review and document the code together for future reference.
2. Emphasize Active Listening
Active listening is a fundamental skill in effective communication. Encourage both partners to listen closely, avoid interrupting each other, and clarify misunderstandings. This attentiveness fosters trust and collaboration.
Example 2: Active Listening
public class Listener {
private String thoughts;
public void listen(String idea) {
// Acknowledge the speaker's input
System.out.println("I appreciate your idea: " + idea);
// Clarify if unsure
if (thesisIsUnclear(idea)) {
System.out.println("Can you elaborate on that?");
}
// Reflect back to confirm understanding
thoughts = reflect(idea);
}
}
3. Utilize Visual Aids
Visual aids such as sketches, diagrams, flowcharts, or even whiteboards can facilitate discussions. They help convey complex ideas more clearly and engage both developers in collaborative problem-solving.
Example 3: Drawing a Flowchart
When discussing an algorithm, sketch out its flow on a shared whiteboard or an online tool like Lucidchart. For instance, if you're implementing a login system, create a flowchart that outlines the process:
1. User enters credentials
2. System validates input
3. If valid, user is authenticated
4. If invalid, display error message
4. Pair Dynamics
Different pair combinations can produce varying results. If personality clashes occur, switch partners to maintain a more harmonious workflow. Ultimate success in pair programming often comes down to working well together.
Example 4: Switching Pairs
// When face difficulties due to personality clashes
// The team should:
1. Facilitate a pair rotation schedule.
2. Encourage feedback on pair dynamics.
3. Stay open to adjustments for optimizing collaboration.
5. Regular Check-Ins
To promote accountability, conduct regular check-ins throughout the session. This practice allows developers to express thoughts on the current status of the project and ensures that both members are on the same page.
Example 5: Check-in Procedure
// Implement a check-in every 30 minutes
// Ask each partner:
1. What have you learned so far?
2. Are there any obstacles you're facing?
3. What are our next steps?
6. Foster an Open Environment
Create a non-judgmental atmosphere where feedback is constructive rather than critical. Encouraging open discussions about mistakes or misunderstandings facilitates learning and growth.
Example 6: Promoting Openness
public class OpenEnvironment {
private List<String> feedbackList = new ArrayList<>();
public void addFeedback(String feedback) {
// Store constructive feedback
feedbackList.add(feedback);
System.out.println("Feedback added: " + feedback);
}
public void shareFeedback() {
// Go through feedback constructively
for (String feedback : feedbackList) {
System.out.println("Let's review: " + feedback);
}
}
}
7. Leverage Pair Programming Tools
Technology can be your ally. Use tools like Visual Studio Code Live Share, GitHub, or Google Docs for collaborative coding and documentation. This promotes real-time communication, irrespective of physical location.
The Last Word
Pair programming is a collaborative method that can enhance productivity and code quality, but communication challenges can arise if not addressed proactively. By setting clear objectives, fostering an open environment, utilizing visual aids, and emphasizing active listening, you can significantly improve communication between developers.
In a time where remote collaboration is becoming the norm, mastering these techniques can help you maximize the benefits of pair programming and achieve your project goals efficiently.
For further reading on improving communication and collaboration in Agile methodologies, check out Scrum Alliance and Atlassian Agile Coach.
Happy coding!