Understanding Hibernate Dirty Checking: Common Pitfalls
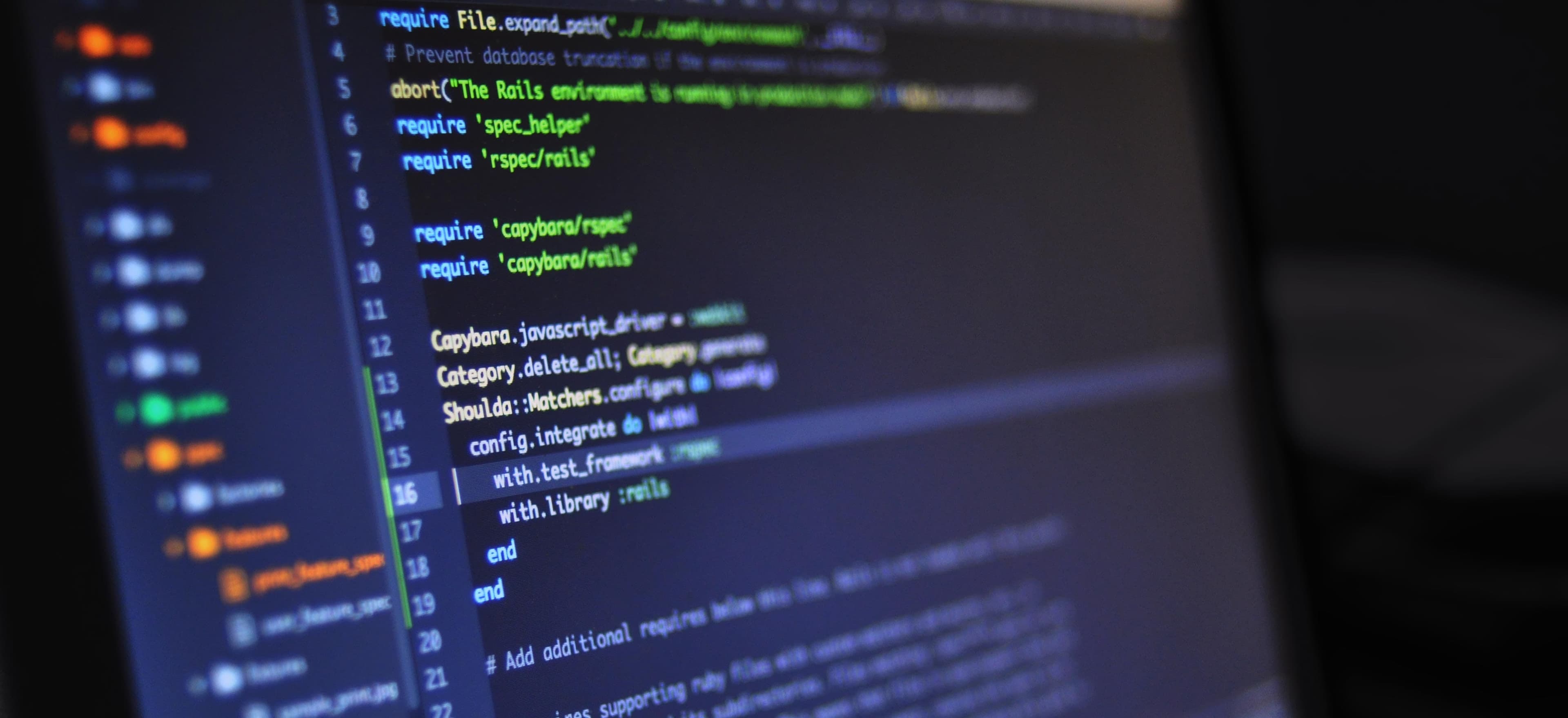
- Published on
Understanding Hibernate Dirty Checking: Common Pitfalls
Hibernate is one of the popular Object-Relational Mapping (ORM) frameworks for Java, allowing developers to manage database records as Java objects. One of its key features is "dirty checking," a performance optimization technique that ensures only modified data is updated in the database. While dirty checking can significantly enhance performance, it also comes with its own set of challenges. In this blog post, we will dive deep into Hibernate dirty checking, identify common pitfalls, and provide tips on how to avoid them.
What is Dirty Checking?
Dirty checking is the process by which Hibernate automatically detects changes made to persistent objects (entities) before committing them to the database. This enables Hibernate to synchronize the in-memory state of your objects with the database, ensuring that only modified entities are updated.
Why is Dirty Checking Important?
