Overcoming Common Pitfalls in Microservices Deployment
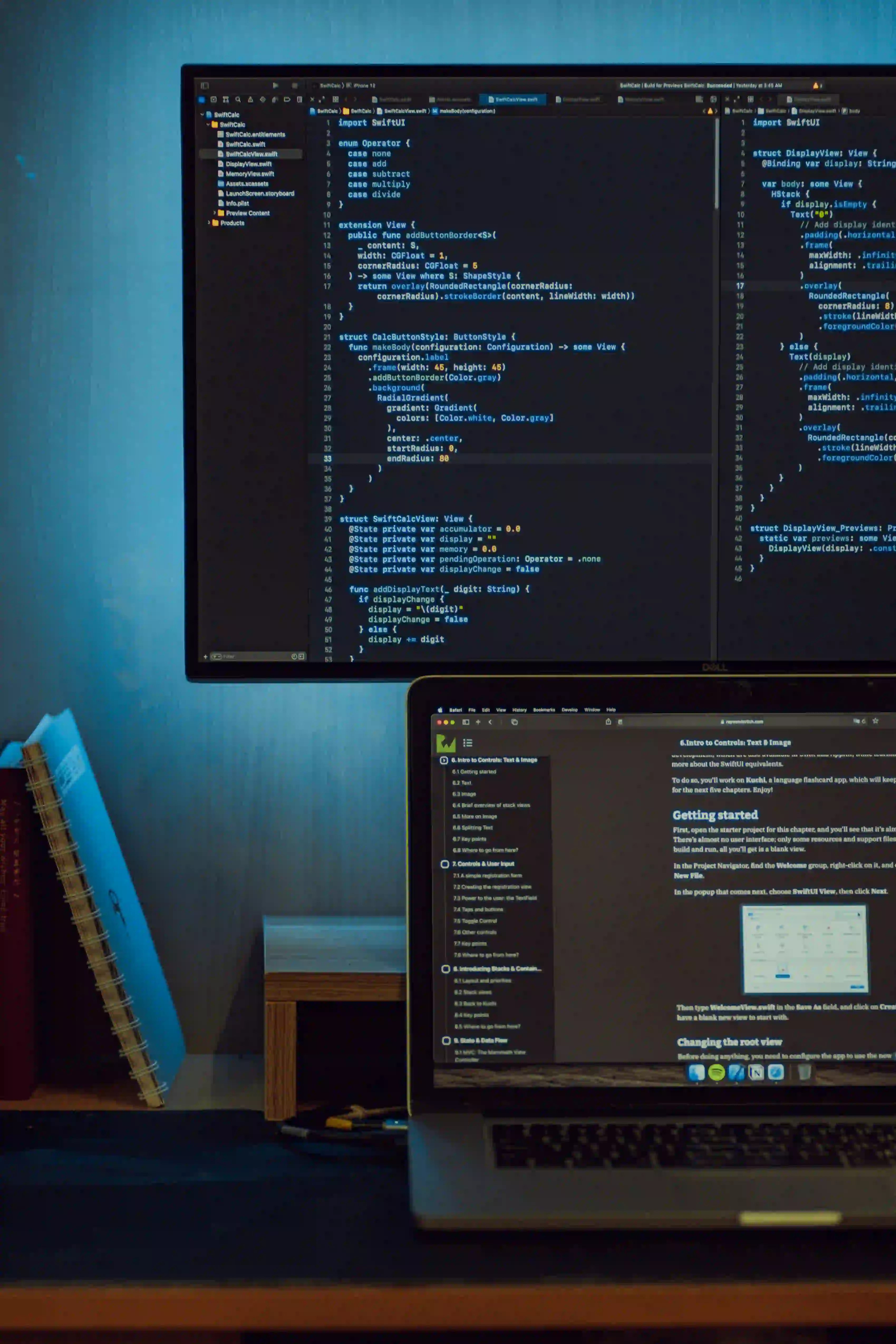
Overcoming Common Pitfalls in Microservices Deployment
The microservices architecture is taking the tech world by storm. The ability to create small, independently deployable services offers unprecedented flexibility and scalability for application development. Nevertheless, transitioning to microservices is not without its challenges. In this blog post, we will navigate through the common pitfalls encountered in microservices deployment and provide best practices for overcoming these hurdles.
Understanding Microservices
Before diving into the pitfalls, let's quickly recap what microservices are. Microservices is an architectural style that structures an application as a collection of loosely coupled services. Each service is responsible for a specific function and can be developed, deployed, and scaled independently. This approach stands in contrast to monolithic architectures, where everything is tightly coupled.
The benefits of microservices are clear:
- Resilience: Failures in one service do not directly impact others.
- Scalability: Individual services can be scaled independently based on demand.
- Agility: Teams can develop and deploy services rapidly using the technology stack best suited for each service.
However, as organizations adopt microservices, they often face several common pitfalls.
Common Pitfalls in Microservices Deployment
1. Service Granularity
Determining the appropriate size for a microservice is a common challenge. Too many small services can lead to increased overhead, while overly large services can negate the benefits of microservices.
