How to Uncover Java Thread Deadlocks Using Groovy and JMX
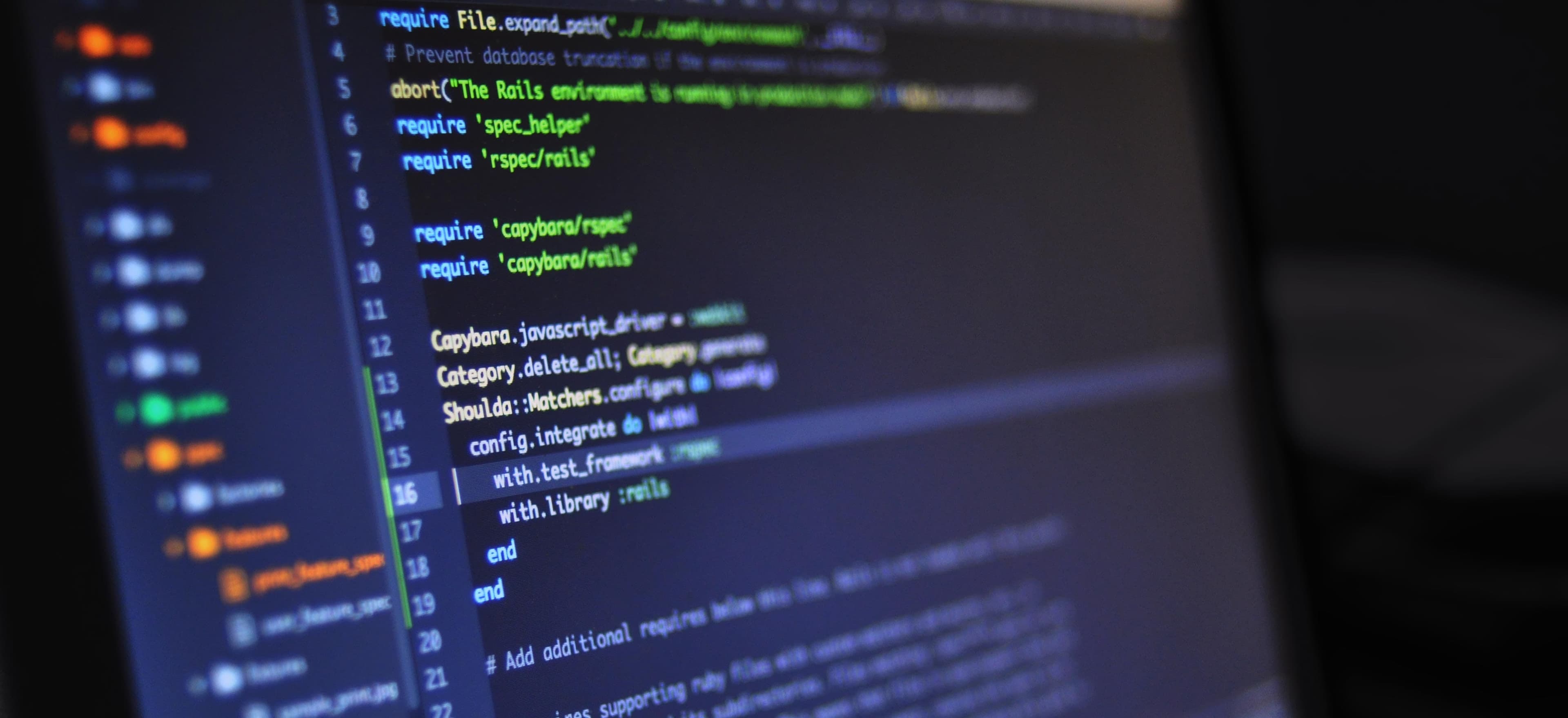
- Published on
How to Uncover Java Thread Deadlocks Using Groovy and JMX
Thread deadlocks are a significant issue in concurrent programming, and they can cause applications to hang indefinitely. This problem occurs when two or more threads are waiting for each other to release resources, leading to a cycle of dependencies that can be challenging to diagnose. Fortunately, tools like Groovy and Java Management Extensions (JMX) are here to help us uncover and analyze these deadlocks.
In this blog post, we will delve into how to identify Java thread deadlocks using Groovy alongside JMX. By the end of this guide, you'll have a solid understanding of how deadlocks work and how to leverage these tools effectively.
Understanding Deadlocks
Before we dive into the technical details, let's clarify what a deadlock is. A deadlock happens when two or more threads are unable to proceed because each is waiting for the other to release a lock. Consider the following example:
- Thread A holds Lock 1 and waits for Lock 2.
