Why Developers Struggle to Keep Up with JDK 17 Features
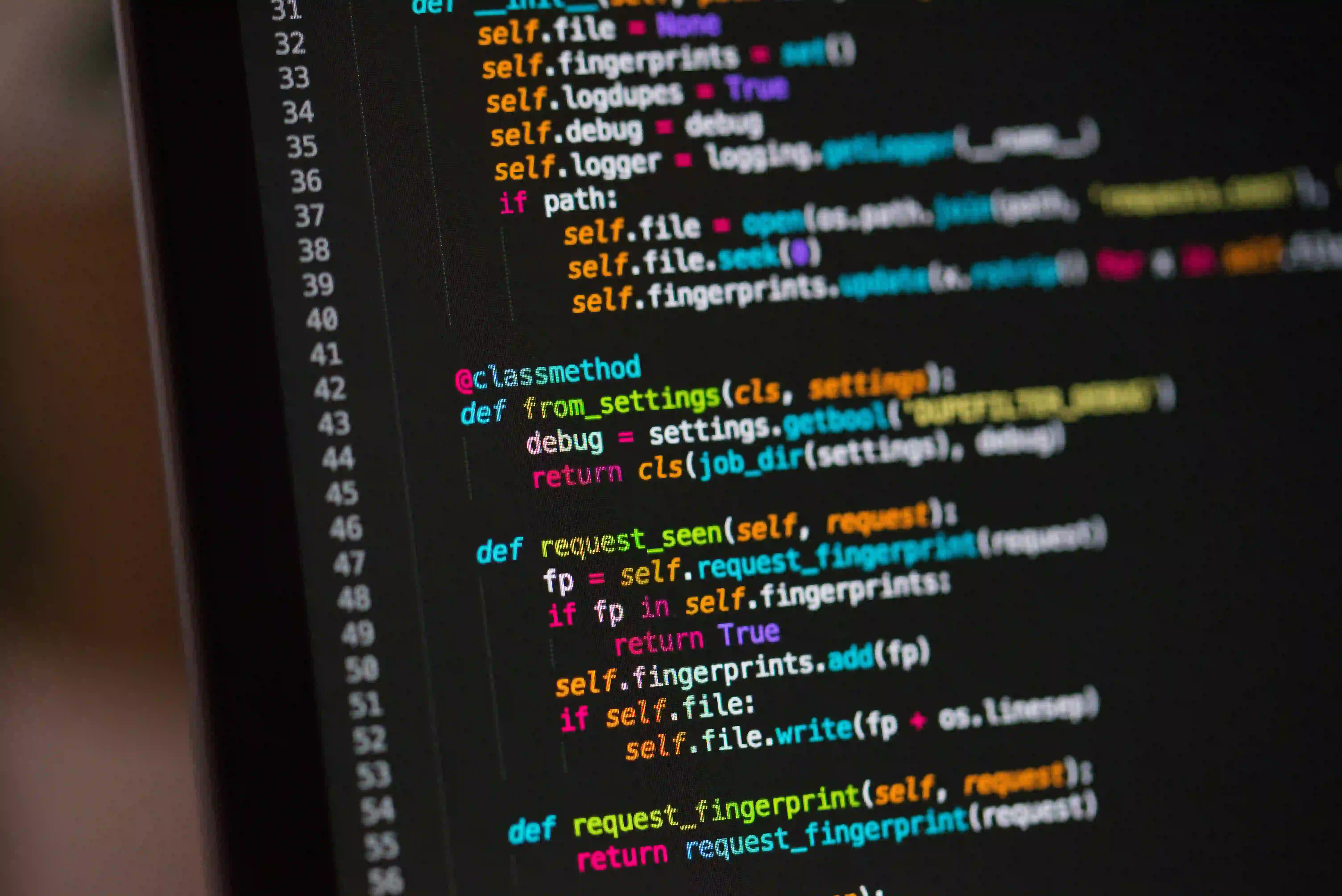
Why Developers Struggle to Keep Up with JDK 17 Features
Java Development Kit (JDK) 17 was released in September 2021, bringing a slew of impressive new features that can significantly improve the development experience. However, many developers struggle to keep pace with these advancements. In this blog post, we'll delve into the primary reasons behind this struggle and explore some vital JDK 17 features that are worth mastering.
The Evolution of Java
Java has evolved tremendously since its inception in the mid-90s. The transition from JDK 8, which introduced major features like Lambda Expressions and Stream APIs, to JDK 17, offers a plethora of new capabilities. Here's a brief overview of the timeline:
- JDK 8 (2014): Introduced Lambda expressions, Stream API, and more.
