Mastering Icon Placement in JavaFX Headers Without Margins
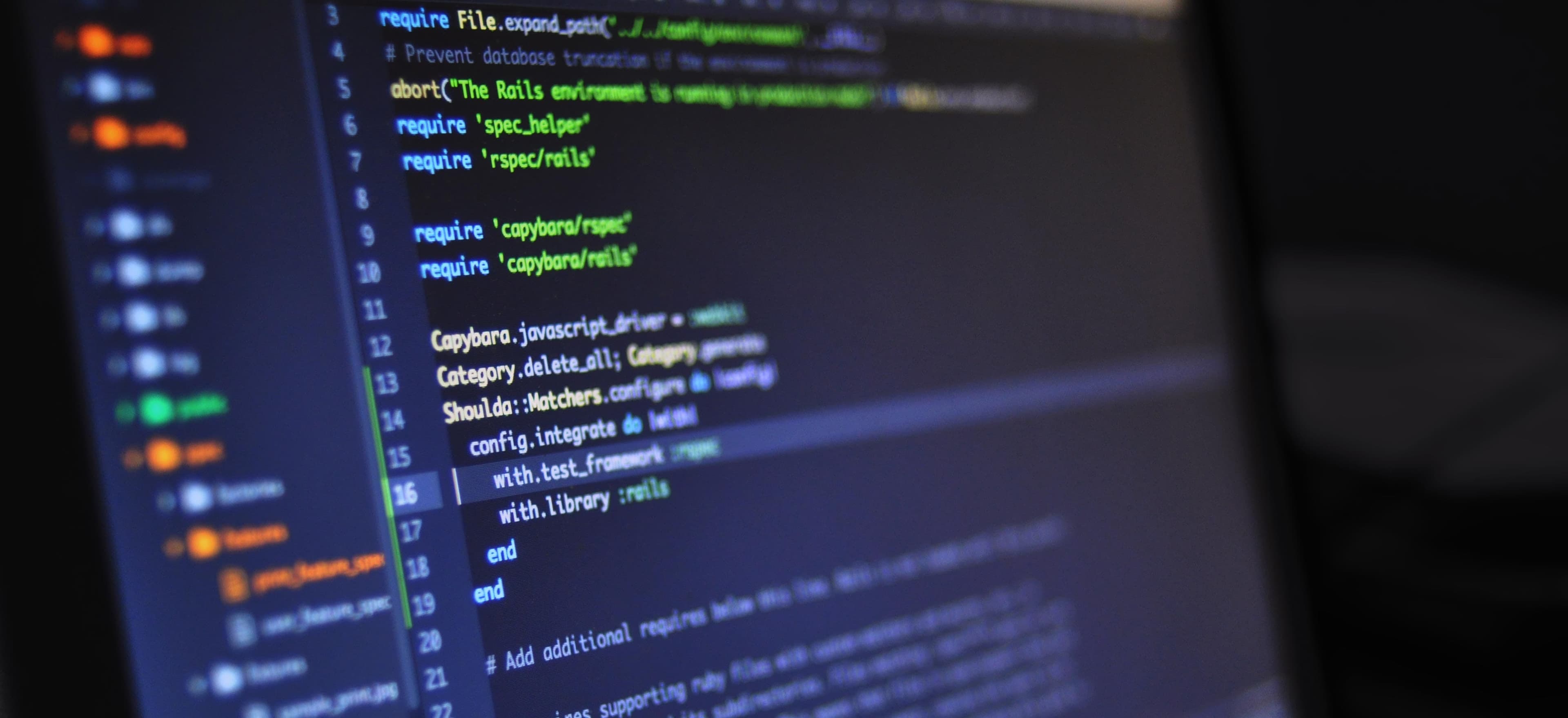
- Published on
Mastering Icon Placement in JavaFX Headers Without Margins
When it comes to designing user interfaces in Java using JavaFX, one key aspect that developers often grapple with is the placement of icons, particularly in headers. Correctly positioning icons enhances the visual appeal and usability of the application. This blog post will guide you through effective icon placement strategies without relying on negative margins. For further reading on this subject, feel free to check out Effortless Icon Placement in Headers: No Negative Margins!.
Understanding JavaFX Layouts
JavaFX provides a rich set of layout managers that facilitate different screen arrangements, making it easier to achieve your desired design without manually calculating positions. The main layout containers include VBox, HBox, GridPane, BorderPane, and more. Here’s a brief look at each:
- VBox: Vertically stacks its children.
