Why Jakarta EE's Package Name Change Could Break Your Code
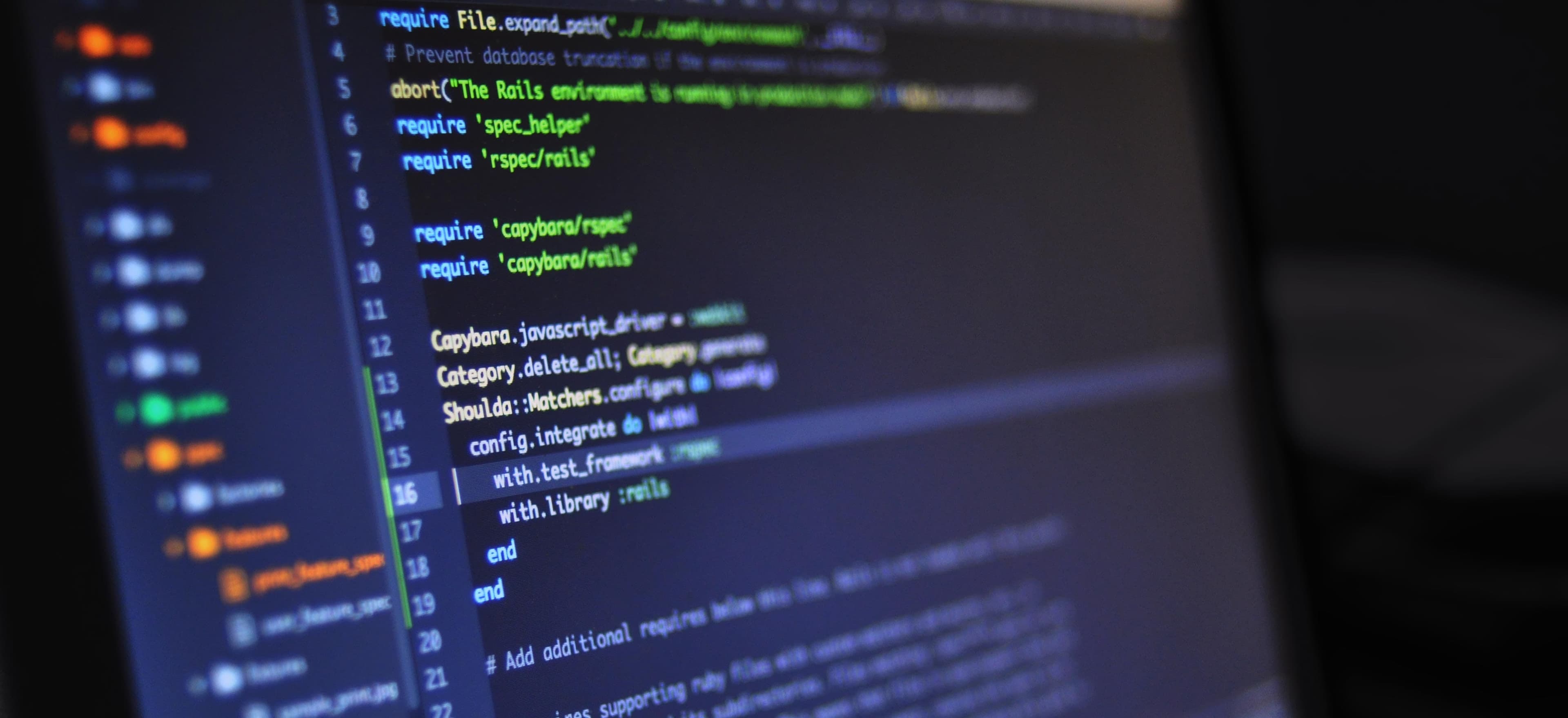
- Published on
Why Jakarta EE's Package Name Change Could Break Your Code
Jakarta EE, the evolution of Java EE, has brought with it exciting features and improvements for enterprise Java development. However, one significant change that developers need to be aware of is the alteration of package names. This shift has implications that may affect your existing codebase and project structure. In this post, we'll explore what the package name changes entail, why they matter, and how to adapt your code to this new environment.
The Shift from javax to jakarta
In 2018, Oracle transferred the stewardship of Java EE to the Eclipse Foundation, initiating a rebranding effort that would lead to Jakarta EE. One of the prominent changes within this transition is the migration from the javax
namespace to the jakarta
namespace. This means that wherever you used packages like javax.servlet
, javax.persistence
, or javax.enterprise
, you’ll now need to use jakarta.servlet
, jakarta.persistence
, and jakarta.enterprise
, respectively.
