The Importance of Test Writing in Effective Testing Techniques
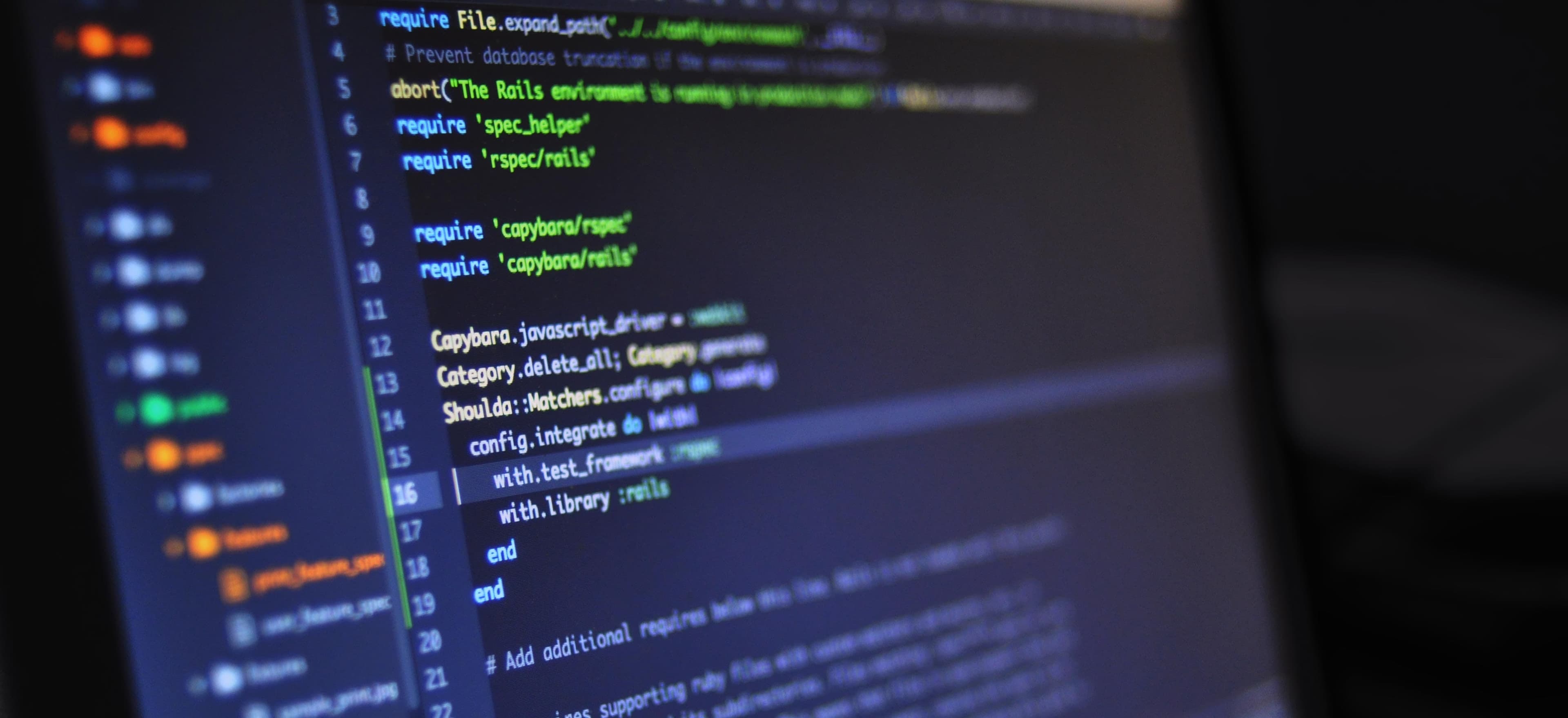
- Published on
The Importance of Test Writing in Effective Testing Techniques
In the world of software development, the adage "test early, test often" rings particularly true. Testing is not merely a stage in the software development lifecycle; it is an ongoing practice that ensures software is reliable, functional, and user-friendly. At the heart of effective testing techniques lies the practice of comprehensive test writing. This blog post delves into the significance of test writing, how to create effective tests, and best practices to elevate your testing game.
Understanding Test Writing
Test writing involves creating a set of conditions or variables under which a tester will determine if a system or one of its components is working as expected. While this may seem straightforward, effective test writing demands a deeper understanding of the application’s functionality and the specific requirements it aims to fulfill.