- Thread B holds Lock 2 and waits for Lock 1.
In this scenario, both threads are stalled indefinitely. This situation necessitates proactive monitoring and diagnostic measures.
Setting Up JMX
Java Management Extensions (JMX) is a powerful framework that allows you to manage and monitor Java applications. It provides a way to expose Java resources such as memory, threads, and performance metrics.
Step 1: Enable JMX for Your Application
To enable JMX, you typically need to add several options when starting your Java application. Below are the commonly used options:
java -Dcom.sun.management.jmxremote \
-Dcom.sun.management.jmxremote.port=9999 \
-Dcom.sun.management.jmxremote.authenticate=false \
-Dcom.sun.management.jmxremote.ssl=false \
-Djava.rmi.server.hostname=localhost \
-jar your-application.jar
- jmxremote: This enables remote monitoring.
- jmxremote.port: The port on which the JMX agent will listen.
- authenticate and ssl: Options for security. Although set to false for simplicity, it is highly recommended to enable these in production.
Using Groovy to Interact with JMX
Once JMX is set up, we need a way to interact with it programmatically. Groovy, a powerful scripting language for the JVM, offers a natural syntax for working with Java classes, including the JMX API.
Step 2: Install Groovy
You can install Groovy in several ways, including via:
- SDKMAN
- Homebrew (macOS)
- Direct download from the Groovy website
Step 3: Groovy Script to Retrieve Thread Information
Here is a simple script that uses Groovy to connect to JMX and retrieve thread information, particularly focusing on deadlocks.
import javax.management.MBeanServerConnection
import javax.management.ObjectName
import javax.management.remote.JMXConnectorFactory
import javax.management.remote.JMXServiceURL
def jmxUrl = new JMXServiceURL("service:jmx:rmi:///jndi/rmi://localhost:9999/jmxrmi")
def jmxConnector = JMXConnectorFactory.connect(jmxUrl, null)
def mBeanServerConnection = jmxConnector.getMBeanServerConnection()
def threadMXBean = ManagementFactory.getPlatformMXBean(ThreadMXBean)
def deadlockedThreadIds = threadMXBean.findDeadlockedThreads()
if (deadlockedThreadIds) {
def deadlockedThreadInfo = threadMXBean.getThreadInfo(deadlockedThreadIds)
deadlockedThreadInfo.each { info ->
println "Thread ${info.threadName} (ID: ${info.threadId}) is deadlocked."
}
} else {
println "No deadlocked threads found."
}
jmxConnector.close()
Explanation of the Script
-
Import Necessary Classes: The script starts by importing required classes to work with JMX.
-
Connect to JMX: The
JMXServiceURL
, along with the desired connection settings, is used to connect to your Java application. -
Retrieve Thread Information: The
ThreadMXBean
interface fetches details about all threads and potential deadlocks. -
Identify Deadlocked Threads: The method
findDeadlockedThreads()
returns the thread IDs of any threads that are currently in a deadlock. -
Display Information: If deadlocked threads are found, their names and IDs are printed out.
Running the Groovy Script
You can execute the Groovy script using the following command:
groovy deadlockMonitor.groovy
Ensure your Java application is running and that JMX is enabled on the specified port. This will allow you to monitor it for deadlocks in real-time.
Analyzing the Output
After running your script, if deadlocks are present, you will see output indicating which threads are involved:
Thread Thread-1 (ID: 13) is deadlocked.
Thread Thread-2 (ID: 14) is deadlocked.
If no deadlocks exist, you may see:
No deadlocked threads found.
Further Analysis via Thread Dumps
While the above script is an excellent starting point, a deeper analysis might require a thread dump. A thread dump provides a snapshot of all threads and the locks they hold or are waiting for.
You can create a thread dump using the jstack
command:
jstack -l <pid>
Replace <pid>
with your process ID. Examining a thread dump can yield insights into not only deadlocks but also thread states, which can help identify potential performance bottlenecks.
Bringing It All Together
Deadlocks can be a silent killer in multi-threaded Java applications, leading to system hangs and poor performance. By combining Groovy and JMX, you can effectively monitor for deadlocks and gain valuable insights into thread behavior.
The provided Groovy script and analysis techniques can significantly streamline your debugging processes. Just remember to regularly monitor and test your applications, and you'll minimize the risk of running into deadlocks.
For further reading on thread management and performance tuning in Java, consider checking out the Java Concurrency in Practice book and explore more about JMX in the Oracle documentation.
Final Notes
As you adopt these practices, enhance your error handling and logging strategies for better diagnostics in real-world applications. Happy coding!
Checkout our other articles