Bridging Java with JavaScript: Essential Packages to Know
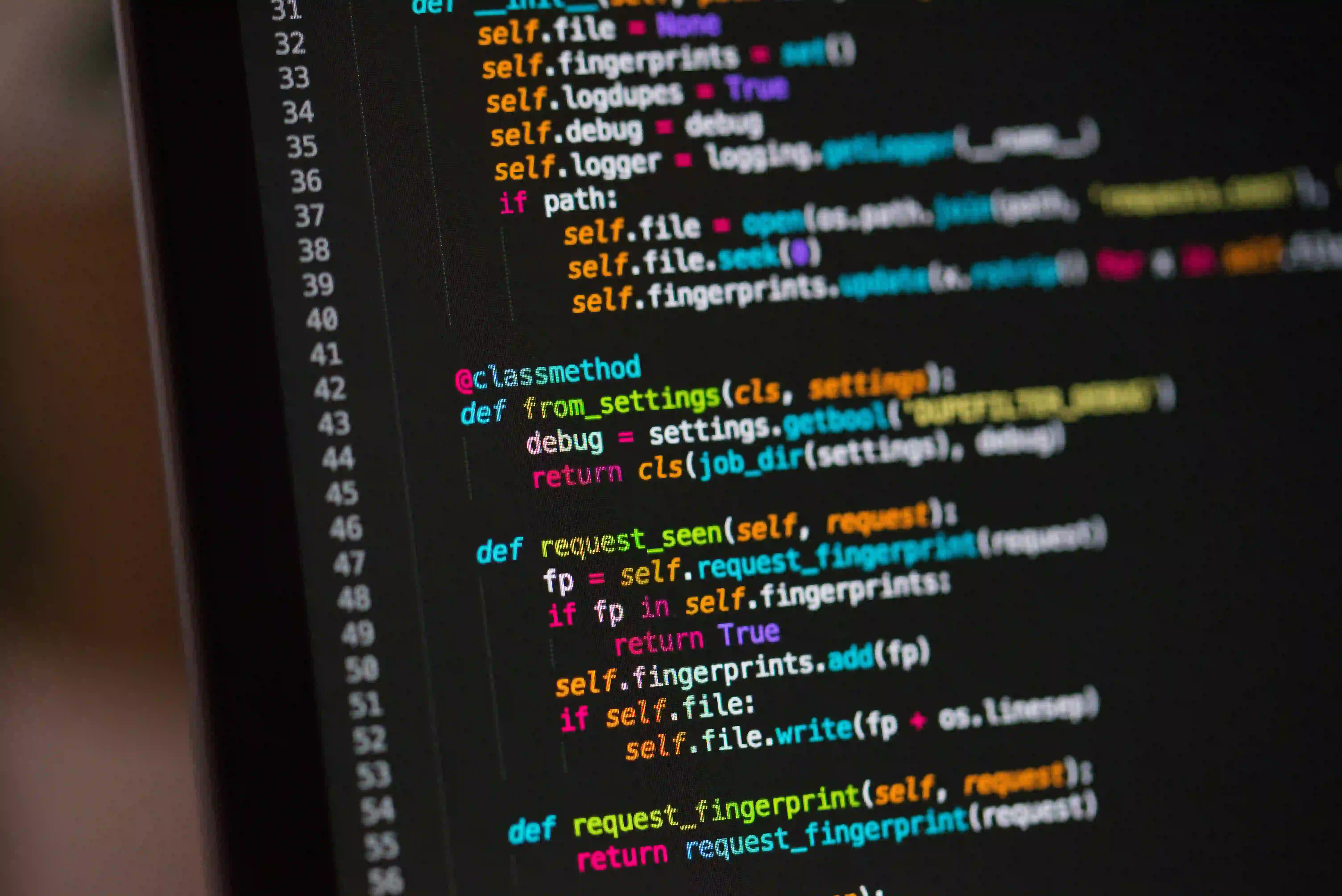
Bridging Java with JavaScript: Essential Packages to Know
In our increasingly interconnected world, the boundaries between programming languages are blurring. One of the most exciting developments in software development is the interplay between Java and JavaScript. While Java has been a staple in enterprise applications for decades, JavaScript has surged to the forefront in web development. This blog post will delve into some essential packages and methodologies that help developers bridge Java with JavaScript, enabling seamless interactions between the two languages.
