Mastering Lazy Sequences: Boosting Java 8 Performance
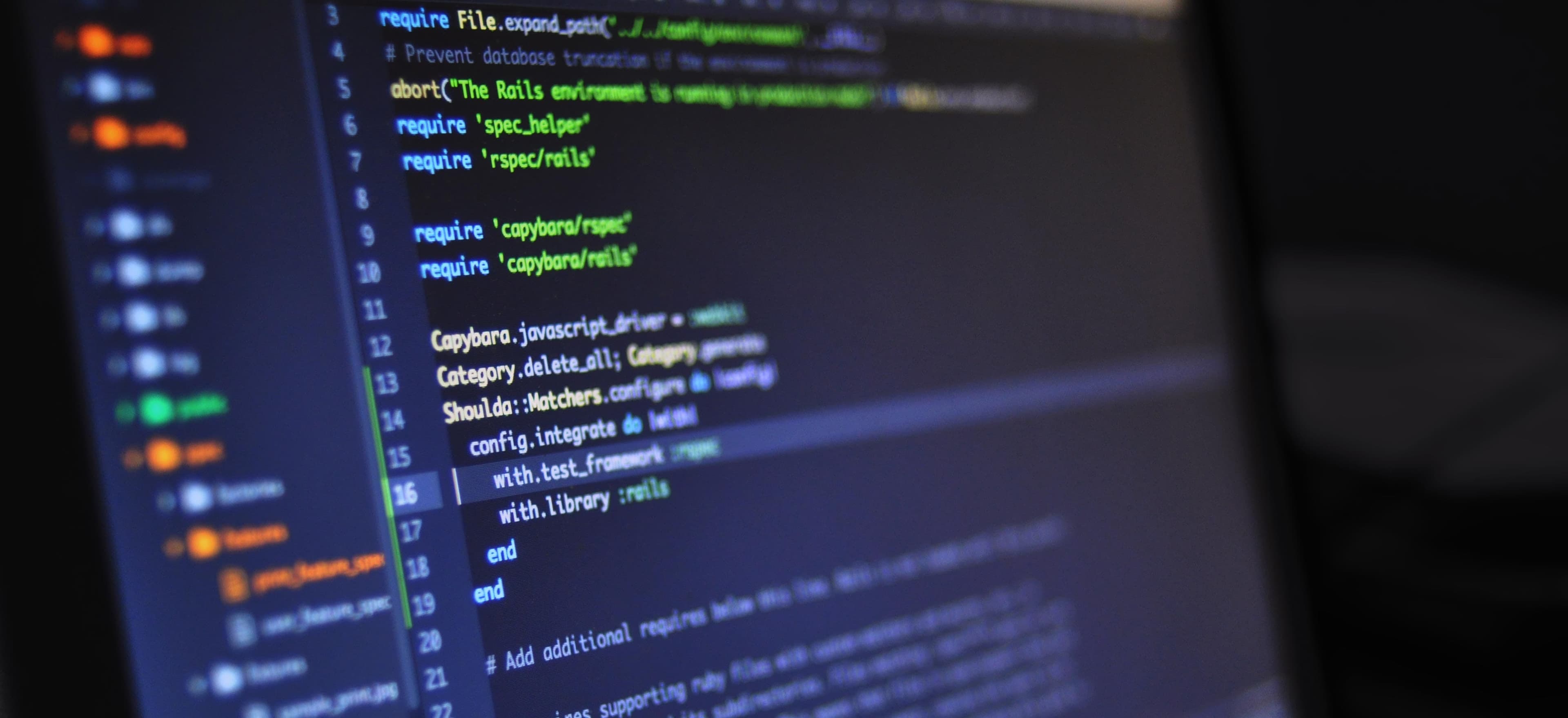
- Published on
Mastering Lazy Sequences: Boosting Java 8 Performance
Java 8 was a revolutionary milestone in the evolution of Java programming. It introduced several powerful features, including lambda expressions, the Stream API, and optional types. Among these features, one of the most valuable is lazy evaluation and lazy sequences. In this blog post, we will delve into the concept of lazy sequences in Java 8, how they work, and the performance optimizations they bring to your applications.
What Are Lazy Sequences?
Lazy sequences are collections or sequences that are not computed until they are specifically needed. This concept can lead to significant performance improvements, particularly when dealing with large data sets or expensive operations.
In Java, lazy evaluation allows you to define a series of transformations without needing to compute intermediate results right away. As a result, you can manage memory more efficiently and improve performance by reducing unnecessary computations.
The Power of Streams
Java 8 introduced the Stream API, which represents a sequence of elements supporting sequential and parallel aggregate operations. Stream operations can be either intermediate (which return a new Stream) or terminal (which produce a non-Stream result, such as a primitive value, a collection, or no value at all).
