Mastering Object Aggregation in Java: Key Mistakes to Dodge
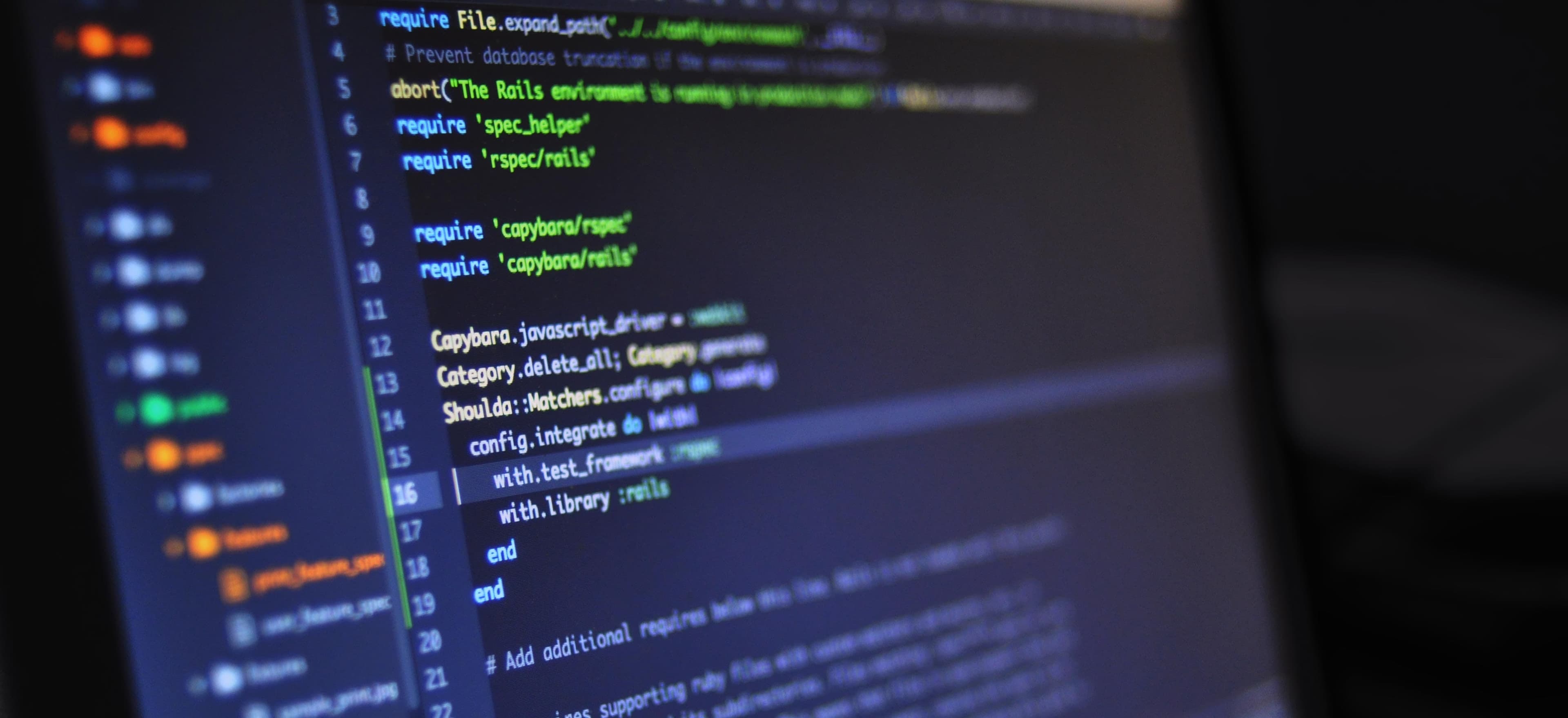
- Published on
Mastering Object Aggregation in Java: Key Mistakes to Dodge
As developers, we often find ourselves grappling with the intricate world of object-oriented programming. One of the foundational concepts we must master is object aggregation. This design principle allows us to create complex data structures where one object contains or references other objects. However, despite its usefulness, improper implementation can lead to numerous pitfalls. Understanding these pitfalls ensures more efficient code and more manageable systems.
In this blog post, we will explore what object aggregation is, illustrate its principles with clear Java examples, and highlight common mistakes to avoid. For deeper insights, we recommend checking out the article "Understanding Object Aggregation: Common Pitfalls to Avoid".
What is Object Aggregation?
