Debugging Connection Issues with Stomp over WebSocket
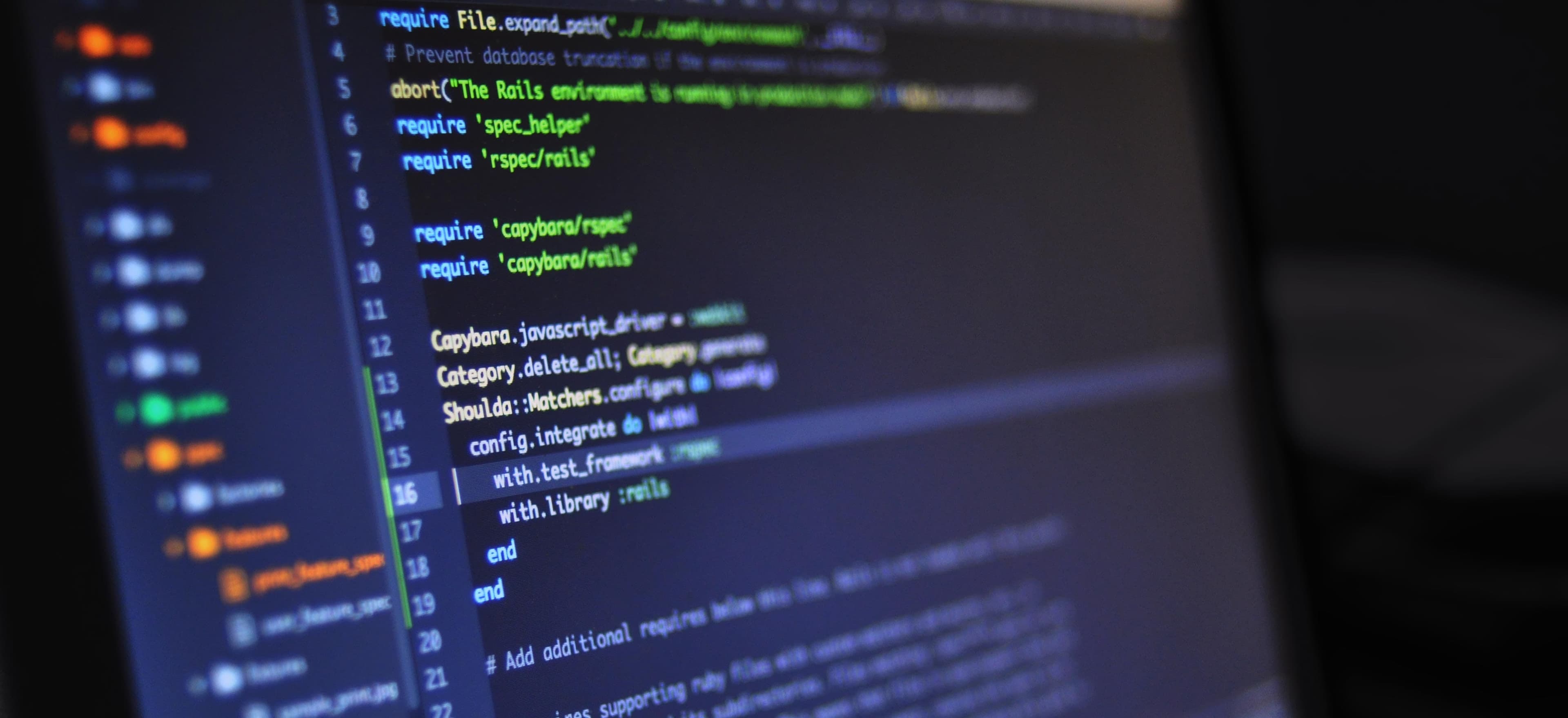
- Published on
Debugging Connection Issues with STOMP over WebSocket
WebSocket is a powerful protocol that allows real-time communication between clients and servers. When combined with the STOMP (Simple Text Oriented Messaging Protocol), developers can create responsive applications that leverage message queues. However, debugging connection issues with STOMP over WebSockets can be tricky. This blog post will guide you through identification, troubleshooting, and fixing common connection issues, ensuring smoother application performance.
Understanding the Basics
What is STOMP?
STOMP is a simple and widely used protocol for messaging. It allows different systems to communicate in a standardized way. Its text-based format makes it easy for developers to debug and trace messages.
What are WebSockets?
