Bridging Java with Node.js: Key Packages for Hybrid Apps
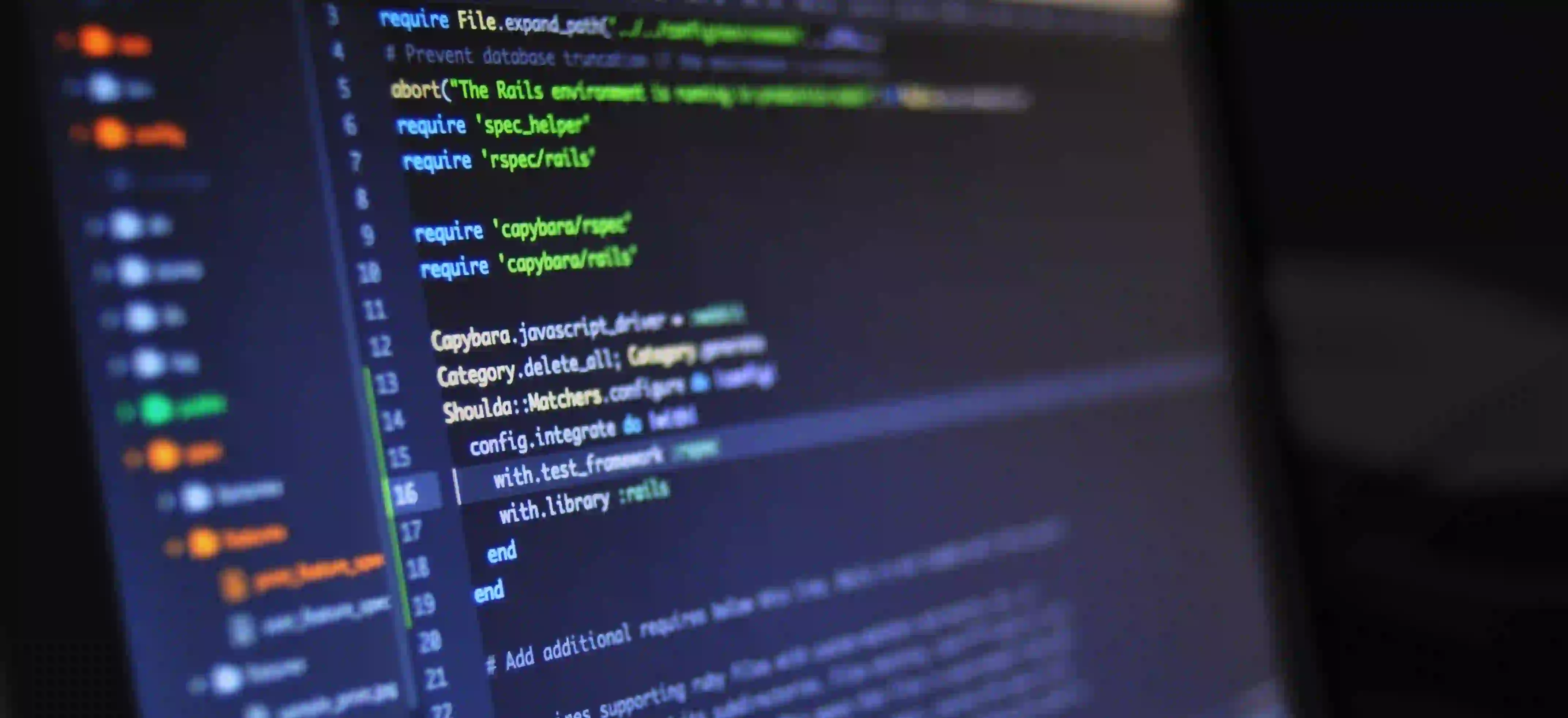
Bridging Java with Node.js: Key Packages for Hybrid Apps
In today’s fast-paced tech landscape, Java and Node.js are two of the most prominent programming environments. Though they cater to different needs – Java is often appreciated for its robustness and scalability, while Node.js shines with its non-blocking, asynchronous capabilities – the integration of both technologies can create hybrid applications that maximize the strengths of both. In this article, we’ll explore key concepts and packages that can help you bridge the gap between Java and Node.js for your next project.
Why Consider a Hybrid Approach?
As the digital landscape evolves, applications must become more responsive and capable of handling large volumes of transactions in real-time. A hybrid app leveraging Java’s backend strengths with Node.js’s high-speed performance offers several advantages:
- Performance: Node.js excels in handling concurrent requests, making it ideal for I/O heavy tasks.