Object aggregation is a relationship between two objects where one object (the container) is said to "aggregate" other objects. It establishes a "has-a" relationship. For instance, consider a Library
class that has a collection of Book
objects.
Understanding the Structure
In aggregation, the aggregated objects can exist independently of the parent object. If the parent object is destroyed, the child objects continue to exist. Here's a simplified example:
import java.util.*;
class Book {
private String title;
public Book(String title) {
this.title = title;
}
public String getTitle() {
return title;
}
}
class Library {
private List<Book> books;
public Library() {
books = new ArrayList<>();
}
public void addBook(Book book) {
books.add(book);
}
public void displayBooks() {
for (Book book : books) {
System.out.println(book.getTitle());
}
}
}
Code Commentary
- In this example, the
Library
class aggregatesBook
objects. TheBook
class is independent of theLibrary
. - The
addBook
method allows for dynamic adding of books to the library's collection.
This setup illustrates the importance of object aggregation in creating structured programs.
Common Pitfalls in Object Aggregation
Despite its advantages, developers frequently encounter issues with object aggregation. Below are some common mistakes and how to avoid them.
1. Overusing Aggregation
One common mistake is the overuse of aggregation. Not all relationships should employ aggregation. For example, if an object truly owns another, composition might be a more suitable option.
Consider the Following Example
class Car {
private Engine engine;
public Car() {
engine = new Engine();
}
}
In this instance, a Car
has an Engine
, which should be composed. If the Car
is destroyed, its engine also ceases to exist.
2. Not Implementing Proper Lifecycle Management
When aggregating objects, it's crucial to manage their lifecycle properly. Failing to do so can result in memory leaks or invalid references.
Lifecycle Management Example
class Library {
private List<Book> books;
public Library() {
books = new ArrayList<>();
}
// Correctly removing a book
public void removeBook(Book book) {
books.remove(book);
}
// Incorrect: Potential for memory leak if not handled
public void clear() {
books.clear();
}
}
Commentary: In the above example, clearing a collection should be done with an understanding of the state of the books. Ensure you only clear or remove objects you no longer need. Otherwise, it may lead to unintentional loss of references.
3. Failing to Use Interfaces Properly
Another common oversight is the underutilization or improper use of interfaces. Interfaces can aid in creating more flexible and maintainable code.
Utilizing Interfaces
interface IBook {
String getTitle();
}
class Book implements IBook {
private String title;
public Book(String title) {
this.title = title;
}
public String getTitle() {
return title;
}
}
class Library {
private List<IBook> books;
public Library() {
books = new ArrayList<>();
}
}
Commentary: By programming against interfaces, the Library
class becomes more adaptable to changes. If you need to implement a different type of Book
, it can easily implement IBook
without altering the Library
class.
4. Inappropriate Visibility Modifiers
Another mistake frequent among developers is not paying attention to visibility modifiers. It is essential to use the proper access modifiers to expose only what is necessary.
Visibility Wish Example
class Library {
private List<Book> books;
public List<Book> getBooks() {
return books; // Dangerous: Directly exposing internal state
}
}
In this example, exposing the list of books directly can lead to unauthorized modifications from outside the class. Instead, return an unmodifiable view of the list:
import Collections.unmodifiableList;
// ...
public List<Book> getBooks() {
return Collections.unmodifiableList(books);
}
5. Ignoring Equals and HashCode
When aggregating objects, especially in collections, failing to implement equals()
and hashCode()
can lead to inconsistent behavior.
Implementing Equals and HashCode
class Book {
private String title;
public Book(String title) {
this.title = title;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof Book)) return false;
Book book = (Book) o;
return title.equals(book.title);
}
@Override
public int hashCode() {
return Objects.hash(title);
}
}
Commentary: By overriding equals()
and hashCode()
, we enable proper usage of books in collections such as HashMap
or HashSet
.
In Conclusion, Here is What Matters
Mastering object aggregation in Java comes with its challenges. By avoiding common pitfalls like overuse of aggregation, failing to manage lifecycles properly, neglecting interfaces, misusing visibility modifiers, and disregarding equals()
and hashCode()
, you can significantly improve the quality of your code. Object aggregation, when utilized correctly, supports effective abstraction and promotes clean code practices, enhancing your software's maintainability.
For further insights into the potential pitfalls of object aggregation, we recommend reading the article "Understanding Object Aggregation: Common Pitfalls to Avoid".
With these principles in hand, you are well on your way to becoming a more proficient Java developer. Happy coding!