Overcoming Challenges with BinarySecurityToken Authentication
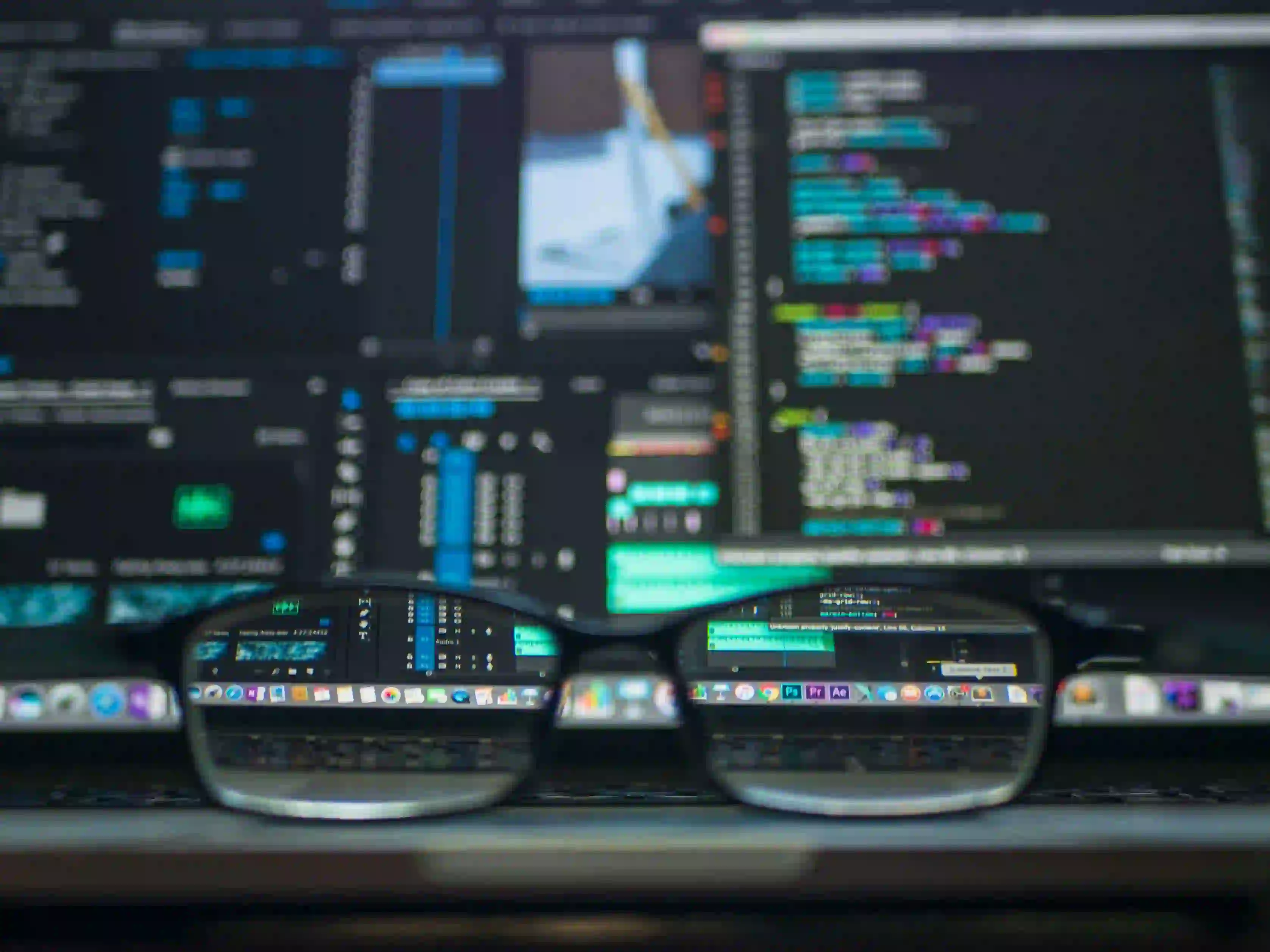
Overcoming Challenges with BinarySecurityToken Authentication
In the world of web services, security is paramount. One often-used method to ensure secure communication is through the BinarySecurityToken (BST) authentication. This technique provides a way to convey security tokens, which can authenticate users and services during operations. However, like any method, BST comes with its own set of challenges. In this post, we will examine the BinarySecurityToken and its usage, explore the common obstacles faced when implementing it, and propose strategies to overcome these hurdles.
What is BinarySecurityToken?
A BinarySecurityToken is an XML-based token that contains security-related information. This token encapsulates authentication credentials that applications use to establish a secure connection. It is an integral part of the WS-Security specification, which delineates the requirements for securing web services.
Example of a BinarySecurityToken
Here’s a sample implementation of a BinarySecurityToken:
<wsse:Security>
<wsse:BinarySecurityToken
ValueType="http://schemas.xmlsoap.org/ws/2002/12/secext"
EncodingType="http://schemas.xmlsoap.org/ws/2009/09/secext"
xmlns:wsse="http://schemas.xmlsoap.org/ws/2002/12/secext">
BASE64ENCODED-TOKEN-VALUE
</wsse:BinarySecurityToken>
</wsse:Security>
This XML snippet illustrates how the BinarySecurityToken is represented within security headers. The token is base64-encoded to ensure safe transmission over web protocols.