- Scalability: Distributed architectures can benefit tremendously from the use of both Java and Node.js.
- Development Speed: Using the right packages to integrate two environments can speed up the overall development process.
To make hybrid development feasible, it’s crucial to identify the right libraries and frameworks.
Key Concepts in Bridging Java and Node.js
Before we delve into the packages, let’s highlight a few concepts essential for integration:
Inter-Process Communication (IPC)
IPC allows different processes to communicate with each other. In hybrid apps, this becomes vital when letting Java services communicate with Node.js services.
RESTful APIs
Creating REST APIs in Java with frameworks like Spring Boot enables you to expose Java functionality to Node.js. Conversely, Node.js can act as a lightweight consumer of Java services.
Message Queuing
Message queues like RabbitMQ or Kafka offer asynchronous communication, allowing Java applications and Node.js applications to operate independently yet remain synchronized.
Key Java Packages for Node.js Integration
1. Spring Boot
What is Spring Boot?
Spring Boot is an extension of the Spring framework that drastically simplifies the setup of new applications. It allows you to create stand-alone applications with minimal configuration.
Why Use Spring Boot?
- Ability to build REST APIs with ease.
- Strong support for microservices architecture.
- Seamless integration with various databases.
Example Code: REST API with Spring Boot
@RestController
@RequestMapping("/api")
public class UserController {
@GetMapping("/users/{id}")
public ResponseEntity<User> getUserById(@PathVariable long id) {
User user = userService.findById(id);
return ResponseEntity.ok(user);
}
}
This REST API exposes user data, which can be consumed by Node.js applications easily.
2. JHipster
What is JHipster?
JHipster is a development platform to generate, develop, and deploy Spring Boot + Angular/React/Vue web applications and Spring microservices.
Why Use JHipster?
- Code generation reduces time and effort in boilerplate code.
- Built-in support for creating microservices.
- Can easily integrate with Node.js via API endpoints.
Example Code: JHipster Generation
To generate a JHipster application, use the following command:
jhipster
Follow the prompts to set up a basic application with REST endpoints for integration with Node.js.
Key Node.js Packages for Java Integration
1. Axios
What is Axios?
Axios is a promise-based HTTP Client for Node.js which can be used to make requests to the Java backend services.
Why Use Axios?
- Simple syntax for making HTTP requests.
- Promises for better async handling.
- Interceptors to manage requests and responses from the Java backend effortlessly.
Example Code: Making a GET Request with Axios
const axios = require('axios');
axios.get('http://localhost:8080/api/users/1')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error fetching user:', error);
});
This piece of code shows how to fetch user data from a Java backend REST API using Axios.
2. Socket.IO
What is Socket.IO?
Socket.IO is a library that enables real-time, bidirectional communication between web clients and servers.
Why Use Socket.IO?
- Facilitates real-time communication for applications that require instant updates (like chat apps).
- Can connect seamlessly with Java backends to push real-time notifications or updates.
Example Code: Setting Up Socket.IO
const io = require('socket.io')(3000);
io.on('connection', socket => {
console.log('New client connected: ' + socket.id);
socket.on('message', data => {
console.log('Message from client:', data);
});
});
The example above illustrates setting up a Socket.IO server that listens for incoming connections and messages.
Best Practices for Integration
Keep Services Decoupled
By ensuring that Java and Node.js services remain decoupled, you can scale them independently according to your needs.
Utilize Docker
Using Docker allows you to create containers for both Java and Node.js applications, helping in maintaining environment consistency and simplifying deployment.
Monitor Performance
Employ monitoring tools (like Prometheus and Grafana) to track both Java and Node.js performance. This aids in identifying bottlenecks or issues within integrated services.
The Closing Argument
Bridging Java and Node.js not only enhances the capabilities of your applications but also optimizes the development workflow. The combination of robust REST APIs built with Spring Boot and high-speed, non-blocking I/O from Node.js creates a potent tech stack for developers.
For those looking to expand their Node.js experience, be sure to check out the article "Top Essential Node.js Packages Every Developer Needs in 2023" here. It can provide additional context for the best packages to complement your hybrid Java-Node.js applications.
By strategically choosing packages and adhering to best practices, building hybrid applications can become a smooth journey, paving the way for innovative solutions that leverage the strengths of both worlds. Now it’s your turn to explore the possibilities and embrace the future of hybrid application development!