Mastering Java: Efficiently Handling HTML with Regex
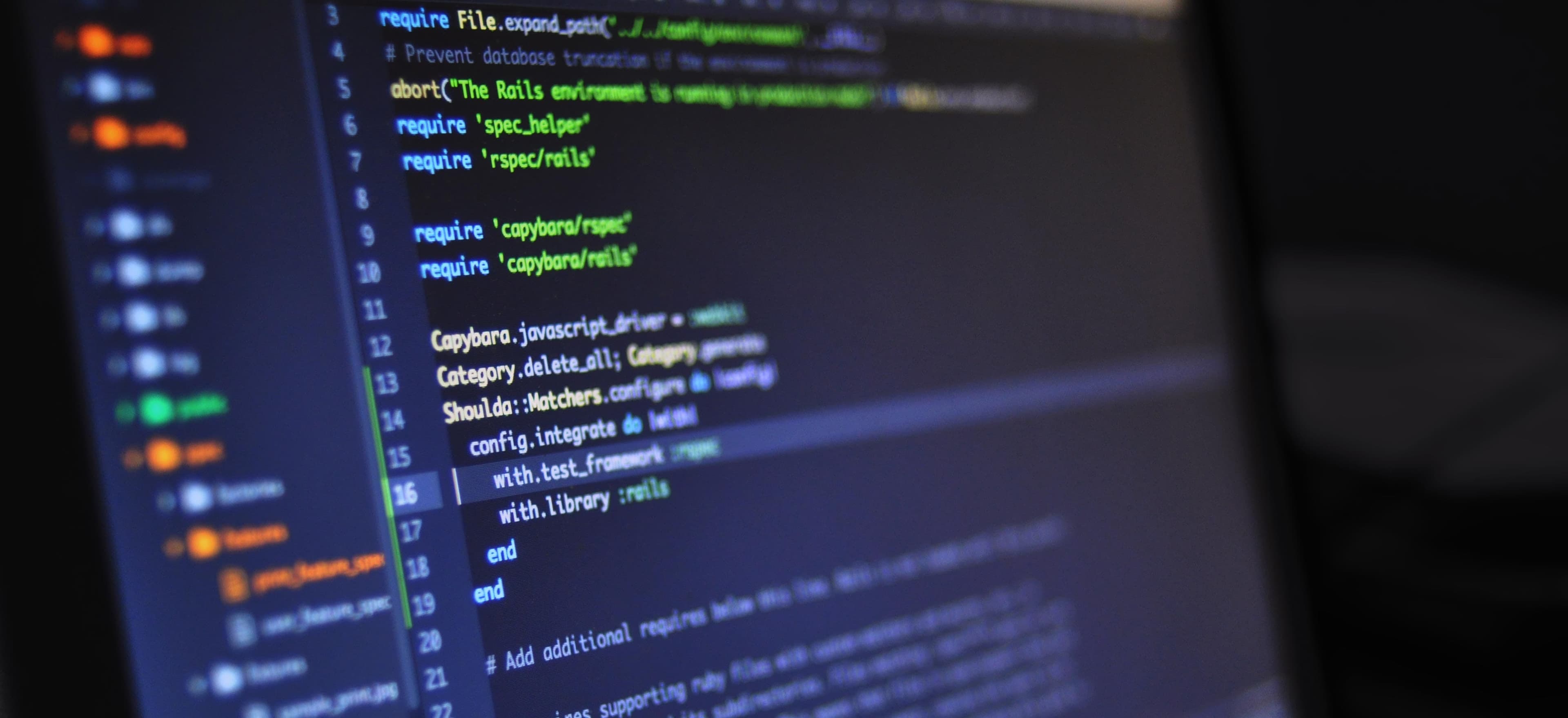
- Published on
Mastering Java: Efficiently Handling HTML with Regex
In the realm of software development, particularly when dealing with web applications, you may find yourself needing to manipulate HTML content. One approach many developers resort to is using Regular Expressions (Regex). Although Regex can be a powerful tool, encountering pitfalls is common, especially when parsing HTML. This blog post delves into the nuances of employing Regex in Java for HTML tag conversion. We will discuss key concepts, potential challenges, and provide practical examples to streamline your application’s performance.
