Centering Elements in JavaFX: A Developer's Guide
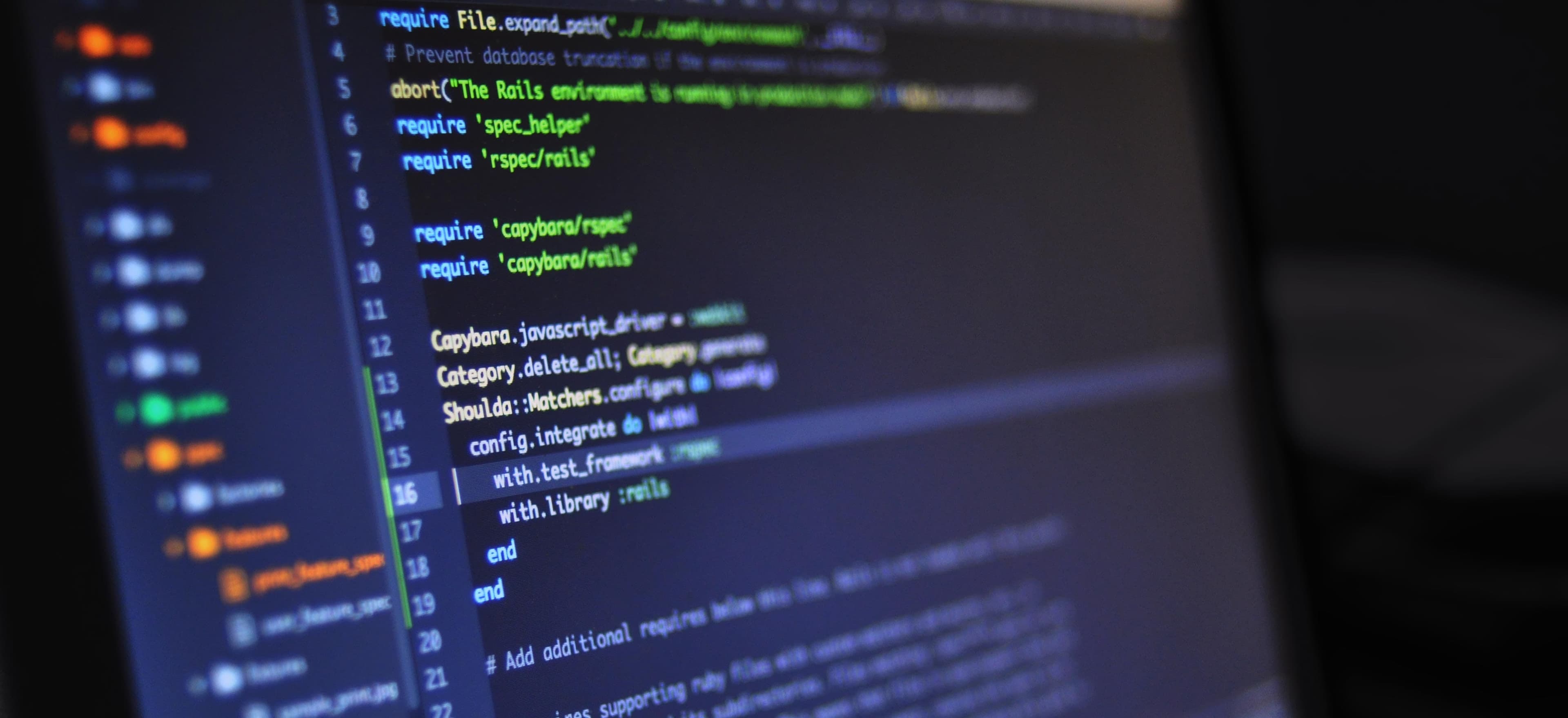
- Published on
Centering Elements in JavaFX: A Developer's Guide
JavaFX provides a powerful toolkit for building dynamic, rich Internet applications. One of the most critical aspects of designing user interfaces is the proper alignment and centering of elements. In this blog post, we will dive into various ways to center elements within JavaFX applications, leveraging the flexibility of layouts and properties that JavaFX offers.
Why Centering Matters
Centering elements contributes to not only visual aesthetics but also usability. An appropriately centered layout can enhance user experience by making applications look polished and functional. Just as the article "Mastering the Art of Centering a Div within a Div" emphasizes here, centering elements is an essential skill in web design; similar principles apply to desktop applications in JavaFX.