Here’s an example that demonstrates the distinction between intermediate and terminal operations using Streams:
Code Example 1: Streams Basics
import java.util.Arrays;
import java.util.List;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Intermediate operations: filter and map
numbers.stream()
.filter(n -> n % 2 == 0) // Keep even numbers
.map(n -> n * n) // Square each number
.forEach(System.out::println); // Terminal operation: print the results
}
}
Commentary
In the code above, filter
and map
are intermediate operations. They do not process the elements until a terminal operation is invoked (forEach
in this case). This delayed computation is the essence of lazy evaluation.
Why Use Lazy Evaluation?
-
Improved Performance: By only computing values when necessary, you can avoid excessive computations that contribute no useful information to your final result.
-
Memory Efficiency: When working with large data sets, lazy evaluation reduces memory consumption. Only the required elements are loaded, avoiding unnecessary storage of intermediate results.
-
Composability: Lazy sequences can be easily combined with other operations, creating flexible workflows without sacrificing performance.
Let’s dive deeper into some specific use cases where lazy sequences shine.
Use Cases for Lazy Evaluation
1. Processing Large Data Sets
Consider a scenario where you need to process a massive collection of user-generated data, such as logs or statistics. Using lazy sequences allows you to filter and transform only the relevant parts of the data without loading everything into memory.
Code Example 2: Processing Large Data Sets
import java.util.stream.Stream;
public class LargeDataSet {
public static void main(String[] args) {
// Simulating a large range of numbers
Stream<Integer> largeStream = Stream.iterate(0, n -> n + 1).limit(1_000_000);
largeStream
.filter(n -> n % 2 == 0) // Keep even numbers
.map(n -> n * n) // Square the numbers
.limit(100) // Take the first 100 results
.forEach(System.out::println);
}
}
Commentary
In this example, we simulate a large data set using an infinite stream with Stream.iterate
. Only even numbers that have been squared are computed, and we limit the output to the first 100 results. The lazy evaluation enables processing an otherwise overwhelming dataset efficiently.
2. Chaining Operations
The ease of chaining multiple Stream operations is a significant advantage of lazy sequences. This chaining is possible because of the lazy nature of Streams, where each intermediate operation is linked together until a terminal operation is called.
Code Example 3: Chaining Operations
import java.util.Arrays;
import java.util.List;
public class ChainingExample {
public static void main(String[] args) {
List<String> words = Arrays.asList("Java", "Stream", "Lazy", "Evaluation", "Performance");
long count = words.stream()
.filter(word -> word.length() > 4) // Filters words longer than 4 characters
.map(String::toUpperCase) // Converts filtered words to uppercase
.count(); // Terminal operation to count
System.out.println("Count of long words: " + count);
}
}
Commentary
In this case, the chain of operations elegantly transforms the data, producing a count of filtered, uppercased words. Each operation works lazily, meaning no unnecessary transformation occurs until we call the count()
operation.
Performance Considerations
Although lazy sequences can significantly improve performance, understanding when and how to use them properly is crucial.
1. Avoiding Early Evaluation
Do not perform operations that might cause early evaluation unintentionally. For example, calling collect()
on a Stream leads to immediate processing, negating the benefits of lazy evaluation.
2. Parallel Streams
Utilizing parallel streams can further enhance performance in scenarios that benefit from concurrent processing. However, be mindful of the overhead associated with parallelization, as it depends on the specific task and environment.
Code Example 4: Using Parallel Streams
import java.util.stream.IntStream;
public class ParallelStreamExample {
public static void main(String[] args) {
int total = IntStream.rangeClosed(1, 1_000_000)
.parallel() // Activate parallel processing
.filter(n -> n % 2 == 0)
.map(n -> n * n)
.sum(); // Terminal operation: sum of squares
System.out.println("Total sum of squares of even numbers: " + total);
}
}
Commentary
In this example, the use of .parallel()
allows us to leverage multiple CPU cores for processing. For tasks capable of parallelization, this approach can reduce execution time considerably.
Lessons Learned
Mastering lazy sequences in Java 8 through the Stream API is a vital skill for developers aiming to write efficient and high-performing applications. Lazy evaluation allows you to handle large datasets more effectively, enabling improved performance and memory management.
By utilizing the concepts of lazy evaluation creatively and understanding when to apply them, you can enhance your Java applications significantly. Remember that each application has different requirements, and while lazy evaluation has many advantages, it's essential to analyze and test performance as needed.
For further reading, you might explore the official Java Documentation on Streams or delve into functional programming concepts in Java.
Harnessing the power of lazy sequences effectively can substantially boost your Java 8 application's performance, making it a crucial aspect of modern software development. Happy coding!