Boost Your Java Testing: Top Pitfall in Mutation Testing
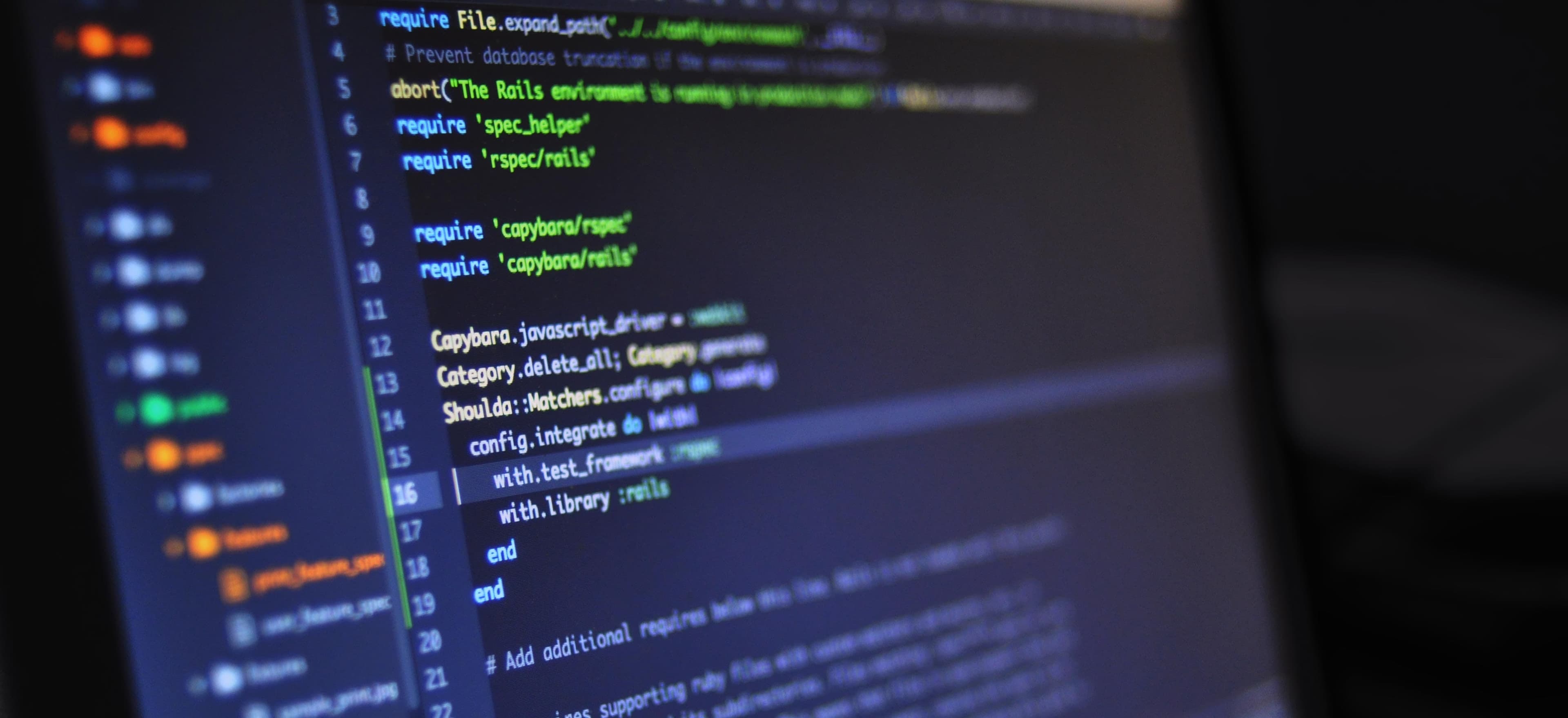
- Published on
Boost Your Java Testing: Top Pitfall in Mutation Testing
Testing is a critical aspect of software development, particularly in the Java ecosystem. While unit and integration testing remain staples, one method that is gaining traction for its efficiency is mutation testing. However, many developers fall into pitfalls during this process. This post will guide you through mutation testing, unveil its common pitfalls, and offer practical tips to enhance your Java testing practices.
Understanding Mutation Testing
Mutation testing works by making small changes (mutations) to your program's source code to check if your existing tests can catch these changes. By simulating faults, you can determine the effectiveness of your testing suite.
Why Use Mutation Testing?
