Struggling with Java Testing? Discover the Best Libraries!
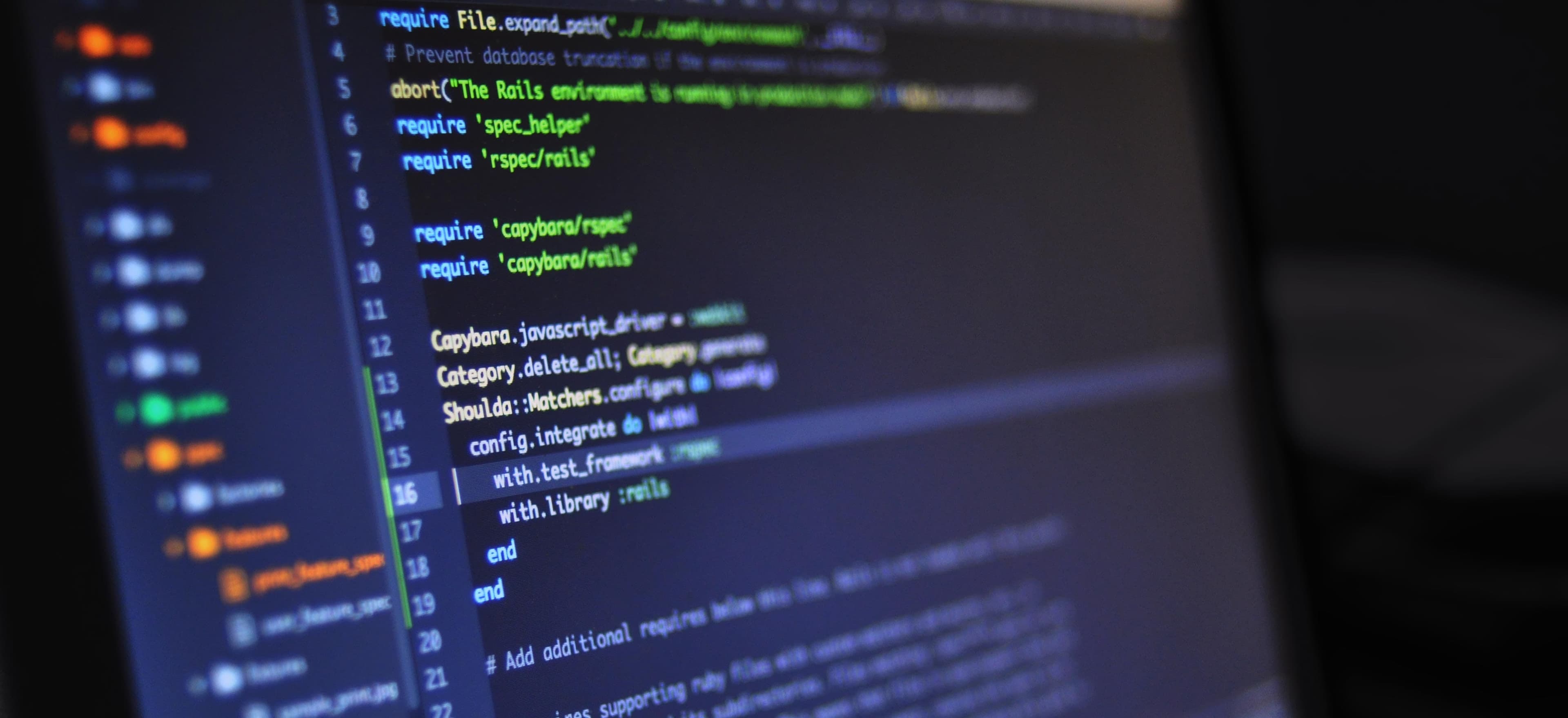
- Published on
Struggling with Java Testing? Discover the Best Libraries!
Java is one of the most versatile programming languages, and testing is a critical part of the development process. Automated tests help ensure that your code runs smoothly, catches bugs early, and increases the overall quality of the application. However, navigating the vast landscape of testing libraries can be overwhelming, especially if you're just getting started. In this post, we'll explore some of the best Java testing libraries available today and how they can make your life easier.
The Importance of Testing in Java
Before jumping into the libraries, it's essential to understand why testing is crucial in software development. Testing not only verifies that your code behaves as intended but also promotes maintainability and scalability. Regular testing can catch regressions early, making it easier to adapt to changes in requirements. In a world where time is of the essence, having a solid testing framework can save you significant time in the long run.
Types of Testing
In Java, various types of testing can be employed:
- Unit Testing: Testing individual components or methods for correctness.
- Integration Testing: Testing the interaction between different components or systems.
- Functional Testing: Verifying that the application behaves as expected from an end-user perspective.
- Performance Testing: Assessing the speed and efficiency of the application.
