Unlocking User Experience: Customizable UI Solutions
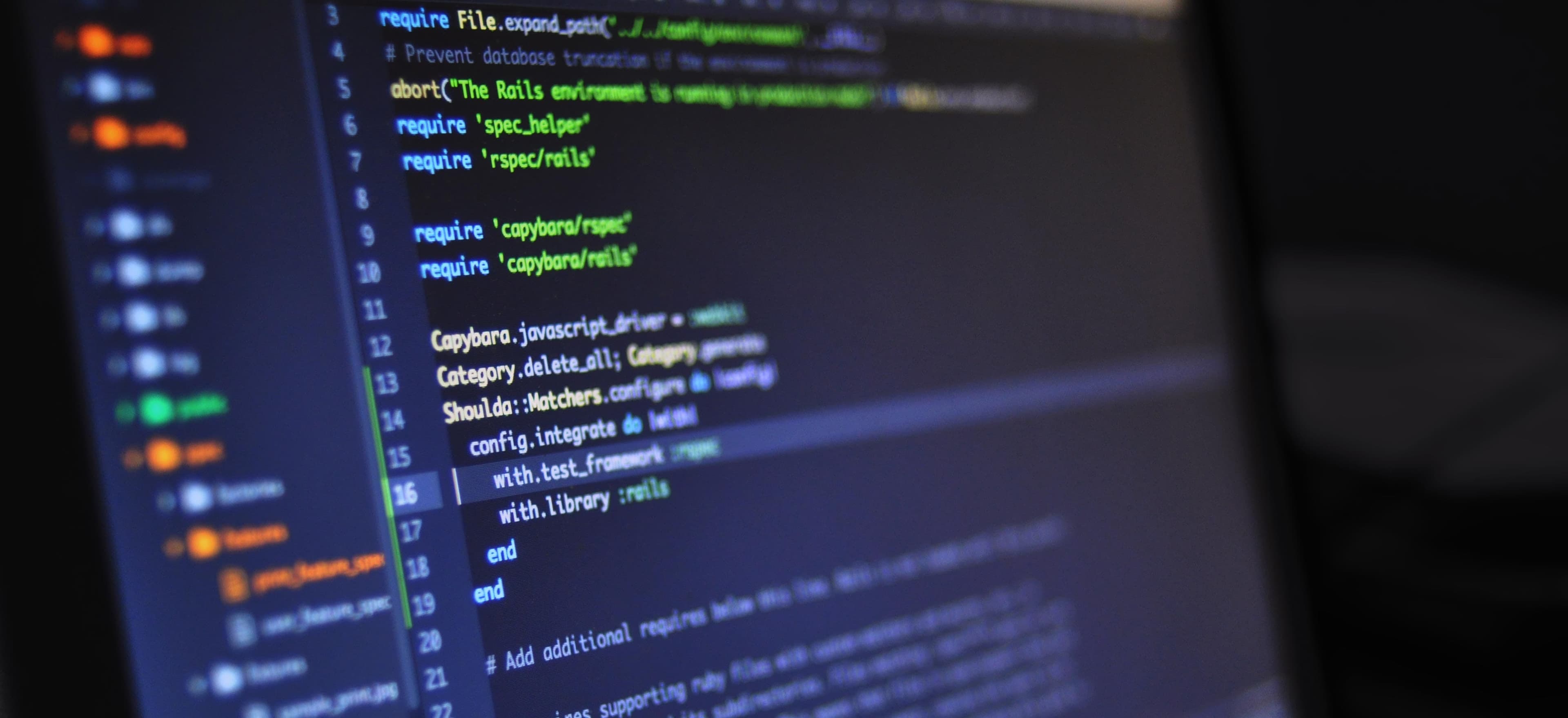
- Published on
Unlocking User Experience: Customizable UI Solutions in Java
User interfaces (UI) are the bridge between users and applications. With the evolving landscape of technology, providing a customizable UI has become a necessity to meet diverse user expectations. In this blog post, we’ll investigate the importance of customizable UI solutions within Java applications. We will explore frameworks, methodologies, and practical examples to help you create flexible UIs that enhance user experience (UX).
Understanding Customization in UI
Customization refers to the ability of users to modify and personalize the interface to suit their preferences. It includes changing themes, layouts, widgets, and even functionality. Why does this matter? Research shows that tailored experiences can significantly increase user satisfaction and engagement.
Key Benefits of Customizable UI
- Improved User Satisfaction: Users feel more in control when they can adjust the layout and features according to their needs.
- Increased Accessibility: Customizable interfaces can be adapted for users with disabilities or specific needs.
- Enhanced Engagement: Users are more likely to interact with a tool that they can personalize.
- Adaptability to Use Cases: Different users may need different tools for varied tasks. Customizable UIs cater to diverse use cases.
Java and UI Customization
Java provides several libraries and frameworks that empower developers to create versatile and customizable UIs. The following are notable frameworks:
- JavaFX: A powerful platform for building rich desktop applications.
- Swing: Although older, Swing remains a powerful toolkit for building graphical user interfaces (GUIs).
