Troubleshooting Java 8 Build Errors: A Complete Guide
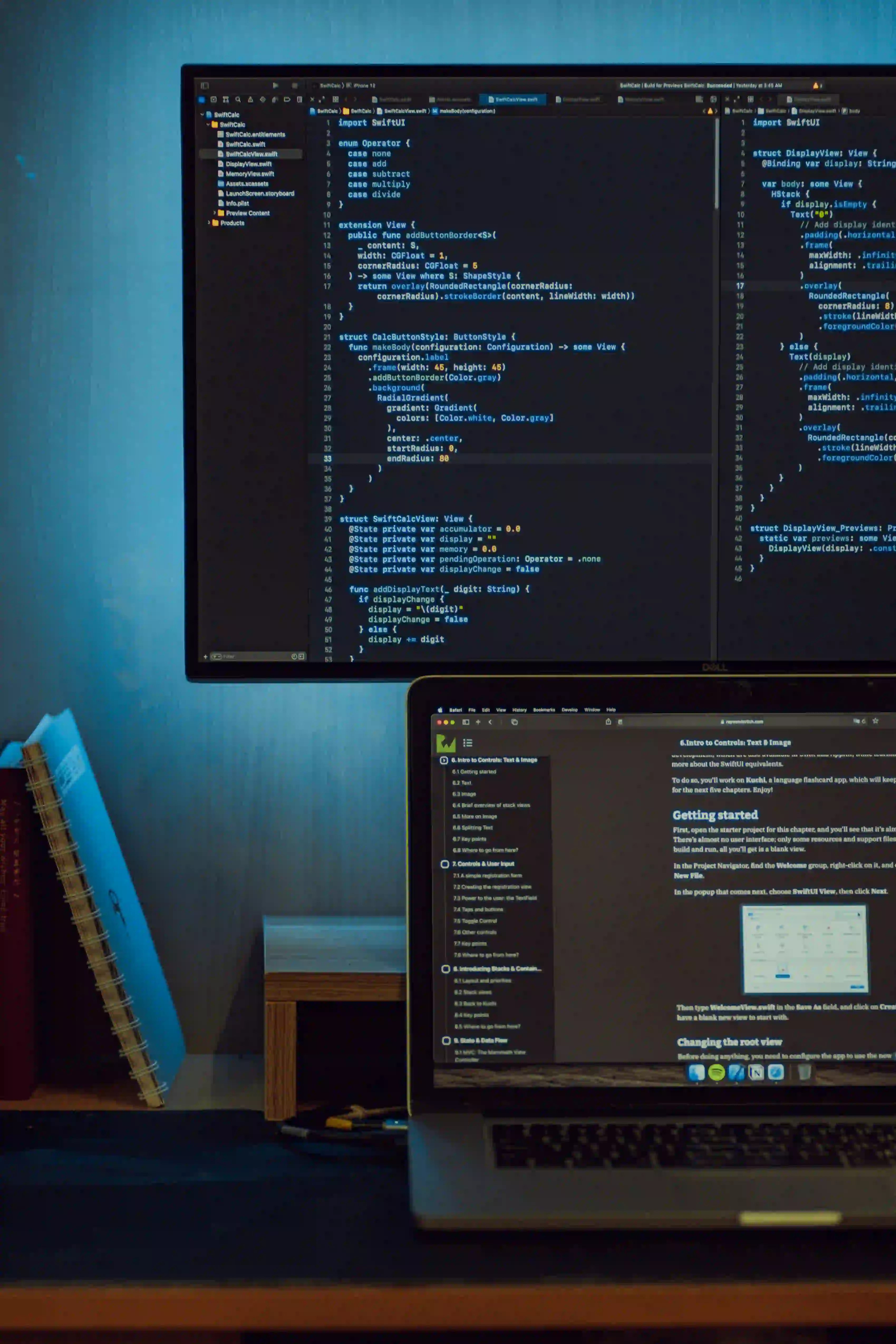
Troubleshooting Java 8 Build Errors: A Complete Guide
Building Java applications can often feel like uncharted territory. As developers, we regularly encounter a myriad of build errors that can halt our progress and significantly affect our productivity. This guide aims to provide you with a structured approach to troubleshoot Java 8 build errors effectively and to help streamline your development workflow.