- Efficiency: Enhances the effectiveness of your existing tests by ensuring they can detect changes.
- Coverage: Helps you identify untested parts of your code.
- Quality: Increases confidence in the robustness of your codebase.
Common Pitfalls in Mutation Testing
Despite its advantages, mutation testing often comes with several pitfalls that can lead developers astray. Here are the top pitfalls and how to avoid them.
1. Overlooking Test Quality
One of the biggest misconceptions is that simply having a myriad of tests guarantees quality. Just increasing the number of tests without assessing their quality may yield incorrect results in mutation testing.
Solution
Invest time in writing well-thought-out, quality tests. For instance, a high-quality test should not only check for expected outcomes but also for edge cases.
Example: Checking for Null Values
public class MyServiceTest {
@Test(expected = NullPointerException.class)
public void testNullInput() {
MyService service = new MyService();
service.processData(null); // this should throw an exception
}
}
2. Ignoring the MUTATION_THRESHOLD
The mutation threshold is a critical parameter in mutation testing tools that defines how many of the mutated test cases should pass for your tests to be considered effective.
Many developers mistakenly set the threshold too low or too high, leading to either false confidence or unnecessary alarm.
Solution
Set an appropriate MUTATION_THRESHOLD based on your specific requirements. Aim for a balance that indicates your tests are solid without being overly lenient.
3. Misinterpreting Results
Another common issue is interpreting the results incorrectly. Just passing all mutations does not mean that your code is bug-free.
Solution
Always dig deeper into mutation results. Focus on the ones that failed to provide actionable insights. Analyze the types of mutations and address them methodically.
Example: Inspecting Failed Mutations
@Test
public void checkAddition() {
assertEquals(2, MyMath.add(1, 1)); // failed mutation should point to a flaw
}
public void checkDivision() {
assertThrows(ArithmeticException.class, () -> MyMath.divide(1, 0));
}
4. Failing to Use a Testing Framework
Not using a proper testing framework can lead to complications in managing mutation tests. Some developers think they can run manual tests, only to realize it's unsustainable.
Solution
Leverage frameworks like JUnit alongside mutation testing tools to streamline your process. These frameworks provide utilities for assertions and can simplify the execution of your tests.
Example: Using JUnit with a Mutation Testing Framework
import static org.junit.jupiter.api.Assertions.*;
class MyMathTest {
@Test
void testMutations() {
MyMath math = new MyMath();
assertEquals(4, math.add(2, 2)); // Good assertion
assertEquals(0, math.add(1, -1)); // Covers edge case
}
}
5. Neglecting Environment Configurations
Many developers overlook the importance of environment configurations. If your environment is not set up correctly for mutation testing, results can be misleading.
Solution
Ensure that your testing environment mimics production as closely as possible. This includes dependencies, configuration files, and even hardware considerations.
Best Practices for Effective Mutation Testing
1. Automated Testing Workflow
Implement an automated testing workflow using CI/CD practices. This way, you can run your mutation tests consistently without manual intervention.
2. Continuous Feedback Loop
Create a feedback loop for your developers. Share mutation results and encourage discussions on how tests can be improved.
3. Leverage Mutation Testing Tools
Utilize tools like PIT (PITest) for mutation testing in Java. These provide comprehensive reports that detail which tests failed to catch mutations.
Example of Using PIT with Maven
To integrate PIT into your project with Maven, add the following dependency to your pom.xml
file:
<plugin>
<groupId>org.pitest</groupId>
<artifactId>pitest-maven</artifactId>
<version>1.7.3</version>
</plugin>
You can then run mutation tests via your terminal:
mvn test pitest:mutationCoverage
4. Prioritize High-Risk Areas
Focus on areas of the codebase that are high risk or complex. The more critical the code, the more effort should be put into ensuring it is robust.
5. Use Code Review
Encourage your team to share their tests through code review systems. This ensures multiple eyes evaluate the robustness of the tests before they are merged.
The Last Word
Mutation testing offers a powerful mechanism to enhance your Java testing capabilities. By being aware of common pitfalls and adhering to best practices, you can increase your test suite's quality and ensure higher code reliability.
Incorporate the strategies discussed in this post to empower your development process further. For more insights on testing and Java development, consider following Java Testing Best Practices and exploring additional tools available in the Java ecosystem.
By focusing on quality and robustness in your testing efforts, your software will not only meet but exceed expectations, ensuring long-term success for your Java applications.
Checkout our other articles