Mastering Regex in Java for Effective HTML Tag Conversion
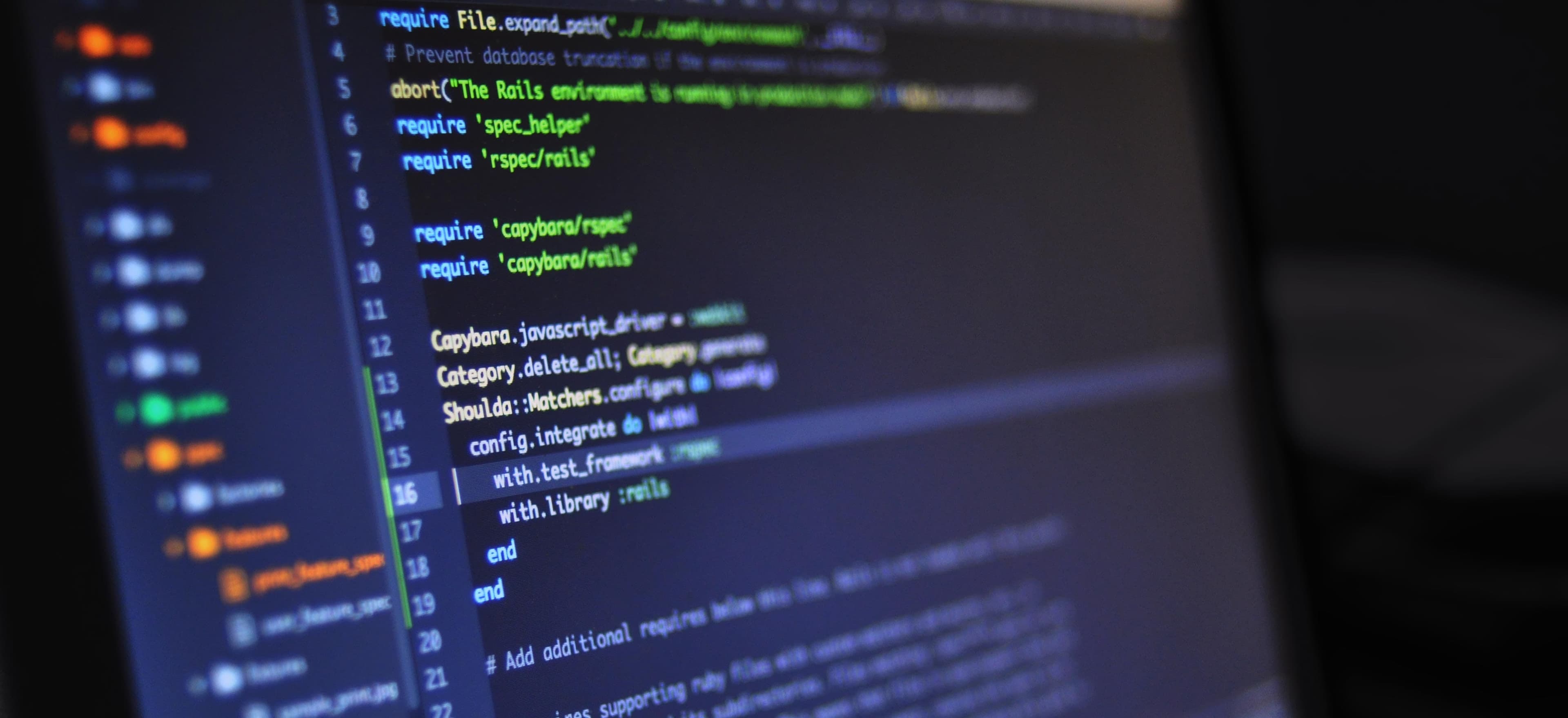
- Published on
Mastering Regex in Java for Effective HTML Tag Conversion
In the realm of web development, the ability to manipulate HTML tags efficiently is vital. Whether you're scraping data, transforming documents, or cleaning up malformed HTML, having a solid grasp of regular expressions (regex) in Java is essential. This tutorial will take you through the nuances of regex for converting HTML tags, culminating in practical examples that you can apply to your projects.
Understanding Regex Basics
Regex is a powerful tool for string manipulation. It allows developers to define search patterns and perform complex text searches and replacements. Before delving into tag conversion, let’s clarify some common regex symbols:
- . - Matches any character except a line break.
- \w - Matches any word character (alphanumeric & underscore).
- \d - Matches any digit.
- \s - Matches any whitespace character.
