Understanding JWT Security Flaws: Protect Your API
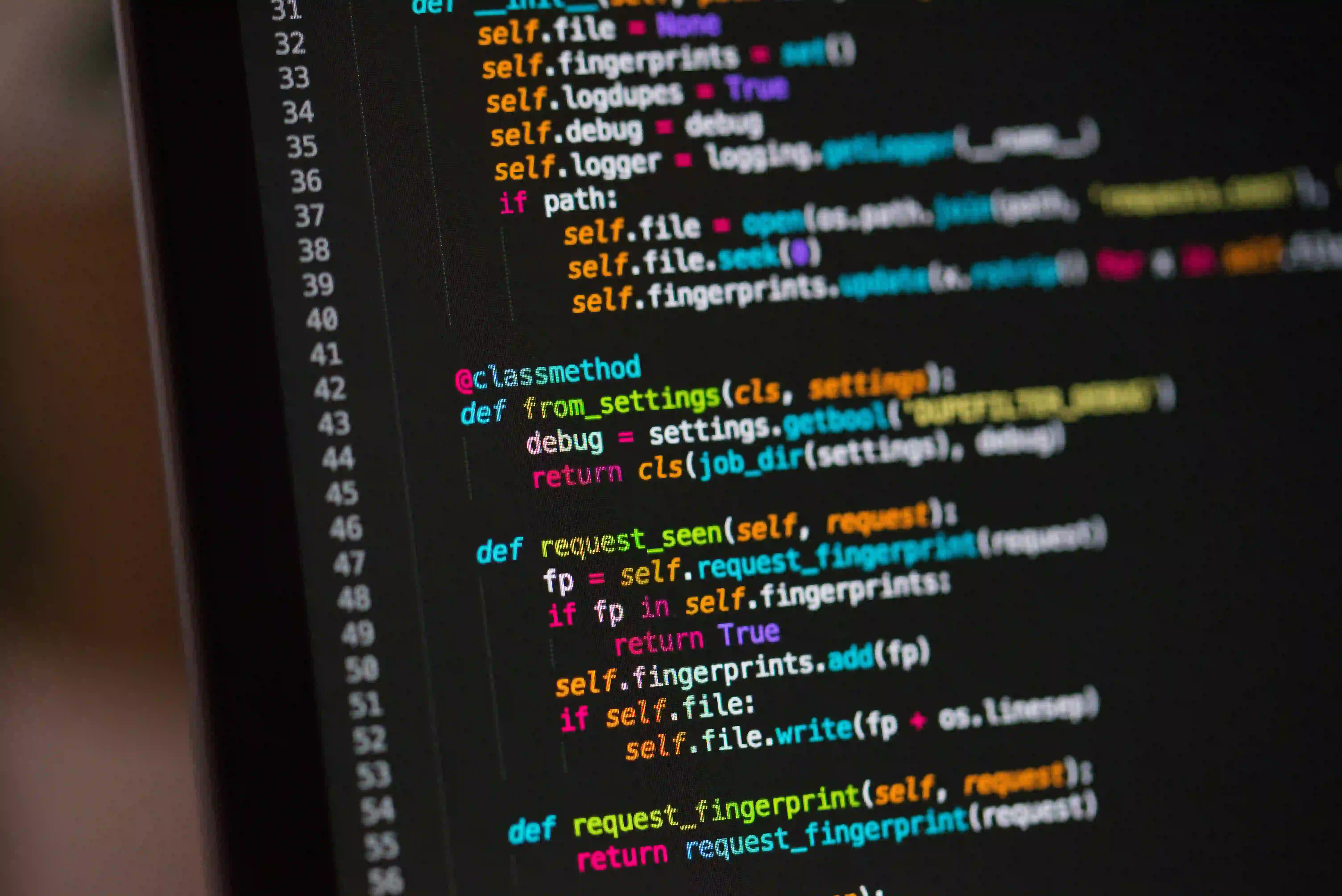
Understanding JWT Security Flaws: Protect Your API
In today's digital landscape, JSON Web Tokens (JWTs) have become a common solution for stateless authentication. Their lightweight structure and ease of implementation make them appealing. However, with great power comes great responsibility. It's crucial to understand the security flaws that can compromise APIs using JWTs. In this article, we'll explore the vulnerabilities of JWTs, best practices for mitigation, and how to secure your API effectively.
What is JWT?
Before diving into its vulnerabilities, let's clarify what a JWT is. JWT is a compact, URL-safe means of representing claims to be transferred between two parties. They can be used to verify the identity of a user and to ensure that the information contained within the token is not altered.
