Common Pitfalls in Integrating GWT, Spring, and Hibernate
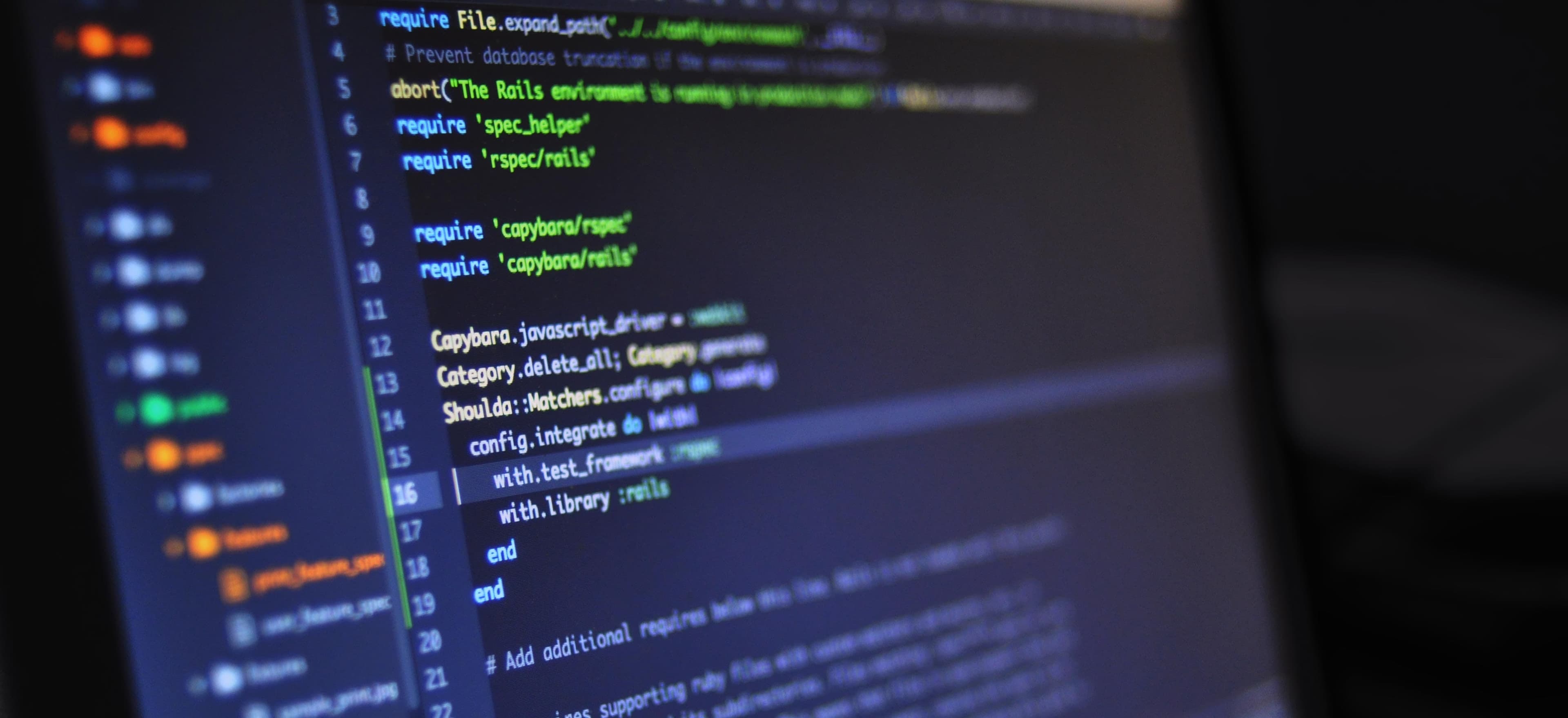
- Published on
Common Pitfalls in Integrating GWT, Spring, and Hibernate
Integrating Google Web Toolkit (GWT), Spring Framework, and Hibernate can open doors to rich web applications that leverage the strengths of each technology. However, this integration is not without its challenges. In this blog post, we will discuss common pitfalls encountered when working with these technologies and offer solutions to ensure a smoother integration experience.
Understanding the Technologies
Google Web Toolkit (GWT)
GWT allows developers to write client-side code in Java, which is then compiled into optimized JavaScript. This lets you build complex web applications while utilizing Java's powerful features.
