Mastering Java Project Structure to Avoid Path Issues
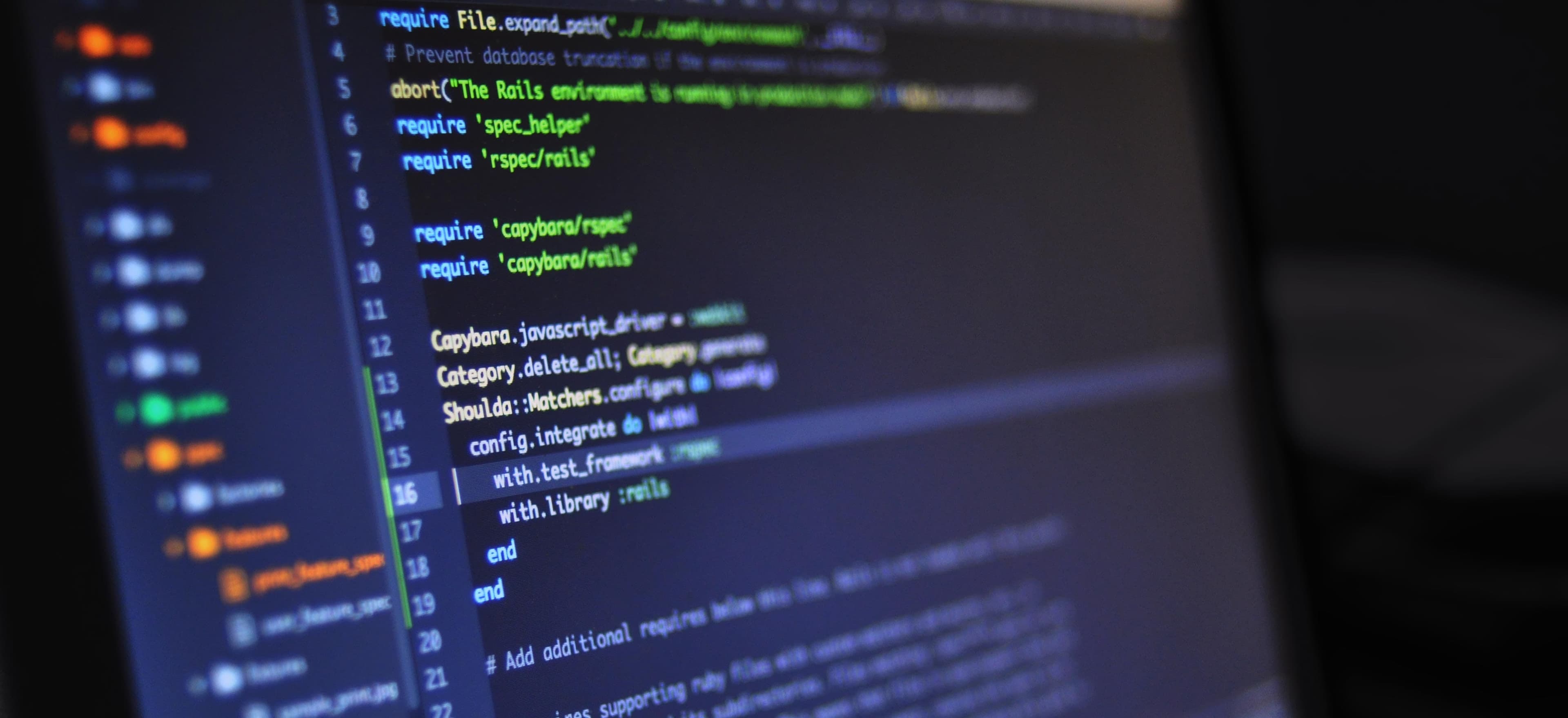
- Published on
Mastering Java Project Structure to Avoid Path Issues
In the world of software development, the structure of your project plays an important role in maintainability and scalability. This post will delve into how you can master your Java project structure to avoid path issues, drawing on key practices and strategies. Along the way, we’ll focus on the importance of a clean directory layout, proper dependency management, and the use of path aliases, which can significantly alleviate common path-related headaches in your projects.