Why Test Writing Matters
-
Clarity of Requirements: Well-written tests clarify what is expected of the software. They ensure that developers and testers share a common understanding of the software's functionality.
-
Facilitates Communication: Tests act as a form of documentation that can be referenced by both developers and stakeholders. This fosters collaboration and ensures alignment on project goals.
-
Reduces Bugs: By writing tests early in the development process (a technique known as Test-Driven Development or TDD), developers can detect and resolve issues before they escalate. This approach significantly reduces the likelihood of bugs slipping into production.
-
Streamlines Refactoring: When code needs to be changed or refactored, having a suite of tests ensures that new changes don't break existing functionality. Instant feedback from tests boosts confidence during modifications.
-
Serves as Regression Checks: Whenever new features are added, tests ensure that previous functionality remains unbroken, making it easier to incorporate changes without fear of unintended consequences.
Types of Tests
To maximize the effectiveness of your testing efforts, it is crucial to understand the various types of tests and their purposes.
1. Unit Tests
Unit tests are designed to validate individual components of the software. They focus on small units of code, such as functions or methods, in isolation from the rest of the application.
Example of a Unit Test
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
private Calculator calculator = new Calculator();
@Test
public void testAdd() {
// Test case for the addition method
assertEquals(5, calculator.add(2, 3));
}
}
Commentary
In this example, we create a simple unit test for a hypothetical Calculator
class. The testAdd
method validates that the add
function produces the correct result. Clear expectations drive developers to implement features that precisely fulfill requirements.
2. Integration Tests
Integration tests evaluate how different modules of an application work together. They help identify interface defects that unit tests typically overlook.
Example of an Integration Test
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertTrue;
public class UserServiceIntegrationTest {
private UserService userService;
@Test
public void testUserCreationWorkflow() {
// Create a user and save it, then retrieve it from the database
User user = new User("Alice", "alice@example.com");
userService.createUser(user);
User retrievedUser = userService.getUserByEmail("alice@example.com");
assertTrue(retrievedUser != null);
assertEquals("Alice", retrievedUser.getName());
}
}
Commentary
In this integration test, we ensure that UserService
creates a user and successfully retrieves it. This interaction is vital for confirming that various components (like database operations and user creation) work in harmony.
3. End-to-End Tests
End-to-end testing simulates a user's interaction with the application, verifying that all components operate correctly together. This approach is crucial for user satisfaction.
Writing Effective Tests
Effective test writing goes beyond syntax; it requires a strategic approach. Here are some tips:
1. Adopt Naming Conventions
Use descriptive names for your test methods that clearly indicate what is being tested. This practice enhances readability and understanding.
@Test
public void shouldReturnUserWhenValidEmailProvided() {
// test implementation
}
2. Keep Tests Independent
Each test should be able to run in isolation. Managing dependencies minimizes false positives and negatives, allowing each test to evaluate its own functionality without side effects.
3. Use Assertions Wisely
Assert statements should reflect not only what you expect but why. This provides additional context for future developers attempting to understand the test's purpose.
assertEquals(expectedResult, actualResult, "The output should match the expected result based on the input values");
4. Maintain a Test Plan
Create and maintain a comprehensive test plan documenting all tests, their purposes, and their statuses. This ensures that tests are regularly updated and relevant to the current codebase.
5. Automation is Key
Invest in test automation frameworks that fit your project's needs. Frameworks like JUnit and TestNG for Java enable automated test execution, enhancing efficiency and time management.
Tools for Test Writing
Numerous tools can aid in writing robust tests. Here are a few notable mentions:
-
JUnit: A widely-used testing framework for Java that simplifies the creation and execution of tests. Learn more about JUnit here.
-
Mockito: A mocking framework for Java that enables creating and configuring mock objects, simplifying the testing of complex interactions.
-
Selenium: An open-source tool that enables automation of web applications for testing purposes, particularly effective for end-to-end testing.
The Closing Argument
Test writing is not just an afterthought; it is an essential practice that empowers developers and organizations to produce high-quality software. By embracing the principles of effective test writing and integrating various types of tests into your development workflow, you create a more robust, reliable, and maintainable codebase.
As you embark on your journey of enhancing your software testing strategies, keep in mind the significant role that well-crafted tests play. With clear expectations, reduced bugs, and the ability to adapt to change, investing in test writing is an invaluable asset for any software development team.
Explore further: Don’t forget to check out resources like Martin Fowler's blog on testing to deepen your understanding of testing techniques and methodologies. Happy Testing!
Checkout our other articles