Spring Framework
Spring, a powerful framework for building enterprise applications, offers a wide range of features including dependency injection, aspect-oriented programming, and comprehensive transaction management. It simplifies the development of Java applications while maintaining flexibility.
Hibernate
Hibernate is an Object-Relational Mapping (ORM) tool that facilitates the mapping of Java objects to database tables. It handles the complexities of database interactions, allowing developers to focus on business logic.
Common Pitfalls and Solutions
1. Improper Event Handling in GWT
Pitfall: When integrating GWT client-side events with Spring's server-side components, you might end up handling events improperly. This can lead to unexpected behavior, such as failing to fetch data from the server or not updating the UI correctly.
Solution: Use asynchronous service calls in GWT and ensure proper error handling. GWT provides AsyncCallback
to handle responses from the server effectively.
public void loadData() {
myServiceAsync.loadData(new AsyncCallback<List<Data>>() {
public void onFailure(Throwable caught) {
Window.alert("Failed to load data: " + caught.getMessage());
}
public void onSuccess(List<Data> result) {
// Update UI with fetched data
updateUI(result);
}
});
}
This structure ensures that your application will not hang indefinitely waiting for a response, giving users feedback if things go awry.
2. Mismanaging Spring Bean Scopes
Pitfall: Spring supports various bean scopes (singleton, prototype, request, session). Misconfiguration can lead to memory leaks or application state issues.
Solution: For web applications, favor session-scoped beans for user-specific data and request-scoped beans for data that doesn’t need to persist longer than the lifecycle of a request.
@Service
@RequestScope
public class MyRequestScopedService {
// logic for handling request-specific data
}
This ensures that each HTTP request gets its instance, avoiding state leakage across requests while conserving resources.
3. Hibernate Lazy Loading Issues
Pitfall: Lazy loading in Hibernate can lead to the "LazyInitializationException" if accessed outside of an active session. This commonly occurs when GWT requests data that has not been fully fetched due to lazy loading.
Solution: Eagerly fetch associations that you know you will need or manage your sessions properly to avoid closing them before accessing lazy-loaded entities.
@Entity
public class Parent {
@OneToMany(fetch = FetchType.EAGER)
private List<Child> children;
// other properties and methods
}
Using eager fetching ensures that "children" are fully loaded when retrieving the "Parent" object, preventing runtime exceptions.
4. Overcomplicating the Architecture
Pitfall: A common mistake is overengineering the integration by adding too many layers of abstraction, making the system harder to maintain.
Solution: Keep your architecture simple. Start with a straightforward design that allows you to manage basic features and then incrementally add complexity as needed based on actual requirements and pain points.
Refer to the Spring documentation for best practices on architecture design.
5. Ignoring Security Aspects
Pitfall: Not focusing on security can leave your application vulnerable to attacks such as SQL injection, CSRF, or XSS.
Solution: Implement security measures at both the client and server sides. For Spring applications, leverage Spring Security to manage authentication and authorization effectively.
Here's a basic configuration:
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/", "/home").permitAll()
.anyRequest().authenticated()
.and()
.formLogin().loginPage("/login").permitAll()
.and()
.logout().permitAll();
}
}
This configuration ensures that only authenticated users can access protected resources.
6. Data Transfer Object (DTO) Mismanagement
Pitfall: Directly exposing your Hibernate entities to the GWT layer can lead to unintended data exposure and increased coupling.
Solution: Use Data Transfer Objects (DTOs) to transfer data between layers. This encapsulates your data and reduces dependency on the underlying entity structure.
public class UserDTO {
private String username;
private String email;
// Getters and setters
}
Implementing DTOs shields your application from changes in the database schema and enhances security by allowing you to expose only necessary fields.
The Closing Argument
Integrating GWT, Spring, and Hibernate can greatly enhance the efficiency and performance of your web applications. However, it requires careful planning and execution to avoid the common pitfalls outlined above. By implementing best practices such as proper event handling, managing bean scopes effectively, and focusing on security, you can significantly mitigate issues that arise during integration.
For further reading, check these resources:
- GWT Official Documentation
- Spring Framework Guide
- Hibernate User Guide
With diligence and awareness of these common pitfalls, you can ensure a successful integration of GWT, Spring, and Hibernate, leading to robust web applications that serve your users well. Happy coding!
Checkout our other articles