- HBox: Horizontally aligns its children.
- GridPane: Lays out its children in a grid.
- BorderPane: Splits the scene into five regions: top, bottom, left, right, and center.
Why Layouts Matter
Using layouts properly ensures that your UI is responsive and adaptive to various screen sizes. It minimizes the need for negative margins, which can lead to undesirable side effects such as overlapping elements or an awkward design. Let’s dive into how you can precisely position icons in header sections without using negative margins.
Anchoring Icons within HBox
The HBox layout is significantly beneficial for creating headers since it stacks the components horizontally. Below is an example of how to correctly place icons in a header using HBox:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.HBox;
import javafx.stage.Stage;
public class HeaderIconExample extends Application {
@Override
public void start(Stage primaryStage) {
HBox header = new HBox(10); // Spacing between elements in the HBox
// Create an icon
Image icon = new Image("icon.png"); // Make sure to replace with your path
ImageView iconView = new ImageView(icon);
iconView.setFitWidth(20);
iconView.setFitHeight(20);
// Create a button
Button button = new Button("Home");
button.setGraphic(iconView); // Set the graphic of the button to the icon
header.getChildren().add(button); // Add button to HBox
Scene scene = new Scene(header, 300, 200);
primaryStage.setScene(scene);
primaryStage.setTitle("Header Icon Placement");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Code Explanation
-
Creating HBox: The HBox constructor is called with a spacing parameter (10 pixels), ensuring proper gaps between elements; this is essential for clean designs.
-
ImageView: The ImageView is created to display the icon.
setFitWidth()
andsetFitHeight()
are used to specify the size, allowing consistent icon dimensions. -
Button and Icon: The icon is set as the graphic for the button, positioning it neatly within the button.
Why Use HBox
Using HBox allows you to easily align and space icons horizontally without overcrowding. This is especially useful for headers where neatness and functionality are critical.
Advanced Icon Placement: Utilizing CSS for Styling
JavaFX comes with a powerful CSS engine that can significantly enhance the design aspect of your application, including icon placements. Here’s an example that showcases how to utilize CSS for styling your header icons:
CSS for Better Styling
styles.css:
.header {
-fx-background-color: #2e2e2e; /* Dark background for header */
-fx-padding: 10; /* Padding inside the header */
}
.button {
-fx-text-fill: white; /* Set text color to white */
}
Updated Java Code
Here’s how you integrate this CSS into your JavaFX application:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.HBox;
import javafx.stage.Stage;
public class HeaderIconExampleWithCSS extends Application {
@Override
public void start(Stage primaryStage) {
HBox header = new HBox(10);
header.getStyleClass().add("header");
Image icon = new Image("icon.png");
ImageView iconView = new ImageView(icon);
iconView.setFitWidth(20);
iconView.setFitHeight(20);
Button button = new Button("Home");
button.setGraphic(iconView);
button.getStyleClass().add("button");
header.getChildren().add(button);
Scene scene = new Scene(header, 300, 200);
scene.getStylesheets().add("styles.css"); // Loading the CSS file
primaryStage.setScene(scene);
primaryStage.setTitle("Header Icon Placement with CSS");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why Use CSS in JavaFX
-
Modularity: It keeps your Java code clean, separating styling from logic.
-
Reusability: The same style rules can be applied to multiple elements without rewriting code, making it easier to maintain.
Managing Icon Placement Dynamically
In a real-world application, you may need to handle resizing dynamically. JavaFX layouts automatically adjust based on the scene size. However, sometimes manual adjustments might be required. For instance, using setAlignment()
can control the alignment of the icons directly:
header.setAlignment(Pos.CENTER_LEFT); // Aligns the elements to the left
Advantages of Dynamic Adjustment
- Responsiveness: The application can respond to various screen sizes effectively, keeping the UI intact.
- Professional Appearance: Implementing a well-structured layout can provide a polished look to your application.
In Conclusion, Here is What Matters: Creating Visually Appealing Headers
Utilizing JavaFX effectively can ease the burdens of UI design, especially when it comes to icon placement in headers. By leveraging the right layout managers like HBox, utilizing CSS for styling, and ensuring dynamic adjustments, you can create visually appealing headers without using negative margins.
For those looking for further techniques in icon placement and UI formatting, I encourage you to read Effortless Icon Placement in Headers: No Negative Margins!. By integrating these practices, you not only enhance usability but also ensure that your application retains a professional look. Happy coding!