With this foundation, let’s dive into the libraries that can help you enhance your testing efforts.
1. JUnit
Overview
JUnit is perhaps the most well-known testing framework for Java. It provides a simple and powerful way to define and run tests.
Features
- Annotations: JUnit uses annotations like
@Test
,@Before
, and@After
to designate test methods and setup/teardown actions. - Assertions: Built-in assertions help verify that the expected result meets actual outcomes.
- Test Runner: JUnit 5 includes a powerful test runner capable of running tests in various environments.
Sample Code
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
private Calculator calculator;
@BeforeEach
public void setUp() {
calculator = new Calculator();
}
@Test
public void testAddition() {
assertEquals(5, calculator.add(2, 3), "2 + 3 should equal 5");
}
}
In this example, setUp()
initializes the Calculator
object before each test runs. This follows the DRY (Don't Repeat Yourself) principle, ensuring that setup code is not duplicated across tests.
Why JUnit?
JUnit is a foundation for testing in Java, making it a must-have for any developer. Its simplicity and extensive documentation make it easy for newcomers to pick up.
2. Mockito
Overview
Mockito is a mocking framework that helps you create test doubles (mock objects) for your dependencies. This is especially useful in unit testing where you want to isolate the class under test.
Features
- Simple API: The API is straightforward, making it easy to create and verify mocks.
- Behavior Verification: Allows you to check if certain methods were called on mocks.
- Stubbing: You can specify return values for mock methods with ease.
Sample Code
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
public class UserServiceTest {
@Test
public void testGetUser() {
UserRepository mockRepository = mock(UserRepository.class);
UserService userService = new UserService(mockRepository);
User user = new User("John Doe");
when(mockRepository.getUser("1")).thenReturn(user);
User result = userService.getUser("1");
assertEquals("John Doe", result.getName());
verify(mockRepository).getUser("1");
}
}
In this code, we create a mocked UserRepository
. By using when(...).thenReturn(...)
, we define the expected behavior of our mock, ensuring we isolate the UserService
logic.
Why Mockito?
Mockito helps you test classes in isolation, making sure that the behavior of a class doesn't depend on the external state. It enhances the focus of unit tests, ensuring that you can validate one component at a time.
3. TestNG
Overview
TestNG is an advanced testing framework inspired by JUnit but introduces powerful new features.
Features
- Annotations: More flexible and powerful usage of annotations compared to JUnit.
- Parallel Execution: Supports running tests in parallel, reducing total execution time.
- Data Parameterization: Easily run the same test with different data.
Sample Code
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
public class CalculatorTest {
@DataProvider(name = "additionData")
public Object[][] additionData() {
return new Object[][] {
{ 1, 2, 3 },
{ 2, 3, 5 },
{ -1, 1, 0 }
};
}
@Test(dataProvider = "additionData")
public void testAddition(int a, int b, int expected) {
Calculator calculator = new Calculator();
assertEquals(expected, calculator.add(a, b));
}
}
This example illustrates how TestNG's data provider can be used to feed multiple sets of parameters into the testAddition
method, thus testing various scenarios with a single test case.
Why TestNG?
TestNG is more flexible and powerful than JUnit, making it suitable for larger projects where advanced testing features are required. If your tests span integration, functional, or performance, TestNG may be the better choice.
Learn more about TestNG
4. AssertJ
Overview
AssertJ provides a rich set of assertions, enhancing readability and expressiveness in tests.
Features
- Fluent API: Allows for cleaner and more intuitive assertions.
- Extensions: Integrates seamlessly with JUnit, Mockito, and other frameworks.
- Type-Safe Assertions: Ensure that your assertions match the type of the object being tested.
Sample Code
import static org.assertj.core.api.Assertions.*;
public class UserServiceTest {
@Test
public void testUserCreation() {
UserService userService = new UserService();
User user = userService.createUser("John Doe");
assertThat(user).isNotNull();
assertThat(user.getName()).isEqualTo("John Doe");
assertThat(user.getAge()).isGreaterThan(0);
}
}
In this code snippet, AssertJ's readable assertions make it clear what we're validating. This boosts both clarity and maintainability.
Why AssertJ?
AssertJ encourages descriptive and fluent assertions, allowing for better readability of tests. This is particularly important as codebases grow and teams expand.
The Closing Argument
Testing is vital for the quality and reliability of your Java applications. The libraries mentioned here—JUnit, Mockito, TestNG, and AssertJ—are instrumental in creating a robust testing environment.
By adopting these libraries, you can enhance the reliability and maintainability of your projects. Remember that the choice of library often depends on the complexity and needs of your specific project.
Stay informed and continue to evolve your testing strategies to reflect the best practices in the industry. Happy testing!
This comprehensive guide serves as a starting point. If you're looking to dive deeper into Java testing, consider exploring Java Testing Best Practices for detailed insights.
Checkout our other articles