Overcoming Common Pitfalls in Factory Design Pattern
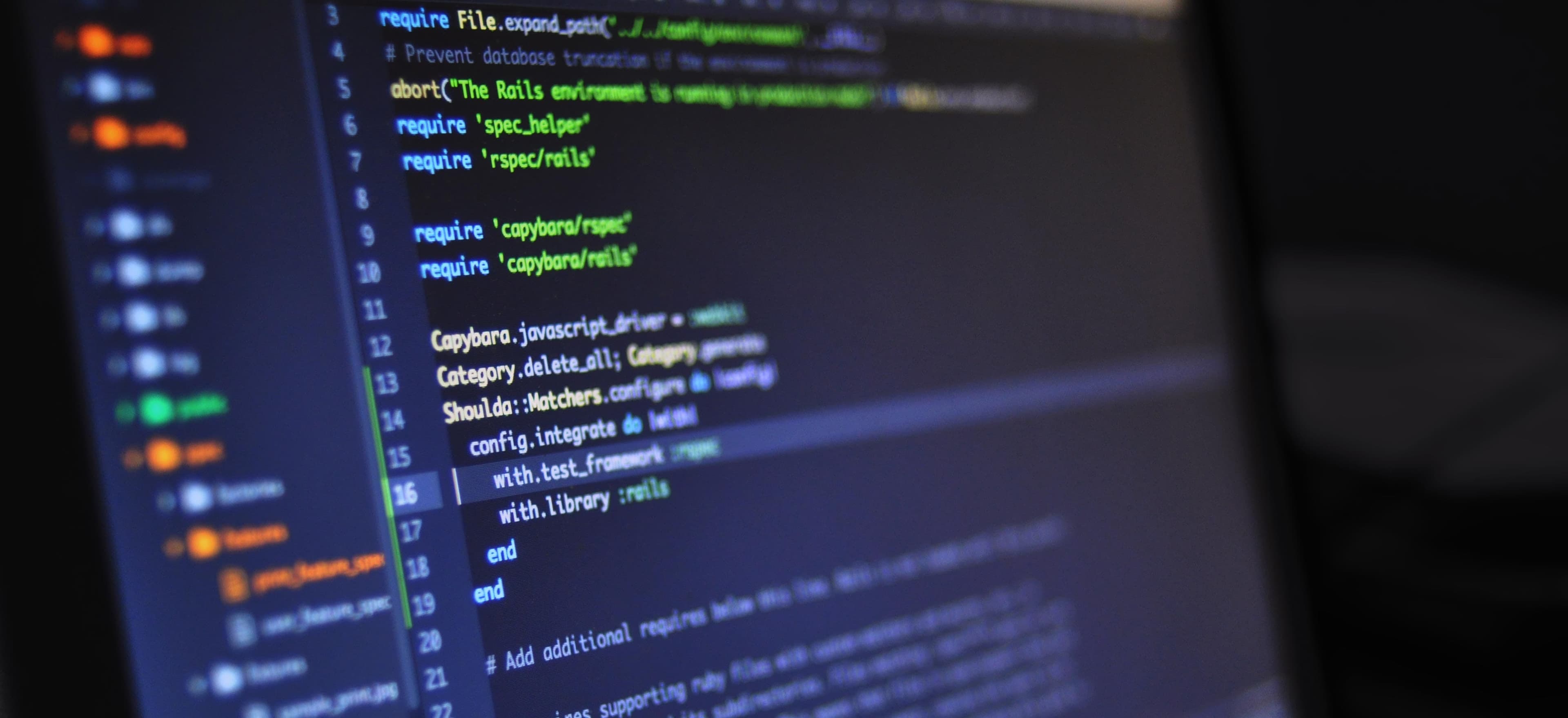
- Published on
Overcoming Common Pitfalls in Factory Design Pattern
The Factory Design Pattern is one of the most widely used design patterns in software engineering, particularly in object-oriented programming. It provides an interface for creating objects in a superclass but allows subclasses to alter the type of objects that will be created. This principle supports the Open/Closed Principle — software entities should be open for extension but closed for modification.
However, while the Factory Design Pattern offers numerous advantages, it is not without its pitfalls. In this blog post, we will discuss common pitfalls in the Factory Design Pattern and strategies to overcome them.
What is the Factory Design Pattern?
Before we dive into the pitfalls, let’s briefly define the Factory Design Pattern. The primary goal of this pattern is to encapsulate the instantiation logic of objects and provide a level of abstraction that allows for flexibility in creating different types of objects without exposing the instantiation logic to the client.
Example of the Factory Pattern
Consider a simple example in Java to illustrate the Factory Design Pattern:
// Step 1: Create an interface Product
interface Product {
void use();
}
// Step 2: Implement Concrete Products
class ConcreteProductA implements Product {
@Override
public void use() {
System.out.println("Using Concrete Product A");
}
}
class ConcreteProductB implements Product {
@Override
public void use() {
System.out.println("Using Concrete Product B");
}
}
// Step 3: Create a Factory Class
class ProductFactory {
public static Product createProduct(String type) {
if ("A".equals(type)) {
return new ConcreteProductA();
} else if ("B".equals(type)) {
return new ConcreteProductB();
}
throw new IllegalArgumentException("Unknown product type: " + type);
}
}
// Step 4: Client code
public class Client {
public static void main(String[] args) {
Product productA = ProductFactory.createProduct("A");
productA.use(); // Outputs: "Using Concrete Product A"
Product productB = ProductFactory.createProduct("B");
productB.use(); // Outputs: "Using Concrete Product B"
}
}
In this example, the ProductFactory
class encapsulates the logic for object creation, allowing the client to create various types of products without knowing the detailed instantiation logic.