Understanding the Build Process
Before we dive deep into troubleshooting, it's essential to understand what occurs during the Java build process. Here's a succinct overview:
- Compilation: Java source files (.java) are compiled into bytecode (.class).
- Testing: Unit tests are run to ensure functionality.
- Packaging: The compiled files are packaged into JAR or WAR files for deployment.
- Deployment: The packaged files are deployed to an environment, where they can be executed.
When any of these stages encounter an issue, errors will arise.
Common Errors and Their Solutions
Let's break down some of the most common Java 8 build errors and how you might resolve them.
1. Missing Dependencies
One prevalent error developers face is the ClassNotFoundException
. This often occurs because the necessary libraries aren't included in the build path.
Solution: Ensure that all required libraries are properly included.
Here's a quick example using Maven:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>4.3.9.RELEASE</version>
</dependency>
In this example, we declare a Spring Framework dependency. Missing this dependency can lead to a ClassNotFoundException during runtime. Always double-check your pom.xml
for the required dependencies.
2. Version Conflicts
Another frequent issue arises from dependency version conflicts. Java projects often build on libraries that have different versions. If two libraries require different versions of the same dependency, you might face a problem known as "dependency hell."
Solution: Use a dependency management tool, like Maven, to exclude unwanted versions.
Here's how you might exclude a version:
<dependency>
<groupId>com.example</groupId>
<artifactId>library-a</artifactId>
<version>1.0</version>
<exclusions>
<exclusion>
<groupId>org.some</groupId>
<artifactId>conflicting-library</artifactId>
</exclusion>
</exclusions>
</dependency>
This snippet shows how to exclude a troublesome dependency explicitly rolled in by another library. By managing your dependencies wisely, you can save time and prevent build errors.
3. Compiler Errors
Compiler errors can arise from syntax issues or type mismatches. Common examples include missing semicolons, unmatched braces, or type incompatibilities.
Solution: Read the error message carefully and resolve the issue in your code.
Consider the following code segment:
public class Example {
public static void main(String[] args) {
System.out.println("Hello, World!"
}
}
The error here is a missing semicolon after the System.out.println
statement. The compiler provides helpful feedback that can guide you in finding the mistake.
4. Java Version Issues
Sometimes, you might encounter issues related to the Java version, especially if your code uses newer Java features, but you are using Java 8.
Solution: Ensure you are compiling your code with the Java 8 SDK.
Check your Java version in your terminal or command prompt:
java -version
If it’s not installed correctly, consider downloading it from the Oracle official site.
5. IDE Configuration Issues
Integrated Development Environments (IDEs) can sometimes cause conflicts due to misconfiguration. You might face errors related to project setup or SDK paths.
Solution: Double-check your IDE settings.
For IntelliJ IDEA, ensure that the SDK is set to the correct version:
- Go to
File -> Project Structure
. - Ensure that the Project SDK is set to Java 8.
- Check the Modules section to verify that each module also targets the correct SDK.
Best Practices for Troubleshooting
While specific errors can often lead to confusion, there are general practices that can be extremely beneficial.
1. Read Error Messages Carefully
Error messages often contain line numbers and descriptions. Follow the provided pointers precisely; they are your first line of defense against confusion.
2. Divide and Conquer
If you’re facing multiple errors, tackle them one at a time. Isolate the problems, fix them individually, and then re-run the build.
3. Utilize Online Resources
Don't hesitate to consult the community. Forums like Stack Overflow offer a wealth of information. Chances are, someone else has faced the same issue.
4. Use Version Control
Maintain a version of your code that you know is stable. This allows you to roll back to a working version when you encounter errors.
Key Takeaways
Troubleshooting Java 8 build errors can feel like a daunting task, but with a systematic approach, it becomes manageable. Understanding your build environment, properly organizing dependencies, and reading error messages effectively are vital skills that will improve your resilience as a developer.
Remember, build errors are opportunities to learn and grow. With the right tools and resources at your disposal, you can overcome these challenges and continue producing robust Java applications.
Feel free to share your thoughts and experiences in the comments below. Let's enhance our knowledge collaboratively!
For further reading about managing dependencies, check out the Maven documentation. Also, being aware of troubleshooting techniques can greatly help; find more about it at Java Build Tools Overview. Happy coding!