Solving Classpath Confusion: A Guide to JHades
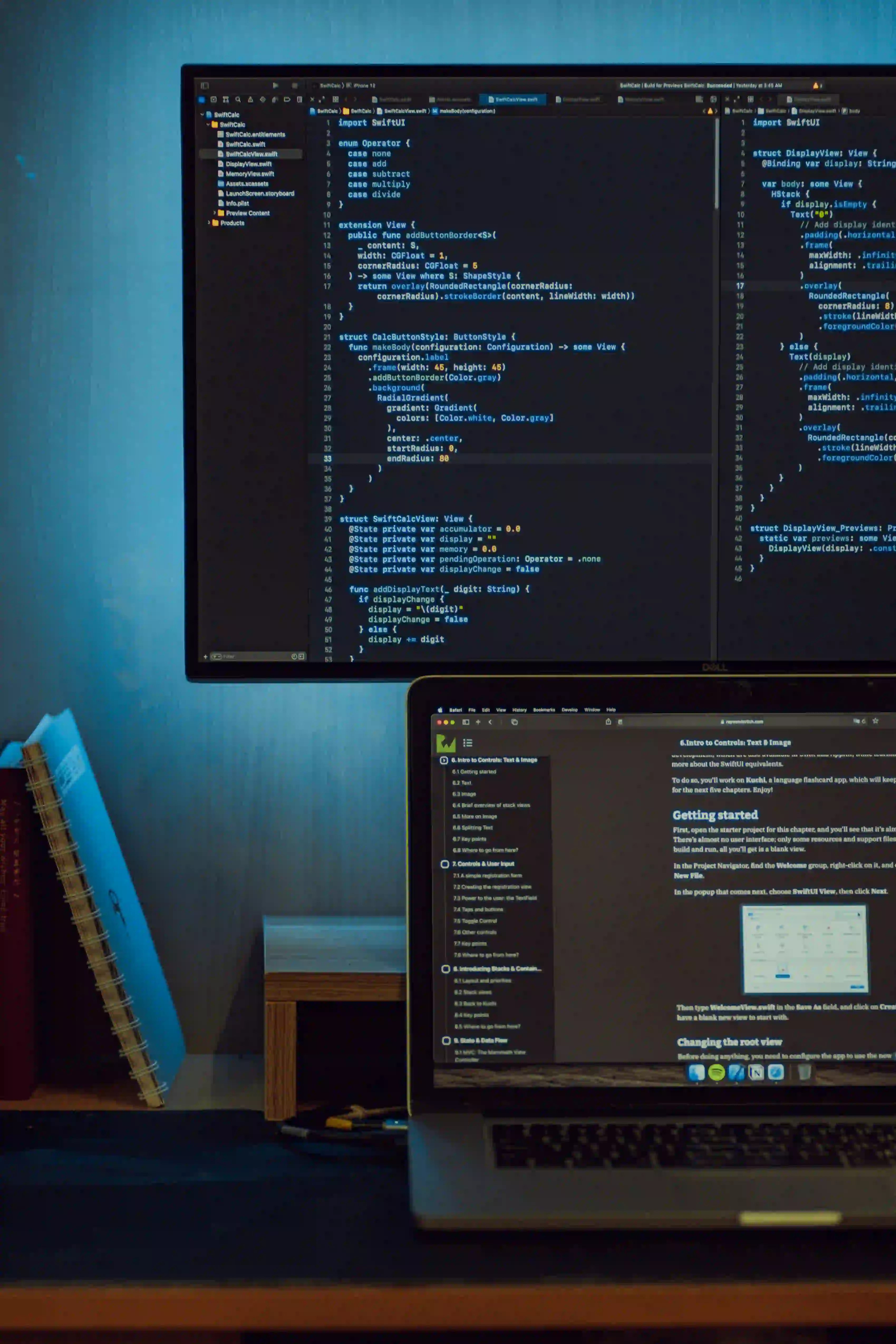
Solving Classpath Confusion: A Guide to JHades
Classpath issues are a common headache for Java developers. When things are not working as expected, the culprit is often found lurking in the vast jungle of classpath settings. However, with the right tools, such as JHades, you can easily navigate this complexity and streamline your development process.