Understanding JavaFX Layouts
JavaFX provides several layout managers, each designed to handle specific alignment tasks. Here are some of the most commonly used ones:
- Pane: A basic layout that allows free positioning of elements.
- VBox: Arranges elements vertically.
- HBox: Arranges elements horizontally.
- GridPane: Allows positioning in a grid format.
- StackPane: Overlays elements on top of each other and allows centering of children.
Centering with StackPane
The StackPane
is perhaps the easiest way to center elements in JavaFX. It naturally centers its child nodes both vertically and horizontally.
Here’s how you can implement it:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class CenteringExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Click Me");
// Create a StackPane, which will center its content
StackPane stackPane = new StackPane();
stackPane.getChildren().add(button);
// Set up the scene with the StackPane
Scene scene = new Scene(stackPane, 300, 200);
primaryStage.setTitle("Centering Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why Choose StackPane?
Using StackPane
is simple and concise. The following lines are doing the heavy lifting:
StackPane stackPane = new StackPane();
stackPane.getChildren().add(button);
This code adds the button to the stack pane, instantly centering the button within the defined scene size.
More Centering Techniques with VBox and HBox
In cases where you need more control over the layout, VBox
and HBox
are great alternatives. They allow for vertical and horizontal arrangements and can also center their content.
Centering with VBox
Here’s how you can center elements in a VBox
:
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class CenteringVBoxExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button1 = new Button("Button 1");
Button button2 = new Button("Button 2");
VBox vbox = new VBox(10); // 10 pixels spacing between elements
vbox.getChildren().addAll(button1, button2);
// Align the VBox content to center
vbox.setAlignment(Pos.CENTER);
Scene scene = new Scene(vbox, 300, 200);
primaryStage.setTitle("Centering VBox Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
The Importance of setAlignment()
By calling vbox.setAlignment(Pos.CENTER)
, all children added to the VBox
will inherently be centered. This method demonstrates the neat functionality of layout controls, allowing for efficient UI design.
Centering with HBox
Similarly, you might want to place elements horizontally centered within an HBox
. This is just as straightforward:
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.HBox;
import javafx.stage.Stage;
public class CenteringHBoxExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button1 = new Button("Button 1");
Button button2 = new Button("Button 2");
HBox hbox = new HBox(10); // 10 pixels spacing between elements
hbox.getChildren().addAll(button1, button2);
// Align the HBox content to center
hbox.setAlignment(Pos.CENTER);
Scene scene = new Scene(hbox, 300, 200);
primaryStage.setTitle("Centering HBox Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Takeaway
The setAlignment(Pos.CENTER)
method proves versatile across different layouts, providing a robust solution for centered designs. Centering elements in JavaFX can be accomplished effortlessly, allowing developers to focus on functionality.
Centering Children in GridPane
Although less commonly used for centering, a GridPane
can also allow for centered elements through proper configuration. Here’s a quick example:
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.GridPane;
import javafx.stage.Stage;
public class CenteringGridPaneExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Centered Button");
GridPane gridPane = new GridPane();
gridPane.setPadding(new Insets(10, 10, 10, 10));
gridPane.setVgap(10);
gridPane.setHgap(10);
// Column and Row Index set to 0, which is centered by default
gridPane.add(button, 0, 0);
gridPane.setAlignment(Pos.CENTER);
Scene scene = new Scene(gridPane, 300, 200);
primaryStage.setTitle("Centering GridPane Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why GridPane?
The GridPane
provides a structured layout that can be useful when you have multiple elements needing to be positioned and aligned in a specific grid format. By using setAlignment(Pos.CENTER)
, even with a structured layout, you can achieve a centered design.
Final Considerations
Centering elements in JavaFX is an essential skill for any developer wishing to create visually appealing user interfaces. The versatility of StackPane
, VBox
, HBox
, and GridPane
provides numerous ways to achieve well-aligned layouts effectively.
Proper knowledge of JavaFX layouts can transform your application's UI from mediocre to professional-level aesthetics. For further insights on similar topics, be sure to explore more design principles like those suggested in the article titled "Mastering the Art of Centering a Div within a Div" at infinitejs.com/posts/mastering-centering-div.
Happy coding and UI designing in JavaFX!
Checkout our other articles