Why Connect Java and JavaScript?
Java and JavaScript each have their strengths. Java offers robust performance, strong typing, and a vast ecosystem of libraries, making it perfect for backend systems. JavaScript, on the other hand, shines in creating dynamic user interfaces and handling asynchronous operations in the browser. By combining these two languages, developers can create powerful full-stack applications. This synergy enables:
- Rich user experiences delivered by JavaScript
- Strong backend capabilities facilitated by Java
- The ability to share code and capabilities across different layers of a tech stack
Key Technologies for Bridging Java and JavaScript
1. Spring Framework
The Spring Framework has become the backbone of Java enterprise applications. It offers comprehensive features that enhance Java's capabilities. Introducing JavaScript into the mix is simplified with Spring, especially with Spring Boot. Using Spring Boot enables embedded Tomcat servers and simplifies Java setups.
Example Usage:
@RestController
@RequestMapping("/api/")
public class HelloWorldController {
@GetMapping("hello")
public String hello() {
return "Hello from Java!";
}
}
This code snippet outlines a simple RESTful API using Spring. It exposes a /api/hello
endpoint that can be consumed by any front-end JavaScript framework (e.g., React, Angular).
2. Node.js with Java
Node.js has skyrocketed in popularity. You might wonder how Java and JavaScript interact in this ecosystem. Java can run alongside Node.js to create a more performant solution, especially when invoking Java's extensive libraries.
If you need to incorporate Java code into a Node application, consider using the node-java package. This package enables communication between Node.js and Java code.
Installation
npm install java
Example Usage:
const java = require('java');
// Setting Java classpath
java.classpath.push('path/to/your/java/classes');
const MyJavaClass = java.import('com.example.MyJavaClass');
MyJavaClass.sayHello((err, result) => {
if (err) {
console.error(err);
} else {
console.log(result); // Logs output from Java
}
});
In this example, we're integrating Java code into a Node.js application. The sayHello
method in MyJavaClass
needs to be defined in your Java class.
3. JHipster
JHipster is an application generator that merges Java and JavaScript ecosystems using Spring Boot for the backend and Angular or React for the frontend. This productivity tool is noteworthy for generating complete and modern web applications.
Key Features:
- Select between Angular and React for client-side frameworks
- Generates a full Microservices architecture
- Offers built-in support for various databases
Engaging with JHipster significantly reduces initial setup times, allowing developers to focus on building unique application features.
Communicating between Java and JavaScript
When connecting Java and JavaScript, the common practice involves REST APIs, enabling Java to serve as a backend service while JavaScript handles the client-side logic.
4. AJAX with Java Backend
Using AJAX to consume API endpoints created in Java is a simple yet robust way to connect the two languages.
Example AJAX Call:
fetch('http://localhost:8080/api/hello')
.then(response => response.text())
.then(data => console.log(data))
.catch(error => console.log('Error fetching data:', error));
The fetch
API makes an asynchronous request to the Java backend, which returns a string response that can be used on the front end.
5. GraalVM for Polyglot Applications
GraalVM enhances Java applications by allowing execution of JavaScript and other languages in the same runtime environment. This polyglot capability can be leveraged for various use cases, from microservices to serverless architectures.
Setting Up GraalVM:
gu install js
By installing the JavaScript component for GraalVM, you can execute JavaScript code directly within your Java application.
Example:
import org.graalvm.polyglot.*;
public class GraalVMExample {
public static void main(String[] args) {
try (Context context = Context.create()) {
context.eval("js", "console.log('Hello from JavaScript!')");
}
}
}
In this example, we leverage GraalVM to run a JavaScript snippet from within our Java application. This builds the foundation for truly hybrid applications.
Learning Resources
To dovetail your Java and JavaScript knowledge, you can explore the article titled "Top Essential Node.js Packages Every Developer Needs in 2023" available at infinitejs.com/posts/essential-node-js-packages-2023. This article provides insights into modern Node.js solutions that can enhance your JavaScript projects.
Closing the Chapter
The interplay between Java and JavaScript creates an endless array of opportunities for developers. By using the aforementioned technologies like Spring Framework, Node.js, JHipster, and GraalVM, you can write powerful applications that leverage the strengths of both languages.
As technology continues to evolve, adopting these best practices will ensure that your applications remain competitive and driven by robust architectures. Happy coding!