- JavaServer Faces (JSF): A Java specification for building component-based user interfaces for web applications.
Let’s dive into practical implementations using JavaFX to demonstrate how we can create a customizable UI.
Setting Up JavaFX
Before we get started, ensure you have JavaFX configured in your IDE. If you're using IntelliJ or Eclipse, you can follow this guide for installation instructions.
Basic JavaFX Application Structure
Here is the basic structure for a JavaFX application:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class CustomizableUIExample extends Application {
@Override
public void start(Stage primaryStage) {
StackPane root = new StackPane();
Scene scene = new Scene(root, 400, 300);
primaryStage.setTitle("Customizable UI Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Explanation
- Application Class: Extending the Application class is necessary for any JavaFX application. It provides a framework for launching the application.
- Stage and Scene: The Stage represents the main window, while the Scene represents the container for all content that appears on the Stage.
- Layout: We are using a StackPane as our layout, which is a simple layout that stacks all children on top of each other.
Building a Customizable Theme Selector
Now, let’s build a more interactive application that allows users to select from different themes.
Step 1: Creating a Theme Class
First, we need a class to manage our themes:
import javafx.scene.Scene;
import javafx.scene.paint.Color;
public class ThemeManager {
public enum Theme {
LIGHT, DARK
}
public static void applyTheme(Scene scene, Theme theme) {
switch (theme) {
case LIGHT:
scene.setFill(Color.WHITE);
break;
case DARK:
scene.setFill(Color.DARKGRAY);
break;
}
}
}
Code Explanation
- Theme Enum: An enumeration that defines different themes (in this case, LIGHT and DARK).
- applyTheme Method: This method modifies the Scene's background color based on the selected theme.
Step 2: Integrating the Theme Manager
Next, we will integrate the ThemeManager into our main application.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class CustomizableUIExample extends Application {
private ThemeManager.Theme currentTheme = ThemeManager.Theme.LIGHT;
@Override
public void start(Stage primaryStage) {
StackPane root = new StackPane();
Scene scene = new Scene(root, 400, 300);
Button toggleButton = new Button("Toggle Theme");
toggleButton.setOnAction(event -> {
currentTheme = (currentTheme == ThemeManager.Theme.LIGHT) ?
ThemeManager.Theme.DARK :
ThemeManager.Theme.LIGHT;
ThemeManager.applyTheme(scene, currentTheme);
});
root.getChildren().add(toggleButton);
ThemeManager.applyTheme(scene, currentTheme);
primaryStage.setTitle("Customizable UI Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Explanation
- Button for Theme Toggle: We add a button that allows users to toggle between themes. An event handler changes the theme based on the current selection.
- Dynamic UI Change: The
applyTheme
method is invoked each time the button is pressed to update the UI.
Enhancing User Experience with Customization
User Preferences Persistence
Incorporating user preferences is crucial in providing a seamless experience. By saving users' theme choices across sessions, you ensure that they return to their desired environment. Here's how you can implement it with Java’s Preferences API:
import java.util.prefs.Preferences;
public class UserPreferences {
private static final String THEME_PREF = "theme_pref";
private Preferences prefs;
public UserPreferences() {
prefs = Preferences.userNodeForPackage(UserPreferences.class);
}
public void setTheme(ThemeManager.Theme theme) {
prefs.put(THEME_PREF, theme.name());
}
public ThemeManager.Theme getTheme() {
String themeName = prefs.get(THEME_PREF, ThemeManager.Theme.LIGHT.name());
return ThemeManager.Theme.valueOf(themeName);
}
}
Code Explanation
- Preferences API: This API allows storing user preferences in a system-dependent way. Here, we’ve defined a method for setting and getting the UI theme preference.
Final Integration
Finally, modify your start
method to load preferences:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class CustomizableUIExample extends Application {
private ThemeManager.Theme currentTheme;
private UserPreferences userPreferences;
@Override
public void start(Stage primaryStage) {
userPreferences = new UserPreferences();
currentTheme = userPreferences.getTheme();
StackPane root = new StackPane();
Scene scene = new Scene(root, 400, 300);
Button toggleButton = new Button("Toggle Theme");
toggleButton.setOnAction(event -> {
currentTheme = (currentTheme == ThemeManager.Theme.LIGHT) ?
ThemeManager.Theme.DARK :
ThemeManager.Theme.LIGHT;
userPreferences.setTheme(currentTheme);
ThemeManager.applyTheme(scene, currentTheme);
});
root.getChildren().add(toggleButton);
ThemeManager.applyTheme(scene, currentTheme);
primaryStage.setTitle("Customizable UI Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
A Final Look
By creating a customizable UI in Java, you offer users a more engaging experience tailored to their needs. Whether through themes, layouts, or functionality, customization enhances user interaction and satisfaction. With libraries like JavaFX, you can easily implement these features to improve the overall user experience.
For further reading, you might want to explore:
- JavaFX Documentation
- A Guide to User-Centered Design
Remember, a great user experience is not just a feature; it’s a vital part of your application’s success. Keep evolving your UIs based on user needs and preferences!