WebSockets provide a full-duplex communication channel over a single TCP connection. This means the server can send messages to the client without the client having to request them first, making it perfect for real-time applications.
Why Use STOMP Over WebSocket?
Using STOMP over WebSockets gives you the benefits of both protocols—real-time communication with easy-to-implement messaging features. It is especially useful in chat applications, online gaming, and collaborative tools.
Common Connection Issues
Before diving into debugging techniques, let's first outline common issues that developers face when dealing with STOMP over WebSockets.
- CORS Issues: Cross-Origin Resource Sharing (CORS) restrictions can prevent connections if the WebSocket server is on a different domain.
- Incorrect Endpoint URL: Providing a wrong WebSocket URL will lead to connection failures.
- Network Issues: Firewalls or proxy servers might block WebSocket traffic.
- Server Not Configured for STOMP: The server might not be set up to handle STOMP messages correctly.
- Incorrect Protocol Implementation: Mismatch between client and server STOMP versions or settings.
Step-by-Step Debugging Process
Step 1: Verify WebSocket Connection
Before testing STOMP, ensure that the WebSocket connection is established properly.
const socket = new WebSocket('ws://your-server.com/websocket');
// Connection opened
socket.addEventListener('open', (event) => {
console.log('WebSocket is connected.');
});
// Listen for messages
socket.addEventListener('message', (event) => {
console.log('Message from server: ', event.data);
});
// Handle error
socket.addEventListener('error', (event) => {
console.error('WebSocket error: ', event);
});
This code snippet helps in establishing a WebSocket connection while logging connection events. If you see a 'WebSocket is connected.' message, your WebSocket layer is functioning correctly.
Step 2: Check CORS Configuration
If your client and server are on different domains, you'll need to make sure your server supports CORS for WebSockets.
To set CORS in a Node.js server using Express, you can use the following code:
const express = require('express');
const cors = require('cors');
const app = express();
// Enable CORS for WebSocket connections
app.use(cors({
origin: 'http://your-client-domain.com',
methods: ['GET', 'POST'],
allowedHeaders: ['Content-Type', 'Authorization']
}));
Step 3: Review Server Logs
Server logs are your best friend when it comes to debugging. Check for any error messages during connection attempts or STOMP message processing on your server.
Log levels should be appropriately set to display connection issues.
Step 4: Validate STOMP Configuration
Make sure the STOMP configuration on both client and server is consistent. Here’s an example of how a client can connect using STOMP with a WebSocket:
const Stomp = require('stompjs');
const url = 'ws://your-server.com/websocket';
const client = Stomp.over(new SockJS(url));
client.connect({}, (frame) => {
console.log(`Connected: ${frame}`);
}, (error) => {
console.error('Connection error: ', error);
});
Ensure the protocol versions and message formats align between the client and server configurations.
Step 5: Use a STOMP Client to Test
Using a STOMP client can help pinpoint where the issue lies. Tools like Stomp.js, or GUI-based clients like RabbitMQ Management Plugin, can be invaluable in this step.
Try connecting directly to your STOMP endpoint using an external STOMP client to verify the server is responsive.
Step 6: Test Network Connectivity
Make sure your network is not blocking WebSocket requests. Use tools like ping
or traceroute
to see if packets reach your server.
You can also use the browser's developer tools to inspect the WebSocket connection. In Chrome, you can find this under the Network tab by filtering for "WS".
Step 7: Fallback Mechanisms
Consider implementing fallback mechanisms to retry connections. WebSocket may fail, but a well-designed application gracefully handles such scenarios.
function connect() {
const socket = new WebSocket('ws://your-server.com/websocket');
socket.onopen = () => {
console.log('WebSocket connected.');
// Subscribe to STOMP topics here
};
socket.onerror = (error) => {
console.error('WebSocket Error:', error);
// Retry connection after a delay
setTimeout(connect, 5000);
};
}
connect();
Step 8: Monitor Server Performance
Sometimes, server performance can lead to connection timeouts. Regularly monitor your server’s CPU and memory usage, and consider scaling if needed.
Closing the Chapter
Debugging STOMP over WebSocket issues can be a complex process, but utilizing the above steps will help pinpoint and resolve common problems efficiently. Always ensure that both your client and server implementations are compatible, and don't hesitate to turn to server logs and monitoring tools for deeper insights.
Additional Resources
By following these guidelines, you should be well on your way to resolving any STOMP over WebSocket connection challenges you encounter. Happy coding!
Checkout our other articles