Common Pitfalls and How to Overcome Them
While the Factory Design Pattern is powerful, there are several common pitfalls developers can encounter. Here’s a breakdown of the most significant issues and strategies to handle them.
1. Over-Engineering
Pitfall: In some cases, developers use the Factory pattern where simpler solutions would suffice. This can lead to unnecessary complexity in small projects.
Solution: Before implementing the Factory pattern, ask whether it is truly needed. If your application only requires the creation of a single type of object or has a limited number of product types, it may be more practical to instantiate objects directly.
2. Tight Coupling
Pitfall: The Factory class can become tightly coupled with the concrete product classes, making it harder to maintain as your application scales.
Solution: Utilize interfaces, as demonstrated in the earlier code snippets. By depending on abstractions rather than concretions, you can keep your Factory class flexible. Furthermore, consider using Dependency Injection frameworks to manage object creation.
// Example of improved Factory flexibility using Dependency Injection
class ProductService {
private final Product product;
public ProductService(Product product) {
this.product = product;
}
public void execute() {
product.use();
}
}
// Client code with Dependency Injection
public class Client {
public static void main(String[] args) {
Product product = ProductFactory.createProduct("A");
ProductService service = new ProductService(product);
service.execute(); // Outputs: "Using Concrete Product A"
}
}
3. Lack of Clear Responsibilities
Pitfall: Factory classes can end up taking on too many responsibilities — not only creating objects but also handling business logic or managing application state.
Solution: Separate concerns diligently. The Factory’s role should strictly focus on creating instances. If there is a need for additional logic, consider using other patterns, such as the Strategy pattern or the Observer pattern, to manage the behavior and responsibility allocation.
4. Inefficient Object Creation
Pitfall: Using the Factory method can sometimes result in poor performance, especially if the object creation process is expensive or if it involves many checks.
Solution: Implement caching for objects that are frequently created. This becomes especially important when dealing with resources such as database connections or network clients.
import java.util.HashMap;
import java.util.Map;
// Caching example in Factory
class CachedProductFactory {
private static final Map<String, Product> productCache = new HashMap<>();
public static Product createProduct(String type) {
if (productCache.containsKey(type)) {
return productCache.get(type);
}
Product product;
if ("A".equals(type)) {
product = new ConcreteProductA();
} else if ("B".equals(type)) {
product = new ConcreteProductB();
} else {
throw new IllegalArgumentException("Unknown product type: " + type);
}
productCache.put(type, product); // Cache the newly created product
return product;
}
}
5. Scaling the Factory
Pitfall: As new products and subclasses are added, the Factory methods become unwieldy and hard to maintain.
Solution: Follow the Open/Closed Principle. By using reflection or the Service Locator pattern, you can dynamically discover and instantiate classes. Additionally, consider using a configuration file or annotations to define product mappings instead of hardcoding types in the factory.
// Service Locator example
class ServiceLocator {
private static Map<String, Class<? extends Product>> registry = new HashMap<>();
public static void registerProduct(String key, Class<? extends Product> productClass) {
registry.put(key, productClass);
}
public static Product getProduct(String key) throws Exception {
Class<? extends Product> productClass = registry.get(key);
if (productClass != null) {
return productClass.getDeclaredConstructor().newInstance();
}
throw new IllegalArgumentException("Unknown product type: " + key);
}
}
6. Not Considering Extensibility
Pitfall: If the Factory isn’t designed with extensibility in mind, it can become a roadblock as the project evolves.
Solution: Create base Factory classes or interfaces that outline the methods for creating products. This allows new factories to be created with minimal changes and encourages developers to follow the established structure when introducing new product types.
// Abstract factory interface example
interface ProductFactory {
Product createProduct();
}
// Concrete factories implementing the interface
class ConcreteProductAFactory implements ProductFactory {
@Override
public Product createProduct() {
return new ConcreteProductA();
}
}
class ConcreteProductBFactory implements ProductFactory {
@Override
public Product createProduct() {
return new ConcreteProductB();
}
}
My Closing Thoughts on the Matter
The Factory Design Pattern is a powerful tool in your software design arsenal. By understanding common pitfalls such as over-engineering, tight coupling, and lack of clear responsibilities, you can strategize to overcome these challenges. Incorporating best practices, such as dependency injection and caching, enhances not only your application performance but also its maintainability.
By creating a flexible, scalable, and modular factory system, you are setting the stage for easier object management and better adherence to design principles. For anyone diving deeper into design patterns, consider reading Refactoring to Patterns by Martin Fowler for more insights.
If you have experiences with the Factory Design Pattern, or if you have your own tips and tricks, feel free to share them in the comments below! Happy coding!
Checkout our other articles