Unlocking Opportunities: Java Skills in High Demand
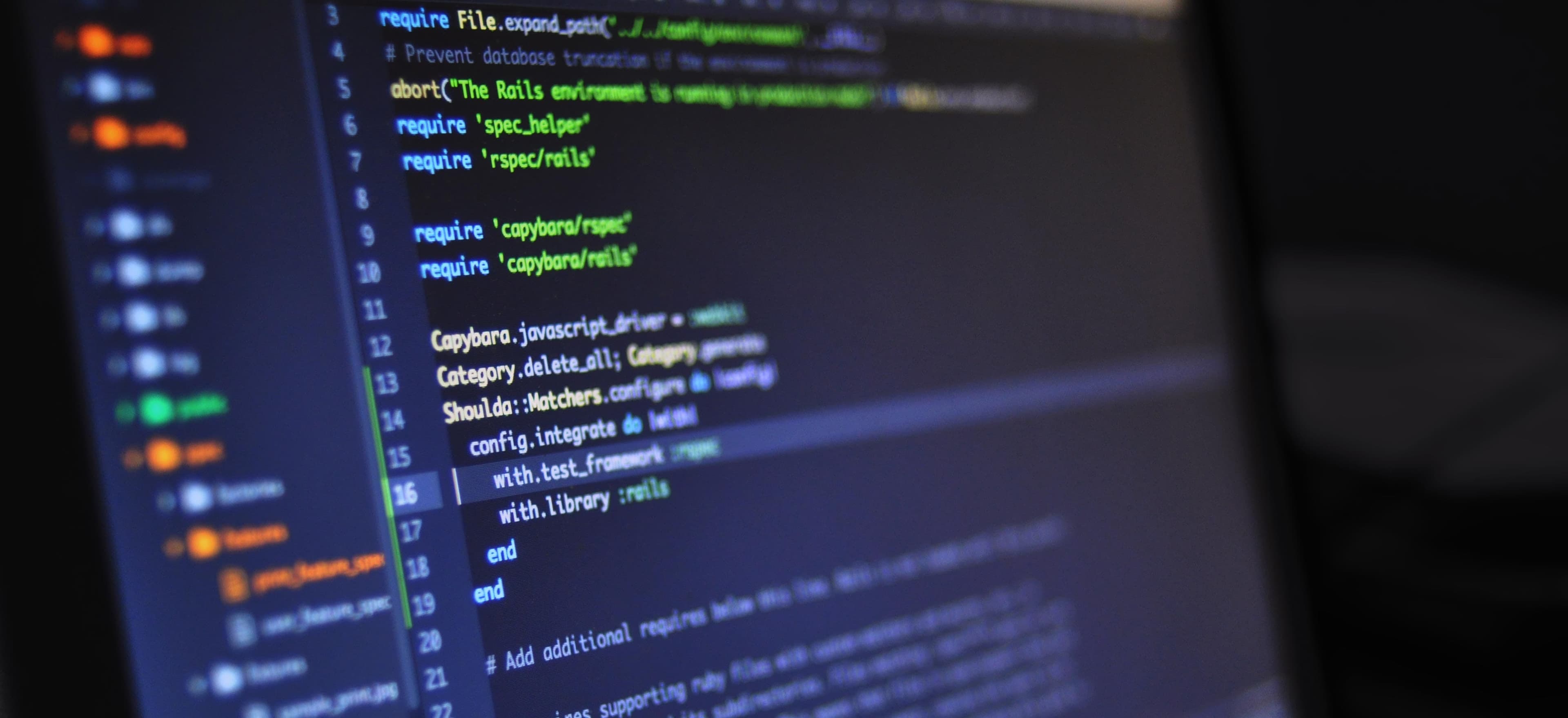
- Published on
Unlocking Opportunities: Java Skills in High Demand
Java has long been a leading programming language, renowned for its versatility, portability, and robust community support. Since its inception in the mid-1990s, Java has evolved significantly, but its core philosophy—the "write once, run anywhere" (WORA) principle—has remained steadfast. In this blog post, we will delve into the current state of Java in the job market and explore the key skills that employers seek. Let's unlock the opportunities Java skills can provide for developers in today's fast-paced tech landscape.
Why Java?
Before we dive into the specific skills, it's important to highlight why Java remains in demand:
- Wide Applicability: Java is used across various domains, from web applications to mobile apps and enterprise software systems.
- Strong Ecosystem: Java boasts a range of libraries and frameworks, such as Spring and Hibernate, which facilitate robust application development.