- JDK 9 (2017): Modular System (Project Jigsaw).
- JDK 10 (2018): Local variable type inference with
var
. - JDK 11 (2018): Long-Term Support (LTS) release, introducing features like the HttpClient.
- JDK 12 (2019) to JDK 16 (2021): Incremental changes and features.
- JDK 17 (2021): Another LTS release, featuring pattern matching and enhanced performance.
With each new version, the learning curve for developers grows steeper. Here are the key reasons why developers often find it challenging to keep up:
1. Rapid Release Cycle
The introduction of a new Java version every six months means that developers are constantly bombarded with new features. While this ensures continuous improvement, it can be overwhelming. Staying updated requires consistent learning and adaptation.
Example of Pattern Matching
One of the eye-catching features in JDK 17 is pattern matching for instanceof
. This simplifies type checks and casts in code.
public void processShape(Shape shape) {
if (shape instanceof Circle circle) {
System.out.println("Circle with area: " + circle.getArea());
} else if (shape instanceof Rectangle rectangle) {
System.out.println("Rectangle with area: " + rectangle.getArea());
}
}
Why: This code reduces boilerplate and enhances readability. You no longer need a separate cast after the instanceof
check. It streamlines the code process, allowing developers to focus more on business logic.
2. Complexity of New Features
Certain JDK features are inherently complex. Understanding how to implement these features effectively requires not just knowledge of the APIs, but also the underlying principles.
Sealed Classes Example
Sealed classes, introduced in JDK 17, restrict which classes can inherit from a base class. This is useful for modeling restricted hierarchies.
public sealed class Shape permits Circle, Rectangle {
// Common properties/methods for all shapes
}
public final class Circle extends Shape {
// Circle specific implementation
}
public final class Rectangle extends Shape {
// Rectangle specific implementation
}
Why: Sealed classes provide control over the inheritance hierarchy, thus making the code safer and more maintainable. However, grasping the full utility of sealed classes requires an understanding of design principles and how inheritance works in Java.
3. Shift in Development Practices
Java is not just a programming language; it's a cornerstone of many software architectures and practices. The rise of microservices, cloud computing, and DevOps practices is changing how Java is used. JDK 17 aligns with these evolving practices but requires developers to adapt.
New Java Features in Context
Consider how the new HttpClient
from JDK 11 and enhanced features in JDK 17 integrate naturally into microservices:
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://api.example.com/data"))
.build();
client.sendAsync(request, HttpResponse.BodyHandlers.ofString())
.thenAccept(response -> System.out.println(response.body()));
Why: The new HttpClient features asynchronous operations, which are crucial when building efficient, service-oriented architectures. This means developers should feel comfortable handling asynchronous programming to capitalize on these advancements.
4. The Skills Gap
Despite Java's popularity, the workforce faces a shortage of developers who are well-versed with newer features and frameworks. This skill gap often leads to a reliance on existing knowledge rather than embracing new techniques.
Example of Switch Expressions
Switch expressions, introduced in earlier JDK versions and enhanced in JDK 17, provide a more concise and readable way to handle switches.
String result = switch (day) {
case MONDAY -> "Start of the week";
case FRIDAY, SATURDAY -> "Almost the weekend!";
default -> "Midweek days";
};
Why: This approach allows for a more expressive syntax. However, not all developers are familiar with the nuances of switch expressions, potentially hindering their full implementation in projects.
5. Learning Resources and Documentation
While there are numerous resources available, sifting through this vast ocean of information can be daunting. How does one decide which resources are reliable and up-to-date? In-depth tutorials, relevant blogs, and official documentation from Oracle often hold the key.
Visit Oracle's official documentation to access the relevant JDK 17 documentation and guides.
Closing the Chapter
In conclusion, several factors contribute to the struggle that developers face to keep up with JDK 17 features. Rapid advancements, increased complexity, evolving practices, skills gaps, and vast resources all play a role. However, investing time in understanding these new capabilities can significantly enhance the development process and efficiency.
By embracing the new features and refining their skills, developers can not only keep pace with JDK 17, but also leverage its power to build robust applications. Remember, adaptability and lifelong learning are essential traits for any developer in today's fast-paced tech landscape.
If you're looking to deepen your understanding of Java and its evolving ecosystem, investing in courses or attending community events can be beneficial. Consider checking out platforms like Codecademy and Pluralsight for dedicated Java courses.
For those who aim to keep their Java skills sharp, dedication to learning and experimenting with new features will pave the way for success in your development endeavors.