Why Project Structure Matters
Proper project structure not only helps you avoid “path hell,” but it also serves as a blueprint for collaboration and development. Here are a few key reasons why project structure is important:
- Improved Readability: A well-organized structure makes it easier for developers to navigate the project.
- Easier Collaboration: Teams can understand project hierarchy quickly, making it simpler for new developers to jump in.
- Maintenance: A clean and consistent structure simplifies the process of updating and maintaining the code.
Basic Java Project Structure
Java projects typically follow a standard directory structure. Below is a commonly used format:
my-java-project
│
├── src
│ ├── main
│ │ └── java
│ │ └── com
│ │ └── mycompany
│ │ └── myproject
│ │ ├── Main.java
│ │ └── utils
│ │ └── Helper.java
│ └── test
│ └── java
│ └── com
│ └── mycompany
│ └── myproject
│ └── MainTest.java
│
├── lib
├── resources
└── README.md
Explanation of Key Directories
- src: This is where your source code will reside. The standard practice is to separate your production code (
main
) and test code (test
). - lib: This directory traditionally holds libraries your project depends on.
- resources: Use this for non-code assets such as configuration files or images.
- README.md: A markdown file where you can explain what the project is, installation steps, and usage details.
Creating a Clear Namespace
The structure shown above facilitates clear namespaces. The subdirectory com/mycompany/myproject
echoes your main package declaration package com.mycompany.myproject;
. This correspondence satisfies Java's package naming conventions and helps Java understand how to locate your classes.
Code Example:
package com.mycompany.myproject;
public class Main {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Commentary:
In the above code, the package
declaration aligns with our directory structure. This set-up ensures that Java can access the Main
class without confusion, preventing path issues.
Dependency Management with Maven
Keeping your dependencies in check is crucial. One way to handle dependencies is through Apache Maven, which manages project complexity efficiently. Maven uses a pom.xml
file to define the project's dependencies and properties.
Example of a pom.xml:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.mycompany</groupId>
<artifactId>myproject</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
Commentary:
In the dependency section, we're adding JUnit for testing our application. Maven handles downloading these dependencies, making sure that your development environment is consistent. This eliminates the headache of managing path issues related to libraries yourself.
Utilizing Path Aliases
Just like the challenges faced in Java, path issues are also a common hurdle in JavaScript projects, particularly in React Native apps. The article titled "Solving Relative Path Hell: React Native Path Aliases" explores how React Native developers deal with intricate paths.
For Java developers, defining aliases in your build tool (like Maven or Gradle) can streamline development. Although Java does not directly support aliases within classpaths, organizing packages logically can serve the same purpose. Instead, consider importing specific classes instead of entire packages.
Example:
import com.mycompany.myproject.utils.Helper;
public class Main {
public static void main(String[] args) {
String message = Helper.getWelcomeMessage();
System.out.println(message);
}
}
Here, we import only the Helper
class, ensuring clarity. Users can easily locate where getWelcomeMessage
is defined.
Best Practices for Avoiding Path Issues
Here are some actionable best practices to take away:
-
Standard Directory Structure: Stick to the standard Java project layout (src/main/java, etc.) to benefit from convention over configuration.
-
Modular Code: Break your code into modules that have concerned packages, making them easier to locate, modify, and understand.
-
Version Control: Use Git or similar tools to keep track of your code. Properly managing versions can avoid confusion.
-
Document Your Structure: Use README files or architecture decision records to guide newcomers in navigating the codebase.
-
Consistent Naming Conventions: Use consistent naming for your packages and classes, ensuring they reflect their contents.
-
Tool Integration: Leverage build tools like Maven and Gradle for effective dependency management and project configuration.
A Final Look
Mastering your Java project structure is an essential skill that pays dividends in the long run. By adhering to clear guidelines and best practices, you avoid path issues and create a codebase that is easy to maintain and extend.
By embracing strategies like dependency management with Maven and thoughtfully organizing your source code, you set yourselves up for success. Don't forget the value of documentation and consistent naming; these practices are the glue that holds everything together.
For further insights into managing paths in other programming environments, check out the article "Solving Relative Path Hell: React Native Path Aliases" here.
By following the principles laid out in this post, you'll enhance your Java development experience and create software that is not only functional but also elegant and maintainable. Happy coding!
Checkout our other articles