- Enterprise Ready: Many large organizations prefer Java for its stability and performance, particularly in backend systems.
Core Java Skills Employers Look For
To be competitive as a Java developer, you need to hone a variety of skills. Here are some of the most sought-after competencies:
1. Java Fundamentals
A solid understanding of Java fundamentals is essential:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Why: The above simple program showcases the basic syntax of Java. Employers expect candidates to have a strong grasp of variables, control structures, data types, and object-oriented principles.
2. Object-Oriented Programming (OOP)
Mastering OOP principles is crucial for any Java developer:
- Encapsulation: Bundling data with methods that operate on the data.
- Inheritance: Mechanism of basing an object or class upon another object or class.
- Polymorphism: Ability for different classes to be treated as instances of the same class.
Example of OOP in Java:
class Animal {
void speak() {
System.out.println("Animal speaks");
}
}
class Dog extends Animal {
void speak() {
System.out.println("Woof!");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.speak(); // Outputs: Woof!
}
}
Why: OOP promotes code reusability and scalability, something highly valued in large systems.
3. Understanding of Java Frameworks
Frameworks streamline Java development. Some popular ones include:
- Spring: For building robust applications and microservices.
- Hibernate: For database interactions using Object-Relational Mapping (ORM).
For example, using Spring's Dependency Injection:
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class App {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class);
MyBean bean = context.getBean(MyBean.class);
bean.doSomething();
}
}
Why: Understanding these frameworks allows for faster development cycles and integration with various systems, giving candidates a significant edge.
4. Database Knowledge
Java applications often require integration with databases. Knowledge of SQL and understanding of Java Database Connectivity (JDBC) is essential.
Here’s a simple JDBC example:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class JdbcExample {
public static void main(String[] args) {
try {
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password");
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM users");
while (resultSet.next()) {
System.out.println(resultSet.getString("name"));
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why: Proficiency in database interaction is critical, as most applications require data management.
5. Understanding of Web Technologies
In the era of web applications, understanding the basics of HTML, CSS, and JavaScript is beneficial. In addition, familiarity with Java-based web technologies like Servlets and JSP is essential.
Example of a simple Servlet:
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
public class HelloServlet extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<h1>Hello, World!</h1>");
}
}
Why: Web applications form a significant portion of the software landscape. Understanding how Java integrates with web technologies is imperative.
6. Familiarity with Version Control Systems
Git has become the de facto standard for version control. Understanding how to use Git enhances collaboration and code management.
Basic Git commands:
git init # Initialize a new Git repository
git clone <repo-url> # Clone an existing repository
git add <file> # Stage a file for commit
git commit -m "Commit message" # Commit staged changes
Why: Version control allows developers to manage changes effectively and collaborate seamlessly within teams.
7. Knowledge of Testing Frameworks
With the emphasis on quality assurance, familiarity with testing frameworks such as JUnit and Mockito is a considerable advantage.
Here’s a simple JUnit test:
import static org.junit.Assert.*;
import org.junit.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calc = new Calculator();
assertEquals(5, calc.add(2, 3));
}
}
Why: Testing ensures code reliability and reduces bugs, which is paramount in software development.
Building Your Java Skill Set
As you work on honing the above skills, consider the following strategies:
1. Online Courses and Certifications
Platforms like Coursera, Udemy, and edX offer extensive Java courses. Certifications can serve as a valuable addition to your resume, making you more appealing to employers.
2. Open Source Contributions
Engaging in open-source projects on platforms like GitHub allows you to collaborate with others and gain practical experience.
3. Personal Projects
Building your own applications can help you practically apply what you’ve learned. Aim to create diverse projects that showcase your skill set.
4. Networking
Connecting with professionals through platforms like LinkedIn or attending Java conferences can open doors to job opportunities and collaborations.
Final Considerations
The demand for Java skills shows no signs of dissipating, making it a wise investment for aspiring and existing developers alike. As long as you continue to grow your skills and adapt to new technologies within the Java ecosystem, you'll unlock numerous opportunities in the world of software development.
Being in tune with the evolving landscape of Java and the associated skills is crucial for professional growth. Whether you're a beginner or an experienced developer, focusing on these key skills will not only enhance your job prospects but also equip you to tackle complex development challenges in the future.
Remember, keeping your skill set updated is not just about meeting employer demands but also about staying relevant in this ever-changing tech landscape. Happy coding!
Checkout our other articles