Common Mistakes in Java Data Type Identification
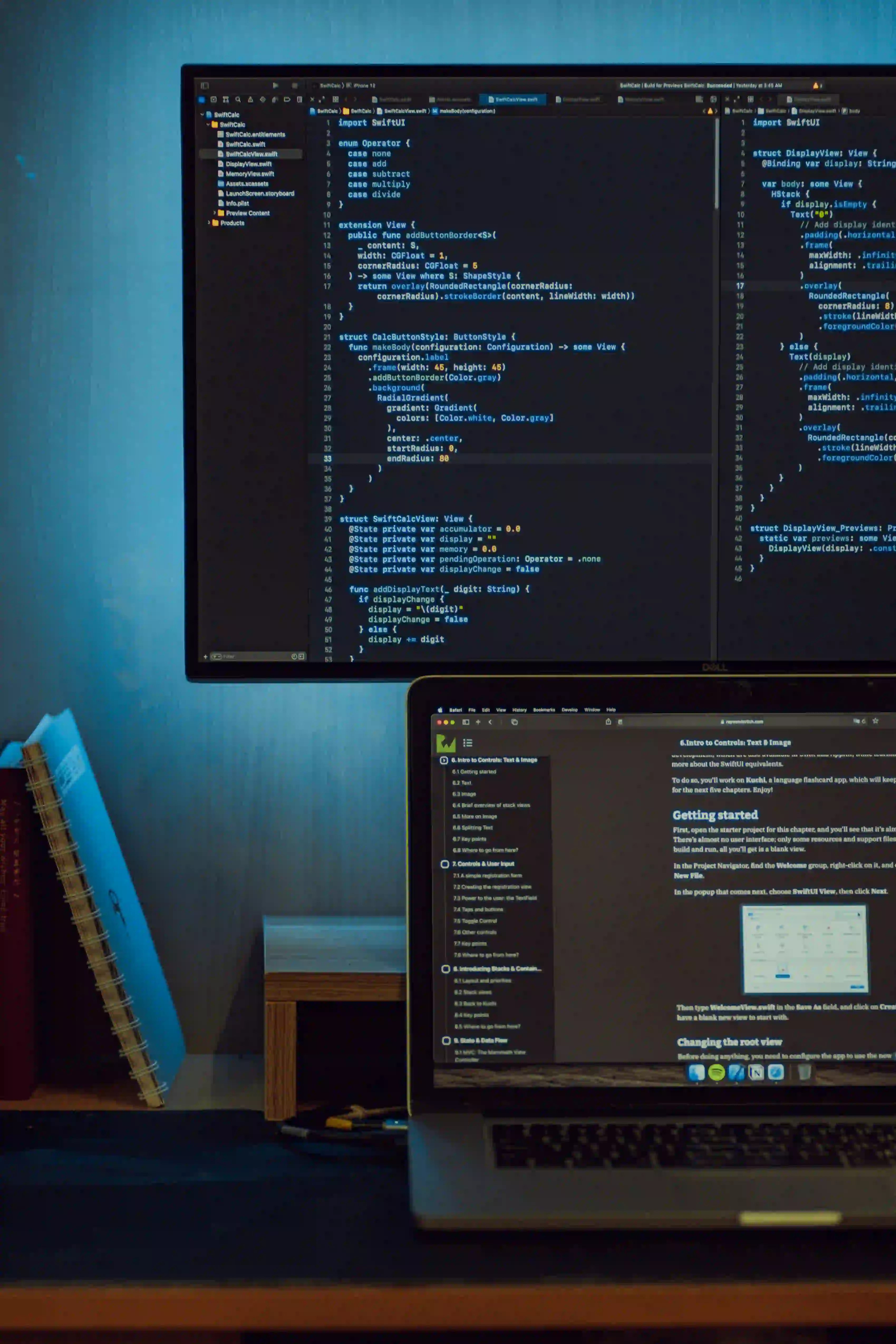
Common Mistakes in Java Data Type Identification
Java is a strongly typed language, meaning that every variable must have a declared data type. Choosing the correct data type is crucial for efficient memory management, preventing errors, and improving the performance of your applications. Despite its importance, many developers—both beginners and experienced—make common mistakes when identifying the correct data type. In this post, we will explore those pitfalls and how to avoid them.
