Integrating Java with Node.js: Best Practices for Developers
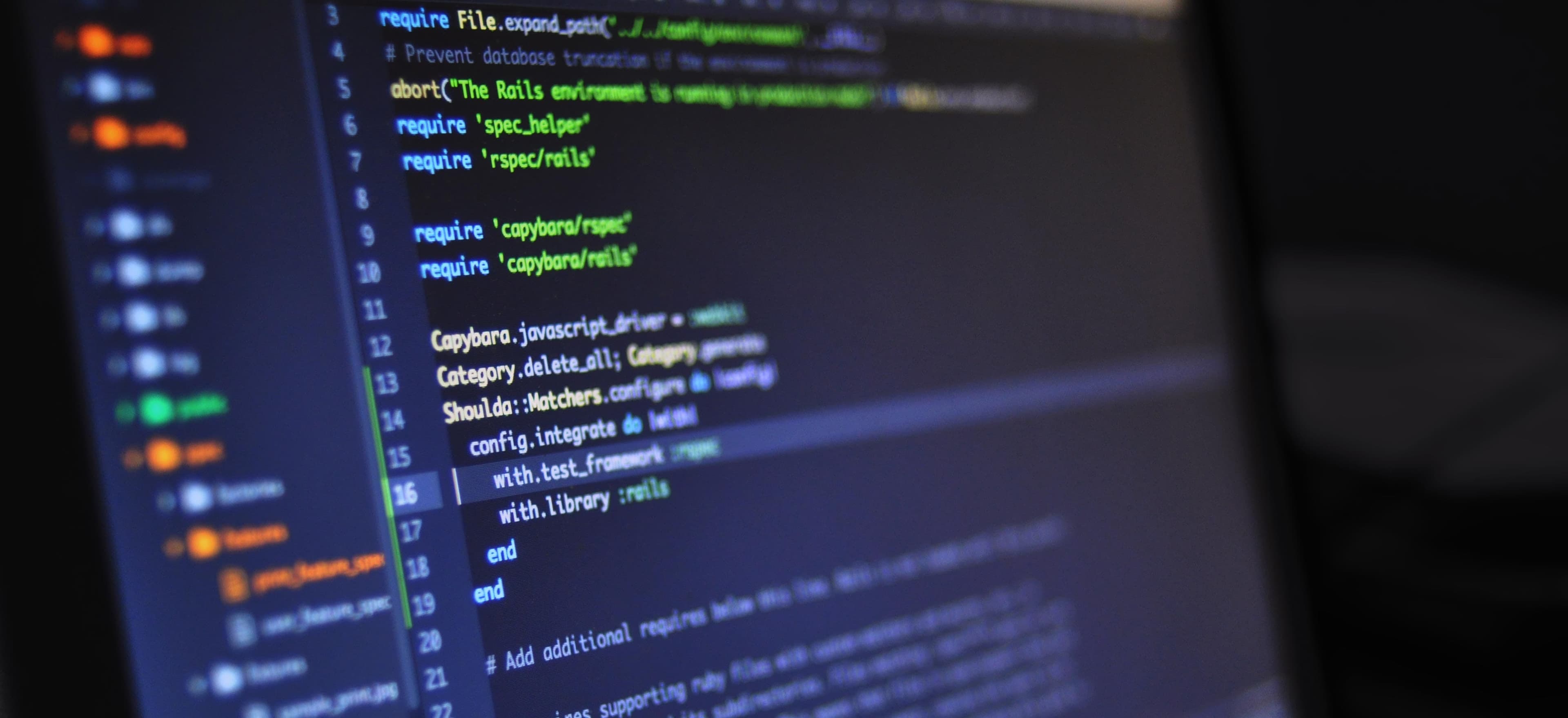
- Published on
Integrating Java with Node.js: Best Practices for Developers
As modern applications evolve, the need for integrating diverse technologies becomes ever more critical. In particular, connecting Java with Node.js can harness the strengths of both platforms, offering robustness and speed. This guide will walk you through the best practices for integrating Java with Node.js, ensuring a seamless experience for developers.
Why Integrate Java and Node.js?
Java is a widely-used object-oriented programming language known for its reliability, while Node.js is recognized for its efficiency and speed in handling asynchronous I/O operations. By combining both, developers gain the ability to build high-performance applications.
