Common SOA Tooling Integration Mistakes in JBoss Developer Studio
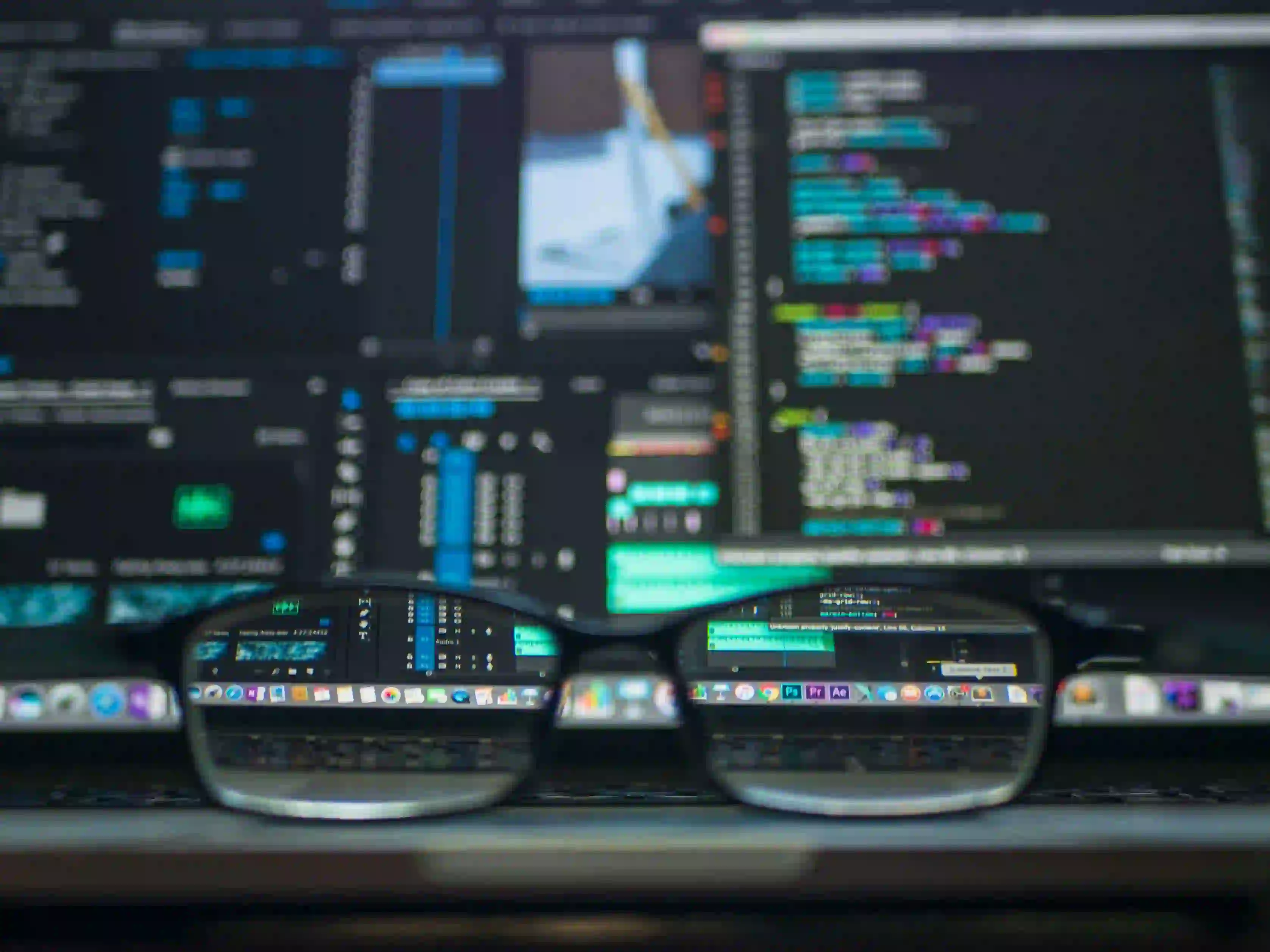
Common SOA Tooling Integration Mistakes in JBoss Developer Studio
Integrating Service-Oriented Architecture (SOA) tools in JBoss Developer Studio (JDS) can be a daunting task, especially for developers who are new to SOA concepts or the JBoss ecosystem. This blog post aims to shed light on the common mistakes one can encounter during this process, with illustrative examples and code snippets. We'll also discuss best practices to ensure a smooth and efficient integration experience.
Understanding SOA and JBoss Developer Studio
Before diving into the common mistakes, it's crucial to understand what SOA is and how JBoss Developer Studio fits into the picture.
What is SOA?
SOA is an architectural principle that focuses on building application components as reusable services. It enables disparate systems to communicate and collaborate over a network through predefined interfaces. This flexibility allows for more agile development, easier maintenance, and better scalability.
