Common Builder Pattern Pitfalls in JUnit Testing
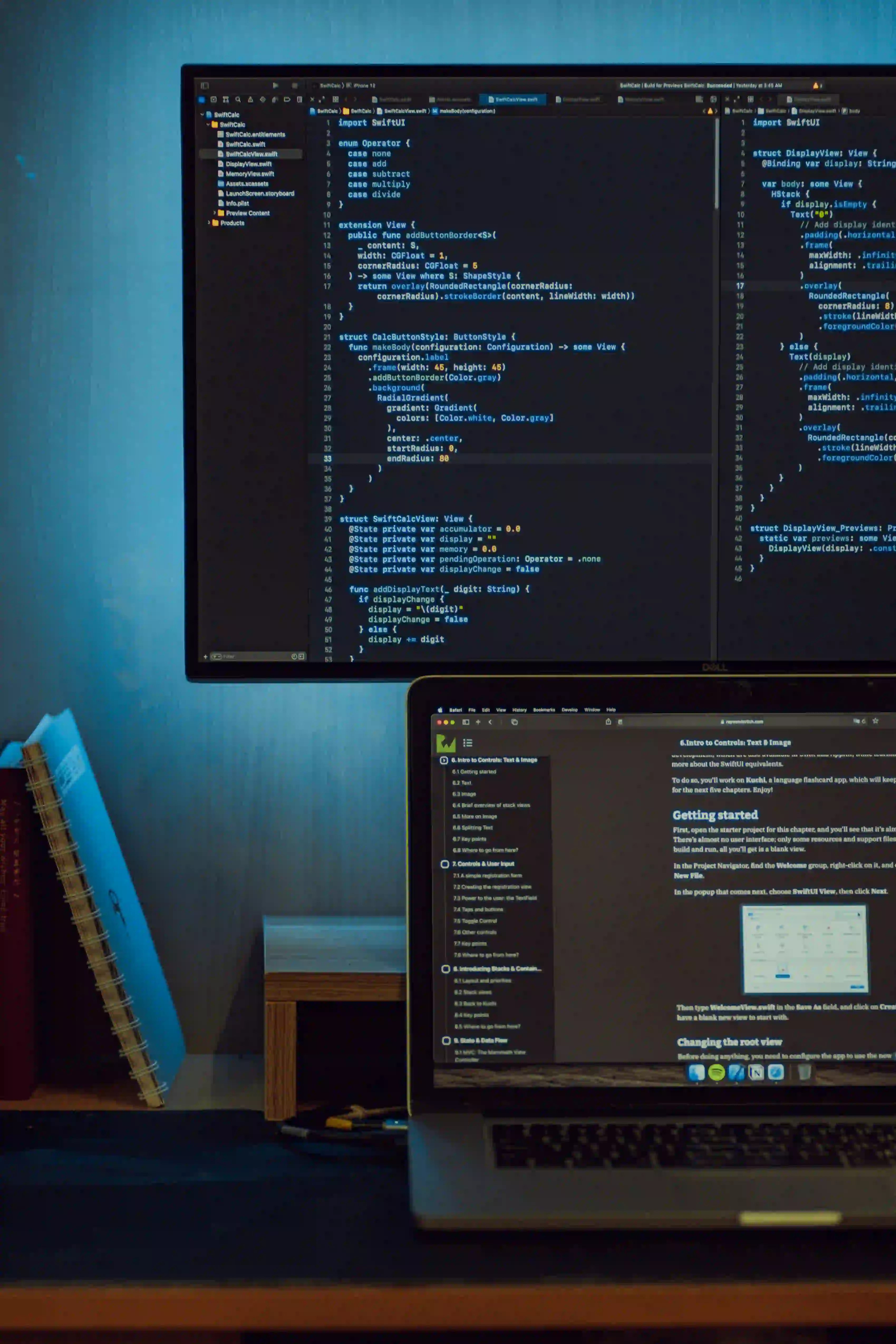
Common Builder Pattern Pitfalls in JUnit Testing
When it comes to software design patterns, the Builder Pattern stands out as a powerful mechanism for constructing complex objects. In cohesive environments like Java, it allows developers to create objects step by step, ensuring that they can set optional parameters only when necessary. However, leveraging this pattern can lead to unexpected challenges, especially when you're writing JUnit tests. In this blog post, we will explore common pitfalls you may encounter while implementing the Builder Pattern in your tests and how to effectively navigate those pitfalls.
Understanding the Builder Pattern
The Builder Pattern separates the construction of a complex object from its representation, thus allowing you to create different representations of an object using the same construction process. Here’s a simplified example to depict its utility:
public class User {
private String username;
private String email;
private String phone;
private User(Builder builder) {
this.username = builder.username;
this.email = builder.email;
this.phone = builder.phone;
}
public static class Builder {
private final String username; // Required
private String email; // Optional
private String phone; // Optional
public Builder(String username) {
this.username = username;
}
public Builder email(String email) {
this.email = email;
return this;
}
public Builder phone(String phone) {
this.phone = phone;
return this;
}
public User build() {
return new User(this);
}
}
}
The User
class has mandatory username
and optional email
and phone
. Concrete usage can look as follows:
User user = new User.Builder("john_doe")
.email("john@example.com")
.build();
While this pattern clarifies and illuminates object construction, developers often face several pitfalls when applying it in unit tests.