Why This Matters
-
Breaking Changes: If your existing projects or libraries rely on the
javax
packages, they may break with the Jakarta EE transition. Many dependencies, frameworks, and tools in your development stack may not yet support thejakarta
namespace. -
Future Compatibility: To leverage new features, improvements, and security updates in Jakarta EE, you’ll need to refactor your code. This is not just about aesthetic changes but adapting to a new, evolving ecosystem.
-
Time and Costs: Changing package names across a large codebase can be time-consuming. It requires testing to ensure that the functionality remains intact after the refactor. If multiple developers are working on the same codebase, coordinating these changes can lead to potential conflicts and delays.
Example: Migrating from javax to jakarta
Before Migration
Suppose you have a simple JAX-RS application that uses the javax.ws.rs
package for building a RESTful web service.
package com.example;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/hello")
public class HelloWorld {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String hello() {
return "Hello, Jakarta EE!";
}
}
After Migration
Once you decide to migrate to Jakarta EE, you need to modify the package imports as follows:
package com.example;
import jakarta.ws.rs.GET;
import jakarta.ws.rs.Path;
import jakarta.ws.rs.Produces;
import jakarta.ws.rs.core.MediaType;
@Path("/hello")
public class HelloWorld {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String hello() {
return "Hello, Jakarta EE!";
}
}
Key Takeaway
The actual code is quite similar, but the important part is recognizing that the package imports have changed. The code change is straightforward; however, the implications are significant because they extend beyond a mere substitution.
Resolving Dependencies
Maven Configuration
If you're using Maven, you'll need to update the dependencies as follows:
<dependencies>
<dependency>
<groupId>jakarta.ws.rs</groupId>
<artifactId>jakarta.ws.rs-api</artifactId>
<version>2.1.6</version>
</dependency>
</dependencies>
Gradle Configuration
For Gradle, the update would look something like this:
dependencies {
implementation 'jakarta.ws.rs:jakarta.ws.rs-api:2.1.6'
}
Importance of Dependency Management
Dependency management is critical to ensure that your project is using compatible versions of libraries. As changes to package names occur, older versions of your libraries that still reference javax
may need to be replaced or updated to compatible jakarta
versions.
Testing After Migration
After making changes to your code and dependencies, it’s crucial to perform thorough testing. Unit tests, integration tests, and regression tests should all be executed to ensure that everything operates as expected.
Example Unit Test
Here’s an example of how you might adjust a unit test for the new package structure:
package com.example;
import jakarta.ws.rs.client.Client;
import jakarta.ws.rs.client.ClientBuilder;
import jakarta.ws.rs.core.MediaType;
import jakarta.ws.rs.core.Response;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class HelloWorldTest {
@Test
public void testHello() {
Client client = ClientBuilder.newClient();
Response response = client.target("http://localhost:8080/hello").request(MediaType.TEXT_PLAIN).get();
assertEquals("Hello, Jakarta EE!", response.readEntity(String.class));
}
}
Preparing for Greater Changes Ahead
Future-Proof Your Code
With this transition also comes the potential for more changes in the future. The Jakarta EE community is continually evolving, which means keeping your libraries and frameworks up-to-date will be crucial. Subscribing to updates from the Eclipse Foundation and participating in community discussions can help you stay ahead of the curve.
Continued Learning
Educate yourself on the new features offered in Jakarta EE. Resources like the official Jakarta EE documentation and community-driven forums such as StackOverflow can greatly assist in this learning process.
Bringing It All Together
The move from the javax
package to the jakarta
namespace in Jakarta EE is more than just syntax updates; it’s about adapting to an evolving ecosystem. Although it can seem daunting, taking systematic steps to update your code, manage dependencies, and test thoroughly will facilitate a smoother transition.
By understanding why these package changes matter and preparing your code accordingly, you will ensure compatibility with future releases of Jakarta EE while taking full advantage of the new features it has to offer.
If your code or dependency landscape still leans on outdated libraries, now is the perfect time to bring them up to speed. Embrace this challenge—after all, adaptability is a core tenet of successful software engineering.
Stay informed, keep coding, and welcome the new era of Jakarta EE!
Checkout our other articles