What is JBoss Developer Studio?
JBoss Developer Studio is an Integrated Development Environment (IDE) tailored for developing applications using JBoss technologies, such as Red Hat JBoss EAP and JBoss Fuse. It provides tools that simplify the development of SOA solutions, including support for Service Component Architecture (SCA), Service Data Objects (SDO), and more.
Common Mistakes in SOA Tooling Integration
1. Ignoring Service Contract Definitions
One of the biggest errors developers make is neglecting to define clear service contracts. A service contract spells out how different services interact with each other. Not defining it leads to ambiguity and misunderstanding about data formats, endpoints, and protocols.
Example Mistake: Assuming service A can communicate with service B without a defined contract.
Solution: Utilize WSDL (Web Services Description Language) or XML Schema Definitions to clearly define service contracts.
<definitions xmlns="http://schemas.xmlsoap.org/wsdl/"
xmlns:tns="http://www.example.com/MyService"
targetNamespace="http://www.example.com/MyService">
<message name="getDataRequest">
<part name="request" type="tns:DataRequestType"/>
</message>
<message name="getDataResponse">
<part name="response" type="tns:DataResponseType"/>
</message>
<portType name="MyServicePortType">
<operation name="getData">
<input message="tns:getDataRequest"/>
<output message="tns:getDataResponse"/>
</operation>
</portType>
</definitions>
Why this Matters: A well-defined service contract helps maintain consistent communication and ensures each service meets the agreed-upon specifications.
2. Overlooking Error Handling Strategies
Error handling is critical in SOA integration, and many developers either overlook it or implement it poorly. As systems are interconnected, failures can occur at multiple levels, resulting in cascading failures if not adequately handled.
Example Mistake: Not leveraging established error handling patterns or frameworks.
Solution: Utilize JBoss SwitchYard or implement robust error handling mechanisms using try-catch blocks and logging.
public void processServiceOperation(String input) {
try {
// Call to an external service
callExternalService(input);
} catch (ServiceException e) {
logError(e);
// Implement fallback logic
performFallback();
}
}
Why this Matters: Good error handling increases system reliability and enhances user experience by providing meaningful feedback instead of generic error messages.
3. Not Testing the Integrated Services
Integration testing is vital in SOA. Failing to conduct proper tests can potentially lead to expensive errors down the line.
Example Mistake: Skipping end-to-end integration tests because unit tests are deemed sufficient.
Solution: Establish a comprehensive testing framework that includes unit, integration, and acceptance tests.
@Test
public void testServiceIntegration() {
// Setup input
String request = "<dataRequest>...</dataRequest>";
// Invoke the service
String response = myService.invoke(request);
// Assert response is valid
assertNotNull(response);
assertTrue(response.contains("<expectedData>"));
}
Why this Matters: Testing not only ensures that your service works as intended but also uncovers integration issues early in the development process, which can save time and resources.
4. Misconfiguring Middleware Components
Another common mistake is not properly configuring middleware components, such as ESBs (Enterprise Service Buses), message brokers, or application servers. Incorrect configurations can hamper performance and lead to service failures.
Example Mistake: Configuring timeouts and retries inefficiently.
Solution: Adjust settings to optimize middleware performance, considering factors like network latency and service availability.
<http:configure>
<http:timeout>30000</http:timeout>
<http:retries>5</http:retries>
</http:configure>
Why this Matters: Proper configuration allows for efficient communication between services, improving overall performance and reducing the chances of failures.
5. Ignoring Security Practices
Security should be an integral part of your SOA strategy. Many developers neglect security best practices, making their services vulnerable to attacks.
Example Mistake: Using unencrypted communication for services that transmit sensitive data.
Solution: Implement secure protocols like HTTPS and utilize OAuth for authentication and authorization.
public class SecureService {
@GetMapping("/secureData")
public ResponseEntity<String> getSecureData(@AuthenticationPrincipal User user) {
// Ensure the user has access
if (!user.hasRole("ROLE_USER")) {
return ResponseEntity.status(HttpStatus.FORBIDDEN).build();
}
// Return secured data
return ResponseEntity.ok("Secure Data");
}
}
Why this Matters: Securing your services protects sensitive information and builds user trust.
Closing the Chapter
Integrating SOA tooling in JBoss Developer Studio can be complex, but by being aware of these common pitfalls, developers can enhance their integration strategies. Proper service contract definitions, robust error handling, comprehensive testing, thoughtful configuration, and strict security measures are all integral to successful SOA implementation.
For further reading on SOA and integration best practices, check out Red Hat's comprehensive guide on SOA and Java error handling techniques.
By diligently applying the suggestions in this post, you will be well on your way to mastering SOA integration in JBoss Developer Studio. Happy coding!