Challenges with BinarySecurityToken Authentication
While BinarySecurityToken authentication offers robust security, it is not without difficulties:
1. Token Generation and Validation Complexity
Generating and validating tokens can be cumbersome. Depending on the architecture of your application, you might end up wrestling with issues like token expiration and ensuring the token is valid at the time of request.
Solution
Utilize a library that abstracts the complexity. For instance, if you're using Java, you can leverage Apache WSS4J, which provides a straightforward way to generate and validate BinarySecurityTokens.
Here’s how to generate a BinarySecurityToken using WSS4J:
import org.apache.wss4j.common.ext.WSSecurityException;
import org.apache.wss4j.dom.handler.WSSHandlerConstants;
import org.apache.wss4j.dom.WSSecurityUtil;
import org.w3c.dom.Document;
import javax.xml.bind.DatatypeConverter;
public class SecurityTokenGenerator {
public static String generateToken(Document doc) throws WSSecurityException {
String rawToken = "your-raw-security-token"; // Example raw token
return DatatypeConverter.printBase64Binary(rawToken.getBytes());
}
}
In this snippet, we leverage DatatypeConverter
to convert our raw token into a Base64 encoded format. This approach simplifies token conversion, ensuring it is compatible with the expected BinarySecurityToken format in WS-Security.
2. Token Management Overhead
Managing tokens, especially when dealing with multiple users or services, can add an administrative burden. Each token needs to be created, stored, validated, and invalidated at appropriate states.
Solution
Implementing a token management system can alleviate this burden. This may involve creating a dedicated service that handles all operations related to token management. Using a centralized database for token storage, it makes it easier to track tokens and their states.
3. Interoperability Issues
Different systems or services may implement security protocols variably, leading to interoperability problems. A service expecting a BST may not understand the format generated by your service.
Solution
To minimize interoperability issues, adhere to industry standards and utilize frameworks that promote compatibility. The following code demonstrates setting up WS-Security parameters using WSS4J:
import org.apache.wss4j.dom.handler.WSSHandlerConstants;
import javax.xml.ws.BindingProvider;
public void configureWSClient(BindingProvider proxy) {
Map<String, Object> context = proxy.getRequestContext();
context.put(WSSHandlerConstants.ACTION, WSSHandlerConstants.BINARY_SECURITY_TOKEN);
context.put(WSSHandlerConstants.USER, "your-username");
context.put(WSSHandlerConstants.PASSWORD_TYPE, WSSHandlerConstants.PASSWORD_TEXT);
}
By appropriately configuring the WS client, we ensure that it generates a BST that is recognizable by other compliant systems, thereby mitigating interoperability issues.
4. Security Vulnerabilities
The use of tokens can introduce security vulnerabilities if not properly managed. Tokens can be intercepted or reused by unauthorized parties if proper measures are not put in place.
Solution
Implement secure token transmission using HTTPS to encrypt data in transit. Additionally, consider implementing a token expiration strategy. In Java, this could be accomplished through:
import java.util.Date;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
public class TokenService {
private static final String SECRET_KEY = "your-secure-secret-key";
public String createToken(String username) {
return Jwts.builder()
.setSubject(username)
.setExpiration(new Date(System.currentTimeMillis() + 3600000)) // 1 hour
.signWith(SignatureAlgorithm.HS256, SECRET_KEY)
.compact();
}
}
In this example, we created a token valid for just one hour. By limiting the lifespan of our tokens, we mitigate the risk of token exploitation.
5. Debugging and Error Handling
Due to the layered complexities of security, debugging issues related to BinarySecurityToken can become a daunting task.
Solution
Implement detailed logging and consistent error handling. Tracking security-related logs can help in quickly identifying and rectifying issues. Here's an example of logging during token generation:
import java.util.logging.Logger;
public class SecureService {
private static final Logger logger = Logger.getLogger(SecureService.class.getName());
public void generateToken(String user) {
try {
// Token generation logic
logger.info("Token generated successfully for user: " + user);
} catch (Exception e) {
logger.severe("Error generating token for user: " + user + " - " + e.getMessage());
}
}
}
In this code snippet, we log both successful generation and any encountered errors, enabling easier diagnostics for troubleshooting.
Closing Remarks
BinarySecurityToken authentication is a powerful component in securing web services, but it does not come without its challenges. By recognizing risks around token management, interoperability, and security vulnerabilities, we can take proactive steps to mitigate these issues. Leveraging libraries such as Apache WSS4J and effectively managing tokens can simplify implementation and bolster security.
As you embark on your journey with BinarySecurityToken, remember that every issue has a potential solution. The key is to remain grounded in best practices, leverage robust libraries, and prioritize security above all.
For further reading and deeper understanding, consider checking out WS-Security Overview and explore available resources and tutorials on Apache WSS4J documentation.
By staying informed and prepared, you can ensure a smoother implementation of BinarySecurityToken authentication in your applications.