A typical JWT consists of three parts:
- Header: Contains metadata about the token, including the type of token and the signing algorithm.
- Payload: Contains the claims, which can include user data and permissions.
- Signature: This is created by taking the encoded header, the encoded payload, and a secret key, and signing them.
A simple representation of a JWT appears as follows:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VySWQiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
The Appeal of JWTs
JWTs are favored for various reasons:
- Statelessness: No need to store sessions on the server. Everything is contained in the token itself.
- Cross-Domain Authentication: They are easily transmitted across different domains and applications.
- Easy to Use: Due to their compact structure, they can be sent via URL, POST parameter, or inside an HTTP header.
Common JWT Security Flaws
Having established what JWT is, let's discuss its potential security flaws. Understanding these vulnerabilities will allow you to secure your APIs effectively.
1. Algorithm Manipulation
One of the notable flaws in JWT is algorithm manipulation. If the token's header claims a specific signing algorithm (for example, HS256), and the server accepts the algorithm specified in the token's header without validating it, attackers can manipulate this.
Example of the Flaw: If an attacker changes the algorithm to "none," the server may accept the token without a signature.
Mitigation: Always validate the algorithm. Only accept a known list of signing algorithms and reject any JWT that specifies "none."
DecodedJWT decodedJWT = JWT.decode(token);
String algorithm = decodedJWT.getAlgorithm();
if (!algorithm.equals("HS256")) {
throw new IllegalArgumentException("Invalid algorithm!");
}
2. Expiry Times Not Implemented
Another common flaw is not utilizing the exp
claim effectively. The expiration time is crucial for limiting the lifetime of a token. Without it, stolen tokens can be used perpetually.
Mitigation: Always have a reasonable expiration time set and use refresh tokens to obtain new access tokens.
Date now = new Date();
Date expiryDate = new Date(now.getTime() + 3600000); // 1 hour expiration
String token = JWT.create()
.withSubject(userId)
.withExpiresAt(expiryDate)
.sign(Algorithm.HMAC256(secret));
3. Lack of Token Revocation
Since JWTs are stateless, once issued, they cannot be revoked easily. This presents a significant risk if a token is compromised.
Mitigation: Implement a token blacklist or a versioning strategy. Track token versions alongside user sessions to allow for revocation when necessary.
// Mock Code: store blacklisted tokens
Set<String> blacklistedTokens = new HashSet<>();
if (blacklistedTokens.contains(token)) {
throw new RuntimeException("Token is revoked!");
}
4. Lack of Audience Claim Verification
The aud
claim identifies the recipients that the JWT is intended for. If checks for this claim are not implemented, tokens issued for one service may be accepted by another.
Mitigation: Enforce audience checks to ensure that tokens are being used correctly.
String expectedAudience = "myapp";
if (!decodedJWT.getAudience().contains(expectedAudience)) {
throw new IllegalArgumentException("Invalid Audience!");
}
5. Insecure Storage
It's essential to be cautious when storing JWTs. Clients that store tokens insecurely can open themselves up to attacks like Cross-Site Scripting (XSS).
Mitigation: Store JWTs in secure HTTP-only cookies rather than local storage whenever possible.
Best Practices for Secure JWT Implementation
Now that we've covered common security flaws, here are best practices for implementing JWTs securely:
Use Strong Signing Algorithms
Always use robust signing algorithms like RS256, which require public/private key pairing. Avoid weak algorithms like HS256 whenever possible.
Keep the Token Size to a Minimum
Since JWTs can become lengthy with many claims, it's best to keep them succinct. A smaller token size means faster transmission and processing.
Implement Refresh Tokens
Use short-lived access tokens in combination with long-lived refresh tokens. This minimizes risks in the event of token leakage.
Monitor and Audit
Regularly monitor and audit token usage. Implement logging mechanisms to track suspicious activity.
Library Choices
Select well-maintained libraries for JWT implementation. Avoid bespoke solutions, as they are likely to be error-prone.
For those looking for a deeper understanding of JWT implementations, you can refer to JWT.io as an excellent resource.
Wrapping Up
As we've explored, while JWTs provide a robust solution for stateless authentication, they come with potential security risks. By understanding and mitigating these vulnerabilities, developers can create more secure APIs. Always enforce best practices and remain vigilant in monitoring your JWT implementations.
By taking the necessary precautions, you can protect your API from common JWT security flaws and ensure a safer user experience.