Resolving Compatibility Issues in JMetro for JavaFX 11
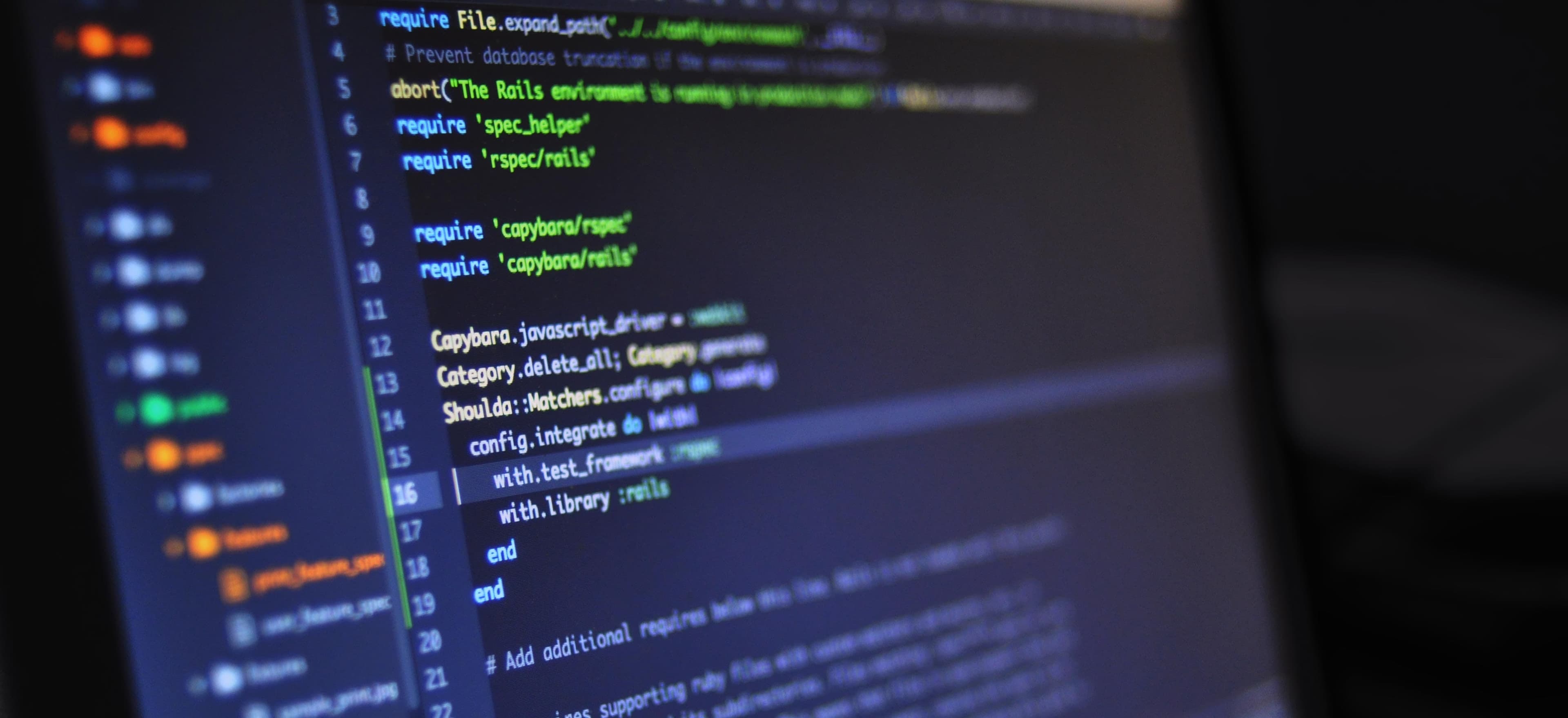
- Published on
Resolving Compatibility Issues in JMetro for JavaFX 11
JavaFX 11 is an upgrade that brought a significant improvement to the semantics and performance of Java desktop applications. As developers migrate to this new version, they often face compatibility issues, particularly when integrating external libraries, such as JMetro, which is a popular library for JavaFX that allows developers to create modern-looking applications with a Metro-style design.
In this blog post, we will delve into resolving compatibility issues encountered when using JMetro with JavaFX 11. We'll explore the key aspects of these issues, provide code snippets as examples, and share best practices to ensure a smooth development experience.