Understanding Java Data Types
Before delving into the common mistakes, let’s quickly review what data types exist in Java. Data types can be categorized into two main groups:
-
Primitive Data Types: These are the basic building blocks of data manipulation in Java. They include:
int
: Integer valuesfloat
: Decimal values with single precisiondouble
: Decimal values with double precisionchar
: Single 16-bit Unicode characterboolean
: Represents true or false
-
Reference Data Types: These refer to objects and are more complex. Common reference data types include:
String
: A sequence of characters- Arrays
- Classes
Common Mistakes in Java Data Type Identification
1. Using the Wrong Primitive Type
One significant mistake developers make is choosing the incorrect primitive data type. For instance, many beginner programmers assume int
can accommodate any number.
Example:
int number = 3000000000; // Incorrect! This exceeds the maximum limit of int.
Why is this wrong?
An int
can hold values in the range of -2,147,483,648 to 2,147,483,647. In this case, utilizing long
, which can accommodate larger numbers, is necessary.
long number = 3000000000L; // Correct.
Use the long
type when you expect values outside the int
range. This understanding helps ensure that your variables can handle any inputs safely.
2. Neglecting Floating-point Precision
Another common pitfall involves neglecting the precision of floating-point types. Java provides both float
(single precision) and double
(double precision) types, but many developers use them interchangeably without understanding their differences.
Example:
float price = 10.5; // Incorrect! Literal is treated as double by default.
Why is this wrong?
The above code will lead to a compilation error since float
requires a literal suffixed by f
or F
.
float price = 10.5f; // Correct.
Whenever you're handling monetary computations or other scenarios requiring precision, prefer double
to minimize rounding errors.
3. Confusing Character and String Types
Often, programmers mistakenly use String
when only a single character is needed. The char
data type is designed to hold single characters.
Example:
String letter = 'A'; // Incorrect! Single quotes denote a char, not a String.
Why is this wrong?
In Java, String
literals require double quotes. Thus, the right declaration would be:
char letter = 'A'; // Correct.
Using the correct type can save memory and improve performance. When only a single character is required, use char
; reserve String
for sequences of characters.
4. Failing to Initialize Variables
Initialization is often overlooked, leading to messy errors and bugs. Java does not allow the use of uninitialized variables.
Example:
int value;
System.out.println(value); // Compile-time error since 'value' is not initialized.
Why is this wrong?
Uninitialized variables pose significant risks and can lead to NullPointerException
in the case of reference types.
int value = 0; // Correct initialization.
Always ensure that your variables are initialized before use. This practice can prevent unexpected behavior in your applications.
5. Overcomplicating Data Type Choices
Sometimes, developers overcomplicate their choices by using complex data types without necessity. For simple applications, utilizing entities like List
or custom classes may be excessive.
Example:
List<Integer> list = new ArrayList<>(); // Overkill for a single integer value.
Why is this wrong?
In this instance, a simple int
would suffice.
int simpleValue = 42; // Correct choice.
For straightforward scenarios, stick to primitive types whenever possible. This approach keeps your code cleaner and facilitates better performance.
Best Practices for Data Type Identification
- Understand the Range: Recognize the limits of the different primitive types and choose accordingly.
- Use the Right Type for the Job: Choose the simplest type that meets your requirements.
- Be Intentional in Initialization: Initialize all variables upon declaration to avoid unintentional consequences.
- Consider Performance: Using primitive types can enhance performance in scenarios where memory usage is critical.
- Follow Naming Conventions: Using descriptive variable names can make your code easier to navigate.
The Bottom Line
In summary, identifying the right data type in Java is crucial for building efficient, reliable applications. Understanding the distinction between data types, knowing their limitations, and avoiding common mistakes can significantly improve your coding style and application performance.
For more insights into Java, check out the Java Tutorials and explore the Java Platform, Standard Edition Documentation. These resources can provide additional guidance as you continue to refine your Java programming skills.
By implementing the strategies discussed, you can mitigate the common mistakes related to Java data type identification and lay a solid foundation for successful programming in Java. Happy coding!