- *** / + / ?** - These are quantifiers that match 0 or more, 1 or more, or 0 or 1 occurrences, respectively.
- ( ) - Group your patterns.
- [ ] - Matches any character within the brackets.
With this understanding, you can start utilizing regex in Java.
Setting Up Your Java Environment
Before you begin coding, ensure that you have the Java Development Kit (JDK) installed on your machine. If you haven't installed it yet, follow the official Oracle documentation to get started.
For this tutorial, you can use any Integrated Development Environment (IDE) like IntelliJ IDEA, Eclipse, or even a simple text editor.
Regex in Java: The Basics
To use regex in Java, you typically rely on the Pattern
and Matcher
classes found in the java.util.regex
package. Here's a quick example demonstrating how to match a simple pattern:
import java.util.regex.*;
public class RegexExample {
public static void main(String[] args) {
String input = "Sample123";
String regex = "\\w+"; // Matches one or more word characters
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(input);
if (matcher.find()) {
System.out.println("Match found: " + matcher.group());
} else {
System.out.println("No match found.");
}
}
}
Why This Matters: The above code demonstrates the foundational skills needed to work with regex in Java. It illustrates how to compile a regex pattern and determine if the string contains any match.
Converting HTML Tags with Java Regex
With the basics in mind, the focus now shifts toward converting HTML tags. While regex may not be the ideal solution for parsing HTML due to its complexity, it can still be effective for specific and simpler cases.
1. Simple Tag Conversion
Let's take a look at how to convert specific HTML tags into equivalent Markdown syntax. For example, if you want to replace <strong>
tags with **
(bold in Markdown), you can achieve it as follows:
import java.util.regex.*;
public class HtmlToMarkdown {
public static void main(String[] args) {
String html = "<strong>This is bold text</strong>";
String markdown = convertStrongToMarkdown(html);
System.out.println(markdown);
}
public static String convertStrongToMarkdown(String input) {
String regex = "<strong>(.*?)</strong>";
String replacement = "**$1**";
return input.replaceAll(regex, replacement);
}
}
Why This Matters: This example highlights the application of capture groups (the .*?
pattern) for extracting text between tags and substituting it with the desired format. Here, $1
refers to the content captured by the first parentheses in the regex.
2. Converting Multiple Tags
Let's enhance our approach to handle multiple tags. We might want to convert <em>
tags to _
, <h1>
to #
, <h2>
to ##
, and so forth. Here’s how we can achieve that:
import java.util.regex.*;
public class MultiTagConverter {
public static void main(String[] args) {
String html = "<h1>Title</h1><strong>Bold Text</strong><em>Italic Text</em>";
String markdown = convertHtmlToMarkdown(html);
System.out.println(markdown);
}
public static String convertHtmlToMarkdown(String input) {
String[] tags = {
"<h1>(.*?)</h1>",
"<strong>(.*?)</strong>",
"<em>(.*?)</em>"
};
String[] replacements = {
"# $1\n",
"**$1**",
"_$1_"
};
for (int i = 0; i < tags.length; i++) {
input = input.replaceAll(tags[i], replacements[i]);
}
return input;
}
}
Why This Matters: The loop iterates over multiple HTML tags and applies the corresponding replacements. This scalability is crucial for real-world applications, where HTML documents can be complex.
Common Pitfalls in HTML Regex
When dealing with regex for HTML, there are several pitfalls to keep in mind:
- Nested Tags: Regular expressions struggle to correctly deal with nested HTML tags, as they lack the "memory" to keep track of levels.
- Malformed HTML: HTML is forgiving; regex is not. Attempting to parse malformed HTML with regex can lead to unforeseen errors.
- Special Characters: HTML entities like
&
,<
, etc., can cause mismatches.
For a more comprehensive discussion on fixing regex for HTML tag conversions, consider checking out the article "Fixing Regex for Converting HTML Tags Efficiently".
Bringing It All Together
Mastering regex in Java for effective HTML tag conversion is an attainable skill, yet it demands careful consideration. Understanding regex patterns and their application within Java is crucial. As demonstrated, you can perform straightforward conversions, but be cautious of the limitations and potential pitfalls.
Regex opens up a world of possibilities. With practice, you can leverage this powerful tool to streamline your web development workflow and enhance your data manipulation capabilities.
Explore Java's regex capabilities further and try to incorporate these examples into your projects. Happy coding!
Resources:
By continuously building on your understanding, you’ll find that regex can be an invaluable asset in any Java developer’s toolkit.
Checkout our other articles