- Java's Strengths: Robust libraries, multithreading capabilities, rich ecosystems (like Spring), and extensive community support.
- Node.js's Strengths: Asynchronous non-blocking architecture, a vast number of available packages, and ease of building APIs.
Best Practices for Integration
1. Use RESTful APIs for Communication
One of the most effective methods to integrate Java with Node.js is through RESTful APIs. This approach decouples the applications and allows them to communicate over HTTP.
Setting Up a Simple REST API with Java (Spring Boot)
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api")
public class HelloController {
@GetMapping("/hello")
public String hello() {
return "Hello from Java!";
}
}
In this example, we created a simple REST API endpoint in Java using Spring Boot. The method hello()
returns a simple greeting.
Why RESTful APIs? Using REST allows systems to be loosely coupled. The Node.js application can make HTTP requests to the Java backend without needing to understand its internal logic.
2. Leverage Message Brokers for Asynchronous Communication
Another approach for integrating Java with Node.js is to use message brokers like RabbitMQ or Apache Kafka. This method provides a robust way to handle asynchronous communication.
Example Setup with RabbitMQ (Node.js)
const amqp = require('amqplib/callback_api');
amqp.connect('amqp://localhost', (error0, connection) => {
if (error0) throw error0;
connection.createChannel((error1, channel) => {
if (error1) throw error1;
const exchange = 'direct_logs';
const msg = 'Hello from Node.js!';
channel.publish(exchange, '', Buffer.from(msg));
console.log(" [x] Sent %s", msg);
});
});
In this code snippet, we establish a connection to RabbitMQ from a Node.js application, sending a message to a predefined exchange.
Why Use Message Brokers? Message brokers provide a way to decouple components of your system, allowing Java and Node.js applications to communicate without direct dependencies.
3. Use Inter-Process Communication (IPC)
In scenarios where performance is crucial, IPC techniques like shared memory or sockets can help you achieve high-speed data communication between your Java and Node.js applications.
Example of TCP Socket Communication in Node.js
const net = require('net');
const client = new net.Socket();
client.connect(1337, '127.0.0.1', () => {
console.log('Connected');
client.write('Hello from Node.js!');
});
Here we create a TCP socket client that connects to a server (potentially a Java application).
What’s the Advantage? IPC provides high speed; however, it introduces complexity in error handling and resource management. It is best reserved for performance-critical situations.
4. Invest in Serialization Performance
When transferring data between Java and Node.js, serialization and deserialization can become bottlenecks. Consider using efficient formats such as Protocol Buffers, Avro, or FlatBuffers to minimize overhead.
Example Using JSON in Node.js
const http = require('http');
http.createServer((req, res) => {
const data = { message: 'Hello from Node.js!' };
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify(data));
}).listen(3000);
Why Focus on Serialization? Efficient serialization minimizes bandwidth usage and improves response times, crucial for integrated applications.
5. Handle Error Management Gracefully
A robust error handling strategy is vital in any application, particularly when integrating technologies. Both Java and Node.js have different handling mechanisms, which means you need to standardize how errors are communicated.
Example of Error Handling in Java
try {
// Your logic
} catch (Exception e) {
// Handle specific exceptions
System.err.println("An error occurred: " + e.getMessage());
}
Node.js Error Handling
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something broke!');
});
By implementing both Java and Node.js error handling techniques, you can ensure that errors are captured and logged across your integrated applications.
6. Conclusion: A Unified Approach to Integration
Integrating Java with Node.js can unlock tremendous potential for your applications. You can also refer to the existing blog post, "Top Essential Node.js Packages Every Developer Needs in 2023", at infinitejs.com/posts/essential-node-js-packages-2023 for invaluable packages that can further enhance your Node.js applications.
By following these best practices, you can harness the strengths of both programming languages to build responsive, efficient, and scalable applications. Whether you choose RESTful APIs, message brokers, or IPC, the right strategy for integration will depend on your particular use case.
Final Thoughts
As you delve into integrating Java with Node.js, always keep scalability and maintainability in mind. Experiment with various approaches and utilize the techniques discussed above to find what works best for your development environment. The possibilities are endless when you choose to blend the power of Java with the speed of Node.js!
By following these guidelines, your integration of Java and Node.js will be streamlined for both performance and maintainability. Happy coding!
Checkout our other articles