Best Practice: Define Boundaries Based on Domain-Driven Design (DDD)
Using techniques from Domain-Driven Design can help define service boundaries. A good rule of thumb is to have each service handle a specific business capability.
// Service for handling user management
public class UserService {
public User createUser(UserDetails userDetails) {
// create user logic
return new User(...);
}
// other user-related methods
}
// Service for handling orders
public class OrderService {
public Order createOrder(User user, List<Item> items) {
// create order logic
return new Order(...);
}
// other order-related methods
}
Why This is Important: Small services allow teams to operate independently, promoting DevOps practices. Conversely, too broad a focus can lead to interdependencies and communication woes.
2. Data Management
With microservices, maintaining a cohesive data model can be challenging. Services often need to communicate and share data, but doing this across many services can lead to inconsistencies and increased latency.
Best Practice: Use a Polyglot Persistence Approach
Different services can use different types of databases tailored to their needs. For example, a product catalog might use a document-oriented database, while user data might rely on a relational database. It is crucial to keep the databases decoupled.
// MongoDB usage for product service
public class ProductService {
private final MongoTemplate mongoTemplate;
public ProductService(MongoTemplate mongoTemplate) {
this.mongoTemplate = mongoTemplate;
}
public void saveProduct(Product product) {
mongoTemplate.save(product);
}
}
// JPA usage for user service
@Entity
public class User {
@Id
private Long id;
private String name;
}
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User createUser(User user) {
return userRepository.save(user);
}
}
Why This is Important: Using different data stores allows each service to be optimized for its functions, but it demands that proper communication and data synchronization mechanisms be established.
3. Inter-Service Communication
Microservices communicate over the network, leading to complexities such as managing API endpoints, data serialization formats, and error handling.
Best Practice: Use Async Communication where Possible
Leveraging asynchronous communication, such as messaging queues and event-driven designs, can help retain system resilience.
For example, a UserService might publish events when users are created.
// Sample code for publishing a user creation event
public class UserCreationEvent {
private User user;
public UserCreationEvent(User user) {
this.user = user;
}
}
public class UserService {
private final EventPublisher eventPublisher;
public UserService(EventPublisher eventPublisher) {
this.eventPublisher = eventPublisher;
}
public User createUser(UserDetails userDetails) {
User user = new User(...);
eventPublisher.publish(new UserCreationEvent(user));
return user;
}
}
Why This is Important: Asynchronous processes reduce dependencies between services while also improving overall throughput and response times.
4. Monitoring and Logging
With multiple services running independently, comprehensive monitoring and logging can become challenging. A lack of visibility into the system can result in difficulties pinpointing issues when they arise.
Best Practice: Implement Centralized Logging and Monitoring Tools
Tools such as ELK Stack (Elasticsearch, Logstash, and Kibana) or Prometheus with Grafana can provide the insights needed to troubleshoot microservices effectively.
Why This is Important: Centralized systems allow teams to analyze performance metrics, errors, and logs easily, improving overall application reliability.
5. Deployment Automation and Management
Microservices can require considerable orchestration. Manual deployments can lead to human error and inconsistencies, particularly in environments that require frequent changes.
Best Practice: Adopt a CI/CD Pipeline
Continuous Integration and Continuous Deployment (CI/CD) techniques automate the deployment process. Tools like Jenkins, GitLab CI, or GitHub Actions can help manage deployment workflows efficiently.
# Example GitHub Actions CI/CD pipeline
name: CI/CD Pipeline
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Build with Maven
run: mvn clean install
- name: Deploy to Production
run: ./deploy.sh
Why This is Important: Automation minimizes manual work, ensuring deployments are consistent and repeatable.
6. Security Concerns
Microservices create new attack vectors that are often overlooked in traditional monolithic applications. Each service requires its own security measures, including authenticating and authorizing requests.
Best Practice: Implement API Gateway and Security Best Practices
An API gateway can centrally manage authentication and routing, safeguarding services from unauthorized access. You might use tools like Spring Cloud Gateway or Kong.
Why This is Important: Securing your microservices architecture effectively helps mitigate risks of breaches and ensures data protection.
In Conclusion, Here is What Matters
Deploying microservices comes with its share of challenges; however, by acknowledging these common pitfalls and implementing best practices, organizations can leverage the full potential of microservices architecture. Each decision regarding service granularity, data management, communication, monitoring, deployment, and security plays a crucial role in building resilient, efficient systems.
Microservices can revolutionize your application development workflows, enhancing scalability and agility. Embracing these best practices can pave the way for smoother adoption.
Are you considering a microservices approach or currently facing complexities? Share your experiences, and let’s better understand how to navigate these waters together!
For further reading on microservices, you might find the following resources useful:
- Microservices.io - A comprehensive collection of patterns for microservices architecture.
- Martin Fowler's Thoughts on Microservices - An insightful overview from one of the thought leaders in software architecture.
Successful microservices deployment is within reach if you stay aware and proactive about challenges. Happy coding!