Overcoming Common Pitfalls in Lombok and AutoValue Integration
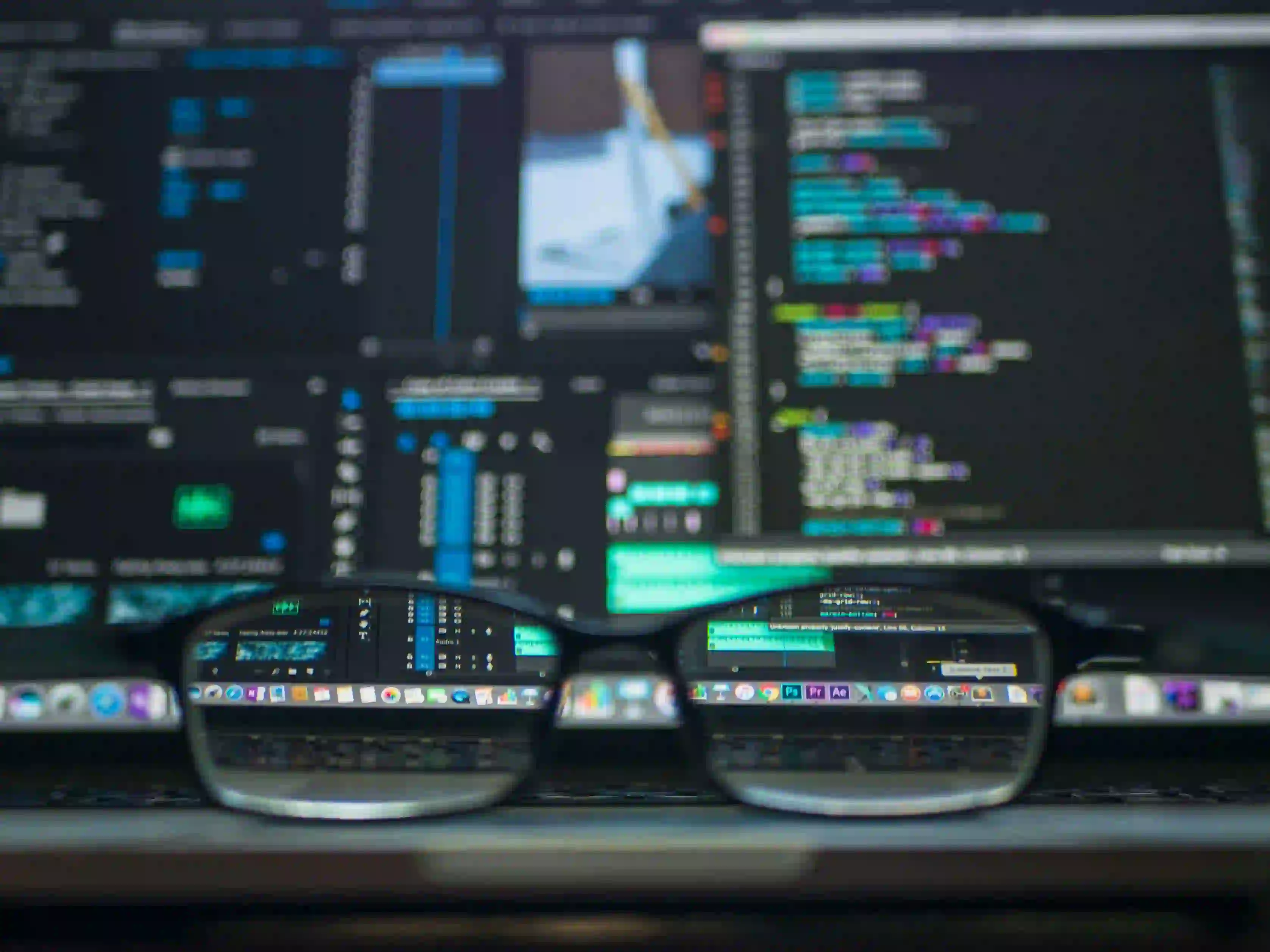
Overcoming Common Pitfalls in Lombok and AutoValue Integration
Integrating Lombok with AutoValue can significantly simplify and reduce boilerplate code in Java applications. Both of these tools enhance productivity by automating the creation of constructors, accessors, and other boilerplate code. However, developers often encounter pitfalls when integrating these two powerful libraries. In this blog post, we'll explore common challenges and provide solutions, allowing you to leverage both Lombok and AutoValue effectively in your Java projects.
What Are Lombok and AutoValue?
