Understanding Common Hibernate Collection Cache Pitfalls
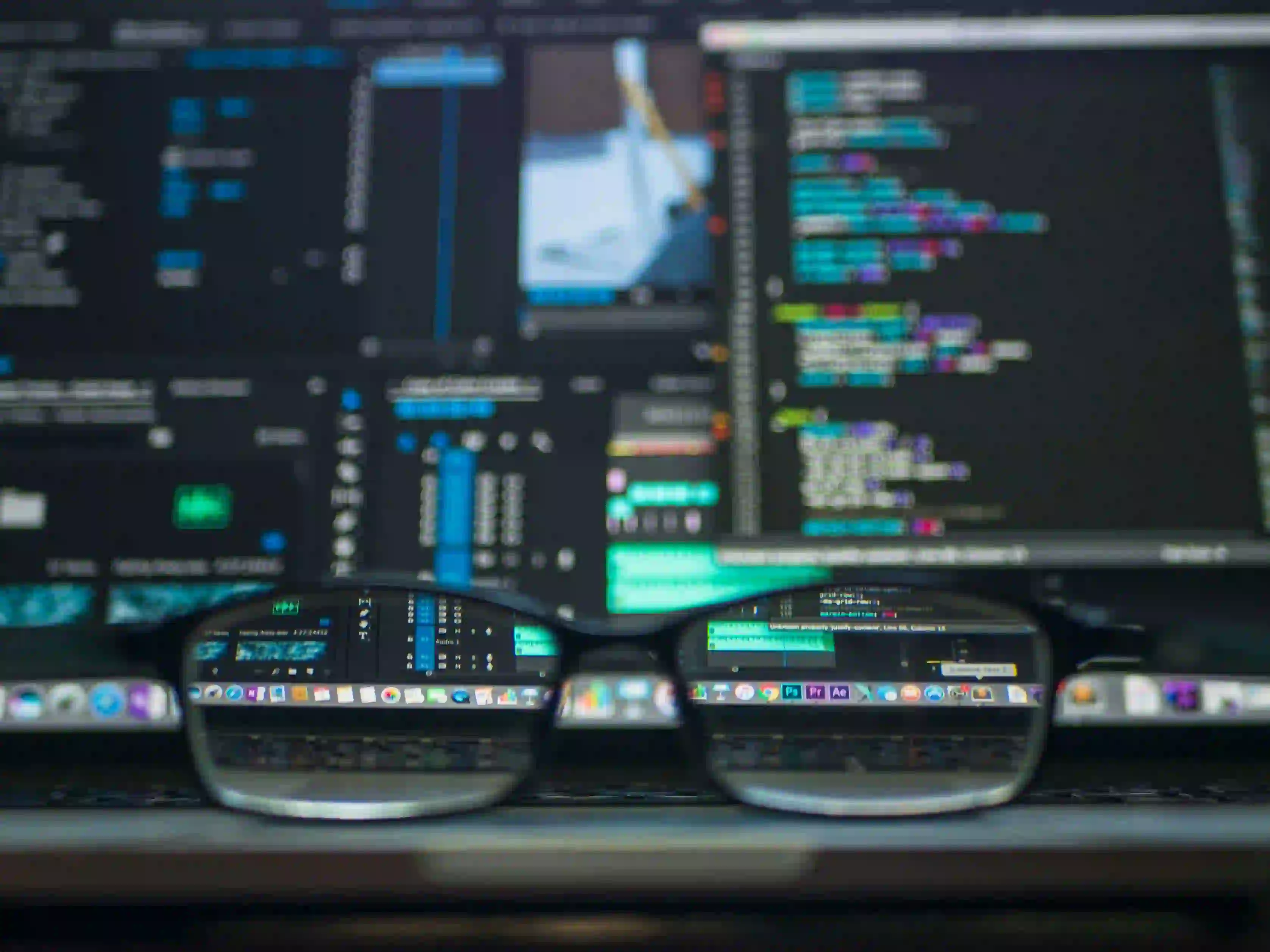
Understanding Common Hibernate Collection Cache Pitfalls
Hibernate is a powerful ORM (Object-Relational Mapping) library that simplifies database interactions within Java applications. Nevertheless, when it comes to using collections with Hibernate, developers often encounter pitfalls, particularly concerning caching. This blog post aims to illuminate these common issues, offering solutions and best practices to enhance the performance of your applications.
What is Caching in Hibernate?
