Troubleshooting LocalDateTime Format Issues in Java 8
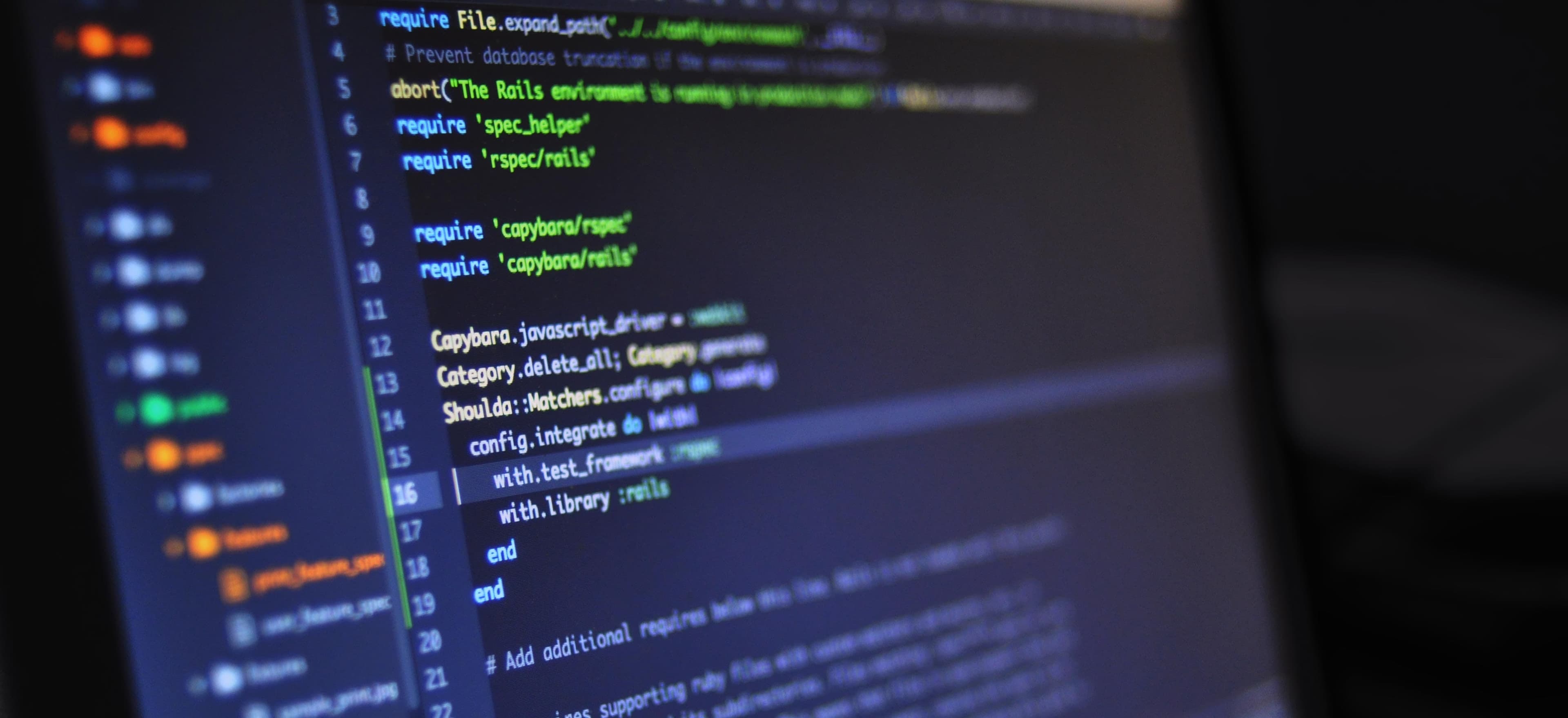
- Published on
Troubleshooting LocalDateTime Format Issues in Java 8
Java 8 introduced a new, powerful date and time API, which includes the LocalDateTime
class. While this is an improvement over previous date handling in Java, developers frequently encounter formatting issues. In this blog post, we will explore the common problems associated with LocalDateTime
formatting, how to troubleshoot them, and provide best practices to avoid future mishaps.