In this blog post, we will delve into the benefits of using JHades, how to set it up, and practical examples that illustrate its power in resolving classpath conflicts.
What is JHades?
JHades is an open-source Java debugging tool designed to help developers analyze and resolve classpath issues. It empowers you to quickly spot conflicts between different versions of libraries, duplicated classes, and other classpath-related problems that can arise during Java development.
Whether you're working on a small project or a large enterprise application, JHades provides valuable insights into your classpath, helping you to prevent bugs that would otherwise take hours to track down.
Getting Started with JHades
Installation
JHades can be easily integrated into your existing project. You can either download it from the JHades GitHub repository or add it as a dependency in your build tool.
Maven
Add the following dependency to your pom.xml
:
<dependency>
<groupId>org.jhades</groupId>
<artifactId>jhades</artifactId>
<version>1.4.1</version>
</dependency>
Gradle
Add this line to your build.gradle
file:
implementation 'org.jhades:jhades:1.4.1'
Once you've added JHades to your project, you can start using it to inspect your classpath.
Basic Usage
The fundamental way to use JHades is by examining the classpath when your application starts. You can call JHades in the following way:
import org.jhades.JHades;
public class MyApp {
public static void main(String[] args) {
new JHades().detectClasspathConflicts();
// Your application code
}
}
In the code above, calling detectClasspathConflicts()
will scan the classpath and report any issues. This easy integration allows you to catch conflicts right at the start of your application.
Exploring Classpath Conflicts
Common Issues
Classpath conflicts can manifest in various forms, including:
- Multiple Versions of the Same Library: You might have different versions of a library included unintentionally.
- Duplicated Classes: Two different libraries might contain the same class.
- Incompatible Dependencies: Libraries may depend on different, incompatible versions of the same dependency.
With JHades, you can easily identify these problems. When detectClasspathConflicts()
is invoked, JHades generates a detailed report of all the conflicts, which aids in debugging your code.
Example: Detecting Conflicts
Consider a scenario where you inadvertently included both versions of the library commons-lang3
: version 3.8
and version 3.9
. The following code snippet demonstrates using JHades to discover this conflict:
public class ConflictExample {
public static void main(String[] args) {
new JHades().detectClasspathConflicts();
// Other application logic
}
}
You would run your application, and JHades would output something like:
Conflict detected:
- commons-lang3-3.8.jar (Loaded from <project-dir>/lib)
- commons-lang3-3.9.jar (Loaded from <another-dir>/lib)
This direct output helps you determine the source of the conflict quickly.
Advanced Usage
JHades also provides options to customize your conflict detection process. You can specify certain packages to ignore or include in your scans. This flexibility helps in situations where you know certain libraries might overlap but are intentionally designed to coexist.
Filtering Results
new JHades()
.ignore("org.apache.commons.*") // Ignore common libraries
.detectClasspathConflicts();
With this approach, JHades will only report conflicts related to libraries outside of the specified package, thereby decluttering your report.
Why Choose JHades?
JHades stands out among many debugging tools for Java for several reasons:
- Simplicity: Integrating JHades into your application is straightforward.
- Real-Time Analysis: You receive immediate feedback during startup about classpath issues.
- Customizable: You can configure what JHades scans and report based on your project requirements.
- Open Source: It’s free to use and given the open-source nature, easy to extend or customize should you need unique functionality.
Closing Remarks
Classpath issues are an inevitable part of Java development, but with JHades, you can turn what was once a labor-intensive and frustrating process into a seamless experience. By effectively detecting and resolving conflicts, JHades keeps your focus on writing quality code rather than chasing down classpath phantoms.
You can enhance your development fluidity by leveraging tools like JHades to ensure your libraries and dependencies work together harmoniously. Don't let classpath confusion hinder your productivity—embrace JHades and keep your Java projects running smoothly.
For more detailed understanding of classpath issues and their resolutions, check out Oracle's Java Classloaders Documentation.
Start integrating JHades into your Java projects today and experience the clarity it brings to classpath management!