- Performance Optimization: By updating only changed records, dirty checking reduces the amount of SQL generated and executed, leading to better application performance.
- Automatic Synchronization: Developers do not need to manually specify which properties have changed, allowing for cleaner and more maintainable code.
How Does It Work?
In Hibernate, dirty checking occurs during the flush phase of a transaction. When the transaction is flushed, Hibernate performs the following actions:
- Comparison: Hibernate compares the current state of the persistent objects with the original snapshot taken when the entity was loaded.
- Change Detection: Hibernate identifies which fields or properties have changed by checking their values.
- SQL Generation: If changes are detected, Hibernate generates the necessary SQL update statements to reflect these modifications in the database.
Example Code Snippet: Basic Dirty Checking
Here is a simple example to demonstrate Hibernate dirty checking:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// Getters and Setters
}
// Updating a User
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
User user = session.get(User.class, userId);
user.setEmail("newemail@example.com"); // Change the email
transaction.commit();
session.close();
Why This Works: When the commit()
method is called, Hibernate detects that the email
field of the User
object has been modified. Only the relevant SQL UPDATE
statement is generated, ensuring that performance impacts are minimized.
Common Pitfalls of Dirty Checking
While dirty checking is powerful, it often leads to confusion. Here are some common pitfalls:
1. Forgetting to Implement equals()
and hashCode()
Not implementing equals()
and hashCode()
can lead to unexpected behavior when Hibernate performs dirty checking. If an entity does not properly implement these methods, comparisons may produce false positives.
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof User)) return false;
User user = (User) o;
return Objects.equals(id, user.id);
}
@Override
public int hashCode() {
return Objects.hash(id);
}
Why This is Important: These methods allow Hibernate to correctly identify instances of entities when checking for changes, preventing errors during updates.
2. Incorrect Session Management
Closing the Hibernate session too early can result in incomplete dirty checking. If the session is closed before modifications are flushed, changes won't be recognized.
Best Practice: Always ensure that the session remains open until the transaction is completed. Use try-with-resources to better manage session lifecycles.
try (Session session = sessionFactory.openSession()) {
Transaction transaction = session.beginTransaction();
// Your entity operations here
transaction.commit();
}
3. Not Understanding Cascade Types
Cascade types dictate how persistence operations propagate from parent entities to child entities. If a parent entity is marked for REMOVE
, the children won't necessarily be saved unless properly handled, resulting in lost updates.
Solution: Always review the cascade types you are using, especially for relationships. For example:
@OneToMany(cascade = CascadeType.ALL)
private List<Order> orders; // Orders are automatically persisted or removed
4. Overusing merge()
Using the merge()
method indiscriminately can lead to unnecessary updates and override changes made during a session. It effectively detaches the current entity, generates a new one, and merges the states.
Recommended Syntax:
Opt for session.update(entity)
instead of session.merge(entity)
when you know that the entity is already attached to the session.
Example:
session.update(user); // Updates user directly
5. Mismanaging Detached Entities
Detached entities are not automatically dirty-checked. If you need to re-attach an entity after it has been detached, you must ensure proper management to avoid stale state snapshots.
Strategy: Use session.lock(entity, LockMode.NONE)
to refresh the state of the detached entity.
Tips for Avoiding Dirty Checking Issues
-
Understand Your Entity Lifecycle: Know when and how entities transition between managed and unmanaged states (transient, persistent, detached).
-
Optimize Your Mappings: Use appropriate fetch types such as
FetchType.LAZY
to prevent unnecessary loading of related entities. -
Implement Proper Transaction Boundaries: Always ensure that your transactions are appropriately defined to enclose only relevant entity operations.
-
Test Thoroughly: Create unit tests that specifically check for entity changes and database updates. This will help identify issues early.
-
Utilize Logging: Enable Hibernate SQL logging to gain insight into the generated SQL statements and understand what's happening behind the scenes.
Lessons Learned
Hibernate's dirty checking is a powerful mechanism that can significantly improve performance by optimizing database access. However, it comes with its own set of challenges. By understanding these common pitfalls and implementing best practices, developers can avoid running into pitfalls and ensure that their applications run smoothly.
For further reading on JDBC performance optimizations, HikariCP is a great resource. Additionally, if you're looking to enhance your understanding of ORM, you can explore the Hibernate documentation for its extensive guide on session management and transaction handling.
By addressing the potential pitfalls of dirty checking and embracing best practices, you can harness the full power of Hibernate to build efficient and robust Java applications. Happy coding!
Checkout our other articles