Understanding LocalDateTime
LocalDateTime
is part of the java.time
package, providing a way to represent date and time without any timezone information. It can be particularly helpful when dealing with dates and times which are not dependent on the current time zone.
Below is a simple example of how to create a LocalDateTime
instance:
import java.time.LocalDateTime;
public class LocalDateTimeExample {
public static void main(String[] args) {
LocalDateTime now = LocalDateTime.now();
System.out.println("Current Date and Time: " + now);
}
}
Output:
Current Date and Time: 2023-10-07T10:15:30.123
In this example, we obtain the current date and time and print it in the default format, which includes the date, time, and nanoseconds.
Common Formatting Issues
-
Default Format Confusion
- The output of
LocalDateTime.now()
is usually not what users expect. It uses the ISO-8601 standard formatyyyy-MM-dd'T'HH:mm:ss.SSS
. - Why is this an issue? Because many applications require a different format for users, like
"dd/MM/yyyy HH:mm"
or"MMMM dd, yyyy"
.
- The output of
-
Parsing Formats
- When converting a string to
LocalDateTime
, one might use the default format, leading to aDateTimeParseException
. - This often occurs when parsing strings without explicitly defining the format.
- When converting a string to
-
Locale Sensitivity
- The
LocalDateTime
class does not account for localization. Formatting a date in different locales might also lead to confusion if not properly managed.
- The
Troubleshooting Formatting Issues
1. Custom Formatting with DateTimeFormatter
The key to managing date and time formats in Java is the DateTimeFormatter
. You can create custom formats as shown below:
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class CustomFormatExample {
public static void main(String[] args) {
LocalDateTime now = LocalDateTime.now();
// Create a custom formatter
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd/MM/yyyy HH:mm");
// Format LocalDateTime to String
String formattedDateTime = now.format(formatter);
System.out.println("Formatted Date and Time: " + formattedDateTime);
}
}
Output:
Formatted Date and Time: 07/10/2023 10:15
Why This Works:
Using DateTimeFormatter
allows you to specify exactly how you want your date and time represented. This can reduce parsing errors since you control the format.
2. Parsing Strings to LocalDateTime
When converting a string representation of date and time into a LocalDateTime
object, the format must match:
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import java.time.format.DateTimeParseException;
public class ParsingExample {
public static void main(String[] args) {
String dateTimeString = "07/10/2023 10:15";
// Create the formatter
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd/MM/yyyy HH:mm");
try {
// Parse String to LocalDateTime
LocalDateTime parsedDateTime = LocalDateTime.parse(dateTimeString, formatter);
System.out.println("Parsed LocalDateTime: " + parsedDateTime);
} catch (DateTimeParseException e) {
System.out.println("Error parsing the date: " + e.getMessage());
}
}
}
Output:
Parsed LocalDateTime: 2023-10-07T10:15
Importance:
When parsing dates, specifying the same format that matches the input string is essential to prevent errors. This delivers predictable results.
Best Practices
-
Always Define Formats
- When performing formatting or parsing, specify a format. This avoids confusion and bugs down the line.
-
Use ISO Formats When Possible
- ISO formats are universally understood and recognized. If possible, stick to them for communication between systems.
-
Handle Time Zones Separately
- Since
LocalDateTime
does not handle time zones, be cautious when your application interacts with users in different regions. UseZonedDateTime
when time zone information is necessary.
- Since
-
Test Extensively
- Test your application with edge cases, such as leap years or end-of-month scenarios. The more exhaustive your test cases, the fewer surprises you will encounter in production.
-
Consider the User Experience
- If your application has users from different locales, consider implementing localization for date formats. External libraries like Joda-Time or modern tools can help handle that complexity.
Closing the Chapter
Navigating formatting issues with LocalDateTime
in Java is manageable once you understand the foundational concepts and best practices. Developing a habit of using DateTimeFormatter
effectively will lead to fewer errors and a more robust application.
With careful formatting, parsing strategies, and a clear understanding of how dates and times work in Java, you'll be able to build applications that run smoothly and provide a great user experience. For more information about date and time handling in Java, check out the Java documentation.
By following these guidelines, you can streamline your development process, avoid common pitfalls, and deliver better solutions to your users. Happy coding!
Checkout our other articles