Caching is a mechanism that helps reduce the number of database calls, thereby improving performance. Hibernate employs a first-level cache, which is session-scoped, and an optional second-level cache, which is session factory scoped. Utilizing caching effectively can lead to significantly reduced latency in data retrieval, especially for applications that require repetitive access to the same data.
Common Collection Cache Pitfalls
1. Lazy Loading vs. Eager Loading
Choosing between lazy loading and eager loading is crucial when designing data models in Hibernate.
- Lazy Loading: This means that the data is loaded only when it is accessed. While this is memory-efficient, it can lead to
LazyInitializationException
if the session is closed before the collection is accessed.
@Entity
public class User {
@OneToMany(mappedBy = "user", fetch = FetchType.LAZY)
private List<Order> orders;
}
In the example above, when the User
entity is fetched, the orders
collection is not loaded immediately. If you try to access orders
outside of an open session, it will throw an exception:
session.close();
user.getOrders(); // throws LazyInitializationException
- Eager Loading: This method loads the entire collection when the parent entity is retrieved.
@Entity
public class User {
@OneToMany(mappedBy = "user", fetch = FetchType.EAGER)
private List<Order> orders;
}
In this scenario, all orders
will be retrieved with the User
, preventing the exception. However, this can lead to inefficient memory usage, especially with large datasets.
Best Practice: Use lazy loading when dealing with large collections and eager loading for small, frequently used associations.
2. Using the Right Caching Strategy
Hibernate allows you to choose a caching strategy for your collections. Failing to configure these correctly can lead to stale data issues or unnecessary hits to the database.
- Read-Only Cache: Suitable for data that does not change often. In this case, you can use the
read-only
andnon-strict
cache strategy.
@Cache(usage = CacheConcurrencyStrategy.READ_ONLY)
@Entity
public class Product {
@Id
private Long id;
private String name;
}
- Read-Write Cache: Useful for data that may change. The
read-write
strategy allows Hibernate to manage the entity's states.
@Cache(usage = CacheConcurrencyStrategy.READ_WRITE)
@Entity
public class User {
@Id
private Long id;
private String username;
}
Best Practice: Be judicious in choosing the caching strategy based on the data's nature—volatile or stable.
3. Collections and Cache Size
When it comes to caching, one cannot overlook the impact of collection sizes. Large collections might slow down performance and lead to memory issues. Hibernate allows you to define the size of collections cached in the second-level cache.
@Cache(usage = CacheConcurrencyStrategy.READ_WRITE)
@Entity
public class Category {
@OneToMany(mappedBy = "category")
@Cache(size = 500)
private List<Product> products;
}
Best Practice: Aim for a balance; cache collections that are frequently accessed but beware of overwhelming the cache with large datasets.
4. Eviction Strategies
Eviction strategies determine how stale data is handled within your caches. It is essential to have a strategy that aligns with your application's overall architecture.
-
Automatic Eviction: With automatic eviction, stale items are removed based on time-to-live (TTL) settings.
-
Manual Eviction: In some scenarios, you may choose to clear specific caches actively. For instance, when entities are modified.
sessionFactory.getStatistics().clear();
Best Practice: Implement a robust eviction strategy, especially in data-heavy applications, to ensure performance remains optimal without serving outdated information.
5. Poorly Designed Collection Mappings
Another common pitfall lies in improperly defining collection mappings. Such issues can lead to high costs in data fetching and memory overheads.
For example, using a Bag
as a collection type can cause duplicates in the collection due to how Hibernate implements it. Instead, use a Set
or List
as appropriate.
@Entity
public class Customer {
@OneToMany(mappedBy = "customer")
private Set<Order> orders = new HashSet<>();
}
Best Practice: Utilize Set
for collections that require unique elements and List
for ordered collections without duplicates.
Summary
To summarize, when dealing with collections in Hibernate, pay attention to the pitfalls mentioned to enhance performance and prevent common issues.
- Understand the implications of lazy vs. eager loading.
- Choose the correct caching strategy.
- Be mindful of collection sizes.
- Implement effective eviction strategies.
- Design your collection mappings judiciously.
By adhering to these best practices, your Hibernate applications will not only perform efficiently but will also be easier to maintain and scale.
For more in-depth reading on Hibernate caching strategies, you can refer to Hibernate Caching Documentation. Additionally, consider exploring Java Persistence API (JPA) to deepen your understanding of ORM practices.
Code Repositories and Further Learning
For practical examples and test codes, check out my GitHub repository where I maintain various examples related to Hibernate and its functioning with collections: GitHub Repository
By integrating these insights into your Hibernate implementations, you can avoid common pitfalls and maximize the effectiveness of caching collections in your Java applications. Happy coding!