Pitfall 1: Overlooking Immutability and Side Effects
One common issue arises when mutable objects are passed as parameters into the Builder. If modifications occur after the object has been built, it might unintentionally affect other tests that rely on a previous state.
Example:
public class UserTest {
@Test
public void testUserBuilder() {
User.Builder builder = new User.Builder("john_doe");
String email = "john@example.com";
User user1 = builder.email(email).build();
// Email reference is now interchangeable.
email = "jane@example.com"; // Unintentionally modifies the original email.
User user2 = builder.email(email).build(); // user2 has the modified email: jane@example.com
}
}
Fix:
Always use immutable data types or deep copies when passing mutable parameters. This ensures that the state remains stable:
public class UserTest {
@Test
public void testUserBuilder() {
User user1 = new User.Builder("john_doe")
.email("john@example.com")
.build();
User user2 = new User.Builder("john_doe")
.email("jane@example.com")
.build();
assertNotEquals(user1.getEmail(), user2.getEmail());
}
}
Pitfall 2: Inconsistent Object State
Another pitfall involves creating builders that might enter inconsistent states. If a builder is used across multiple test cases without resets, it can lead to confusion and failed tests.
Example:
public class UserTest {
private User.Builder builder;
@Before
public void setUp() {
builder = new User.Builder("john_doe");
}
@Test
public void testUserBuilderWithEmail() {
User user = builder.email("john@example.com").build();
assertEquals("john@example.com", user.getEmail());
}
@Test
public void testUserBuilderWithPhone() {
User user = builder.phone("123456789").build(); // Uses previous email
assertNull(user.getEmail()); // This might fail due to previous email setting.
}
}
Fix:
Create a fresh builder instance for each test case. This ensures a consistent state in each test:
public class UserTest {
@Test
public void testUserBuilderWithEmail() {
User user = new User.Builder("john_doe")
.email("john@example.com")
.build();
assertEquals("john@example.com", user.getEmail());
}
@Test
public void testUserBuilderWithPhone() {
User user = new User.Builder("john_doe")
.phone("123456789")
.build();
assertNull(user.getEmail());
}
}
Pitfall 3: Ignoring Builder Validation
Sometimes, validation that checks for proper properties of the object can be overlooked. In critical scenarios, failing to validate can lead to the creation of invalid domain objects.
Example:
public class User {
// existing fields …
public static class Builder {
// existing fields …
public User build() {
if (username == null || username.isEmpty()) {
throw new IllegalArgumentException("Username is required");
}
return new User(this);
}
}
}
Test it:
While creating a User
, make sure validations are respected in a unit test.
public class UserTest {
@Test(expected = IllegalArgumentException.class)
public void testUserBuilderMissingUsername() {
new User.Builder("").build(); // should throw an exception
}
// More tests ...
}
By incorporating validation into your builder, you enhance robustness and maintainability.
Pitfall 4: Not Leveraging Parameterized Tests
When testing multiple configurations of a builder, developers often write multiple method implementations, which can lead to duplication. Instead, parameterized tests are valuable.
Example:
Without parameterized tests:
public class UserTest extends BaseTest {
@Test
public void testUserBuilderWithDifferentEmails() {
User user1 = new User.Builder("john_doe")
.email("john@example.com")
.build();
User user2 = new User.Builder("john_doe")
.email("jane@example.com")
.build();
assertNotEquals(user1.getEmail(), user2.getEmail());
}
@Test
public void testUserBuilderWithDifferentPhones() {
User user1 = new User.Builder("john_doe")
.phone("123456789")
.build();
User user2 = new User.Builder("john_doe")
.phone("987654321")
.build();
assertNotEquals(user1.getPhone(), user2.getPhone());
}
}
Fix:
Utilize JUnit's parameterized tests to consolidate your tests:
@RunWith(Parameterized.class)
public class UserTest {
@Parameterized.Parameter(0)
public String userEmail;
@Parameterized.Parameter(1)
public String expectDifferentEmail;
@Parameterized.Parameters
public static Object[][] data() {
return new Object[][] {
{"john@example.com", "jane@example.com"},
{"alice@example.com", "bob@example.com"},
};
}
@Test
public void testUserBuilderWithDifferentEmails() {
User user1 = new User.Builder("john_doe")
.email(userEmail)
.build();
User user2 = new User.Builder("john_doe")
.email(expectDifferentEmail)
.build();
assertNotEquals(user1.getEmail(), user2.getEmail());
}
}
Final Considerations
The Builder Pattern is a well-designed approach to simplify the construction of complex objects. However, it also presents unique challenges, especially in test scenarios. By recognizing and addressing common pitfalls such as mutability issues, inconsistent states, validation oversights, and the importance of using parameterized tests, developers can leverage this pattern more effectively.
For more comprehensive information on JUnit testing strategies and the Builder Pattern, consider checking out JUnit 5 Documentation and Effective Java, which provide extensive insights on handling situations effectively.
Now that you're aware of these pitfalls, you're better prepared to utilize the Builder Pattern and ensure reliable and maintainable code that works harmoniously with your JUnit tests. Happy coding!