Understanding JMetro
JMetro is a JavaFX library inspired by Microsoft's Windows metro design language. It allows developers to apply a sleek and modern look to their JavaFX applications effortlessly. JMetro introduces several design components and themes to enhance user interaction.
However, with the release of JavaFX 11, which moved to a modular architecture, some issues arose regarding the compatibility of JMetro and JavaFX, leading developers to scramble for solutions.
Common Compatibility Issues
1. Missing Modules
One of the most common issues with JavaFX 11 is the need for explicit module declarations when using external libraries like JMetro. If you simply try to run a JavaFX application without configuring the module path correctly, you may encounter the dreaded error about missing modules.
Solution
To avoid these errors, ensure that your module-info.java
file declares the necessary modules. A typical module declaration for a JavaFX application using JMetro may look like this:
module my.application {
requires javafx.controls;
requires javafx.fxml;
requires javafx.graphics;
requires JMetro; // Assuming you're using JMetro as an external module
exports my.application;
}
2. Classpath Issues
Another frequent pitfall for many developers transitioning to JavaFX 11 is the confusion between the classpath and the module path. JavaFX has now moved its libraries to a module-based structure, which means you need to execute your application differently.
Solution
Ensure you are using the module path to run your application. The Java command should be modified to include the module path. You can do this with the following command in your terminal:
java --module-path /path/to/javafx-sdk-11/lib --add-modules javafx.controls,javafx.fxml, JMetro -jar myApplication.jar
Replace /path/to/javafx-sdk-11/lib
with the actual path to your local JavaFX library.
3. UI Rendering Issues
After integrating JMetro with JavaFX 11, you might encounter rendering issues such as components not displaying correctly or unexpected behavior in layout. This usually stems from incompatible CSS or styling.
Solution
When applying styles, ensure you're using the correct CSS files provided by JMetro. Also, ensure that you call JMetro
and apply the styles properly in your main application class.
Here's an example:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import org.jmetro.JMetro;
import org.jmetro.Style;
public class MyApp extends Application {
@Override
public void start(Stage primaryStage) {
StackPane root = new StackPane();
Scene scene = new Scene(root, 400, 300);
// Apply JMetro styling
JMetro jMetro = new JMetro(Style.LIGHT);
jMetro.setScene(scene);
primaryStage.setTitle("Hello JMetro with JavaFX 11");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this snippet, we're importing JMetro
and applying it to our scene. The JMetro
constructor receives a Style
as an argument, and you're free to choose between LIGHT
and DARK
.
4. API Differences
JavaFX 11 introduced several refinements in its API. Developers accustomed to older JavaFX versions may find themselves perplexed by deprecated methods or changed functionality.
Solution
It's crucial to refer to the JavaFX 11 documentation for any changes or deprecations that affect your code. If a certain API method has been deprecated, look for alternative methods provided, or seek community-driven solutions to common problems.
5. System Font Issues
Some developers reported that after integrating JMetro, their applications did not use the correct system font, leading to display issues.
Solution
Here's a simple solution to configure fonts:
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import org.jmetro.JMetro;
import org.jmetro.Style;
public class FontApp extends Application {
@Override
public void start(Stage stage) {
StackPane root = new StackPane();
Label label = new Label("Welcome to JMetro with JavaFX 11");
root.getChildren().add(label);
Scene scene = new Scene(root, 300, 200);
// Apply JMetro styling
JMetro jMetro = new JMetro(Style.LIGHT);
jMetro.setScene(scene);
// Set a preferred font
scene.getStylesheets().add(getClass().getResource("styles.css").toExternalForm());
stage.setTitle("Font Example");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In your styles.css
file, you can define the font:
.label {
-fx-font-family: "Segoe UI";
-fx-font-size: 14px;
}
Closing the Chapter
Transitioning to JavaFX 11 while integrating libraries like JMetro might present some compatibility challenges, but these hurdles can be effectively managed. By paying attention to module declarations, running configurations, correct method usage, and styling, you can ensure a smooth operation of your JavaFX applications.
For more resources and community assistance, consider visiting platforms like Stack Overflow or the OpenJFX community.
With this guide, you should be able to navigate the complexities of using JMetro with JavaFX 11 confidently. Happy coding!
Checkout our other articles