Understanding the Basics of Regex in Java
Regular Expressions are patterns that specify sets of strings. In Java, you can utilize the java.util.regex
package for working with Regex patterns. Here’s a simple breakdown of its components:
- Pattern: A compiled representation of a Regex.
- Matcher: An engine that performs matching operations on a character sequence.
- Flags: Options that change how the regex engine interprets the pattern.
Why Use Regex?
- Performance: Regex can outperform other methods for simple search-and-replace tasks due to its optimized engine.
- Conciseness: Regex allows you to express complex string operations succinctly.
Common Mistakes When Using Regex with HTML
When manipulating HTML with Regex, developers often end up with flawed solutions. HTML is a complex language, and regex is ill-equipped to understand its hierarchical nature. For instance:
Misinterpreting Nested Tags
Consider the following regex attempting to match a <div>
tag:
String regex = "<div>(.*?)</div>";
What happens if there's a <div>
inside another <div>
? This pattern will fail to capture nested structures accurately.
Ignoring Edge Cases
HTML can be very unpredictable. For instance, an unclosed tag or improperly nested tags could lead to unexpected behavior. It’s vital to consider various scenarios when crafting your expressions.
A Better Approach
To enhance your understanding, let’s look at an improved regex pattern that factors in multiple attributes that a <div>
tag might have.
String regex = "<div(.*?)>(.*?)</div>";
Explanation
- (.*?) captures any attributes present within the opening tag without including the closing angle bracket.
- The second (.*?) captures all content inside the
<div>
tags.
Example: Converting HTML Tags to Custom Format
Let’s implement a simple Java program that converts HTML <strong>
tags to markdown **strong**
format. The process involves:
- Defining the regex to identify the
<strong>
and</strong>
tags. - Using a Matcher to perform the replacement.
Here is how you can implement this:
import java.util.regex.*;
public class HtmlToMarkdown {
public static void main(String[] args) {
String htmlInput = "hello <strong>world</strong>!";
String markdownOutput = convertHtmlToMarkdown(htmlInput);
System.out.println(markdownOutput);
}
public static String convertHtmlToMarkdown(String html) {
String regex = "<strong>(.*?)</strong>";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(html);
return matcher.replaceAll("**$1**");
}
}
Commentary on the Code
- Pattern.compile(regex): Compiles the regex for search operations.
- matcher.replaceAll("$1**")**: This method replaces all occurrences of the pattern with our desired markdown format, where
$1
represents the first captured group.
Pitfalls to Avoid
-
Over-reliance on Regex: Always remember that regex isn't a one-size-fits-all solution for parsing HTML. Consider libraries like Jsoup for more complex manipulations.
-
Failure to Escape Special Characters: If your HTML includes characters that have special significance in regex (like
.
or*
), be sure to escape them. -
Failure to Test Edge Cases: Always make sure to test your regex with a variety of HTML samples to confirm it behaves as expected.
Links to Reference
Using regex to manipulate HTML can be informative and is sometimes necessary. However, we recommend exploring alternatives such as the article titled Fixing Regex for Converting HTML Tags Efficiently for insights on the limitations and nuances connected with regex and HTML parsing.
Wrapping Up
Handling HTML with Regex in Java can be both powerful and tricky. While it is beneficial for simple string transformations, bear in mind that HTML's complexity can lead to serious pitfalls if not handled properly.
For scenarios demanding more sophistication in parsing and converting HTML, consider leveraging a well-documented library like Jsoup. This will enhance reliability and maintainability compared to using regex, which, while appealing for its brevity, lacks the depth needed for comprehensive HTML manipulation.
By mastering Regex and understanding its limitations, you can proficiently decide when to use it and when to reach for a more robust solution. Perhaps the most important takeaway is to maintain caution and rigor in your approach, ensuring that edge cases are accounted for, preventing unforeseen issues in production environments.
Checkout our other articles