Lombok
Lombok is a Java library that automatically plugs into your editor and build tools. It helps reduce boilerplate code by generating getters, setters, toString, equals, and hashCode methods, along with other features. With a simple annotation-driven approach, Lombok allows you to keep your Java classes clean and readable.
AutoValue
AutoValue, on the other hand, is a library developed by Google for creating immutable value classes. It allows developers to define a class in a minimalistic style while AutoValue generates the necessary code behind the scenes to make it immutable and providing methods like equals, hashCode, and toString.
Here's an example of a simple value class with AutoValue:
import com.google.auto.value.AutoValue;
@AutoValue
public abstract class User {
public abstract String name();
public abstract int age();
public static User create(String name, int age) {
return new AutoValue_User(name, age);
}
}
In this example, User
is defined as an abstract class. The @AutoValue
annotation signals the compiler to generate the implementation.
Why Lombok and AutoValue Together?
When you combine Lombok and AutoValue, you can benefit from both libraries in a project, thus reducing boilerplate code while maintaining immutability. However, their integration can be tricky. Let's dive into some common pitfalls.
Common Pitfalls in Lombok and AutoValue Integration
1. Conflicting Annotations
Both Lombok and AutoValue generate methods for classes, which can lead to conflicts, particularly with the @Getter
, @Setter
, and @Value
annotations. AutoValue already provides the toString
, equals
, and hashCode
methods, which can conflict with Lombok's auto-generated methods.
Solution:
Use Lombok's @Value
annotation carefully. Since AutoValue is designed for immutable classes, you don't typically need to use Lombok's setter methods. Stick with AutoValue's generated methods by removing Lombok getter and other conflicting annotations where necessary.
Example of problematic conflicting annotations:
import com.google.auto.value.AutoValue;
import lombok.Getter;
@AutoValue
public abstract class Product {
@Getter // This is not needed; AutoValue generates getter
public abstract String name();
public static Product create(String name) {
return new AutoValue_Product(name);
}
}
Remove the @Getter
annotation to avoid confusion.
2. Misunderstanding Field Accessibility
By default, AutoValue requires abstract methods to define fields. If you try to use Lombok's annotations on non-public fields, it may lead to compilation errors. To avoid issues, you should declare your abstract methods as public.
Solution:
Define methods for fields publicly while using AutoValue to ensure that Lombok does not interfere.
@AutoValue
public abstract class Order {
public abstract String item();
public abstract double price();
public static Order create(String item, double price) {
return new AutoValue_Order(item, price);
}
}
By ensuring methods are public, we prevent accessibility issues and retain the generated code's intended functionality.
3. Generating Constructors
If you're using Lombok's @Builder
alongside AutoValue, be cautious about constructor generation. AutoValue creates a constructor that requires all arguments, while Lombok can generate an inconsistent builder interface.
Solution:
Instead of using @Builder
, utilize AutoValue's builder generated methods. Use the @AutoValue.Builder
annotation to enable builder functionality directly within AutoValue.
@AutoValue
public abstract class Employee {
public abstract String id();
public abstract String name();
@AutoValue.Builder
public abstract static class Builder {
public abstract Builder id(String id);
public abstract Builder name(String name);
public abstract Employee build();
}
public static Builder builder() {
return new AutoValue_Employee.Builder();
}
}
This approach avoids conflicts with Lombok’s builder logic and ensures that the AutoValue-generated builder works seamlessly.
4. IDE Support
Another common pitfall occurs with IDE support. Many developer tools can have varying levels of compatibility with Lombok or AutoValue integrations. Annotations may not be recognized, leading to confusion while coding.
Solution:
Ensure that your IDE has the appropriate plugins installed:
- For IntelliJ IDEA, install the Lombok Plugin and enable annotation processing.
- For Eclipse, install the Lombok jar and run the installer.
Resources for Further Learning
By paying attention to these common pitfalls, you can successfully integrate Lombok and AutoValue in your Java projects, allowing you to write more concise and maintainable code.
Closing Remarks
Integrating Lombok with AutoValue can provide significant advantages in terms of reducing boilerplate code and simplifying your Java classes. However, as we've discussed, it's essential to navigate the integration carefully to avoid common pitfalls, such as conflicting annotations, field accessibility, constructor generation issues, and ensuring IDE support.
The enhancements brought about by using both libraries in tandem can lead not only to cleaner codebase but also improve developer productivity and maintainability in the long term. By adhering to best practices and understanding how to effectively use these two tools, you position yourself and your team for long-term success in Java development.
Final Thoughts
As always, encourage your development team to stay updated with the latest versions of both Lombok and AutoValue, as they may bring new features and enhancements, further streamlining your development process. Happy coding!